licenses
sequencelengths 1
3
| version
stringclasses 677
values | tree_hash
stringlengths 40
40
| path
stringclasses 1
value | type
stringclasses 2
values | size
stringlengths 2
8
| text
stringlengths 25
67.1M
| package_name
stringlengths 2
41
| repo
stringlengths 33
86
|
---|---|---|---|---|---|---|---|---|
[
"MIT"
] | 0.1.0 | 50e804aa999e452ce4bf5edc50493838bd744e09 | code | 2365 | # try include("sparse_eigen_test.jl") catch end
# try include("./test/sparse_eigen_test.jl") catch end
# test_native_eigen()
import LinearAlgebra
using MatrixCompletion.Utilities.FastEigen
using Test
function create_symmetric_matrix(n)
a = rand(n,n) * 5
return a+a'
end
function correct_output_sparseeigen(input,k)
eigen_dcp = LinearAlgebra.eigen(input);
eigen_val = eigen_dcp.values;
eigen_vec = eigen_dcp.vectors;
first_k_idx = Base.sortperm(eigen_val,rev=true)[1:k];
return eigen_val[first_k_idx],eigen_vec[:,first_k_idx];
end
function project(v,e)
return e * LinearAlgebra.Diagonal(v) * e';
end
function test_native_eigen(;n = 200, nev = 20)
input = create_symmetric_matrix(n);
@time λ, X = eigs(NativeEigen(), input ;nev = nev);
λ₀, X₀ = correct_output_sparseeigen(input, nev);
p = project(λ, X)
p₀ = project(λ₀, X₀)
@test LinearAlgebra.norm(p - p₀) < 0.01
end
function test_krylov(;n = 200, nev = 20)
a = create_symmetric_matrix(n) * 5
@info("doing krylov eigen")
@time λ, X = eigs(KrylovMethods(), a, nev = nev)
@info("doing full eigen")
@time λ₀, X₀ = correct_output_sparseeigen(a, nev)
p = project(λ, X)
p₀ = project(λ₀, X₀)
@test LinearAlgebra.norm(p - p₀)^2 / LinearAlgebra.norm(p₀)^2 < 0.01
end
function test_arpack(;n = 200, nev = 20)
a = create_symmetric_matrix(n) * 5
@info("doing Arpack eigen")
# @time λ, X = eigs(ARPACK(), a, nev = nev)
@info("doing full eigen")
@time λ₀, X₀ = correct_output_sparseeigen(a, nev)
p = project(λ, X)
p₀ = project(λ₀, X₀)
@test LinearAlgebra.norm(p - p₀)^2 / LinearAlgebra.norm(p₀)^2 < 0.01
end
test_arpack(n = 4000, nev = 1000)
function test_lobpcg(;dim= 2000,nev=20,repeat=5)
input = create_symmetric_matrix(dim);
for i = 1:repeat
@time begin
λ, X = eigs(NativeLOBPCG(), input; nev=nev)
p1 = get_projection(λ, X)
end
@time begin
v0, e0 = correct_output_sparseeigen(input, 20)
p2 = get_projection(v0, e0)
end
@test LinearAlgebra.norm(p1 - p2) < 0.01
end
end
@testset "$(format("Sparse Eigen: KrylovKit Wrapper"))" begin
let
for i in 1:10
test_krylov(n = 2000, nev = 20)
end
end
end
# @testset "$(format("Sparse Eigen: NativeEigen Wrapper"))" begin
# let
# for i in 1:10
# test_native_eigen(n = 2000, nev = 20)
# end
# end
# end
| MatrixCompletion | https://github.com/jasonsun0310/MatrixCompletion.jl.git |
|
[
"MIT"
] | 0.1.0 | 50e804aa999e452ce4bf5edc50493838bd744e09 | code | 386 | @info "Visualization: [PoissonMatrix (5x5) (λ=5)]"
display(PoissonMatrix(5,5,rank=3,λ=5))
@info "Visualization: [GaussianMatrix (5x5) (μ=0,σ=1)]"
display(GaussianMatrix(5,5,rank=3,σ=1,μ=0))
@info "Visualization: [BinomialMatrix (5x5) (p=0.5)]"
display(BernoulliMatrix(5,5,rank=3,p=0.5))
@info "Visualization: [GammaMatrix (5,5) (α=5,θ=0.5)]"
display(GammaMatrix(5,5,rank=3,α=5,θ=0.5))
| MatrixCompletion | https://github.com/jasonsun0310/MatrixCompletion.jl.git |
|
[
"MIT"
] | 0.1.0 | 50e804aa999e452ce4bf5edc50493838bd744e09 | docs | 7479 | # MatrixCompletion.jl
MatrixCompletion.jl is Julia package for completion low rank matrices with missing entries. The problem of matrix completion has a wide range of applications, such as collaborative filtering, system identification, data imputation, and Internet of things localization.
MatrixCompletion.jl by default uses algorithm proposed in Robust Matrix Completion with Mix Data Types, see [paper](), which is a convex algorithm that minimizes the joint likelihood reguluaized by both nuclear norm and max norm. It can complete low rank matrices with hetergenous data (columnwise or row wise) data types, which are in the exponential family such as:
* Bernoulli (binary data)
* Poisson (count data)
* Gaussian (continuous data)
* Negative Binomial (count)
* Gamma (skewed continuous data)
## Installation
To install, simply type
```julia
Pkg.add("MatrixCompletion)
```
in the Julia REPL.
## Minimal Working Example
For example, the following simulation snippet demonstrates how to complete a manually generated mixed-type low rank matrix.
```
using MatrixCompletion
import Distributions, Random
input_rank = 20
truth_matrix = rand([(FixedRankMatrix(Distributions.Gaussian(0, 1), rank = input_rank), 500, 100),
(FixedRankMatrix(Distributions.Bernoulli(0.5), rank = input_rank), 500, 100),
(FixedRankMatrix(Distributions.Gamma(10, 0.5), rank = input_rank), 500, 100),
(FixedRankMatrix(Distributions.Poisson(5), rank = input_rank), 500, 100),
(FixedRankMatrix(Distributions.NegativeBinomial(6, 0.8), rank = input_rank), 500, 100)])
sample_model = provide(Sampler{BernoulliModel}(), rate = 80 / 100)
input_matrix = sample_model.draw(truth_matrix)
manual_type_matrix = Array{Symbol}(undef, 500, 500)
manual_type_matrix[:, 1:100] .= :Gaussian
manual_type_matrix[:, 101:200] .= :Bernoulli
manual_type_matrix[:, 201:300] .= :Gamma
manual_type_matrix[:, 301:400] .= :Poisson
manual_type_matrix[:, 401:500] .= :NegativeBinomial
user_input_estimators = Dict(:NegativeBinomial=> Dict(:r=>6, :p=>0.8))
completed_matrix, type_tracker, tracker = complete(A = input_matrix,
maxiter = 200,
ρ = 0.3,
use_autodiff = false,
gd_iter = 3,
debug_mode = false,
user_input_estimators = user_input_estimators,
project_rank = input_rank * 10,
io = io,
type_assignment = manual_type_matrix)
predicted_matrix = predict(MatrixCompletionModel(),
completed_matrix = completed_matrix,
type_tracker = type_tracker,
estimators = user_input_estimators)
summary_object = summary(MatrixCompletionModel(),
predicted_matrix = predicted_matrix,
truth_matrix = truth_matrix,
type_tracker = type_tracker,
tracker = tracker)
```
## Automatic data type detection
We note that in the MWE, the data type of the observed matrix was entered manually. Although doing so in most cases guarantees maximum recovery rate, in reality it is often unknown that what are the exact distributions of the underlying data. To address this issue, we provided an API that allows the algorithm to automatically detect the best fitting distributed within the supported range and after doing so, also acquire the MLE of the corresponding parameters. Traditional goodness-and-fit often has less power when the input data size are large. To address this problem, we adopted a different approach combining a simple trivial decision tree and comparing the empirical distribution to its exponential family candidates in the frequency domain, i.e. MGF. In order to use automatic data type detection, one simply need to not provide the `user_input_estimators` parameter in the `complete` function.
**Note.** While useful, according to preliminary simulations results, using automatic data type detection often results in recovery rate loss when the underlying data is continuous and strongly skewed.
## Simulation Utilities
### Different Fast Eigen Solvers
Due to potential unforseen numerical instabilitis, multiple fast eigen libraries are shipped with MatrixCompletion.jl. Arpack is tested to be the fastest when using the MKL patched Julia 1.2. By default, this is not enabled due to licensing issues; and a Julia native Lancozs library is used by default.
### Sampling Schemes
MatrixCompletion.jl supports two sampling schemes, Uniform Sampling and Bernoulli Sampling. Researchers can use `x = provide(Sampler{BernoulliModel}(), rate = 80 / 100)` to get an instance of the corresponding sampler and bind it to `x`, and use `x.draw(M)` to draw a partially observed matrix from full input matrix `M`.
### Random Fixed Rank Matrix Generator
MatrixCompletion.jl also provides a random fixed rank matrix generator that supports generating low rank matrix of target rank and specified (combination of) distributions. For example,
```julia
input_rank = 10
M = rand([(FixedRankMatrix(Distributions.Gaussian(0, 1), rank = input_rank), 500, 100),
(FixedRankMatrix(Distributions.Bernoulli(0.5), rank = input_rank), 500, 100),
(FixedRankMatrix(Distributions.Gamma(10, 0.5), rank = input_rank), 500, 100),
(FixedRankMatrix(Distributions.Poisson(5), rank = input_rank), 500, 100),
(FixedRankMatrix(Distributions.NegativeBinomial(6, 0.8), rank = input_rank), 500, 100)])
```
generates a 500*500 matrix `M` with target rank 50, with the five listed distribution divided according to the dimension parameters.
# On going work
## GUI front end
A Gui front end is to be created using Genie to
* provide interactive plotting of convergence path and regularization path.
* provide a simple and easy to use interface for user to try to apply the algorithm to pictures of users' choice.
## More Algorithms
MatrixCompletion.jl aims to be the most comprehensive matrix completion algorithm library in Julia consisting of both popular non-convex and convex algorithms proposed in the past decade, including (on going work):
* LMaFit: Low-Rank Matrix Fitting [(Wen et al. 2012)](http://link.springer.com/article/10.1007%2Fs12532-012-0044-1) [website](http://lmafit.blogs.rice.edu/)
* OptSpace: Matrix Completion from Noisy Entries [(Keshavan et al. 2009)](http://arxiv.org/pdf/0906.2027v1.pdf) [website](http://web.engr.illinois.edu/~swoh/software/optspace/code.html)
* OR1MP: Orthogonal rank-one matrix pursuit for low rank matrix completion [(Wang et al. 2015)](https://arxiv.org/abs/1404.1377)
* SVT: A singular value thresholding algorithm for matrix completion [(Cai et al. 2008)](http://arxiv.org/pdf/0810.3286.pdf) [website](http://svt.stanford.edu/)
| MatrixCompletion | https://github.com/jasonsun0310/MatrixCompletion.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 4147 | # Note that this script can accept some limited command-line arguments, run
# `julia build_tarballs.jl --help` to see a usage message.
using BinaryBuilder
name = "GR"
version = v"0.73.7"
# Collection of sources required to complete build
sources = [
GitSource("https://github.com/sciapp/gr.git", "a8f4f0b867ad8e40e9a927d72e4ada571fdca461"),
FileSource("https://github.com/sciapp/gr/releases/download/v$version/gr-$version.js",
"89a114eac7e96f0a32441da01e1929068aeaa286ad2ea22f923420cff8d4e2a4", "gr.js"),
ArchiveSource("https://github.com/phracker/MacOSX-SDKs/releases/download/10.15/MacOSX10.14.sdk.tar.xz",
"0f03869f72df8705b832910517b47dd5b79eb4e160512602f593ed243b28715f")
]
# Bash recipe for building across all platforms
script = raw"""
cd $WORKSPACE/srcdir/gr
update_configure_scripts
make -C 3rdparty/qhull -j${nproc}
if [[ $target == *"mingw"* ]]; then
winflags=-DCMAKE_C_FLAGS="-D_WIN32_WINNT=0x0f00"
tifflags=-DTIFF_LIBRARY=${libdir}/libtiff-6.dll
else
tifflags=-DTIFF_LIBRARY=${libdir}/libtiff.${dlext}
fi
if [[ "${target}" == x86_64-apple-darwin* ]]; then
apple_sdk_root=$WORKSPACE/srcdir/MacOSX10.14.sdk
sed -i "s!/opt/x86_64-apple-darwin14/x86_64-apple-darwin14/sys-root!$apple_sdk_root!" $CMAKE_TARGET_TOOLCHAIN
export MACOSX_DEPLOYMENT_TARGET=10.14
fi
if [[ "${target}" == *apple* ]]; then
make -C 3rdparty/zeromq ZEROMQ_EXTRA_CONFIGURE_FLAGS="--host=${target}"
fi
if [[ "${target}" == arm-* ]]; then
export CXXFLAGS="-Wl,-rpath-link,/opt/${target}/${target}/lib"
fi
mkdir build
cd build
cmake $winflags -DCMAKE_INSTALL_PREFIX=$prefix -DCMAKE_FIND_ROOT_PATH=$prefix -DCMAKE_TOOLCHAIN_FILE=${CMAKE_TARGET_TOOLCHAIN} -DGR_USE_BUNDLED_LIBRARIES=ON $tifflags -DCMAKE_BUILD_TYPE=Release ..
VERBOSE=ON cmake --build . --config Release --target install -- -j${nproc}
cp ../../gr.js ${libdir}/
install_license $WORKSPACE/srcdir/gr/LICENSE.md
if [[ $target == *"apple-darwin"* ]]; then
cd ${bindir}
ln -s ../Applications/gksqt.app/Contents/MacOS/gksqt ./
ln -s ../Applications/grplot.app/Contents/MacOS/grplot ./
ln -s ../Applications/GKSTerm.app/Contents/MacOS/GKSTerm ./
fi
"""
# These are the platforms we will build for by default, unless further
# platforms are passed in on the command line
platforms = [
Platform("armv7l", "linux"; libc="glibc"),
Platform("aarch64", "linux"; libc="glibc"),
Platform("x86_64", "linux"; libc="glibc"),
Platform("i686", "linux"; libc="glibc"),
Platform("powerpc64le", "linux"; libc="glibc"),
Platform("x86_64", "windows"),
Platform("i686", "windows"),
Platform("x86_64", "macos"),
Platform("aarch64", "macos"),
Platform("x86_64", "freebsd"),
]
platforms = expand_cxxstring_abis(platforms)
# The products that we will ensure are always built
products = [
LibraryProduct("libGR", :libGR, dont_dlopen=true),
LibraryProduct("libGR3", :libGR3, dont_dlopen=true),
LibraryProduct("libGRM", :libGRM, dont_dlopen=true),
LibraryProduct("libGKS", :libGKS, dont_dlopen=true),
ExecutableProduct("gksqt", :gksqt),
ExecutableProduct("grplot", :grplot),
]
# Dependencies that must be installed before this package can be built
dependencies = [
Dependency("Bzip2_jll"; compat="1.0.8"),
Dependency("Cairo_jll"; compat="1.16.1"),
Dependency("FFMPEG_jll"),
Dependency("Fontconfig_jll"),
Dependency("FreeType2_jll"; compat="2.10.4"),
Dependency("GLFW_jll"),
Dependency("JpegTurbo_jll"),
Dependency("libpng_jll"),
Dependency("Libtiff_jll"; compat="~4.5.1"),
Dependency("Pixman_jll"),
HostBuildDependency("Qt6Base_jll"),
Dependency("Qt6Base_jll"; compat="~6.7.1"), # Never allow upgrading more than the minor version without recompilation
BuildDependency("Xorg_libX11_jll"),
BuildDependency("Xorg_xproto_jll"),
Dependency("Zlib_jll"),
]
# Build the tarballs, and possibly a `build.jl` as well.
# GCC version 10 because of Qt6.7
build_tarballs(ARGS, name, version, sources, script, platforms, products, dependencies;
preferred_gcc_version = v"10", julia_compat="1.6")
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 110 | using GR
inline("iterm", false)
x = collect(0:0.01:2*pi)
for i = 1:100
plot(x, sin.(x .+ i / 10.0))
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1464 | # Compare line drawing performance of Matplotlib vs. GR
#
# The results depend strongly on which backend is used in GR and/or Matplotlib.
# Depending on the selection, however, improvements by a factor of 50 are possible.
# The best results can be achieved with the Qt driver (gksqt).
#
# The increase in drawing speed through the GR Matplotlib backend is comparatively
# small, since most of the time is spent in Matplotlib/Python.
ENV["JULIA_PYTHONCALL_EXE"] = "/usr/local/bin/python3"
ENV["GKSwstype"] = "gksqt"
ENV["MPLBACKEND"]="module://gr.matplotlib.backend_gr"
import PythonPlot
import GR
function mpl()
x = collect(0:0.01:2*pi)
PythonPlot.plot(x, sin.(x))
PythonPlot.ion()
t = time_ns()
for i = 1:100
PythonPlot.cla()
PythonPlot.plot(x .+ i / 10.0, sin.(x .+ i / 10.0))
if haskey(ENV, "MPLBACKEND")
PythonPlot.show()
else
PythonPlot.pause(0.0001)
end
end
fps = round(Int64, 100 / (1e-9 * (time_ns() - t)))
end
function gr()
x = collect(0:0.01:2*pi)
GR.plot(x, sin.(x))
t = time_ns()
for i = 1:100
GR.plot(x .+ i / 10.0, sin.(x .+ i / 10.0))
end
fps = round(Int64, 100 / (1e-9 * (time_ns() - t)))
end
using Printf
for i in 1:10
fps_mpl = mpl()
fps_gr = gr()
speedup = float(fps_gr) / fps_mpl
@printf("MPL: %4d fps GR: %4d fps => speedup: %6.1f\r",
fps_mpl, fps_gr, speedup)
end
@printf("\n")
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 173 | ENV["GKS_WSTYPE"] = "mov" # or "webm", "mp4"
ENV["GKS_VIDEO_OPTS"] = "600x450@25@2x"
using GR
x = LinRange(0, 2π, 200)
for i = 0:100
plot(x, sin.(x .+ i / 10.0))
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 190 | using GR
using SpecialFunctions
x = 0:0.1:20
j0, j1, j2, j3 = [besselj.(order, x) for order in 0:3]
plot(x, j0, x, j1, x, j2, x, j3,
labels=["J_0", "J_1", "J_2", "J_3"], location=11)
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 827 | #!/usr/bin/env julia
import GR
const gr3 = GR.gr3
function draw(mesh::Cint)
gr3.clear()
gr3.drawmesh(mesh, 1, (0,0,0), (0,0,1), (0,1,0), (1,1,1), (1,1,1))
GR.clearws()
gr3.drawimage(0, 1, 0, 1, 500, 500, gr3.DRAWABLE_GKS)
GR.updatews()
end
data = open(stream -> read!(stream, Array{UInt16}(undef, 93, 64, 64)), "mri.raw")
data = min.(data, 2000) / 2000.0 * typemax(UInt16)
data = convert(Array{UInt16, 3}, floor.(data))
data = permutedims(data, [3, 2, 1])
GR.setviewport(0, 1, 0, 1)
gr3.cameralookat(-3, 2, -2, 0, 0, 0, 0, 0, -1)
mesh = gr3.createisosurfacemesh(data, (2.0/63, 2.0/63, 2.0/92), (-1.0, -1.0, -1.0), 40000)
GR.setcolormap(1)
gr3.setlightsources(2, [0, -1, 1, 1, -1, 1], [0.45, 0.7, 0.9, 0.93, 0.91, 0.2])
directions, colors = gr3.getlightsources(2)
@show directions, colors
draw(mesh)
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1747 | import GLFW
import GR
const gr3 = GR.gr3
function display()
steps = (1.0 / size(data)[1], 1.0 / size(data)[2], 1.0 / size(data)[3])
offsets = (-0.5, -0.5, -0.5)
mesh = gr3.createisosurfacemesh(data, steps, offsets, isolevel)
gr3.drawmesh(mesh, 1, (0, 0, 0), (0, 0, 1), (0, 1, 0), (1, 1, 1), (1, 1, 1))
r = 1.5
gr3.cameralookat(center[1], center[2], center[3], 0, 0, 0, up[1], up[2], up[3])
gr3.drawimage(0.0, w, 0.0, h, width, height, gr3.DRAWABLE_OPENGL)
GLFW.SwapBuffers(window)
gr3.deletemesh(mesh)
gr3.clear()
end
function spherical_to_cartesian(r, theta, phi)
x = r * sin.(theta) * cos.(phi)
y = r * sin.(theta) * sin.(phi)
z = r * cos.(theta)
return (x, y, z)
end
function cursor(motion, x, y)
global center, up
global isolevel
if GLFW.GetMouseButton(window, GLFW.MOUSE_BUTTON_1)
center = spherical_to_cartesian(-2, pi * y / height + pi/2, pi * x / width)
up = spherical_to_cartesian(1, pi * y / height + pi, pi * x / width)
elseif GLFW.GetMouseButton(window, GLFW.MOUSE_BUTTON_2)
if y >= 0
isolevel = round(Int, 255 * y / height)
end
end
display()
end
ENV["GR3_USE_OPENGL"] = "true"
f = open("brain.bin", "r")
data = Vector{UInt16}(undef, 5120000)
data = reshape(read!(f, data), 200, 160, 160)
close(f)
width = height = 500
isolevel = 128
center = (0., 0., 2.)
up = (-1., 0., 0.)
monitor = GLFW.GetPrimaryMonitor()
xscale, yscale = GLFW.GetMonitorContentScale(monitor)
w = xscale * width
h = yscale * height
window = GLFW.CreateWindow(width, height, "MRI example")
GLFW.MakeContextCurrent(window)
GLFW.SetCursorPosCallback(window, cursor)
gr3.setbackgroundcolor(1, 1, 1, 0)
while !GLFW.WindowShouldClose(window)
GLFW.PollEvents()
end
GLFW.DestroyWindow(window)
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 446 | using GR
using LaTeXStrings
formula = L"- \frac{{\hbar ^2}}{{2m}}\frac{{\partial ^2 \psi (x,t)}}{{\partial x^2 }} + U(x)\psi (x,t) = i\hbar \frac{{\partial \psi (x,t)}}{{\partial t}}"
selntran(0)
settextfontprec(232, 3)
settextalign(2, 3)
setbordercolorind(6)
mathtex(0.5, 0.5, formula)
tbx, tby = inqmathtex(0.5, 0.5, formula)
Δx = (tbx[2] - tbx[1]) / √2
Δy = (tby[3] - tby[1]) / √2
path([0.5+Δx, Δx, 2π], [0.5, Δy, 0], "MAs");
updatews()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1134 | using GR
const maps=["uniform", "temperature", "grayscale", "glowing", "rainbowlike", "geologic", "greenscale", "cyanscale", "bluescale", "magentascale", "redscale", "flame", "brownscale", "pilatus", "autumn", "bone", "cool", "copper", "gray", "hot", "hsv", "jet", "pink", "spectral", "spring", "summer", "winter", "gist_earth", "gist_heat", "gist_ncar", "gist_rainbow", "gist_stern", "afmhot", "brg", "bwr", "coolwarm", "CMRmap", "cubehelix", "gnuplot", "gnuplot2", "ocean", "rainbow", "seismic", "terrain", "viridis", "inferno", "plasma", "magma"]
function main()
a = round.(Int32,LinRange(1000,1255,256))
xl = 0
setwsviewport(0, 0.25, 0, 0.125)
setwswindow(0, 1, 0, 0.5)
setviewport(0.05, 0.95, 0.025, 0.475)
setcharheight(0.010)
setcharup(-1,0)
settextcolorind(255)
for cmap in 0:47
setcolormap(cmap)
xr = (cmap + 1) / 48.0
cellarray(xl+0.002, xr-0.002, 0.2, 1, 1, 256, a)
settextalign(1,3)
text(0.04 + xr * 0.9, 0.48, string(cmap))
settextalign(3,3)
text(0.04 + xr * 0.9, 0.1, string(maps[cmap+1]))
xl = xr
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1157 | using GR
function points_from_image(img, npts)
w, h = size(img)
xpts = Float64[]
ypts = Float64[]
cols = Int[]
for i in 1:npts
x = rand() * w
y = rand() * h
c = img[Int64(floor(x)) + 1, Int64(floor(h-y)) + 1]
r = ( c & 0xff) / 255.0
g = ((c >> 8 ) & 0xff) / 255.0
b = ((c >> 16) & 0xff) / 255.0
if 0.2989 * r + 0.5870 * g + 0.1140 * b > 0.8
if rand() < 0.1
push!(xpts, x)
push!(ypts, y)
push!(cols, 1)
end
continue
end
push!(xpts, x)
push!(ypts, y)
push!(cols, inqcolorfromrgb(r, g, b))
end
xpts, ypts, cols
end
w, h, img = readimage("julia_logo.png")
x, y, cols = points_from_image(img, 30_000)
setwsviewport(0, 0.24, 0, 0.16)
setwswindow(0, 1, 0, 2/3)
setviewport(0, 1, 0, 2/3)
setwindow(0, w, 0, h)
setmarkersize(2/3)
setmarkertype(GR.MARKERTYPE_SOLID_CIRCLE)
settransparency(0.5)
polymarker(x, y)
n, tri = delaunay(x, y)
for i in 1:n
if all(cols[tri[i,:]] .!= 1)
settransparency(0.5)
setfillcolorind(cols[tri[i,1]])
else
settransparency(0.1)
setfillcolorind(1)
end
fillarea(x[tri[i,:]], y[tri[i,:]])
end
updatews()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 2180 | #!/usr/bin/env julia
import GR
const g = 9.8 # gravitational constant
function main()
function rk4(x, h, y, f)
k1 = h * f(x, y)
k2 = h * f(x + 0.5 * h, y + 0.5 * k1)
k3 = h * f(x + 0.5 * h, y + 0.5 * k2)
k4 = h * f(x + h, y + k3)
x + h, y + (k1 + 2 * (k2 + k3) + k4) / 6.0
end
function derivs(t, state)
# The following derivation is from:
# http://scienceworld.wolfram.com/physics/DoublePendulum.html
t1, w1, t2, w2 = state
a = (m1 + m2) * l1
b = m2 * l2 * cos(t1 - t2)
c = m2 * l1 * cos(t1 - t2)
d = m2 * l2
e = -m2 * l2 * w2^2 * sin(t1 - t2) - g * (m1 + m2) * sin(t1)
f = m2 * l1 * w1^2 * sin(t1 - t2) - m2 * g * sin(t2)
[w1, (e*d-b*f) / (a*d-c*b), w2, (a*f-c*e) / (a*d-c*b)]
end
function pendulum(theta, length, mass)
l = length[1] + length[2]
GR.clearws()
GR.setviewport(0, 1, 0, 1)
GR.setwindow(-l, l, -l, l)
GR.setmarkertype(GR.MARKERTYPE_SOLID_CIRCLE)
GR.setmarkercolorind(86)
GR.setborderwidth(0)
pivot = [0, 0.775] # draw pivot point
GR.fillarea([-0.2, 0.2, 0.2, -0.2], [0.75, 0.75, 0.8, 0.8])
for i in 1:2
x = [pivot[1], pivot[1] + sin(theta[i]) * length[i]]
y = [pivot[2], pivot[2] - cos(theta[i]) * length[i]]
GR.polyline(x, y) # draw rod
GR.setmarkersize(3 * mass[i])
GR.polymarker([x[2]], [y[2]]) # draw bob
pivot = [x[2], y[2]]
end
GR.updatews()
end
l1 = 1.2 # length of rods
l2 = 1.0
m1 = 1.0 # weights of bobs
m2 = 1.5
t1 = 100.0 # inintial angles
t2 = -20.0
w1 = 0.0
w2 = 0.0
t = 1.0
dt = 0.04
state = [t1, w1, t2, w2] * pi / 180
now = start = time_ns()
while t < 30
t, state = rk4(t, dt, state, derivs)
t1, w1, t2, w2 = state
pendulum([t1, t2], [l1, l2], [m1, m2])
now = (time_ns() - start) / 1000000000
if t > now
sleep(t - now)
end
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 2494 | #!/usr/bin/env julia
import GR
const gr3 = GR.gr3
const g = 9.8 # gravitational constant
function rk4(x, h, y, f)
k1 = h * f(x, y)
k2 = h * f(x + 0.5 * h, y + 0.5 * k1)
k3 = h * f(x + 0.5 * h, y + 0.5 * k2)
k4 = h * f(x + h, y + k3)
return x + h, y + (k1 + 2 * (k2 + k3) + k4) / 6.0
end
function double_pendulum(theta, length, mass)
GR.clearws()
GR.setviewport(0, 1, 0, 1)
direction = zeros(3, 2)
position = zeros(3, 3)
for i=1:2
direction[:,i] = [ sin(theta[i]) * length[i] * 2,
-cos(theta[i]) * length[i] * 2, 0]
position[:,i+1] = position[:,i] + direction[:,i]
end
gr3.clear()
# draw pivot point
gr3.drawcylindermesh(1, [0,0.2,0], [0,1,0], [0.4,0.4,0.4], [0.4], [0.05])
gr3.drawcylindermesh(1, [0,0.2,0], [0,-1,0], [0.4,0.4,0.4], [0.05], [0.2])
gr3.drawspheremesh(1, [0,0,0], [0.4,0.4,0.4], [0.05])
# draw rods
gr3.drawcylindermesh(2, position, direction,
[0.6 for i=1:6], [0.05, 0.05], length * 2)
# draw bobs
gr3.drawspheremesh(2, position[:,2:3], [1 for i=1:6], mass * 0.2)
gr3.drawimage(0, 1, 0, 1, 500, 500, gr3.DRAWABLE_GKS)
GR.updatews()
end
function main()
function derivs(t, state)
# The following derivation is from:
# http://scienceworld.wolfram.com/physics/DoublePendulum.html
t1, w1, t2, w2 = state
a = (m1 + m2) * l1
b = m2 * l2 * cos(t1 - t2)
c = m2 * l1 * cos(t1 - t2)
d = m2 * l2
e = -m2 * l2 * w2^2 * sin(t1 - t2) - g * (m1 + m2) * sin(t1)
f = m2 * l1 * w1^2 * sin(t1 - t2) - m2 * g * sin(t2)
return ([w1, (e*d-b*f) / (a*d-c*b), w2, (a*f-c*e) / (a*d-c*b)])
end
l1 = 1.2 # length of rods
l2 = 1.0
m1 = 1.0 # weights of bobs
m2 = 1.5
t1 = 100.0 # inintial angles
t2 = -20.0
w1 = 0.0
w2 = 0.0
t = 1.0
dt = 0.04
state = [t1, w1, t2, w2] * pi / 180
GR.setprojectiontype(2)
gr3.setcameraprojectionparameters(45, 1, 100)
gr3.cameralookat(6, -2, 4, 0, -2, 0, 0, 1, 0)
gr3.setbackgroundcolor(1, 1, 1, 1)
gr3.setlightdirection(1, 1, 10)
now = start = time_ns()
while t < 30
t, state = rk4(t, dt, state, derivs)
t1, w1, t2, w2 = state
double_pendulum([t1, t2], [l1, l2], [m1, m2])
now = (time_ns() - start) / 1000000000
if t > now
sleep(t - now)
end
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 124 | #!/usr/bin/env julia
using GR
x = [-3.3 + t*0.1 for t in 0:66]
y = [(t^5 - 13*t^3 + 36*t)::Float64 for t in x]
plot(x, y)
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 496 | using GR
using LaTeXStrings
𝒩(μ, σ) = 1 / (σ * √(2π)) * exp.(-0.5 * ((x .- μ) / σ) .^ 2)
x = LinRange(-5, 5, 500);
y = hcat(𝒩(0, √0.2), 𝒩(0, √1), 𝒩(0, √5), 𝒩(-2, √0.5));
plot(x, y, xlabel=L"\mathcal{X}", ylabel=L"\mathcal{N}(\mu,\,\sigma^{2})", title=L"\frac{1}{\sigma\sqrt{2\pi}} e^{-\frac{1}{2} \left({\frac{x-\mu}{\sigma}}\right)^2}", labels=(L"\mu=0, \sigma^2=0.2", L"\mu=0, \sigma^2=1", L"\mu=0, \sigma^2=5", L"\mu={-2}, \sigma^2=0.5"), xlim=(-5.2, 5.2), ylim=(-0.05, 1.05), linewidth=3)
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 656 | #!/usr/bin/env julia
import Random
srand(seed) = Random.seed!(seed)
import GR
function main()
srand(0);
xd = -2 .+ 4 * rand(100)
yd = -2 .+ 4 * rand(100)
zd = [xd[i] * exp(-xd[i]^2 - yd[i]^2) for i = 1:100]
GR.setviewport(0.1, 0.95, 0.1, 0.95)
GR.setwindow(-2, 2, -2, 2)
GR.setmarkersize(1)
GR.setmarkertype(GR.MARKERTYPE_SOLID_CIRCLE)
GR.setcharheight(0.024)
GR.settextalign(2, 0)
GR.settextfontprec(3, 0)
x, y, z = GR.gridit(xd, yd, zd, 200, 200)
h = -0.6:0.05:0.6
GR.contourf(x, y, h, z, 2)
GR.polymarker(xd, yd)
GR.axes(0.25, 0.25, -2, -2, 2, 2, 0.01)
GR.updatews()
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 647 | using Gtk4
using GR
using Printf
c = GtkCanvas()
function _plot(ctx, w, h)
ENV["GKS_WSTYPE"] = "142"
ENV["GKSconid"] = @sprintf("%lu", UInt64(ctx.ptr))
plot(randn(10, 3), size=(w, h))
end
@guarded draw(c) do widget
ctx = getgc(c)
w = width(c)
h = height(c)
@show w, h
rectangle(ctx, 0, 0, w, h)
set_source_rgb(ctx, 1, 1, 1)
fill(ctx)
_plot(ctx, w, h)
end
win = GtkWindow("Gtk4 example", 600, 450)
win[] = c
e = GtkEventControllerMotion(c)
function on_motion(controller, x, y)
win.title = @sprintf("(%g, %g)", x, y)
reveal(c) # triggers a redraw
end
signal_connect(on_motion, e, "motion")
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1444 | using Gtk.ShortNames, Gtk.GConstants
using Printf
using GR
x = randn(1000000)
y = randn(1000000)
function plot(ctx, w, h)
global sl
ENV["GKS_WSTYPE"] = "142"
ENV["GKSconid"] = @sprintf("%lu", UInt64(ctx.ptr))
plt = kvs()
plt[:size] = (w, h)
nbins = Int64(Gtk.GAccessor.value(sl))
hexbin(x, y, nbins=nbins)
end
function draw(widget)
ctx = Gtk.getgc(widget)
w = Gtk.width(widget)
h = Gtk.height(widget)
Gtk.rectangle(ctx, 0, 0, w, h)
Gtk.set_source_rgb(ctx, 1, 1, 1)
Gtk.fill(ctx)
plot(ctx, w, h)
end
function resize_event(widget)
ctx = Gtk.getgc(widget)
h = Gtk.height(widget)
w = Gtk.width(widget)
Gtk.paint(ctx)
end
function motion_notify_event(widget::Gtk.GtkCanvas, event::Gtk.GdkEventMotion)
Gtk.GAccessor.text(lb, @sprintf("(%g, %g)", event.x, event.y))
end
function value_changed(widget::Gtk.GtkScale)
global canvas
draw(canvas)
reveal(canvas, true)
end
win = Window("Gtk") |> (bx = Box(:v))
lb = Label("(-, -)")
sl = Scale(false, 10, 100, 1)
Gtk.GAccessor.value(sl, 30)
canvas = Canvas(600, 450)
push!(bx, lb, sl, canvas)
signal_connect(motion_notify_event, canvas, "motion-notify-event")
signal_connect(value_changed, sl, "value_changed")
canvas.resize = resize_event
canvas.draw = draw
Gtk.showall(win)
if !isinteractive()
c = Condition()
signal_connect(win, :destroy) do widget
notify(c)
end
wait(c)
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 491 | using GR
function hopalong(num, a, b, c)
x::Float64, y::Float64 = 0, 0
u, v, d = Float64[], Float64[], Float64[]
for i = 1:num
xx = y - sign(x) * sqrt(abs(b*x - c)); yy = a - x; x = xx; y = yy;
push!(u, x); push!(v, y); push!(d, sqrt(x^2 + y^2))
end
setborderwidth(0)
scatter(u, v, ones(num), d, colormap=GR.COLORMAP_TERRAIN, title="Orbit of Hopalong attractor, num=$num, a=$a, b=$b, c=$c")
end
hopalong(1_000_000, 17.0, 0.314, 0.7773)
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 842 | using Plots
const x = [ 0, 0.850651, 0.850651, -0.850651, -0.850651, -0.525731, 0.525731, 0.525731, -0.525731, 0, 0, 0 ]
const y = [ -0.525731, 0, 0, 0, 0, 0.850651, 0.850651, -0.850651, -0.850651, -0.525731, 0.525731, 0.525731 ]
const z = [ 0.850651, 0.525731, -0.525731, -0.525731, 0.525731, 0, 0, 0, 0, -0.850651, -0.850651, 0.850651 ]
const connections = [
[ 3, 7, 2 ],
[ 8, 3, 2 ],
[ 5, 6, 4 ],
[ 4, 9, 5 ],
[ 6, 12, 7 ],
[ 7, 11, 6 ],
[ 11, 3, 10 ],
[ 10, 4, 11 ],
[ 9, 10, 8 ],
[ 8, 1, 9 ],
[ 1, 2, 12 ],
[ 12, 5, 1 ],
[ 3, 11, 7 ],
[ 7, 12, 2 ],
[ 6, 11, 4 ],
[ 5, 12, 6 ],
[ 8, 10, 3 ],
[ 2, 1, 8 ],
[ 10, 9, 4 ],
[ 9, 1, 5 ]
]
mesh3d(x, y, z; connections = connections, title = "triangles", legend = false,
xlabel = "x", ylabel = "y", zlabel = "z", fillcolor = RGBA.(palette(:tab20)))
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1117 | ENV["GKS_DOUBLE_BUF"] = "1"
using GR
const x = [ 0, 0.850651, 0.850651, -0.850651, -0.850651, -0.525731, 0.525731, 0.525731, -0.525731, 0, 0, 0 ]
const y = [ -0.525731, 0, 0, 0, 0, 0.850651, 0.850651, -0.850651, -0.850651, -0.525731, 0.525731, 0.525731 ]
const z = [ 0.850651, 0.525731, -0.525731, -0.525731, 0.525731, 0, 0, 0, 0, -0.850651, -0.850651, 0.850651 ]
const connections = [
[ 3, 3, 7, 2 ],
[ 3, 8, 3, 2 ],
[ 3, 5, 6, 4 ],
[ 3, 4, 9, 5 ],
[ 3, 6, 12, 7 ],
[ 3, 7, 11, 6 ],
[ 3, 11, 3, 10 ],
[ 3, 10, 4, 11 ],
[ 3, 9, 10, 8 ],
[ 3, 8, 1, 9 ],
[ 3, 1, 2, 12 ],
[ 3, 12, 5, 1 ],
[ 3, 3, 11, 7 ],
[ 3, 7, 12, 2 ],
[ 3, 6, 11, 4 ],
[ 3, 5, 12, 6 ],
[ 3, 8, 10, 3 ],
[ 3, 2, 1, 8 ],
[ 3, 10, 9, 4 ],
[ 3, 9, 1, 5 ]
]
# use GR's distinct colors
const colors = signed.([inqcolor(979+i) | 0xdf000000 for i in 1:size(connections)[1]])
setviewport(0, 1, 0, 1)
setwindow(-1, 1, -1, 1)
setwindow3d(-1, 1, -1, 1, -1, 1)
for angle in 0:360
clearws()
setspace3d(30 + angle, 80, 0, 0)
polygonmesh3d(x, y, z, vcat(connections...), colors)
updatews()
sleep(0.01)
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 623 | ENV["GKS_WSTYPE"] = "100"
using GR
using Printf
using BenchmarkTools
w, h = (800, 600)
image = rand(UInt32, w, h) # allocate the memory
mem = Printf.@sprintf("%p", pointer(image))
conid = Printf.@sprintf("!%dx%d@%s.mem", w, h, mem[3:end])
@btime begin
beginprint(conid)
plot(rand(1000), size=(w, h))
endprint()
end
emergencyclosegks()
delete!(ENV, "GKS_WSTYPE")
mwidth, mheight, width, height = inqdspsize()
size = 400 * mwidth / width
setwsviewport(0, size, 0, 3/4 * size)
setwswindow(0, 1, 0, 3/4)
setviewport(0, 1, 0, 3/4)
setwindow(0, 1, 0, 3/4)
drawimage(0, 1, 0, 3/4, w, h, image)
updatews()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 3782 | # This example requires CImGui#v1.82.2
#
# import Pkg; Pkg.add(name="CImGui", rev="v1.82.2")
#
using CImGui
using CImGui.ImGuiGLFWBackend
using CImGui.ImGuiGLFWBackend.LibCImGui
using CImGui.ImGuiGLFWBackend.LibGLFW
using CImGui.ImGuiOpenGLBackend
using CImGui.ImGuiOpenGLBackend.ModernGL
using CImGui.CSyntax
using CImGui.CSyntax.CStatic
using Printf
using GR
using LaTeXStrings
glfwDefaultWindowHints()
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3)
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 2)
if Sys.isapple()
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE) # 3.2+ only
glfwWindowHint(GLFW_OPENGL_FORWARD_COMPAT, GL_TRUE) # required on Mac
end
function draw(phi)
formulas = (
L"- \frac{{\hbar ^2}}{{2m}}\frac{{\partial ^2 \psi (x,t)}}{{\partial x^2 }} + U(x)\psi (x,t) = i\hbar \frac{{\partial \psi (x,t)}}{{\partial t}}",
L"\zeta \left({s}\right) := \sum_{n=1}^\infty \frac{1}{n^s} \quad \sigma = \Re(s) > 1",
L"\zeta \left({s}\right) := \frac{1}{\Gamma(s)} \int_{0}^\infty \frac{x^{s-1}}{e^x-1} dx" )
selntran(0)
settextfontprec(232, 3)
settextalign(2, 3)
settextcolorind(0) # white
chh = 0.036
clearws()
setcharheight(chh)
setcharup(sin(phi), cos(phi))
y = 0.6
for s in formulas
mathtex(0.5, y, s)
tbx, tby = inqmathtex(0.5, y, s)
fillarea(tbx, tby)
y -= 0.2
end
updatews()
end
# create window
window = glfwCreateWindow(960, 720, "Demo", C_NULL, C_NULL)
@assert window != C_NULL
glfwMakeContextCurrent(window)
glfwSwapInterval(1) # enable vsync
# create OpenGL and GLFW context
window_ctx = ImGuiGLFWBackend.create_context(window)
gl_ctx = ImGuiOpenGLBackend.create_context()
# setup Dear ImGui context
ctx = CImGui.CreateContext()
# create texture for image drawing
img_width, img_height = 500, 500
image_id = ImGuiOpenGLBackend.ImGui_ImplOpenGL3_CreateImageTexture(img_width, img_height)
ENV["GKS_WSTYPE"] = "100"
image = rand(GLuint, img_width, img_height) # allocate the memory
mem = Printf.@sprintf("%p",pointer(image))
conid = Printf.@sprintf("!%dx%d@%s.mem", img_width, img_height, mem[3:end])
# setup Platform/Renderer bindings
ImGuiGLFWBackend.init(window_ctx)
ImGuiOpenGLBackend.init(gl_ctx)
try
clear_color = Cfloat[0.45, 0.55, 0.60, 1.00]
while glfwWindowShouldClose(window) == 0
glfwPollEvents()
# start the Dear ImGui frame
ImGuiOpenGLBackend.new_frame(gl_ctx)
ImGuiGLFWBackend.new_frame(window_ctx)
CImGui.NewFrame()
# show image example
CImGui.Begin("GR Demo")
@cstatic phi = Cfloat(0.0) begin
@c CImGui.SliderFloat("Angle", &phi, 0, 360, "%.4f")
beginprint(conid)
draw(phi * π/180)
endprint()
ImGuiOpenGLBackend.ImGui_ImplOpenGL3_UpdateImageTexture(image_id, image, img_width, img_height)
CImGui.Image(Ptr{Cvoid}(image_id), CImGui.ImVec2(img_width, img_height))
CImGui.End()
end
# rendering
CImGui.Render()
glfwMakeContextCurrent(window)
width, height = Ref{Cint}(), Ref{Cint}() #! need helper fcn
glfwGetFramebufferSize(window, width, height)
display_w = width[]
display_h = height[]
glViewport(0, 0, display_w, display_h)
glClearColor(clear_color...)
glClear(GL_COLOR_BUFFER_BIT)
ImGuiOpenGLBackend.render(gl_ctx)
glfwMakeContextCurrent(window)
glfwSwapBuffers(window)
end
catch e
@error "Error in renderloop!" exception=e
Base.show_backtrace(stderr, catch_backtrace())
finally
ImGuiOpenGLBackend.shutdown(gl_ctx)
ImGuiGLFWBackend.shutdown(window_ctx)
CImGui.DestroyContext(ctx)
glfwDestroyWindow(window)
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1358 | #!/usr/bin/env julia
# Calculate Julia set in Julia
import GR
function julia(z, c, iters)
ci = 0
inc = 1
for i in 0:iters
z = z^2 + c
if abs2(z) >= 4
return ci
end
ci += inc
if ci == 0 || ci == 255
inc = -inc
end
end
return 255
end
function main()
function create_fractal(min_x, max_x, min_y, max_y, image, iters)
height = size(image, 1)
width = size(image, 2)
pixel_size_x = (max_x - min_x) / width
pixel_size_y = (max_y - min_y) / height
for i in 1:width
real = min_x + (i - 1) * pixel_size_x
for j in 1:height
imag = min_y + (j - 1) * pixel_size_y
color = julia(complex(real, imag), seed, iters)
image[j, i] = color
end
end
end
seed = complex(-0.156844471694257101941, -0.649707745759247905171)
x, y = (-0.16, -0.64)
f = 1.5
for i in 0:100
image = zeros(Int32, 500, 500)
dt = @elapsed create_fractal(x-f, x+f, y-f, y+f, image, 500)
println("Julia set created in $dt s")
GR.clearws()
GR.setviewport(0, 1, 0, 1)
GR.setcolormap(13)
GR.cellarray(0, 1, 0, 1, 500, 500, image .+ 1000)
GR.updatews()
f *= 0.9
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 869 | ENV["GKS_ENCODING"] = "latin1"
import GR
GR.selntran(0)
GR.setcharheight(0.024)
function main()
GR.settextfontprec(2, 0)
y = 0
for i in 0:1
GR.text(0.05, 0.85-y, " !\"#\$\$%&'()*+,-./")
GR.text(0.05, 0.80-y, "0123456789:;<=>?")
GR.text(0.05, 0.75-y, "@ABCDEFGHIJKLMNO")
GR.text(0.05, 0.70-y, "PQRSTUVWXYZ[\\]^_")
GR.text(0.05, 0.65-y, "`abcdefghijklmno")
GR.text(0.05, 0.60-y, "pqrstuvwxyz{|}~ ")
GR.text(0.5, 0.85-y, collect(0xa0:0x1:0xaf))
GR.text(0.5, 0.80-y, collect(0xb0:0x1:0xbf))
GR.text(0.5, 0.75-y, collect(0xc0:0x1:0xcf))
GR.text(0.5, 0.70-y, collect(0xd0:0x1:0xdf))
GR.text(0.5, 0.65-y, collect(0xe0:0x1:0xef))
GR.text(0.5, 0.60-y, collect(0xf0:0x1:0xff))
GR.settextfontprec(233, 3)
y = 0.4
end
GR.updatews()
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 641 | ENV["GKS_WSTYPE"] = "gksqt"
using GR
function simulation(c::Channel)
z = peaks()
for step = 10:0.1:100
put!(c, z .* step)
end
end
function main()
mouse = Nothing
rotation = 30
tilt = 60
for data in Channel(simulation)
x, y, buttons = samplelocator()
if mouse != Nothing && buttons != 0
rotation += 50 * (x - mouse[1])
tilt += 50 * (y - mouse[2])
end
mouse = [x, y]
surface(data, rotation=rotation, tilt=tilt, title="Press MB1 to rotate, MB2 to quit")
if buttons & 0x02 != 0
break
end
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 402 | #!/usr/bin/env julia
import GR
function main()
x = LinRange(0, 20, 50)
y = x .^ 2
GR.setviewport(0.1, 0.95, 0.1, 0.95)
GR.setwindow(0, 20, 0.2, 400)
GR.setscale(GR.OPTION_Y_LOG2)
GR.settextfontprec(233, 3)
GR.setmarkersize(1)
GR.setmarkertype(GR.MARKERTYPE_SOLID_CIRCLE)
GR.polymarker(x, y)
GR.axes(2, 2, 0, 0.2, 1, 2, 0.005)
GR.updatews()
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 5616 | import GR
j = [0.19454, 0.1194, 0.19454, 0.41129, 0.10807, 0.3875, 0.10807, 0.07711, 0.10807, 0.06075, 0.10681, 0.04973, 0.1043, 0.04407, 0.10178, 0.0384, 0.09813, 0.03557, 0.09335, 0.03557, 0.09108, 0.03557, 0.08856, 0.03645, 0.08579, 0.03822, 0.08303, 0.03998, 0.07963, 0.0435, 0.0756, 0.04879, 0.07207, 0.05357, 0.06761, 0.05842, 0.06219, 0.06333, 0.05678, 0.06824, 0.04954, 0.07069, 0.04048, 0.07069, 0.0284, 0.07069, 0.01877, 0.06767, 0.0116, 0.06163, 0.00442, 0.05559, 0.00083, 0.04816, 0.00083, 0.03935, 0.00083, 0.02878, 0.00725, 0.01971, 0.02009, 0.01216, 0.03293, 0.00461, 0.05143, 0.00083, 0.0756, 0.00083, 0.09347, 0.00083, 0.10971, 0.00228, 0.12431, 0.00518, 0.13891, 0.00807, 0.15143, 0.0138, 0.16188, 0.02236, 0.17233, 0.03092, 0.18038, 0.04294, 0.18605, 0.05842, 0.19171, 0.0739, 0.19454, 0.09423, 0.19454, 0.1194]
u = [0.31464, 0.40298, 0.22855, 0.40298, 0.22855, 0.20323, 0.22855, 0.1909, 0.23113, 0.17932, 0.23629, 0.16849, 0.24145, 0.15767, 0.24856, 0.14823, 0.25763, 0.14017, 0.26669, 0.13212, 0.27726, 0.12576, 0.28935, 0.1211, 0.30143, 0.11644, 0.31452, 0.11412, 0.32862, 0.11412, 0.3407, 0.11412, 0.35316, 0.11701, 0.366, 0.1228, 0.37884, 0.12859, 0.39092, 0.13627, 0.40225, 0.14583, 0.40225, 0.1194, 0.48834, 0.1194, 0.48834, 0.40298, 0.40225, 0.40298, 0.40225, 0.19832, 0.3957, 0.18825, 0.38803, 0.17988, 0.37922, 0.17321, 0.3704, 0.16654, 0.36273, 0.1632, 0.35618, 0.1632, 0.35039, 0.1632, 0.34498, 0.16427, 0.33994, 0.16641, 0.33491, 0.16855, 0.3305, 0.17139, 0.32673, 0.17491, 0.32295, 0.17843, 0.31999, 0.18265, 0.31785, 0.18756, 0.31571, 0.19247, 0.31464, 0.19769, 0.31464, 0.20323]
l = [0.60797, 0.1194, 0.60797, 0.54081, 0.52225, 0.51702, 0.52225, 0.1194]
i = [0.64226, 0.3875, 0.64226, 0.1194, 0.72835, 0.1194, 0.72835, 0.41129]
a = [0.91001, 0.26591, 0.91001, 0.18397, 0.9012, 0.17743, 0.89321, 0.17201, 0.88604, 0.16773, 0.87886, 0.16345, 0.87175, 0.16132, 0.8647, 0.16132, 0.86118, 0.16132, 0.8579, 0.16238, 0.85488, 0.16452, 0.85186, 0.16666, 0.84909, 0.1695, 0.84658, 0.17302, 0.84406, 0.17655, 0.84211, 0.18076, 0.84072, 0.18567, 0.83934, 0.19058, 0.83865, 0.19568, 0.83865, 0.20096, 0.83865, 0.20776, 0.84079, 0.21431, 0.84507, 0.2206, 0.84934, 0.22689, 0.85495, 0.23281, 0.86187, 0.23835, 0.86879, 0.24388, 0.87647, 0.24898, 0.8849, 0.25364, 0.89334, 0.2583, 0.90171, 0.26239, 0.91001, 0.26591, 0.99648, 0.1194, 0.99648, 0.33199, 0.99648, 0.34382, 0.99422, 0.35452, 0.98969, 0.36409, 0.98516, 0.37365, 0.97811, 0.38184, 0.96854, 0.38863, 0.95898, 0.39543, 0.94683, 0.40065, 0.9321, 0.4043, 0.91738, 0.40795, 0.89982, 0.40978, 0.87943, 0.40978, 0.86281, 0.40978, 0.84708, 0.40802, 0.83223, 0.40449, 0.81737, 0.40097, 0.80428, 0.39618, 0.79296, 0.39014, 0.78163, 0.3841, 0.77263, 0.37686, 0.76596, 0.36843, 0.75929, 0.36, 0.75595, 0.35087, 0.75595, 0.34105, 0.75595, 0.33048, 0.75973, 0.32173, 0.76728, 0.31481, 0.77483, 0.30789, 0.78465, 0.30443, 0.79673, 0.30443, 0.80454, 0.30443, 0.81102, 0.30556, 0.81618, 0.30783, 0.82134, 0.31009, 0.8253, 0.31311, 0.82807, 0.31689, 0.83084, 0.32066, 0.83279, 0.32507, 0.83393, 0.3301, 0.83506, 0.33514, 0.83563, 0.3403, 0.83563, 0.34559, 0.83563, 0.3549, 0.83827, 0.36283, 0.84356, 0.36937, 0.84884, 0.37592, 0.85828, 0.37919, 0.87188, 0.37919, 0.88346, 0.37919, 0.89271, 0.37542, 0.89963, 0.36786, 0.90655, 0.36031, 0.91001, 0.34747, 0.91001, 0.32935, 0.91001, 0.30141, 0.90057, 0.29914, 0.88899, 0.29562, 0.87729, 0.2919, 0.86546, 0.288, 0.85362, 0.2841, 0.84223, 0.27982, 0.83128, 0.27516, 0.82033, 0.27051, 0.81007, 0.26541, 0.80051, 0.25987, 0.79094, 0.25433, 0.78257, 0.24804, 0.7754, 0.24099, 0.76822, 0.23394, 0.76256, 0.22601, 0.75841, 0.2172, 0.75425, 0.20839, 0.75217, 0.19857, 0.75217, 0.18775, 0.75217, 0.17717, 0.75413, 0.16742, 0.75803, 0.15848, 0.76193, 0.14955, 0.76753, 0.14181, 0.77483, 0.13526, 0.78213, 0.12872, 0.791, 0.12355, 0.80145, 0.11978, 0.8119, 0.116, 0.82367, 0.11411, 0.83676, 0.11411, 0.84632, 0.11411, 0.85463, 0.11481, 0.86168, 0.11619, 0.86873, 0.11758, 0.87508, 0.11953, 0.88075, 0.12204, 0.88641, 0.12456, 0.89151, 0.12752, 0.89604, 0.13092, 0.90057, 0.13432, 0.90523, 0.13803, 0.91001, 0.14206, 0.91001, 0.1194]
function draw_path(p, codes, fill, border)
GR.setfillcolorind(fill)
GR.setbordercolorind(border)
op = border == 0 ? 'f' : 'F'
GR.path(p[1:2:end], p[2:2:end], codes)
end
function circle(xmin, ymin, xmax, ymax, fill, border)
GR.setfillintstyle(4)
GR.setfillcolorind(fill)
GR.setbordercolorind(border)
GR.fillarc(xmin, xmax, ymin, ymax, 0, 360)
end
GR.setcolorrep(1, 0.14, 0.14, 0.14)
GR.setcolorrep(2, 0.251, 0.388, 0.847) # darker blue
GR.setcolorrep(3, 0.796, 0.235, 0.2) # darker red
GR.setcolorrep(4, 0.584, 0.345, 0.698) # darker purple
GR.setcolorrep(5, 0.22, 0.596, 0.149) # darker green
GR.setcolorrep(6, 0.4, 0.51, 0.878) # lighter blue
GR.setcolorrep(7, 0.835, 0.388, 0.361) # lighter red
GR.setcolorrep(8, 0.667, 0.475, 0.757) # lighter purple
GR.setcolorrep(9, 0.376, 0.678, 0.318) # lighter green
GR.setviewport(0, 1, 0, 1)
GR.setwindow(0, 1, 0, 1)
GR.updatews()
for s in 0.1:0.01:0.5
GR.clearws()
GR.setviewport(0.5 - s, 0.5 + s, 0.5 - s, 0.5 + s)
GR.setborderwidth(s * 8)
draw_path(j, "MLLLCCCCCCCCCCCCCCf", 1, 0)
draw_path(u, "MLLCCCCCCLLLLLCCCCCCf", 1, 0)
draw_path(l, "MLLLf", 1, 0)
draw_path(i, "MLLLf", 1, 0)
draw_path(a, "MLCCCCCCCCCCZMLCCCCCCCCCCCCCCCCCCLLCCCCCCCCCCCCCCLf", 1, 0)
circle(0.09698, 0.43274, 0.20364, 0.5394, 2, 6)
circle(0.63794, 0.43274, 0.7446, 0.5394, 3, 7)
circle(0.70794, 0.55274, 0.8146, 0.6594, 4, 8)
circle(0.77794, 0.43274, 0.8846, 0.5394, 5, 9)
GR.updatews()
sleep(0.01)
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 655 | using GR
Base.@kwdef mutable struct Lorenz
dt::Float64 = 0.02
σ::Float64 = 10
ρ::Float64 = 28
β::Float64 = 8/3
x::Float64 = 1
y::Float64 = 1
z::Float64 = 1
end
function step!(l::Lorenz)
dx = l.σ * (l.y - l.x)
l.x += l.dt * dx
dy = l.x * (l.ρ - l.z) - l.y
l.y += l.dt * dy
dz = l.x * l.y - l.β * l.z
l.z += l.dt * dz
end
attractor = Lorenz()
x = [1.0]
y = [1.0]
z = [1.0]
for i = 1:1500
step!(attractor)
push!(x, attractor.x)
push!(y, attractor.y)
push!(z, attractor.z)
plot3(x, y, z, xlim = (-30, 30), ylim = (-30, 30), zlim = (0, 60), title = "Lorenz Attractor")
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 405 | using DSP
using GR
fs = 96_000
tmax = (8192 - 1) / fs
t = 0:1/fs:tmax
N = length(t)
signal = randn.(N) # noise
for f in (261.3, 329.63, 392.00)
global signal
signal = signal .+ sin.(2π * f .* t)
end
responsetype = Lowpass(500; fs)
designmethod = FIRWindow(hanning(128; zerophase=false))
f = filt(digitalfilter(responsetype, designmethod), signal)
plot(t, f, title="Low-pass filtered signal")
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1356 | #!/usr/bin/env julia
import GR
function mandel(x, y, iters)
c = complex(x, y)
z = 0.0im
ci = 0
inc = 1
for i in 0:iters
z = z^2 + c
if abs2(z) >= 4
return ci
end
ci += inc
if ci == 0 || ci == 255
inc = -inc
end
end
return 255
end
function main()
function create_fractal(min_x, max_x, min_y, max_y, image, iters)
height = size(image, 1)
width = size(image, 2)
pixel_size_x = (max_x - min_x) / width
pixel_size_y = (max_y - min_y) / height
for i in 1:width
real = min_x + (i - 1) * pixel_size_x
for j in 1:height
imag = min_y + (j - 1) * pixel_size_y
color = mandel(real, imag, iters)
image[j, i] = color
end
end
end
x = -0.9223327810370947027656057193752719757635
y = 0.3102598350874576432708737495917724836010
f = 0.5
for i in 0:200
image = zeros(Int32, 500, 500)
dt = @elapsed create_fractal(x-f, x+f, y-f, y+f, image, 400)
println("Mandelbrot created in $dt s")
GR.clearws()
GR.setviewport(0, 1, 0, 1)
GR.setcolormap(13)
GR.cellarray(0, 1, 0, 1, 500, 500, image .+ 1000)
GR.updatews()
f *= 0.9
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 2581 | #!/usr/bin/env julia
# Calculate Mandelbrot set using OpenCL
using OpenCL
import GR
const mandel_kernel = "
#pragma OPENCL EXTENSION cl_khr_byte_addressable_store : enable
__kernel void mandelbrot(__global double2 *q, __global ushort *output,
double const min_x, double const max_x,
double const min_y, double const max_y,
ushort const width, ushort const height,
ushort const iters)
{
int ci = 0, inc = 1;
int gid = get_global_id(0);
double nreal, real = 0;
double imag = 0;
q[gid].x = min_x + (gid % width) * (max_x - min_x) / width;
q[gid].y = min_y + (gid / width) * (max_y - min_y) / height;
output[gid] = iters;
for (int curiter = 0; curiter < iters; curiter++) {
nreal = real * real - imag * imag + q[gid].x;
imag = 2 * real * imag + q[gid].y;
real = nreal;
if (real * real + imag * imag >= 4) {
output[gid] = ci;
return;
}
ci += inc;
if (ci == 0 || ci == 255)
inc = -inc;
}
}"
for device in reverse(cl.available_devices(cl.platforms()[1]))
global ctx, queue, prg
ctx = cl.Context(device)
queue = cl.CmdQueue(ctx)
try
prg = cl.Program(ctx, source = mandel_kernel) |> cl.build!
println(device[:name])
break
catch
true
end
end
function calc_fractal(q, min_x, max_x, min_y, max_y, width, height, iters)
global ctx, queue, prg
output = Array{UInt16}(undef, size(q))
q_opencl = cl.Buffer(ComplexF64, ctx, (:r, :copy), hostbuf=q)
output_opencl = cl.Buffer(UInt16, ctx, :w, length(output))
k = cl.Kernel(prg, "mandelbrot")
queue(k, length(q), nothing, q_opencl, output_opencl,
min_x, max_x, min_y, max_y,
UInt16(width), UInt16(height), UInt16(iters))
cl.copy!(queue, output, output_opencl)
return output
end
function create_fractal(min_x, max_x, min_y, max_y, width, height, iters)
q = zeros(ComplexF64, (width, height))
output = calc_fractal(q, min_x, max_x, min_y, max_y, width, height, iters)
return output
end
x = -0.9223327810370947027656057193752719757635
y = 0.3102598350874576432708737495917724836010
for i in 1:200
f = 0.5 * 0.9^i
dt = @elapsed image = create_fractal(x-f, x+f, y-f, y+f, 500, 500, 400)
println("Mandelbrot created in $dt s")
GR.clearws()
GR.setviewport(0, 1, 0, 1)
GR.setcolormap(13)
GR.cellarray(0, 1, 0, 1, 500, 500, image .+ 1000)
GR.updatews()
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 800 | using GR
using LaTeXStrings
hor_align = Dict("Left" => 1, "Center" => 2, "Right" => 3)
vert_align = Dict("Top" => 1, "Cap" => 2, "Half" => 3, "Base" => 4, "Bottom" => 5)
selntran(0)
setcharheight(0.018)
settextfontprec(232, 3)
for angle in 0:360
setcharup(sin(-angle * pi/180), cos(-angle * pi/180))
setmarkertype(GR.MARKERTYPE_SOLID_PLUS)
setmarkercolorind(4)
clearws()
for halign in keys(hor_align)
for valign in keys(vert_align)
settextalign(hor_align[halign], vert_align[valign])
x = -0.1 + hor_align[halign] * 0.3;
y = 1.1 - vert_align[valign] * 0.2;
s = L"1+\frac{1+\frac{a}{b}}{1+\frac{1}{1+\frac{1}{a}}}"
mathtex(x, y, s)
tbx, tby = inqmathtex(x, y, s)
fillarea(tbx, tby)
polymarker([x], [y])
end
end
updatews()
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 728 | using GR
using LaTeXStrings
formulas = (
L"- \frac{{\hbar ^2}}{{2m}}\frac{{\partial ^2 \psi (x,t)}}{{\partial x^2 }} + U(x)\psi (x,t) = i\hbar \frac{{\partial \psi (x,t)}}{{\partial t}}",
L"\zeta \left({s}\right) := \sum_{n=1}^\infty \frac{1}{n^s} \quad \sigma = \Re(s) > 1",
L"\zeta \left({s}\right) := \frac{1}{\Gamma(s)} \int_{0}^\infty \frac{x^{s-1}}{e^x-1} dx" )
selntran(0)
settextfontprec(232, 3)
settextalign(2, 3)
chh = 0.036
for phi in LinRange(0, 2pi, 360)
clearws()
setcharheight(chh)
setcharup(sin(phi), cos(phi))
y = 0.6
for s in formulas
mathtex(0.5, y, s)
tbx, tby = inqmathtex(0.5, y, s)
fillarea(tbx, tby)
y -= 0.2
end
updatews()
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 660 | using GR
using LaTeXStrings
s = "Using inline math \$\\frac{2hc^2}{\\lambda^5} \\frac{1}{e^{\\frac{hc}{\\lambda k_B T}} - 1}\$ in GR text\nmixed with LaTeXStrings " * L"- \frac{{\hbar ^2}}{{2m}}\frac{{\partial ^2 \psi (x,t)}}{{\partial x^2 }} + U(x)\psi (x,t) = i\hbar \frac{{\partial \psi (x,t)}}{{\partial t}}" * "\n– with line breaks\nand UTF-8 characters (ħπ),\nand rendered using GR's text attributes"
selntran(0)
settextfontprec(232, 3)
settextalign(2, 3)
setcharheight(0.02)
for ϕ in LinRange(0, 2π, 360)
clearws()
setcharup(sin(ϕ), cos(ϕ))
text(0.5, 0.5, s)
tbx, tby = inqtext(0.5, 0.5, s)
fillarea(tbx, tby)
updatews()
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 162 | using MAT
D = matread("mri.mat")
vol = dropdims(D["D"], dims=3)
using GR
for slice in 1:27
imshow(255 .- vol[:, : , slice], colormap=GR.COLORMAP_BONE)
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1458 | #!/usr/bin/env julia
import GR
const theta = 70.0 # initial angle
const gamma = 0.1 # damping coefficient
const L = 0.2 # wire length
w, h, ball = GR.readimage("ball.png")
function rk4(x, h, y, f)
k1 = h * f(x, y)
k2 = h * f(x + 0.5 * h, y + 0.5 * k1)
k3 = h * f(x + 0.5 * h, y + 0.5 * k2)
k4 = h * f(x + h, y + k3)
return x + h, y + (k1 + 2 * (k2 + k3) + k4) / 6.0
end
function deriv(t, state)
theta, omega = state
return [omega, -gamma * omega - 9.81 / L * sin(theta)]
end
function draw_cradle(theta)
GR.clearws()
GR.setviewport(0, 1, 0, 1)
GR.setcolorrep(1, 0.7, 0.7, 0.7)
# draw pivot point
GR.fillarea([0.3, 0.7, 0.7, 0.3], [0.79, 0.79, 0.81, 0.81])
# draw balls
for i = -2:2
x = [0.5, 0.5] .+ i * 0.06
y = [0.8, 0.4]
if (theta < 0 && i == -2) || (theta > 0 && i == 2)
x[2] += sin(theta) * 0.4
y[2] = 0.8 - cos(theta) * 0.4
end
GR.polyline(x, y) # draw wire
GR.drawimage(x[2]-0.03, x[2]+0.03, y[2]-0.03, y[2]+0.03, 50, 50, ball)
end
GR.updatews()
end
function main()
t = 0.0
dt = 0.01
state = [theta * pi / 180, 0]
start = refresh = time_ns()
while t < 30
t, state = rk4(t, dt, state, deriv)
theta, omega = state
if time_ns() - refresh > 20 * 1000000 # 20ms
draw_cradle(theta)
refresh = time_ns()
end
now = (time_ns() - start) / 1000000000
if t > now
sleep(t - now)
end
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1648 | #
# Atomic Orbitals - based on Christina C. Lee's blog post
# (see https://albi3ro.github.io/M4/prerequisites/Atomic-Orbitals.html)
#
using GSL # GSL holds the special functions
using GR
a0 = 5.291772109217e-11
# The unitless radial coordinate
ρ(r,n) = 2r/(n*a0)
# The θ and ϕ dependence
function Yml(m::Int,l::Int,θ::Real,ϕ::Real)
(-1.0)^m*sf_legendre_Plm(l,abs(m),cos(θ))*ℯ^(im*m*ϕ)
end
# The Radial dependence
function R(n::Int,l::Int,ρ::Real)
sf_laguerre_n(n-l-1,2*l+1,ρ)*ℯ^(-ρ/2)*ρ^l
end
# A normalization: This is dependent on the choice of polynomial representation
function norm(n::Int,l::Int)
sqrt((2/n)^3 * factorial(n-l-1)/(2n*factorial(n+l)))
end
# Generates an Orbital Funtion of (r,θ,ϕ) for a specificied n,l,m.
function Orbital(n::Int,l::Int,m::Int)
# we make sure l and m are within proper bounds
if l > n || abs(m) > l
throw(DomainError())
end
Ψ(ρ,θ,ϕ) = norm(n,l) * R(n,l,ρ) * abs(Yml(m,l,θ,ϕ))
Ψ
end
function CarttoSph(x::Array,y::Array,z::Array)
r = sqrt.(x.^2+y.^2+z.^2)
θ = acos.(z./r)
ϕ = atan.(y./x)
r,θ,ϕ
end
function calculate_electronic_density(n,l,m)
N = 50
r = 1e-9
s = LinRange(-r,r,N)
x,y,z = meshgrid(s,s,s)
r,θ,ϕ = CarttoSph(x,y,z)
Ψ = Orbital(n,l,m)
Ψv = zeros(Float32,N,N,N)
for i in 1:N
for j in 1:N
for k in 1:N
Ψv[i,j,k] = abs(Ψ(ρ(r[i,j,k],n),θ[i,j,k],ϕ[i,j,k]))
end
end
end
(Ψv.-minimum(Ψv))./(maximum(Ψv).-minimum(Ψv))
end
Ψv = calculate_electronic_density(3,2,0)
for alpha in 0:360
isosurface(Ψv,isovalue=0.25,rotation=alpha)
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 184 | using GR
r = 1
θ, φ = meshgrid(range(0, stop=2π, length=200), range(0, stop=2π, length=100))
x = r * sin.(θ) .* sin.(φ)
y = r * sin.(θ) .* cos.(φ)
z = r * cos.(θ)
surface(x, y, z)
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1993 | using GR
using Printf
using LinearAlgebra
# Simple particle simulation
const N = 500 # number of particles
M = 0.05 * ones(Float64, N) # masses
const S = 0.04 # size of particles
const dt = 1.0 / 30
function step(dt, p, v)
# update positions
p[:,:] += dt * v[:,:]
# find pairs of particles undergoing a collision
for i in 1:N
for j in i+1:N
dx = p[i,1] - p[j,1]
dy = p[i,2] - p[j,2]
d = sqrt(dx*dx + dy*dy)
if d < 2*S
# relative location & velocity vectors
r_rel = p[i] - p[j]
v_rel = v[i] - v[j]
# momentum vector of the center of mass
v_cm = (M[i] * v[i] + M[j] * v[j]) / (M[i] + M[j])
# collisions of spheres reflect v_rel over r_rel
rr_rel = dot(r_rel, r_rel)
vr_rel = dot(v_rel, r_rel)
v_rel = 2 * r_rel * vr_rel / rr_rel - v_rel
# assign new velocities
v[i] = v_cm + v_rel * M[j] / (M[i] + M[j])
v[j] = v_cm - v_rel * M[i] / (M[i] + M[j])
end
end
end
# check for crossing boundary
for i in 1:N
if p[i,1] < -2 + S
p[i,1] = -2 + S
v[i,1] *= -1
elseif p[i,1] > 2 - S
p[i,1] = 2 - S
v[i,1] *= -1
end
if p[i,2] < -2 + S
p[i,2] = -2 + S
v[i,2] *= -1
elseif p[i,2] > 2 - S
p[i,2] = 2 - S
v[i,2] *= -1
end
end
return p, v
end
function main()
setwindow(-2, 2, -2, 2)
setviewport(0, 1, 0, 1)
setmarkertype(GR.MARKERTYPE_SOLID_CIRCLE)
setmarkersize(1.0)
n = 0
t = 0.0
p = (rand(N,2) .- 0.5) .* (4 .- 2 .* S) # initial positions
v = rand(N,2) .- 0.5 # initial velocities
s = time_ns()
while n < 300
p, v = step(dt, p, v)
clearws()
setmarkercolorind(983)
polymarker(p[:,1], p[:,2])
if n > 0
text(0.01, 0.95, @sprintf("%10s: %4d fps", "Julia", round(n / (1e-9 * (time_ns() - s)))))
end
updatews()
n += 1
t += dt
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 2609 | #!/usr/bin/env julia
import GR
using LaTeXStrings
using Printf
const g = 9.81 # gravity acceleration
const Γ = 0.1 # damping coefficient
function main()
function rk4(x, h, y, f)
k1 = h * f(x, y)
k2 = h * f(x + 0.5 * h, y + 0.5 * k1)
k3 = h * f(x + 0.5 * h, y + 0.5 * k2)
k4 = h * f(x + h, y + k3)
x + h, y + (k1 + 2 * (k2 + k3) + k4) / 6.0
end
function derivs(t, state)
θ, ω = state
[ω, -Γ * ω - g / L * sin(θ)]
end
function pendulum(t, θ, ω, acceleration)
GR.clearws()
GR.setviewport(0, 1, 0, 1)
x = [0.5, 0.5 + sin(θ) * 0.4]
y = [0.8, 0.8 - cos(θ) * 0.4]
# draw pivot point
GR.fillarea([0.46, 0.54, 0.54, 0.46], [0.79, 0.79, 0.81, 0.81]),
GR.setlinecolorind(1)
GR.setlinewidth(2)
GR.polyline(x, y) # draw rod
GR.setmarkersize(5)
GR.setmarkertype(GR.MARKERTYPE_SOLID_CIRCLE)
GR.setmarkercolorind(86)
GR.setborderwidth(0)
GR.polymarker([x[2]], [y[2]]) # draw bob
GR.setlinecolorind(4)
V = 0.05 * ω # show angular velocity
GR.drawarrow(x[2], y[2], x[2] + V * cos(θ), y[2] + V * sin(θ))
GR.setlinecolorind(2)
A = 0.05 * acceleration # show angular acceleration
GR.drawarrow(x[2], y[2], x[2] + A * sin(θ), y[2] + A * cos(θ))
GR.settextfontprec(232, 3) # CM Serif Roman
GR.setcharheight(0.032)
GR.settextcolorind(1)
GR.text(0.05, 0.95, "Damped Pendulum")
GR.setcharheight(0.040)
GR.text(0.4, 0.22, L"\omega=\dot{\theta}")
GR.text(0.4, 0.1, L"\dot{\omega}=-\gamma\omega-\frac{g}{l}sin(\theta)")
GR.settextfontprec(GR.FONT_COURIER, 0) # Courier
GR.setcharheight(0.024)
GR.text(0.05, 0.22, "t:$(@sprintf("%7.2f", t))")
GR.text(0.05, 0.16, "θ:$(@sprintf("%7.2f", θ / π * 180))")
GR.settextcolorind(4)
GR.text(0.05, 0.10, "ω:$(@sprintf("%7.2f", ω))")
GR.settextcolorind(2)
GR.text(0.05, 0.04, "A:$(@sprintf("%7.2f", acceleration))")
GR.updatews()
end
θ = 70.0 # initial angle
L = 1 # pendulum length
t = 0
dt = 0.04
state = [θ * π / 180, 0]
now = start = time_ns()
while t < 30
t, state = rk4(t, dt, state, derivs)
θ, ω = state
acceleration = sqrt(2 * g * L * (1 - cos(θ)))
pendulum(t, θ, ω, acceleration)
now = (time_ns() - start) / 1000000000
if t > now
sleep(t - now)
end
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 214 | using GR
using LaTeXStrings
f(x) = x*exp(-2x*im)
x = 0:0.01:π
w = f.(x)
plot(real.(w), imag.(w), x, linewidth=5, title=L"f(x) = xe^{-2xi} \forall x \in [0,\pi]", xlabel=L"\Re e\{f(x)\}", ylabel=L"\Im e\{f(x)\}")
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 263 | using GR
using LaTeXStrings
f(x) = x*exp(-2x*im)
x = 0:0.01:π
w = f.(x)
setscientificformat(3)
plot3(real.(w), imag.(w), abs.(w), linewidth=3, xlabel=L"\Re e\{f(x)\}", ylabel=L"\Im e\{f(x)\}", zlabel=L"|f(x)|", title=L"f(x) = xe^{-2xi} \forall x \in [0,\pi]")
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 2545 | ### A Pluto.jl notebook ###
# v0.19.27
using Markdown
using InteractiveUtils
# This Pluto notebook uses @bind for interactivity. When running this notebook outside of Pluto, the following 'mock version' of @bind gives bound variables a default value (instead of an error).
macro bind(def, element)
quote
local iv = try Base.loaded_modules[Base.PkgId(Base.UUID("6e696c72-6542-2067-7265-42206c756150"), "AbstractPlutoDingetjes")].Bonds.initial_value catch; b -> missing; end
local el = $(esc(element))
global $(esc(def)) = Core.applicable(Base.get, el) ? Base.get(el) : iv(el)
el
end
end
# ╔═╡ 43e5cbb6-5557-492e-9aeb-f7cbde220e15
begin
import Pkg
Pkg.activate() # disable Pluto's package management
end
# ╔═╡ e2d1cb38-2698-11eb-2e59-632faa201d6f
begin
using DelimitedFiles
using PlutoUI
ENV["GRDISPLAY"] = "pluto"
using GR
end
# ╔═╡ 49d120ea-2699-11eb-203d-fddbb33bb08b
begin
url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_covid19_confirmed_global.csv"
download(url, "covid19.csv")
end;
# ╔═╡ 4b5f800a-2699-11eb-2528-b5917c0461cf
begin
data = readdlm("covid19.csv", ',')
ncountries, ncols = size(data)
ndays = ncols - 4
end;
# ╔═╡ 1c4aec86-269a-11eb-2204-098352e8267c
@bind countries MultiSelect(["Germany", "Austria", "Belgium", "Netherlands", "France", "Italy", "Spain", "US"], default=["Germany"])
# ╔═╡ 75ad9770-2699-11eb-0db7-5948da39d6e1
begin
cummulated = Dict()
for i in 1:ncountries
country = data[i,2]
if country in countries
if !haskey(cummulated, country) cummulated[country] = zeros(ndays) end
cummulated[country] .+= collect(data[i,5:end])
end
end
end
# ╔═╡ 86c70334-2699-11eb-1b52-cfa50b314e2b
begin
day = collect(Float64, 1:ndays);
confirmed = hcat([cummulated[country] for country in countries]...)
end;
# ╔═╡ 918f640a-2699-11eb-02d8-998ed76c614a
plot(day, confirmed, xlim=(0, ndays+1), ylim=(10, 100_000_000), ylog=true,
title="Confirmed SARS–CoV–2 infections", xlabel="Day", ylabel="Confirmed",
labels=countries, location=4)
# ╔═╡ Cell order:
# ╠═43e5cbb6-5557-492e-9aeb-f7cbde220e15
# ╠═e2d1cb38-2698-11eb-2e59-632faa201d6f
# ╠═49d120ea-2699-11eb-203d-fddbb33bb08b
# ╠═4b5f800a-2699-11eb-2528-b5917c0461cf
# ╠═75ad9770-2699-11eb-0db7-5948da39d6e1
# ╠═86c70334-2699-11eb-1b52-cfa50b314e2b
# ╠═1c4aec86-269a-11eb-2204-098352e8267c
# ╠═918f640a-2699-11eb-02d8-998ed76c614a
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1480 | ### A Pluto.jl notebook ###
# v0.19.22
using Markdown
using InteractiveUtils
# This Pluto notebook uses @bind for interactivity. When running this notebook outside of Pluto, the following 'mock version' of @bind gives bound variables a default value (instead of an error).
macro bind(def, element)
quote
local iv = try Base.loaded_modules[Base.PkgId(Base.UUID("6e696c72-6542-2067-7265-42206c756150"), "AbstractPlutoDingetjes")].Bonds.initial_value catch; b -> missing; end
local el = $(esc(element))
global $(esc(def)) = Core.applicable(Base.get, el) ? Base.get(el) : iv(el)
el
end
end
# ╔═╡ 1954018c-4a07-4ca5-9011-d340ccd9996f
begin
import Pkg
Pkg.activate() # disable Pluto's package management
end
# ╔═╡ 1b8554d8-fd89-11ea-3760-d960e6f55ebd
begin
using SpecialFunctions
x = 0:0.1:15
j0, j1, j2, j3 = [besselj.(order, x) for order in 0:3]
end
# ╔═╡ 05ec2818-fd89-11ea-3da8-23ea406ba0da
begin
ENV["GRDISPLAY"] = "pluto"
using GR
end
# ╔═╡ 6a7c464e-fd8b-11ea-042f-cfbcaddf2d0e
begin
using PlutoUI
@bind xmax Slider(5:15)
end
# ╔═╡ 25f44cb2-fd89-11ea-1bfb-ad13a07d717e
plot(x, j0, x, j1, x, j2, x, j3,
labels=["J_0", "J_1", "J_2", "J_3"], location=11, xlim=(0, xmax))
# ╔═╡ Cell order:
# ╠═1954018c-4a07-4ca5-9011-d340ccd9996f
# ╠═1b8554d8-fd89-11ea-3760-d960e6f55ebd
# ╠═05ec2818-fd89-11ea-3da8-23ea406ba0da
# ╠═6a7c464e-fd8b-11ea-042f-cfbcaddf2d0e
# ╠═25f44cb2-fd89-11ea-1bfb-ad13a07d717e
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 3751 | ### A Pluto.jl notebook ###
# v0.19.0
using Markdown
using InteractiveUtils
# This Pluto notebook uses @bind for interactivity. When running this notebook outside of Pluto, the following 'mock version' of @bind gives bound variables a default value (instead of an error).
macro bind(def, element)
quote
local iv = try Base.loaded_modules[Base.PkgId(Base.UUID("6e696c72-6542-2067-7265-42206c756150"), "AbstractPlutoDingetjes")].Bonds.initial_value catch; b -> missing; end
local el = $(esc(element))
global $(esc(def)) = Core.applicable(Base.get, el) ? Base.get(el) : iv(el)
el
end
end
# ╔═╡ 5323b5ac-0c51-4952-8f27-4f489c1f2d1a
begin
import Pkg
Pkg.activate() # disable Pluto's package management
end
# ╔═╡ d8aeb9f4-990c-11eb-0dbb-078cda4928d5
begin
using GSL # provides the special functions
using GR
using PlutoUI
end
# ╔═╡ 6127fb6a-22fc-4f35-8228-cc06ffebf9c0
begin
a0 = 5.291772109217e-11
# The unitless radial coordinate
ρ(r, n) = 2r/(n*a0)
end
# ╔═╡ 7aa443bd-b706-41e2-bdc8-0d8c10c99b02
# The θ and ϕ dependence
function Yml(m::Int, l::Int, θ::Real, ϕ::Real)
(-1.0)^m*sf_legendre_Plm(l, abs(m), cos(θ))*ℯ^(im*m*ϕ)
end
# ╔═╡ 778c4fa2-68c0-494f-ae22-477189a27f2b
# The Radial dependence
function R(n::Int, l::Int, ρ::Real)
sf_laguerre_n(n-l-1, 2*l+1, ρ)*ℯ^(-ρ/2)*ρ^l
end
# ╔═╡ cf4d7a50-afea-4f10-b08d-0f0150b7da7a
# A normalization: This is dependent on the choice of polynomial representation
function norm(n::Int, l::Int)
sqrt((2/n)^3 * factorial(n-l-1)/(2n*factorial(n+l)))
end
# ╔═╡ d3ff17f0-3dd6-4ffe-a045-5b573aec02a5
# Generates an Orbital Funtion of (r, θ, ϕ) for a specificied n, l, m.
function Orbital(n::Int, l::Int, m::Int)
# we make sure l and m are within proper bounds
if l > n || abs(m) > l
throw(DomainError())
end
Ψ(ρ, θ, ϕ) = norm(n, l) * R(n, l, ρ) * abs(Yml(m, l, θ, ϕ))
Ψ
end
# ╔═╡ 94158386-447f-40cd-a321-8b333053d558
function CarttoSph(x::Array, y::Array, z::Array)
r = sqrt.(x.^2+y.^2+z.^2)
θ = acos.(z./r)
ϕ = atan.(y./x)
r, θ, ϕ
end
# ╔═╡ ad5ffb61-b2cc-499a-87a4-ad71833de766
function calculate_electronic_density(n, l, m)
N = 50
r = 1e-9
s = LinRange(-r, r, N)
x, y, z = meshgrid(s, s, s)
r, θ, ϕ = CarttoSph(x, y, z)
Ψ = Orbital(n, l, m)
Ψv = zeros(Float32, N, N, N)
for i in 1:N
for j in 1:N
for k in 1:N
Ψv[i, j, k] = abs(Ψ(ρ(r[i, j, k], n), θ[i, j, k], ϕ[i, j, k]))
end
end
end
(Ψv.-minimum(Ψv))./(maximum(Ψv).-minimum(Ψv))
end
# ╔═╡ a4c51114-4bf9-4d40-b123-1629a3be54e1
@bind Ψ Select(["2,0,0", "3,0,0", "2,1,0", "3,1,0", "3,1,1", "2,1,1", "3,2,0", "3,2,1", "3,2,2", "4,0,0", "4, 1,0", "4,2,0", "4,2,1", "4,2,2", "4,3,0", "4,3,1", "4,3,2", "4,3,3"], default="3,2,0")
# ╔═╡ 11cfffdf-91d6-4909-bb59-e8d12a95e5e8
@bind ϕ Slider(0:360, default=30)
# ╔═╡ 9899f86c-2fba-4d72-abab-acbef1dc6988
@bind iso Slider(0.1:0.05:0.8, default=0.2)
# ╔═╡ 16697f21-f879-48d1-ad41-f39e1dde5830
begin
n, m, l = parse.(Int, split(Ψ, ','))
Ψv = calculate_electronic_density(n, m, l)
isosurface(Ψv, isovalue=iso, rotation=ϕ)
end
# ╔═╡ Cell order:
# ╠═5323b5ac-0c51-4952-8f27-4f489c1f2d1a
# ╠═d8aeb9f4-990c-11eb-0dbb-078cda4928d5
# ╠═6127fb6a-22fc-4f35-8228-cc06ffebf9c0
# ╠═7aa443bd-b706-41e2-bdc8-0d8c10c99b02
# ╠═778c4fa2-68c0-494f-ae22-477189a27f2b
# ╠═cf4d7a50-afea-4f10-b08d-0f0150b7da7a
# ╠═d3ff17f0-3dd6-4ffe-a045-5b573aec02a5
# ╠═94158386-447f-40cd-a321-8b333053d558
# ╠═ad5ffb61-b2cc-499a-87a4-ad71833de766
# ╠═a4c51114-4bf9-4d40-b123-1629a3be54e1
# ╠═11cfffdf-91d6-4909-bb59-e8d12a95e5e8
# ╠═9899f86c-2fba-4d72-abab-acbef1dc6988
# ╠═16697f21-f879-48d1-ad41-f39e1dde5830
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1070 | using GR
d, h = 200, 1200 # pixel density (= image width) and image height
n, r = 800, 1000 # number of iterations and escape radius (r > 2)
x = range(0, 2, length=d+1)
y = range(0, 2 * h / d, length=h+1)
A, B = x .* pi, y .* pi
C = (.- 8.0im) .* exp.((A' .+ B .* im) .* im) .- 0.7436636774 .+ 0.1318632144im
Z, dZ = zero(C), zero(C)
D = zeros(size(C))
for k in 1:n
M = abs2.(Z) .< abs2(r)
Z[M], dZ[M] = Z[M] .^ 2 .+ C[M], 2 .* Z[M] .* dZ[M] .+ 1
end
N = abs.(Z) .> 2 # exterior distance estimation
D[N] = 0.5 .* log.(abs.(Z[N])) .* abs.(Z[N]) ./ abs.(dZ[N])
X, Y = real(C), imag(C) # zoom images (adjust circle size 120 and zoom level 20 as needed)
R, c, z = 120 .* 2 ./ d .* pi .* exp.(.- ones(d+1)' .* B), min(d, h) + 1, max(0, h - d) ÷ 20
setwsviewport(0, 0.3, 0, 0.3)
setviewport(0, 1, 0, 1)
setwindow(-2.5, 1, -1.6, 1.9)
setcolormap(GR.COLORMAP_UNIFORM)
setborderwidth(0)
setmarkertype(GR.MARKERTYPE_SOLID_CIRCLE)
polymarker(vec(X[1*z+1:1*z+c,:]), vec(Y[1*z+1:1*z+c,:]), vec(R[1*z+1:1*z+c,:]), vec(D[1*z+1:1*z+c,:].^0.5))
updatews()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 571 | using GR
const x = [ 0, 1, 0, 1, 0.707 ]
const y = [ 0, 0, 1, 1, 0.707]
const z = [ 0, 0, 0, 0, 0.707 ]
const connections = [
[ 4, 1, 2, 4, 3 ], # 1 quadrilateral
[ 3, 1, 2, 5 ], # 4 triangles
[ 3, 2, 4, 5 ],
[ 3, 4, 3, 5 ],
[ 3, 3, 1, 5 ]
]
# AABBGGRR
const colors = signed.(UInt32[0xdf753e80, 0xdf0068ff, 0xdfc0a35a, 0xdf2000c1, 0xdf62a2ce])
setviewport(0, 1, 0, 1)
setwindow(-1, 1, -1, 1)
setwindow3d(0, 1, 0, 1, 0, 1)
setspace3d(30, 80, 0, 0)
polygonmesh3d(x, y, z, vcat(connections...), colors)
updatews()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 3481 | ENV["QSG_RENDER_LOOP"] = "basic"
using CxxWrap # for safe_cfunction
using QML
using Observables
using GR
const qmlfile = joinpath(dirname(Base.source_path()), "qml_3d.qml")
rot = -30
tilt = 45
fov = Observable(30)
cam = Observable(0.0)
w, h = (500, 500)
mouse = Nothing
function paint(p::CxxPtr{QPainter}, item::CxxPtr{JuliaPaintedItem})
global rot, tilt
ENV["GKSwstype"] = 381
ENV["GKSconid"] = split(repr(p.cpp_object), "@")[2]
dev = device(p[])[]
r = effectiveDevicePixelRatio(window(item[])[])
w, h = width(dev) / r, height(dev) / r
clearws()
mwidth, mheight, pwidth, pheight = inqdspsize()
if w > h
ratio = float(h) / w
msize = mwidth * w / pwidth
setwsviewport(0, msize, 0, msize * ratio)
setwswindow(0, 1, 0, ratio)
else
ratio = float(w) / h
msize = mheight * h / pheight
setwsviewport(0, msize * ratio, 0, msize)
setwswindow(0, ratio, 0, 1)
end
x = LinRange(-1, 1, 49)
y = LinRange(-1, 1, 49)
z = peaks()
selntran(1)
setwindow(-1, 1, -1, 1)
setviewport(0.05, 0.95, 0.05, 0.95)
setlinewidth(0.5) # should be adjusted internally!
setresamplemethod(0x2020202) # linear
setcharheight(0.016)
settextfontprec(232, 4)
setwindow3d(-1, 1, -1, 1, -10, 10)
setspace3d(rot, tilt, fov[], cam[])
settransparency(0.5)
xtick, ytick, ztick = (0.1, 0.1, 1)
xorg = (-1, 1)
yorg = (-1, 1)
zorg = (-10, 10)
rotation = -rot
while rotation < 0 rotation += 360 end
while tilt < 0 tilt += 360 end
zi = 0 <= tilt <= 90 ? 1 : 2
if 0 <= rotation < 90
grid3d(xtick, 0, ztick, xorg[1], yorg[2], zorg[zi], 2, 0, 2)
grid3d(0, ytick, 0, xorg[1], yorg[2], zorg[zi], 0, 2, 0)
elseif 90 <= rotation < 180
grid3d(xtick, 0, ztick, xorg[2], yorg[2], zorg[zi], 2, 0, 2)
grid3d(0, ytick, 0, xorg[2], yorg[2], zorg[zi], 0, 2, 0)
elseif 180 <= rotation < 270
grid3d(xtick, 0, ztick, xorg[2], yorg[1], zorg[zi], 2, 0, 2)
grid3d(0, ytick, 0, xorg[2], yorg[1], zorg[zi], 0, 2, 0)
else
grid3d(xtick, 0, ztick, xorg[1], yorg[1], zorg[1], 2, 0, 2)
grid3d(0, ytick, 0, xorg[1], yorg[1], zorg[zi], 0, 2, 0)
end
settransparency(0.3)
gr3.surface(x, y, z', 4)
gr3.surface(x, y, z', 1)
settransparency(0.8)
setcharheight(0.016 * 1.5)
titles3d("X title", "Y title", "Z title")
setcharheight(0.016)
if 0 <= rotation < 90
axes3d(xtick, 0, ztick, xorg[1], yorg[1], zorg[zi], 2, 0, 2, -0.01)
axes3d(0, ytick, 0, xorg[2], yorg[1], zorg[zi], 0, 2, 0, 0.01)
elseif 90 <= rotation < 180
axes3d(0, 0, ztick, xorg[1], yorg[2], zorg[zi], 0, 0, 2, -0.01)
axes3d(xtick, ytick, 0, xorg[1], yorg[1], zorg[zi], 2, 2, 0, -0.01)
elseif 180 <= rotation < 270
axes3d(xtick, 0, ztick, xorg[2], yorg[2], zorg[zi], 2, 0, 2, 0.01)
axes3d(0, ytick, 0, xorg[1], yorg[1], zorg[zi], 0, 2, 0, -0.01)
else
axes3d(0, 0, ztick, xorg[2], yorg[1], zorg[zi], 0, 0, 2, -0.01)
axes3d(xtick, ytick, 0, xorg[2], yorg[2], zorg[zi], 2, 2, 0, 0.01)
end
updatews()
return
end
function mousePosition(eventx, eventy, buttons)
global mouse, rot, tilt
if mouse != Nothing
rot += 0.2 * (mouse[1] - eventx)
tilt += 0.2 * (mouse[2] - eventy)
end
if buttons != 0
mouse = [eventx, eventy]
else
mouse = Nothing
end
end
loadqml(qmlfile,
paint_cfunction = @safe_cfunction(paint, Cvoid, (CxxPtr{QPainter}, CxxPtr{JuliaPaintedItem})),
parameters = JuliaPropertyMap("fov" => fov, "cam" => cam))
@qmlfunction mousePosition
exec()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1545 | ENV["QSG_RENDER_LOOP"] = "basic"
using CxxWrap # for safe_cfunction
using QML
using Observables
using GR
const qmlfile = joinpath(dirname(Base.source_path()), "qml_ex.qml")
x = randn(1000000)
y = randn(1000000)
nbins = Observable(30)
w, h = (600, 450)
zoom = Nothing
# Arguments here need to be the "reference types", hence the "Ref" suffix
function paint(p::CxxPtr{QPainter}, item::CxxPtr{JuliaPaintedItem})
global w, h
global zoom
ENV["GKSwstype"] = 381
ENV["GKSconid"] = split(repr(p.cpp_object), "@")[2]
dev = device(p[])[]
r = effectiveDevicePixelRatio(window(item[])[])
w, h = width(dev) / r, height(dev) / r
plt = kvs()
plt[:size] = (w, h)
if zoom === Nothing
xmin, xmax, ymin, ymax = (-5, 5, -5, 5)
elseif zoom != 0
xmin, xmax, ymin, ymax = panzoom(0, 0, zoom)
else
xmin, xmax, ymin, ymax = inqwindow()
end
num_bins = Int64(round(nbins[]))
hexbin(x, y, nbins=num_bins, xlim=(xmin, xmax), ylim=(ymin, ymax), title="nbins: $num_bins")
return
end
function mousePosition(eventx, eventy, deltay)
global zoom
if deltay != 0
zoom = deltay < 0 ? 1.02 : 1/1.02
else
zoom = 0
end
if w > h
xn = eventx / w
yn = (h - eventy) / w
else
xn = eventx / h
yn = (h - eventy) / h
end
x, y = ndctowc(xn, yn)
"($(round(x,digits=4)), $(round(y,digits=4)))"
end
loadqml(qmlfile,
paint_cfunction = @safe_cfunction(paint, Cvoid, (CxxPtr{QPainter}, CxxPtr{JuliaPaintedItem})),
parameters = JuliaPropertyMap("nbins" => nbins))
@qmlfunction mousePosition
exec()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1182 | ENV["QSG_RENDER_LOOP"] = "basic"
using CxxWrap # for safe_cfunction
using QML
using Observables
using Plots
import GR
ENV["GKSwstype"] = "use_default"
gr(show=true)
const qmlfile = joinpath(dirname(Base.source_path()), "qml_ex.qml")
nbins = Observable(30)
w, h = (600, 450)
# Arguments here need to be the "reference types", hence the "Ref" suffix
function paint(p::CxxPtr{QPainter}, item::CxxPtr{JuliaPaintedItem})
global w, h
ENV["GKS_WSTYPE"] = 381
ENV["GKS_CONID"] = split(repr(p.cpp_object), "@")[2]
dev = device(p[])[]
r = effectiveDevicePixelRatio(window(item[])[])
w, h = width(dev) / r, height(dev) / r
num_bins = Int64(round(nbins[]))
histogram(randn(10000), nbins=num_bins, size=(w, h))
return
end
function mousePosition(eventx, eventy, deltay)
if w > h
xn = eventx / w
yn = (h - eventy) / w
else
xn = eventx / h
yn = (h - eventy) / h
end
x, y = GR.ndctowc(xn, yn)
"($(round(x,digits=4)), $(round(y,digits=4)))"
end
loadqml(qmlfile,
paint_cfunction = @safe_cfunction(paint, Cvoid, (CxxPtr{QPainter}, CxxPtr{JuliaPaintedItem})),
parameters = JuliaPropertyMap("nbins" => nbins))
@qmlfunction mousePosition
exec()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 323 | using GR
function tα_qubit(β, ψ1, ψ2, fα, f)
2 + 2 * β - cos(ψ1) - cos(ψ2) - 2 * β * cos(π * fα) * cos(2 * π * f + π * fα - ψ1 - ψ2)
end
ψ1 = ψ2 = range(0, 4 * π, length=100)
z = [tα_qubit(0.61, x, y, 0.2, 0.1) for x in ψ1, y in ψ2]
contour(ψ1, ψ2, z, levels=20, xlabel="ψ1", ylabel="ψ2", xlim=(0, 4π), ylim=(0, 4π))
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 763 | import GR
import Random
srand(seed) = Random.seed!(seed)
function main()
srand(37)
y = randn(20, 500)
GR.setviewport(0.1, 0.95, 0.1, 0.95)
GR.setcharheight(0.020)
GR.settextcolorind(82)
GR.setfillcolorind(90)
GR.setfillintstyle(1)
for x in 1:5000
GR.clearws()
GR.setwindow(x, x+500, -200, 200)
GR.fillrect(x, x+500, -200, 200)
GR.setlinecolorind(0); GR.grid(50, 50, 0, -200, 2, 2)
GR.setlinecolorind(82); GR.axes(50, 50, x, -200, 2, 2, -0.005)
y = hcat(y, randn(20))
for i in 1:20
GR.setlinecolorind(980 + i)
s = cumsum(reshape(y[i,:], x+500))
GR.polyline([x:x+500;], s[x:x+500])
end
GR.updatews()
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 2231 | #!/usr/bin/env julia
# A view of the Riemann Zeta function using the domain coloring method
import GR
import SpecialFunctions
function domain_colors(w, n)
H = mod.(angle.(w[:]) / 2pi .+ 1, 1)
m = 0.7
M = 1
isol = m .+ (M - m) * (H[:] * n - floor.(H[:] * n))
modul = abs.(w[:])
Logm = log.(modul[:])
modc = m .+ (M - m) * (Logm[:] - floor.(Logm[:]))
V = [modc[i] * isol[i] for i = 1:length(modc)]
S = 0.9 .* fill!(similar(H), 1)
HSV = cat(H, S, V, dims=2)
return HSV
end
function func_vals(f, re, im, N)
# evaluates the complex function at the nodes of the grid
# re and im are tuples defining the rectangular region
# N is the number of nodes per unit interval
l = re[2] - re[1]
h = im[2] - im[1]
resL = N * l # horizontal resolution
resH = N * h # vertical resolution
x = LinRange(re[1], re[2], resL)
y = LinRange(im[1], im[2], resH)
z = complex.(x', y)
w = f.(z)
return w
end
function plot_domain(color_func, f; re=[-1, 1], im=[-1, 1], N=100, n=15)
w = func_vals(f, re, im, N)
domc = color_func(w, n) * 255
h = round.(domc[:,1])
s = round.(domc[:,2])
v = round.(domc[:,3])
alpha = 255
width, height = size(w)
c = Array{UInt32, 1}(undef, width * height)
c = h .+ 256 * (s .+ 256 * (v .+ 256 * alpha))
c = rotr90(reshape(c, width, height))
GR.clearws()
GR.setviewport(0.3725, 0.6275, 0.1, 0.95)
GR.setwindow(-6, 6, -20, 20)
GR.drawimage(-6, 6, -20, 20, height, width, c, GR.MODEL_HSV)
GR.settextalign(GR.TEXT_HALIGN_CENTER, GR.TEXT_VALIGN_HALF)
GR.setcharheight(0.018)
GR.mathtex(0.825, 0.575, "\\zeta \\left({s}\\right) := \\sum_{n=1}^\\infty \\frac{1}{n^s} \\quad \\sigma = \\Re(s) > 1")
GR.mathtex(0.825, 0.475, "\\zeta \\left({s}\\right) := \\frac{1}{\\Gamma(s)} \\int_{0}^\\infty \\frac{x^{s-1}}{e^x-1} dx")
GR.axes(1, 1, -6, -20, 3, 10, -0.005)
GR.setcharheight(0.024)
GR.mathtex(0.5, 0.975, "\\zeta \\left({s}\\right)")
GR.mathtex(0.5, 0.025, "\\Re(z)")
GR.mathtex(0.3, 0.525, "\\Im(z)")
GR.updatews()
end
f = SpecialFunctions.zeta
for n = 5:30
plot_domain(domain_colors, f, re=(-6, 6), im=(-20, 20), N=15, n=n)
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 319 | using GR
using DelimitedFiles
function main()
z = Z = readdlm("sans.dat", skipstart=3)
G = [ exp(-x^2 -y^2) for x in LinRange(-1.5, 1.5, 128), y in LinRange(-1.5, 1.5, 128) ]
for t = 0:500
surface(z, title="Time: $t s")
z += 0.05 * Z .* G .* rand(Float64, (128, 128))
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 582 | using GR
const hello = Dict(
"Chinese" => "你好世界",
"Dutch" => "Hallo wereld",
"English" => "Hello world",
"French" => "Bonjour monde",
"German" => "Hallo Welt",
"Greek" => "γειά σου κόσμος",
"Italian" => "Ciao mondo",
"Japanese" => "こんにちは世界",
"Korean" => "여보세요 세계",
"Portuguese" => "Olá mundo",
"Russian" => "Здравствуй, мир",
"Spanish" => "Hola mundo"
)
function say_hello()
y = 0.9
for (lang, trans) in hello
text(0.1, y, lang)
text(0.4, y, trans)
y -= 0.072
end
updatews()
end
say_hello()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1278 | #!/usr/bin/env julia
import Random
srand(seed) = Random.seed!(seed)
import GR
function draw(selection, x_offset, y_offset)
GR.clearws()
srand(0)
xd = -2 .+ 4 * rand(100)
yd = -2 .+ 4 * rand(100)
zd = [xd[i] * exp(-xd[i]^2 - yd[i]^2) for i = 1:100]
GR.setviewport(0.1, 0.95, 0.1, 0.95)
GR.setwindow(-2, 2, -2, 2)
GR.setmarkersize(1)
GR.setmarkertype(GR.MARKERTYPE_SOLID_CIRCLE)
GR.setcharheight(0.024)
GR.settextalign(2, 0)
GR.settextfontprec(3, 0)
x, y, z = GR.gridit(xd, yd, zd, 200, 200)
h = -0.6:0.05:0.6
GR.contourf(x, y, h, z, 2)
GR.polymarker(xd, yd)
if selection > 0
GR.beginselection(selection, 0)
end
GR.axes(0.25, 0.25, -2, -2, 2, 2, 0.01)
if selection > 0
GR.endselection()
GR.moveselection(x_offset, y_offset)
end
GR.updatews()
end
function main()
mouse = Nothing
x_offset = y_offset = 0
draw(0, 0, 0)
while true
x, y, buttons = GR.samplelocator()
if mouse != Nothing && buttons != 0
x_offset += x - mouse[1]
y_offset += y - mouse[2]
end
mouse = [x, y]
draw(1, x_offset, y_offset)
if buttons & 0x02 != 0
break
end
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 950 | using GR
const viridis = [0x440154, 0x472c7a, 0x3b518b, 0x2c718e, 0x21908d, 0x27ad81, 0x5cc863, 0xaadc32, 0xfde725]
const inferno = [0x000004, 0x1f0c48, 0x550f6d, 0x88226a, 0xa83655, 0xe35933, 0xf9950a, 0xf8c932, 0xfcffa4]
const plasma = [0x0c0887, 0x4b03a1, 0x7d03a8, 0xa82296, 0xcb4679, 0xe56b5d, 0xf89441, 0xfdc328, 0xf0f921]
const magma = [0x000004, 0x1c1044, 0x4f127b, 0x812581, 0xb5367a, 0xe55964, 0xfb8761, 0xfec287, 0xfbfdbf]
red(a) = ((a .>> 16) .& 0xff ) ./ 256
green(a) = ((a .>> 8) .& 0xff ) ./ 256
blue(a) = ( a .& 0xff ) ./ 256
function show_colormaps()
setwindow(1, 5, 0, 1)
for (x, cmap) in enumerate((viridis, inferno, plasma, magma))
setcolormapfromrgb(red(cmap), green(cmap), blue(cmap))
cellarray(x, x + 0.25, 0, 1, 1, 255, 1000:1255)
setcolormap(GR.COLORMAP_VIRIDIS + x - 1)
cellarray(x + 0.5, x + 0.75, 0, 1, 1, 255, 1000:1255)
end
updatews()
end
show_colormaps()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 580 | using Distributions
function dists(specs; N=50)
X = Float64[]
Y = Float64[]
for (x, y, σ) in specs
xd = rand(Normal(x, σ), N)
yd = rand(Normal(y, σ), N)
append!(X, xd)
append!(Y, yd)
end
X, Y
end
N = 1000000
x, y = dists([(2,2,0.02), (2,-2,0.1), (-2,-2,0.5), (-2,2,1.0), (0,0,3.0)], N=N)
println("# of points: ", length(x))
using GR
setviewport(0.1, 0.95, 0.1, 0.95)
setwindow(-10, 10, -10, 10)
setcharheight(0.02)
axes2d(0.5, 0.5, -10, -10, 4, 4, -0.005)
setcolormap(GR.COLORMAP_HOT)
@time shadepoints(x, y, xform=GR.XFORM_EQUALIZED)
updatews()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 190 | using WAV
using GR
file = joinpath(dirname(Base.find_package("GR")), "..", "examples", "Monty_Python.wav")
y, fs = WAV.wavread(file)
shade(y, colormap=-GR.COLORMAP_BLUESCALE, ylim=(-1,1))
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1111 | #!/usr/bin/env julia
# Rendering slices and an isosurface of MRI data.
import GR
const gr3 = GR.gr3
function draw(mesh::Cint; x::Union{Real, Nothing}=nothing, y::Union{Real, Nothing}=nothing, z::Union{Real, Nothing}=nothing)
gr3.clear()
gr3.drawmesh(mesh, 1, (0,0,0), (0,0,1), (0,1,0), (1,1,1), (1,1,1))
gr3.drawslicemeshes(data, x=x, y=y, z=z)
GR.clearws()
gr3.drawimage(0, 1, 0, 1, 500, 500, gr3.DRAWABLE_GKS)
GR.updatews()
end
data = open(stream -> read!(stream, Array{UInt16}(undef, 93, 64, 64)), "mri.raw")
data = min.(data, 2000) / 2000.0 * typemax(UInt16)
data = convert(Array{UInt16, 3}, floor.(data))
data = permutedims(data, [3, 2, 1])
GR.setviewport(0, 1, 0, 1)
gr3.cameralookat(-3, 2, -2, 0, 0, 0, 0, 0, -1)
mesh = gr3.createisosurfacemesh(data, (2.0/63, 2.0/63, 2.0/92), (-1.0, -1.0, -1.0), 40000)
GR.setcolormap(1)
for z in 0:0.005:1
draw(mesh, x=0.9, z=z)
end
for y in 1:-0.0025:0.5
draw(mesh, x=0.9, y=y, z=1)
end
GR.setcolormap(19)
for x in 0.9:-0.003:0
draw(mesh, x=x, y=0.5, z=1)
end
for x in 0:0.003:0.9
draw(mesh, x=x, z=1)
end
gr3.terminate()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 3129 | using Random
rng = MersenneTwister(1234)
import Plots
const GR = Plots.GR
x = 0:π/100:2π
y = sin.(x)
GR.plot(x, y)
x = LinRange(0, 1, 51)
y = x .- x.^2
GR.scatter(x, y)
sz = LinRange(0.5, 3, length(x))
c = LinRange(0, 255, length(x))
GR.scatter(x, y, sz, c)
GR.stem(x, y)
GR.histogram(randn(rng, 10000))
GR.plot(randn(rng, 50))
GR.oplot(randn(rng, 50, 3))
x = LinRange(0, 30, 1000)
y = cos.(x) .* x
z = sin.(x) .* x
GR.plot3(x, y, z)
angles = LinRange(0, 2pi, 40)
radii = LinRange(0, 2, 40)
GR.polar(angles, radii)
x = 2 .* rand(rng, 100) .- 1
y = 2 .* rand(rng, 100) .- 1
z = 2 .* rand(rng, 100) .- 1
GR.scatter3(x, y, z)
c = 999 .* rand(rng, 100) .+ 1
GR.scatter3(x, y, z, c)
x = randn(rng, 100000)
y = randn(rng, 100000)
GR.hexbin(x, y)
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
GR.contour(x, y, z)
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
GR.contour(x, y, z)
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
GR.contourf(x, y, z)
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
GR.contourf(x, y, z)
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) + cos.(y)
GR.tricont(x, y, z)
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
GR.surface(x, y, z)
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
GR.surface(x, y, z)
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
GR.trisurf(x, y, z)
z = GR.peaks()
GR.surface(z)
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
GR.wireframe(x, y, z)
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
GR.heatmap(z)
GR.imshow(z)
s = LinRange(-1, 1, 40)
v = 1 .- (s .^ 2 .+ (s .^ 2)' .+ reshape(s,1,1,:) .^ 2) .^ 0.5
GR.isosurface(v, isovalue=0.2)
GR.GR3.terminate()
GR.volume(randn(rng, 50, 50, 50))
N = 1_000_000
x = randn(rng, N)
y = randn(rng, N)
GR.shade(x, y)
GR.setprojectiontype(0)
GR.clearws()
xd = -2 .+ 4 * rand(rng, 100)
yd = -2 .+ 4 * rand(rng, 100)
zd = [xd[i] * exp(-xd[i]^2 - yd[i]^2) for i = 1:100]
GR.setviewport(0.1, 0.95, 0.1, 0.70)
GR.setwindow(-2, 2, -2, 2)
GR.setspace(-0.5, 0.5, 0, 90)
GR.setcolormap(0)
GR.setlinecolorind(1)
GR.setmarkersize(1)
GR.setmarkertype(-1)
GR.setmarkercolorind(1)
GR.setcharheight(0.024)
GR.settextalign(2, 0)
GR.settextfontprec(3, 0)
x, y, z = GR.gridit(xd, yd, zd, 200, 200)
h = -0.5:0.05:0.5
GR.surface(x, y, z, 5)
GR.contour(x, y, h, z, 0)
GR.polymarker(xd, yd)
GR.axes(0.25, 0.25, -2, -2, 2, 2, 0.01)
GR.updatews()
sleep(3)
GR.emergencyclosegks()
Plots.gr(show=true)
x = 1:10; y = rand(rng, 10);
Plots.plot(x,y)
Plots.scatter(x,y)
Plots.histogram(randn(rng, 10000))
z = GR.peaks();
Plots.surface(z)
Plots.contour(z)
x = LinRange(0, 30, 1000); y = cos.(x) .* x; z = sin.(x) .* x
Plots.plot3d(x, y, z)
import StatsPlots
y = rand(rng, 100, 4)
StatsPlots.violin(["Series 1" "Series 2" "Series 3" "Series 4"], y, leg = false)
StatsPlots.boxplot!(["Series 1" "Series 2" "Series 3" "Series 4"], y, leg = false)
sleep(3)
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 3019 | using Random
rng = MersenneTwister(1234)
using GR
x = 0:π/100:2π
y = sin.(x)
plot(x, y)
x = LinRange(0, 1, 51)
y = x .- x.^2
scatter(x, y)
sz = LinRange(0.5, 3, length(x))
c = LinRange(0, 255, length(x))
scatter(x, y, sz, c)
stem(x, y)
histogram(randn(rng, 10000))
plot(randn(rng, 50))
oplot(randn(rng, 50, 3))
x = LinRange(0, 30, 1000)
y = cos.(x) .* x
z = sin.(x) .* x
plot3(x, y, z)
angles = LinRange(0, 2pi, 40)
radii = LinRange(0, 2, 40)
polar(angles, radii)
x = 2 .* rand(rng, 100) .- 1
y = 2 .* rand(rng, 100) .- 1
z = 2 .* rand(rng, 100) .- 1
scatter3(x, y, z)
c = 999 .* rand(rng, 100) .+ 1
scatter3(x, y, z, c)
x = randn(rng, 100000)
y = randn(rng, 100000)
hexbin(x, y)
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
contour(x, y, z)
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
contour(x, y, z)
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
contourf(x, y, z)
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
contourf(x, y, z)
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) + cos.(y)
tricont(x, y, z)
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
surface(x, y, z)
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
surface(x, y, z)
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
trisurf(x, y, z)
z = peaks()
surface(z)
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
wireframe(x, y, z)
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
wireframe(x, y, z)
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
heatmap(z)
imshow(z)
if !haskey(ENV, "GRDISPLAY")
ρ = LinRange(0, 7, 200)
θ = LinRange(0, 2π, 360)
polarheatmap(θ, ρ, sin.(2ρ) .* cos.(θ'))
end
s = LinRange(-1, 1, 40)
v = 1 .- (s .^ 2 .+ (s .^ 2)' .+ reshape(s, 1, 1, :) .^ 2) .^ 0.5
isosurface(v, isovalue=0.2)
volume(randn(rng, 50, 50, 50))
N = 1_000_000
x = randn(rng, N)
y = randn(rng, N)
shade(x, y)
if !haskey(ENV, "GRDISPLAY")
setprojectiontype(0)
clearws()
xd = -2 .+ 4 * rand(rng, 100)
yd = -2 .+ 4 * rand(rng, 100)
zd = [xd[i] * exp(-xd[i]^2 - yd[i]^2) for i = 1:100]
setwsviewport(0, 0.1, 0, 0.1)
setwswindow(0, 1, 0, 1)
setviewport(0.1, 0.95, 0.1, 0.95)
setwindow(-2, 2, -2, 2)
setspace(-0.5, 0.5, 0, 90)
setcolormap(0)
setlinecolorind(1)
setmarkersize(1)
setmarkertype(-1)
setmarkercolorind(1)
setcharheight(0.024)
settextalign(2, 0)
settextfontprec(3, 0)
x, y, z = gridit(xd, yd, zd, 200, 200)
h = -0.5:0.05:0.5
surface(x, y, z, 5)
contour(x, y, h, z, 0)
polymarker(xd, yd)
x_axis = axis('X', tick=0.25, org=-2, major_count=2, tick_size=0.01)
y_axis = axis('Y', tick=0.25, org=-2, major_count=2, tick_size=0.01)
drawaxes(x_axis, y_axis, GR.AXES_SIMPLE_AXES)
updatews()
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 908 | import PortAudio, FileIO
using GR
using FFTW
function play(c::Channel)
data, = FileIO.load("Monty_Python.wav")
stream = PortAudio.PortAudioStream(0, 1)
spectrum = zeros(Int32, 300, 225)
offset = 1
while offset + 1024 < length(data)
amplitudes = data[offset:offset+1024]
offset += 1024
PortAudio.write(stream, amplitudes)
power = log.(abs.(fft(float(amplitudes))) .+ 1) * 50
spectrum[1, :] = round.(Int, power[1:225])
spectrum = circshift(spectrum, [-1, 0])
put!(c, spectrum')
end
put!(c, nothing)
end
function main()
c = Channel(0)
task = @task play(c::Channel)
bind(c, task)
schedule(task)
start = time_ns()
while isopen(c)
spectrum = take!(c)
if spectrum === nothing
break
end
if time_ns() - start > 20 * 1000000 # 20ms
imshow(spectrum, colormap=-13, yflip=true)
start = time_ns()
end
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 425 | # Plot a real-time spectrogram
# see https://github.com/JuliaAudio/PortAudio.jl
using GR, PortAudio, SampledSignals, FFTW
const N = 1024
const stream = PortAudioStream(1, 0)
const buf = read(stream, N)
const fmin = 0Hz
const fmax = 10000Hz
const fs = Float32[float(f) for f in domain(fft(buf)[fmin..fmax])]
while true
read!(stream, buf)
plot(fs, abs.(fft(buf)[fmin..fmax]), xlim=(fs[1],fs[end]), ylim=(0,100))
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1680 | using LinearAlgebra
import GR
const GR3 = GR.gr3
rate = 100
const μ0 = [0.03, 0., 0.09]
const Β = [0., 0., 0.8]
const γ = 2.0
const η = 0.1
const ΔT = 0.001
function calcualate_magnetic_moment(c::Channel)
μ = μ0
while true
put!(c, μ)
P = - γ * μ × Β # precession
G = η * (μ / norm(μ)) × P # Gilbert damping
dμ = (P + G) * ΔT
if norm(dμ) < 1e-6
break
end
μ += dμ
end
put!(c, nothing)
end
function calculate_cone_length(radius, cone_angle)
2 * radius / tand(cone_angle)
end
function drawarrowmesh(start, end_, color, radius, cone_angle=20)
direction = end_ - start
length = norm(direction)
cone_length = calculate_cone_length(radius, cone_angle)
cylinder_length = length - cone_length
direction /= length
cone_start = start + cylinder_length * direction
if cylinder_length > 0
GR3.drawcylindermesh(1, start, direction, color, [radius], [cylinder_length])
end
GR3.drawconemesh(1, cone_start, direction, color, [2 * radius], [cone_length])
end
function main()
c = Channel(calcualate_magnetic_moment)
i = 0
GR.setviewport(0, 1, 0, 1)
GR3.setbackgroundcolor(1, 1, 1, 1)
while isopen(c)
magmom = take!(c)
if magmom === nothing
break
end
i += 1
if i % rate != 0
continue
end
point = 5 * magmom / norm(magmom)
GR.clearws()
GR3.clear()
GR3.drawspheremesh(1, [0, 0, 0], [0, 0.75, 0.75], [1])
drawarrowmesh([0, 0, 0], point, [1, 0, 0], 0.1)
GR3.drawspheremesh(1, point, [0, 1, 0], [0.2])
GR3.cameralookat(10, 0, 10, 0, 0, 0, 0, 0, 1)
GR3.drawimage(0, 1, 0, 1, 500, 500, GR3.DRAWABLE_GKS)
GR.updatews()
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 528 | using Plots, Test
@info("Interactive tests")
Plots.test_examples(:gr, disp=true)
@info("Figure output tests")
prefix = tempname()
@time for i ∈ 1:length(Plots._examples)
i ∈ Plots._backend_skips[:gr] && continue # skip unsupported examples
Plots._examples[i].imports ≡ nothing || continue # skip examples requiring optional test deps
pl = Plots.test_examples(:gr, i; disp = false)
for ext in (".png", ".pdf", ".svg")
fn = string(prefix, i, ext)
Plots.savefig(pl, fn)
@test filesize(fn) > 1_000
end
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 112808 | using GR
paths = [
[0xffffff, 0, [0.12949, 0.12949, 0.12966, 0.12829, 0.12692, 0.09949, 0.06528, 0.06528, 0.09492, 0.12949], [0.52619, 0.52619, 0.52304, 0.52307, 0.5231, 0.60322, 0.59949, 0.59949, 0.61174, 0.52619], "MCCCZ"],
[0xffffff, 0, [0.13538, 0.13538, 0.13446, 0.13318, 0.1319, 0.13337, 0.09993, 0.09993, 0.13197, 0.13538], [0.53123, 0.53123, 0.5282, 0.5287, 0.5292, 0.61387, 0.622, 0.622, 0.62344, 0.53123], "MCCCZ"],
[0xffffff, 0, [0.18119, 0.18119, 0.18392, 0.18314, 0.18235, 0.1004, 0.08464, 0.08464, 0.09077, 0.18119], [0.46068, 0.46068, 0.45908, 0.45796, 0.45683, 0.47816, 0.44757, 0.44757, 0.47905, 0.46068], "MCCCZ"],
[0xffffff, 0, [0.17651, 0.17651, 0.1796, 0.17922, 0.17883, 0.09432, 0.089, 0.089, 0.08491, 0.17651], [0.44366, 0.44366, 0.443, 0.44169, 0.44037, 0.4348, 0.4008, 0.4008, 0.4326, 0.44366], "MCCCZ"],
[0xffffff, 0, [0.16949, 0.16949, 0.17246, 0.17188, 0.1713, 0.08689, 0.07667, 0.07667, 0.07726, 0.16949], [0.45287, 0.45287, 0.45177, 0.45052, 0.44928, 0.45609, 0.42323, 0.42323, 0.45529, 0.45287], "MCCCZ"],
[0xffffff, 0, [0.15165, 0.15165, 0.15383, 0.15277, 0.1517, 0.0788, 0.05522, 0.05522, 0.06979, 0.15165], [0.48321, 0.48321, 0.48092, 0.48006, 0.47919, 0.52228, 0.49721, 0.49721, 0.52578, 0.48321], "MCCCZ"],
[0xffffff, 0, [0.13908, 0.13908, 0.1415, 0.14054, 0.13958, 0.06227, 0.04168, 0.04168, 0.05292, 0.13908], [0.47623, 0.47623, 0.4742, 0.47321, 0.47223, 0.5068, 0.47922, 0.47922, 0.50926, 0.47623], "MCCCZ"],
[0xffffff, 0, [0.13474, 0.13474, 0.13742, 0.13661, 0.13579, 0.05449, 0.03785, 0.03785, 0.04489, 0.13474], [0.46944, 0.46944, 0.46776, 0.46666, 0.46555, 0.48926, 0.45913, 0.45913, 0.49042, 0.46944], "MCCCZ"],
[0xffffff, 0, [0.15263, 0.15263, 0.1545, 0.15334, 0.15217, 0.08538, 0.05879, 0.05879, 0.0769, 0.15263], [0.46842, 0.46842, 0.46587, 0.46514, 0.46442, 0.5165, 0.49466, 0.49466, 0.52112, 0.46842], "MCCCZ"],
[0xffffff, 0, [0.11967, 0.11967, 0.12067, 0.11933, 0.118, 0.07033, 0.03833, 0.03833, 0.06367, 0.11967], [0.51667, 0.51667, 0.51367, 0.51333, 0.513, 0.583, 0.57033, 0.57033, 0.59, 0.51667], "MCCCZ"],
[0xffffff, 0, [0.12082, 0.12082, 0.12245, 0.12122, 0.11999, 0.05835, 0.02983, 0.02983, 0.05033, 0.12082], [0.50504, 0.50504, 0.50232, 0.50171, 0.50109, 0.55917, 0.5399, 0.5399, 0.56456, 0.50504], "MCCCZ"],
[0xffffff, 0, [0.12063, 0.12063, 0.1225, 0.12134, 0.12017, 0.05339, 0.02679, 0.02679, 0.0449, 0.12063], [0.49775, 0.49775, 0.4952, 0.49448, 0.49375, 0.54583, 0.52399, 0.52399, 0.55046, 0.49775], "MCCCZ"],
[0xffffff, 0, [0.11695, 0.11779, 0.11944, 0.12233, 0.12233, 0.11633, 0.131, 0.131, 0.13033, 0.133, 0.133, 0.13967, 0.14767, 0.15414, 0.16868, 0.18511, 0.18511, 0.21367, 0.20833, 0.20833, 0.20767, 0.20167, 0.20167, 0.221, 0.205, 0.19767, 0.19767, 0.24033, 0.21433, 0.19767, 0.19767, 0.23033, 0.21833, 0.213, 0.213, 0.285, 0.23367, 0.23367, 0.247, 0.25433, 0.25433, 0.26567, 0.26433, 0.26433, 0.22967, 0.22367, 0.22367, 0.23767, 0.23233, 0.233, 0.233, 0.23967, 0.239, 0.239, 0.271, 0.25167, 0.25167, 0.25167, 0.277, 0.26633, 0.26633, 0.283, 0.27633, 0.27633, 0.275, 0.28233, 0.28233, 0.309, 0.299, 0.299, 0.29767, 0.30567, 0.30567, 0.30633, 0.32167, 0.32167, 0.31967, 0.341, 0.34767, 0.34767, 0.35233, 0.35167, 0.35167, 0.37633, 0.365, 0.365, 0.39033, 0.385, 0.385, 0.37233, 0.375, 0.375, 0.403, 0.405, 0.405, 0.40167, 0.41967, 0.41967, 0.429, 0.42433, 0.42433, 0.43767, 0.43633, 0.43633, 0.444, 0.44867, 0.44867, 0.45167, 0.45433, 0.45433, 0.461, 0.463, 0.463, 0.46567, 0.45367, 0.45367, 0.455, 0.45033, 0.45033, 0.473, 0.461, 0.461, 0.47967, 0.48167, 0.48167, 0.459, 0.47367, 0.47367, 0.465, 0.495, 0.495, 0.46833, 0.49767, 0.49767, 0.511, 0.49834, 0.49834, 0.47433, 0.511, 0.511, 0.53034, 0.53167, 0.53167, 0.515, 0.50767, 0.50767, 0.52167, 0.59034, 0.59034, 0.60167, 0.609, 0.609, 0.63034, 0.649, 0.649, 0.65567, 0.65434, 0.65434, 0.66567, 0.66434, 0.66434, 0.68634, 0.68833, 0.68833, 0.69967, 0.70034, 0.70034, 0.71567, 0.71234, 0.71234, 0.727, 0.72767, 0.73167, 0.735, 0.735, 0.73767, 0.73634, 0.735, 0.76967, 0.77767, 0.781, 0.781, 0.791, 0.78834, 0.78834, 0.797, 0.79767, 0.79767, 0.80434, 0.79634, 0.79634, 0.80367, 0.80567, 0.80567, 0.80567, 0.81767, 0.81767, 0.81767, 0.825, 0.82834, 0.82834, 0.80767, 0.83834, 0.83834, 0.85034, 0.84434, 0.83834, 0.83167, 0.83967, 0.83967, 0.841, 0.83767, 0.83434, 0.83967, 0.83967, 0.83967, 0.84767, 0.839, 0.839, 0.84967, 0.82967, 0.82967, 0.83434, 0.82767, 0.82767, 0.841, 0.84567, 0.84567, 0.845, 0.83967, 0.83967, 0.80367, 0.83767, 0.83767, 0.85742, 0.84675, 0.84675, 0.83157, 0.83286, 0.11695], [0.49489, 0.48481, 0.47386, 0.46866, 0.46866, 0.448, 0.426, 0.426, 0.414, 0.40866, 0.40866, 0.39466, 0.39333, 0.39225, 0.38714, 0.3848, 0.3848, 0.36133, 0.34, 0.34, 0.31266, 0.31, 0.31, 0.32867, 0.30066, 0.26933, 0.26933, 0.30533, 0.27466, 0.23133, 0.23133, 0.262, 0.248, 0.23333, 0.23333, 0.27866, 0.22933, 0.22933, 0.23533, 0.23066, 0.23066, 0.23266, 0.23, 0.23, 0.21266, 0.182, 0.182, 0.19866, 0.18066, 0.16133, 0.16133, 0.19733, 0.13466, 0.13466, 0.16466, 0.13, 0.102, 0.102, 0.12933, 0.108, 0.108, 0.12266, 0.09733, 0.09733, 0.08, 0.09867, 0.09867, 0.14966, 0.106, 0.106, 0.074, 0.09867, 0.09867, 0.08133, 0.06933, 0.06933, 0.154, 0.094, 0.06667, 0.06667, 0.082, 0.09066, 0.09066, 0.118, 0.07733, 0.07733, 0.11533, 0.09333, 0.09333, 0.06667, 0.05867, 0.05867, 0.11667, 0.11933, 0.11933, 0.04866, 0.10867, 0.10867, 0.08867, 0.08133, 0.08133, 0.09466, 0.1, 0.1, 0.11367, 0.091, 0.091, 0.07533, 0.08066, 0.08066, 0.04066, 0.07867, 0.07867, 0.10133, 0.12066, 0.12066, 0.126, 0.13266, 0.13266, 0.09667, 0.14466, 0.14466, 0.13133, 0.13133, 0.13133, 0.17, 0.162, 0.162, 0.17933, 0.15933, 0.15933, 0.186, 0.17, 0.17, 0.15933, 0.176, 0.176, 0.20266, 0.17266, 0.17266, 0.14533, 0.14067, 0.14067, 0.18933, 0.194, 0.194, 0.25467, 0.22866, 0.22866, 0.2, 0.23066, 0.23066, 0.24133, 0.19533, 0.19533, 0.218, 0.22266, 0.22266, 0.22066, 0.22266, 0.22266, 0.21533, 0.21667, 0.21667, 0.20533, 0.21133, 0.21133, 0.20667, 0.21266, 0.21266, 0.18667, 0.18066, 0.204, 0.19933, 0.19933, 0.212, 0.214, 0.216, 0.20266, 0.168, 0.154, 0.154, 0.17867, 0.18533, 0.18533, 0.184, 0.17667, 0.17667, 0.21533, 0.22533, 0.22533, 0.22667, 0.22066, 0.23266, 0.23266, 0.23133, 0.23533, 0.23533, 0.242, 0.234, 0.234, 0.29266, 0.26066, 0.26066, 0.24266, 0.274, 0.30533, 0.308, 0.30867, 0.30867, 0.31467, 0.31733, 0.32, 0.31733, 0.31733, 0.31733, 0.31067, 0.34733, 0.34733, 0.34466, 0.39333, 0.39333, 0.39733, 0.41133, 0.41133, 0.404, 0.40666, 0.40666, 0.40933, 0.416, 0.416, 0.50733, 0.47066, 0.47066, 0.44808, 0.48608, 0.48608, 0.5261, 0.53326, 0.49489], "MCCCCCCCCLCLCLCCCCCLCCLCCCCCCCLCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCLLCCLCCCCLCCCCCCCCCCCCCCCLZ"],
[0xcc7226, 0, [0.83286, 0.83391, 0.83758, 0.83967, 0.83967, 0.851, 0.84234, 0.84234, 0.827, 0.84167, 0.84167, 0.85167, 0.84634, 0.83989, 0.83567, 0.83567, 0.83567, 0.855, 0.81034, 0.825, 0.825, 0.79234, 0.75634, 0.743, 0.743, 0.807, 0.78567, 0.78567, 0.77434, 0.75834, 0.75834, 0.74767, 0.73767, 0.73767, 0.68634, 0.683, 0.67967, 0.62167, 0.51234, 0.51234, 0.50367, 0.49634, 0.49634, 0.46567, 0.38433, 0.38433, 0.36767, 0.365, 0.36233, 0.35767, 0.34433, 0.331, 0.33033, 0.327, 0.327, 0.29967, 0.29167, 0.29167, 0.27233, 0.265, 0.259, 0.259, 0.25633, 0.25567, 0.25567, 0.24767, 0.24633, 0.24633, 0.23167, 0.23233, 0.23233, 0.22967, 0.22833, 0.22833, 0.21633, 0.21767, 0.21767, 0.205, 0.207, 0.207, 0.19633, 0.19167, 0.19167, 0.19033, 0.18767, 0.18767, 0.183, 0.187, 0.187, 0.18433, 0.18367, 0.18367, 0.185, 0.17767, 0.17767, 0.167, 0.17033, 0.17033, 0.171, 0.16633, 0.16633, 0.16033, 0.17433, 0.17433, 0.17567, 0.17033, 0.17033, 0.14167, 0.13767, 0.13767, 0.115, 0.115, 0.115, 0.11545, 0.11662, 0.11662, 0.11567, 0.16167, 0.20767, 0.83286, 0.83286], [0.53292, 0.53262, 0.53075, 0.528, 0.528, 0.51, 0.54067, 0.54067, 0.58867, 0.57, 0.57, 0.558, 0.58067, 0.60804, 0.61867, 0.61867, 0.61867, 0.61067, 0.67667, 0.67067, 0.67067, 0.73667, 0.74533, 0.75533, 0.75533, 0.81867, 0.88, 0.88, 0.88867, 0.87333, 0.87333, 0.86533, 0.868, 0.868, 0.866, 0.866, 0.866, 0.928, 0.89867, 0.89867, 0.89533, 0.89733, 0.89733, 0.924, 0.886, 0.886, 0.88267, 0.88267, 0.88267, 0.88267, 0.872, 0.86133, 0.86, 0.85733, 0.85733, 0.83867, 0.83733, 0.83733, 0.82667, 0.81, 0.808, 0.808, 0.796, 0.794, 0.794, 0.788, 0.77867, 0.77867, 0.76867, 0.76133, 0.76133, 0.75267, 0.74467, 0.74467, 0.73667, 0.732, 0.732, 0.70867, 0.69733, 0.69733, 0.698, 0.694, 0.694, 0.686, 0.68533, 0.68533, 0.68333, 0.67667, 0.67667, 0.672, 0.66933, 0.66933, 0.66467, 0.65533, 0.65533, 0.624, 0.61533, 0.61533, 0.60733, 0.60467, 0.60467, 0.60533, 0.58533, 0.58533, 0.58333, 0.57933, 0.57933, 0.57333, 0.546, 0.546, 0.52133, 0.51267, 0.50882, 0.50358, 0.49589, 0.49589, 0.482, 0.48066, 0.47933, 0.53292, 0.53292], "MCCCCCCLCLCCCCCCCCCCCCLCCCCCCCCCCCCCCCCCCCCZ"],
[0xcc7226, -1, [0.14067, 0.099, 0.123, 0.123, 0.13767, 0.35367, 0.35367, 0.35367, 0.635, 0.65367, 0.67234, 0.831, 0.831, 0.82167, 0.71367, 0.68167, 0.659, 0.63634, 0.64034, 0.635, 0.62967, 0.56434, 0.55367, 0.543, 0.50076, 0.52567, 0.55234, 0.42833, 0.40033, 0.37233, 0.41233, 0.41233, 0.443, 0.38567, 0.38567, 0.32833, 0.28833, 0.283, 0.27767, 0.26967, 0.26833, 0.267, 0.25448, 0.20167, 0.16833, 0.14533, 0.14533, 0.14067], [0.49567, 0.56133, 0.46733, 0.46733, 0.41, 0.47266, 0.47266, 0.47266, 0.52333, 0.53, 0.53667, 0.526, 0.526, 0.554, 0.63133, 0.59267, 0.59933, 0.606, 0.59, 0.58867, 0.58733, 0.62867, 0.62733, 0.626, 0.66558, 0.61267, 0.556, 0.54733, 0.566, 0.58467, 0.53533, 0.53533, 0.502, 0.53, 0.53, 0.55133, 0.50867, 0.50733, 0.506, 0.50067, 0.51133, 0.522, 0.54983, 0.506, 0.47833, 0.515, 0.515, 0.49567], "MCCCCLCCCCCCCCCCCLZ"],
[0xe87f3a, -1, [0.55585, 0.54518, 0.50291, 0.52785, 0.55552, 0.43052, 0.40252, 0.37452, 0.41452, 0.41452, 0.44518, 0.38785, 0.38785, 0.33052, 0.29052, 0.28518, 0.27985, 0.27185, 0.27052, 0.26918, 0.25688, 0.20385, 0.16846, 0.14597, 0.14597, 0.14064, 0.09897, 0.12409, 0.12409, 0.13876, 0.35585, 0.35585, 0.35585, 0.63718, 0.65585, 0.67452, 0.83173, 0.83173, 0.82252, 0.71452, 0.68385, 0.66118, 0.63852, 0.64252, 0.63718, 0.63185, 0.56652, 0.55585], [0.62442, 0.62309, 0.66266, 0.60976, 0.55109, 0.54442, 0.56309, 0.58176, 0.53242, 0.53242, 0.49909, 0.52709, 0.52709, 0.54842, 0.50576, 0.50442, 0.50309, 0.49776, 0.50842, 0.51909, 0.54665, 0.50309, 0.47418, 0.50994, 0.50994, 0.49309, 0.55942, 0.46224, 0.46224, 0.40491, 0.46976, 0.46976, 0.46976, 0.52042, 0.52709, 0.53376, 0.52321, 0.52321, 0.55206, 0.62939, 0.58976, 0.59642, 0.60309, 0.58709, 0.58576, 0.58442, 0.62576, 0.62442], "MCCCCCCCCLCCCCLCCCZ"],
[0xea8c4d, -1, [0.55803, 0.54736, 0.50614, 0.53003, 0.55703, 0.4327, 0.4047, 0.3767, 0.4167, 0.4167, 0.44736, 0.39003, 0.39003, 0.3327, 0.2927, 0.28736, 0.28203, 0.27403, 0.2727, 0.27136, 0.25928, 0.20603, 0.16858, 0.14661, 0.14661, 0.14061, 0.10094, 0.12518, 0.12518, 0.13985, 0.35803, 0.35803, 0.35803, 0.63937, 0.65803, 0.6767, 0.83246, 0.83246, 0.82337, 0.71536, 0.68603, 0.66336, 0.6407, 0.6447, 0.63937, 0.63403, 0.5687, 0.55803], [0.62152, 0.62018, 0.66023, 0.60685, 0.54651, 0.54151, 0.56018, 0.57885, 0.52951, 0.52951, 0.49618, 0.52418, 0.52418, 0.54551, 0.50285, 0.50151, 0.50018, 0.49485, 0.50551, 0.51618, 0.54346, 0.50018, 0.47003, 0.50488, 0.50488, 0.49051, 0.55551, 0.45715, 0.45715, 0.39982, 0.46685, 0.46685, 0.46685, 0.51751, 0.52418, 0.53085, 0.52042, 0.52042, 0.55012, 0.62745, 0.58685, 0.59352, 0.60018, 0.58418, 0.58285, 0.58151, 0.62285, 0.62152], "MCCCCCCCCLCCCCLCCCZ"],
[0xec9961, -1, [0.56021, 0.54955, 0.50833, 0.53221, 0.56021, 0.43399, 0.40688, 0.37888, 0.41888, 0.41888, 0.44955, 0.39221, 0.39221, 0.33488, 0.29488, 0.28955, 0.28421, 0.27621, 0.27488, 0.27355, 0.26168, 0.20821, 0.1687, 0.14724, 0.14724, 0.14058, 0.10424, 0.12627, 0.12627, 0.14094, 0.36021, 0.36021, 0.36021, 0.64155, 0.66022, 0.67888, 0.83318, 0.83318, 0.82422, 0.71622, 0.68821, 0.66555, 0.64288, 0.64688, 0.64155, 0.63621, 0.57088, 0.56021], [0.61861, 0.61727, 0.65732, 0.60394, 0.54135, 0.5392, 0.55727, 0.57594, 0.5266, 0.5266, 0.49327, 0.52127, 0.52127, 0.5426, 0.49994, 0.4986, 0.49727, 0.49194, 0.5026, 0.51327, 0.54027, 0.49727, 0.46588, 0.49982, 0.49982, 0.48794, 0.54927, 0.45206, 0.45206, 0.39472, 0.46394, 0.46394, 0.46394, 0.51461, 0.52127, 0.52794, 0.51764, 0.51764, 0.54818, 0.62552, 0.58394, 0.59061, 0.59727, 0.58127, 0.57994, 0.5786, 0.61994, 0.61861], "MCCCCCCCCLCCCCLCCCZ"],
[0xeea575, -1, [0.5624, 0.55173, 0.51136, 0.53439, 0.5624, 0.43706, 0.40906, 0.38106, 0.42106, 0.42106, 0.45173, 0.3944, 0.3944, 0.33706, 0.29706, 0.29173, 0.28639, 0.2784, 0.27706, 0.27573, 0.26409, 0.2104, 0.16882, 0.14788, 0.14788, 0.14054, 0.10754, 0.12736, 0.12736, 0.14203, 0.3624, 0.3624, 0.3624, 0.64373, 0.6624, 0.68106, 0.83391, 0.83391, 0.82506, 0.71706, 0.6904, 0.66773, 0.64506, 0.64906, 0.64373, 0.6384, 0.57306, 0.5624], [0.6157, 0.61436, 0.65478, 0.60103, 0.5357, 0.5357, 0.55436, 0.57303, 0.5237, 0.5237, 0.49036, 0.51836, 0.51836, 0.5397, 0.49703, 0.4957, 0.49436, 0.48903, 0.4997, 0.51036, 0.53708, 0.49436, 0.46172, 0.49476, 0.49476, 0.48536, 0.54336, 0.44697, 0.44697, 0.38963, 0.46103, 0.46103, 0.46103, 0.5117, 0.51836, 0.52503, 0.51485, 0.51485, 0.54624, 0.62358, 0.58103, 0.5877, 0.59436, 0.57836, 0.57703, 0.5757, 0.61703, 0.6157], "MCCCCCCCCLCCCCLCCCZ"],
[0xf1b288, -1, [0.56458, 0.55391, 0.51064, 0.53658, 0.56858, 0.43924, 0.41124, 0.38324, 0.42324, 0.42324, 0.45391, 0.39658, 0.39658, 0.33924, 0.29924, 0.29391, 0.28858, 0.28058, 0.27924, 0.27791, 0.26649, 0.21258, 0.16894, 0.14851, 0.14851, 0.14052, 0.11052, 0.12845, 0.12845, 0.14312, 0.36458, 0.36458, 0.36458, 0.64591, 0.66458, 0.68324, 0.83464, 0.83464, 0.82591, 0.71791, 0.69258, 0.66991, 0.64724, 0.65125, 0.64591, 0.64058, 0.57524, 0.56458], [0.61279, 0.61145, 0.65054, 0.59812, 0.53345, 0.53279, 0.55145, 0.57012, 0.52079, 0.52079, 0.48745, 0.51545, 0.51545, 0.53679, 0.49412, 0.49279, 0.49145, 0.48612, 0.49679, 0.50745, 0.53389, 0.49145, 0.45757, 0.4897, 0.4897, 0.48279, 0.53812, 0.44188, 0.44188, 0.38454, 0.45812, 0.45812, 0.45812, 0.50879, 0.51545, 0.52212, 0.51206, 0.51206, 0.5443, 0.62163, 0.57812, 0.58479, 0.59145, 0.57545, 0.57412, 0.57279, 0.61412, 0.61279], "MCCCCCCCCLCCCCLCCCZ"],
[0xf3bf9c, -1, [0.56676, 0.55609, 0.51219, 0.53876, 0.57276, 0.44142, 0.41342, 0.38542, 0.42542, 0.42542, 0.45609, 0.39876, 0.39876, 0.34142, 0.30142, 0.29609, 0.29076, 0.28276, 0.28142, 0.28009, 0.26889, 0.21476, 0.16906, 0.14915, 0.14915, 0.14048, 0.11382, 0.12954, 0.12954, 0.14421, 0.36676, 0.36676, 0.36676, 0.64809, 0.66676, 0.68543, 0.83537, 0.83537, 0.82676, 0.71876, 0.69476, 0.67209, 0.64943, 0.65343, 0.64809, 0.64276, 0.57743, 0.56676], [0.60988, 0.60854, 0.64731, 0.59521, 0.52854, 0.52988, 0.54854, 0.56721, 0.51788, 0.51788, 0.48454, 0.51254, 0.51254, 0.53388, 0.49121, 0.48988, 0.48854, 0.48321, 0.49388, 0.50454, 0.5307, 0.48854, 0.45342, 0.48464, 0.48464, 0.48021, 0.53054, 0.43678, 0.43678, 0.37945, 0.45521, 0.45521, 0.45521, 0.50588, 0.51254, 0.51921, 0.50927, 0.50927, 0.54236, 0.6197, 0.57521, 0.58188, 0.58854, 0.57254, 0.57121, 0.56988, 0.61121, 0.60988], "MCCCCCCCCLCCCCLCCCZ"],
[0xf5ccb0, -1, [0.56894, 0.55828, 0.51254, 0.54094, 0.57761, 0.44361, 0.41561, 0.38761, 0.42761, 0.42761, 0.45827, 0.40094, 0.40094, 0.34361, 0.30361, 0.29827, 0.29294, 0.28494, 0.28361, 0.28227, 0.27129, 0.21694, 0.16918, 0.14979, 0.14979, 0.14046, 0.11645, 0.13064, 0.13064, 0.1453, 0.36894, 0.36894, 0.36894, 0.65027, 0.66894, 0.68761, 0.83609, 0.83609, 0.82761, 0.71961, 0.69694, 0.67428, 0.65161, 0.65561, 0.65027, 0.64494, 0.57961, 0.56894], [0.60697, 0.60563, 0.64343, 0.5923, 0.5263, 0.52697, 0.54564, 0.5643, 0.51497, 0.51497, 0.48164, 0.50964, 0.50964, 0.53097, 0.4883, 0.48697, 0.48563, 0.4803, 0.49097, 0.50163, 0.52751, 0.48563, 0.44927, 0.47957, 0.47957, 0.47764, 0.52497, 0.4317, 0.4317, 0.37436, 0.4523, 0.4523, 0.4523, 0.50297, 0.50964, 0.5163, 0.50648, 0.50648, 0.54042, 0.61776, 0.5723, 0.57897, 0.58563, 0.56964, 0.5683, 0.56697, 0.6083, 0.60697], "MCCCCCCCCLCCCCLCCCZ"],
[0xf8d8c4, -1, [0.57112, 0.56046, 0.51472, 0.54312, 0.57979, 0.44579, 0.41779, 0.38979, 0.42979, 0.42979, 0.46046, 0.40312, 0.40312, 0.34579, 0.30579, 0.30045, 0.29512, 0.28712, 0.28579, 0.28446, 0.27369, 0.21912, 0.1693, 0.15042, 0.15042, 0.14042, 0.11776, 0.13173, 0.13173, 0.1464, 0.37112, 0.37112, 0.37112, 0.65246, 0.67112, 0.68979, 0.83682, 0.83682, 0.82846, 0.72046, 0.69912, 0.67646, 0.65379, 0.65779, 0.65246, 0.64712, 0.58179, 0.57112], [0.60406, 0.60272, 0.64052, 0.58939, 0.52339, 0.52406, 0.54272, 0.56139, 0.51206, 0.51206, 0.47872, 0.50672, 0.50672, 0.52806, 0.48539, 0.48406, 0.48272, 0.47739, 0.48806, 0.49872, 0.52433, 0.48272, 0.44512, 0.47451, 0.47451, 0.47506, 0.51906, 0.4266, 0.4266, 0.36927, 0.44939, 0.44939, 0.44939, 0.50006, 0.50672, 0.51339, 0.5037, 0.5037, 0.53848, 0.61582, 0.56939, 0.57606, 0.58272, 0.56672, 0.56539, 0.56406, 0.60539, 0.60406], "MCCCCCCCCLCCCCLCCCZ"],
[0xfae5d7, -1, [0.5733, 0.56264, 0.51755, 0.54531, 0.58197, 0.44797, 0.41997, 0.39197, 0.43197, 0.43197, 0.46264, 0.4053, 0.4053, 0.34797, 0.30797, 0.30264, 0.2973, 0.2893, 0.28797, 0.28664, 0.27609, 0.2213, 0.16942, 0.15106, 0.15106, 0.14039, 0.11873, 0.13282, 0.13282, 0.14748, 0.3733, 0.3733, 0.3733, 0.65464, 0.6733, 0.69197, 0.83755, 0.83755, 0.8293, 0.7213, 0.7013, 0.67864, 0.65597, 0.65997, 0.65464, 0.6493, 0.58397, 0.5733], [0.60115, 0.59982, 0.63796, 0.58648, 0.51848, 0.52115, 0.53982, 0.55848, 0.50915, 0.50915, 0.47582, 0.50382, 0.50382, 0.52515, 0.48248, 0.48115, 0.47982, 0.47448, 0.48515, 0.49582, 0.52114, 0.47982, 0.44097, 0.46945, 0.46945, 0.47248, 0.51248, 0.42151, 0.42151, 0.36418, 0.44648, 0.44648, 0.44648, 0.49715, 0.50382, 0.51048, 0.50091, 0.50091, 0.53654, 0.61388, 0.56648, 0.57315, 0.57982, 0.56382, 0.56248, 0.56115, 0.60248, 0.60115], "MCCCCCCCCLCCCCLCCCZ"],
[0xfcf2eb, -1, [0.57549, 0.56482, 0.52153, 0.54749, 0.58282, 0.45015, 0.42215, 0.39415, 0.43415, 0.43415, 0.46482, 0.40749, 0.40749, 0.35015, 0.31015, 0.30482, 0.29949, 0.29149, 0.29015, 0.28882, 0.27849, 0.22349, 0.16954, 0.1517, 0.1517, 0.14036, 0.11903, 0.13391, 0.13391, 0.14858, 0.37549, 0.37549, 0.37549, 0.65682, 0.67549, 0.69416, 0.83828, 0.83828, 0.83016, 0.72215, 0.70349, 0.68082, 0.65816, 0.66216, 0.65682, 0.65149, 0.58615, 0.57549], [0.59824, 0.59691, 0.63598, 0.58357, 0.51224, 0.51824, 0.53691, 0.55557, 0.50624, 0.50624, 0.47291, 0.50091, 0.50091, 0.52224, 0.47957, 0.47824, 0.47691, 0.47157, 0.48224, 0.49291, 0.51795, 0.47691, 0.43682, 0.46439, 0.46439, 0.46991, 0.50857, 0.41642, 0.41642, 0.35909, 0.44357, 0.44357, 0.44357, 0.49424, 0.50091, 0.50757, 0.49812, 0.49812, 0.53461, 0.61194, 0.56357, 0.57024, 0.57691, 0.56091, 0.55957, 0.55824, 0.59958, 0.59824], "MCCCCCCCCLCCCCLCCCZ"],
[0xffffff, -1, [0.14033, 0.119, 0.135, 0.135, 0.14967, 0.37767, 0.37767, 0.37767, 0.659, 0.67767, 0.69633, 0.839, 0.839, 0.831, 0.723, 0.70567, 0.683, 0.66034, 0.66434, 0.659, 0.65367, 0.58833, 0.57767, 0.567, 0.52497, 0.54967, 0.58671, 0.4484, 0.42433, 0.39633, 0.43633, 0.43633, 0.467, 0.40967, 0.40967, 0.35233, 0.31233, 0.307, 0.30167, 0.29367, 0.29233, 0.291, 0.28089, 0.22567, 0.16967, 0.15233, 0.15233, 0.14033], [0.46733, 0.504, 0.41133, 0.41133, 0.354, 0.44066, 0.44066, 0.44066, 0.49133, 0.498, 0.50467, 0.49533, 0.49533, 0.53267, 0.61, 0.56067, 0.56733, 0.574, 0.558, 0.55667, 0.55533, 0.59667, 0.59533, 0.594, 0.63368, 0.58067, 0.50116, 0.51796, 0.534, 0.55267, 0.50333, 0.50333, 0.47, 0.498, 0.498, 0.51933, 0.47667, 0.47533, 0.474, 0.46866, 0.47933, 0.49, 0.51476, 0.474, 0.43266, 0.45933, 0.45933, 0.46733], "MCCCCLCCCCCCCCCCCLZ"],
[0, -1, [0.20967, 0.20967, 0.19767, 0.23233, 0.23233, 0.23467, 0.20467, 0.20467, 0.19433, 0.19167, 0.19167, 0.18367, 0.17567, 0.16767, 0.20967, 0.20967], [0.41733, 0.41733, 0.398, 0.376, 0.376, 0.37366, 0.38066, 0.38066, 0.384, 0.40133, 0.40133, 0.40866, 0.418, 0.42733, 0.41733, 0.41733], "MCCCCCZ"],
[0xcccccc, -1, [0.443, 0.443, 0.4725, 0.4715, 0.46933, 0.469, 0.47433, 0.47967, 0.49434, 0.49434, 0.49434, 0.49367, 0.51434, 0.51434, 0.53367, 0.50034, 0.50034, 0.44167, 0.443], [0.49667, 0.49667, 0.45196, 0.444, 0.42666, 0.41066, 0.404, 0.39733, 0.342, 0.342, 0.342, 0.34, 0.40333, 0.40333, 0.43, 0.46066, 0.46066, 0.50867, 0.49667], "MCCCCCCZ"],
[0, -1, [0.243, 0.243, 0.26167, 0.23767, 0.24833, 0.24833, 0.247, 0.24167, 0.25367, 0.25367, 0.26167, 0.255, 0.255, 0.283, 0.28167, 0.28167, 0.29233, 0.28567, 0.279, 0.267, 0.26833, 0.25367, 0.25367, 0.263, 0.263, 0.24967, 0.24967, 0.27545, 0.25767, 0.24767, 0.243, 0.243], [0.37266, 0.37266, 0.36066, 0.30867, 0.31266, 0.31266, 0.294, 0.29, 0.29533, 0.29533, 0.282, 0.274, 0.274, 0.26066, 0.25, 0.25, 0.26333, 0.274, 0.28466, 0.278, 0.30867, 0.30333, 0.30333, 0.318, 0.32867, 0.32467, 0.32467, 0.36896, 0.37133, 0.37266, 0.37266, 0.37266], "MCLCLCCCCLCLCCZ"],
[0xcccccc, -1, [0.297, 0.297, 0.30167, 0.297, 0.29233, 0.24033, 0.23033, 0.23033, 0.28767, 0.297], [0.34466, 0.34466, 0.352, 0.35066, 0.34933, 0.32467, 0.30867, 0.30867, 0.34933, 0.34466], "MCCCZ"],
[0xcccccc, -1, [0.31433, 0.31433, 0.319, 0.31433, 0.30967, 0.25767, 0.24767, 0.24767, 0.305, 0.31433], [0.33133, 0.33133, 0.33866, 0.33733, 0.336, 0.31133, 0.29533, 0.29533, 0.336, 0.33133], "MCCCZ"],
[0xcccccc, -1, [0.33633, 0.33633, 0.341, 0.33633, 0.33167, 0.27967, 0.26967, 0.26967, 0.327, 0.33633], [0.35666, 0.35666, 0.364, 0.36266, 0.36133, 0.33666, 0.32066, 0.32066, 0.36133, 0.35666], "MCCCZ"],
[0xcccccc, -1, [0.29767, 0.29767, 0.29767, 0.293, 0.28833, 0.22833, 0.21833, 0.21833, 0.28833, 0.29767], [0.284, 0.284, 0.294, 0.29266, 0.29133, 0.262, 0.246, 0.246, 0.28866, 0.284], "MCCCZ"],
[0xcccccc, -1, [0.29967, 0.29967, 0.30167, 0.297, 0.29367, 0.24967, 0.23967, 0.23967, 0.289, 0.29967], [0.302, 0.302, 0.31, 0.30867, 0.30867, 0.28933, 0.27333, 0.27333, 0.30933, 0.302], "MCCCZ"],
[0xcccccc, -1, [0.27567, 0.259, 0.259, 0.27633, 0.28233, 0.28233, 0.271, 0.26967, 0.26967, 0.287, 0.29633, 0.29633, 0.309, 0.309, 0.309, 0.31833, 0.32367, 0.32367, 0.32567, 0.32367, 0.32367, 0.33033, 0.337, 0.337, 0.34767, 0.34633, 0.34633, 0.34633, 0.345, 0.345, 0.35433, 0.35833, 0.35833, 0.37167, 0.37967, 0.37967, 0.373, 0.381, 0.381, 0.399, 0.40433, 0.40433, 0.393, 0.40233, 0.40233, 0.41367, 0.41567, 0.41567, 0.42967, 0.433, 0.433, 0.42033, 0.423, 0.423, 0.41967, 0.42833, 0.42833, 0.423, 0.43233, 0.44167, 0.449, 0.463, 0.463, 0.479, 0.48234, 0.27567], [0.22266, 0.21, 0.21, 0.22266, 0.22066, 0.22066, 0.202, 0.19333, 0.19333, 0.21466, 0.214, 0.214, 0.21333, 0.19533, 0.19533, 0.21266, 0.212, 0.212, 0.20133, 0.19, 0.19, 0.20266, 0.2, 0.2, 0.20333, 0.184, 0.184, 0.16667, 0.162, 0.162, 0.206, 0.20667, 0.20667, 0.20866, 0.194, 0.194, 0.20667, 0.20333, 0.20333, 0.20066, 0.18933, 0.18933, 0.20933, 0.204, 0.204, 0.204, 0.19333, 0.19333, 0.158, 0.15533, 0.15533, 0.19133, 0.19133, 0.19133, 0.21133, 0.18667, 0.18667, 0.21, 0.20866, 0.20733, 0.19066, 0.19466, 0.19466, 0.18533, 0.30066, 0.22266], "MLCCCCCCCCCCCCCCCCCCCCCLZ"],
[0, -1, [0.28367, 0.28367, 0.30833, 0.375, 0.375, 0.387, 0.39833, 0.40967, 0.45433, 0.465, 0.481, 0.48234, 0.48234, 0.503, 0.50367, 0.50434, 0.47967, 0.46367, 0.44767, 0.43167, 0.39967, 0.39967, 0.365, 0.32233, 0.32233, 0.27367, 0.269, 0.26433, 0.28767, 0.28767, 0.28767, 0.295, 0.293, 0.291, 0.29167, 0.28367], [0.37733, 0.37733, 0.38733, 0.37733, 0.37733, 0.37666, 0.39133, 0.406, 0.418, 0.41466, 0.404, 0.402, 0.402, 0.38466, 0.372, 0.35933, 0.27933, 0.25266, 0.226, 0.20533, 0.20933, 0.20933, 0.216, 0.20933, 0.20933, 0.212, 0.22533, 0.23866, 0.264, 0.264, 0.264, 0.278, 0.302, 0.326, 0.37266, 0.37733], "MCCCLLCCCCCCCCZ"],
[0xe5668c, -1, [0.32033, 0.33433, 0.285, 0.285, 0.28167, 0.3061, 0.323, 0.34124, 0.40833, 0.40833, 0.44767, 0.469, 0.469, 0.469, 0.48633, 0.457, 0.42767, 0.32033, 0.32033], [0.374, 0.34333, 0.23466, 0.23466, 0.232, 0.22192, 0.226, 0.2304, 0.22333, 0.22333, 0.24933, 0.32333, 0.32333, 0.32333, 0.36333, 0.36866, 0.374, 0.374, 0.374], "MCCCCCCZ"],
[0xb23259, -1, [0.31695, 0.32249, 0.32513, 0.32033, 0.32033, 0.42433, 0.443, 0.45007, 0.47467, 0.474, 0.474, 0.369, 0.34433, 0.31695], [0.3225, 0.34382, 0.36349, 0.374, 0.374, 0.36333, 0.398, 0.41112, 0.36, 0.344, 0.344, 0.32, 0.33866, 0.3225], "MCCCCLZ"],
[0xa5264c, -1, [0.32433, 0.32433, 0.32767, 0.32367, 0.32367, 0.321, 0.319, 0.319, 0.321, 0.331, 0.331, 0.33433, 0.33833, 0.34233, 0.35033, 0.357, 0.36367, 0.38233, 0.38233, 0.38233, 0.39167, 0.40633, 0.40633, 0.41029, 0.411, 0.41183, 0.417, 0.42033, 0.42367, 0.43967, 0.43767, 0.43567, 0.32433, 0.32433], [0.29533, 0.29533, 0.28333, 0.27666, 0.27666, 0.27533, 0.27466, 0.27466, 0.26867, 0.266, 0.266, 0.25867, 0.258, 0.25733, 0.248, 0.25, 0.252, 0.25867, 0.25867, 0.25867, 0.264, 0.258, 0.258, 0.25934, 0.266, 0.27383, 0.28, 0.28333, 0.28666, 0.308, 0.30867, 0.30933, 0.29533, 0.29533], "MCCCCCCCCCCCZ"],
[0xff727f, 0, [0.317, 0.317, 0.31233, 0.31767, 0.323, 0.32167, 0.32033, 0.319, 0.32633, 0.33567, 0.35567, 0.35567, 0.381, 0.39633, 0.39633, 0.41132, 0.417, 0.417, 0.425, 0.437, 0.449, 0.461, 0.45433, 0.44767, 0.42367, 0.397, 0.37033, 0.367, 0.317], [0.376, 0.376, 0.33866, 0.32467, 0.31067, 0.30733, 0.30066, 0.294, 0.27733, 0.26733, 0.26466, 0.26466, 0.27066, 0.266, 0.266, 0.26376, 0.28866, 0.28866, 0.29933, 0.304, 0.30867, 0.378, 0.39133, 0.40466, 0.412, 0.386, 0.36, 0.388, 0.376], "MCCCLCCCCCCZ"],
[0xffffcc, 0, [0.31967, 0.31967, 0.31833, 0.311, 0.311, 0.27367, 0.25967, 0.25967, 0.24833, 0.25567, 0.25567, 0.273, 0.28433, 0.28433, 0.31167, 0.31967], [0.25133, 0.25133, 0.25467, 0.25533, 0.25533, 0.26133, 0.282, 0.282, 0.29133, 0.272, 0.272, 0.238, 0.23333, 0.23333, 0.22667, 0.25133], "MCCCCCZ"],
[0xcc3f4c, -1, [0.4529, 0.454, 0.45726, 0.45433, 0.44359, 0.4153, 0.397, 0.37033, 0.367, 0.317, 0.317, 0.31409, 0.31549, 0.31549, 0.37767, 0.379, 0.379, 0.38167, 0.397, 0.41233, 0.45024, 0.4529], [0.35795, 0.37113, 0.38548, 0.39133, 0.41282, 0.40384, 0.386, 0.36, 0.388, 0.376, 0.376, 0.35273, 0.33604, 0.33604, 0.35533, 0.346, 0.346, 0.35133, 0.35133, 0.35133, 0.35328, 0.35795], "MCCCCCCCZ"],
[-1, 0xa51926, [0.381, 0.381, 0.389, 0.383, 0.383, 0.359, 0.36233], [0.37466, 0.37466, 0.36666, 0.35066, 0.35066, 0.324, 0.30066], "MCC"],
[0xffffcc, 0, [0.301, 0.301, 0.29367, 0.30833, 0.30833, 0.31633, 0.31433, 0.31233, 0.303, 0.301], [0.23333, 0.23333, 0.25467, 0.24333, 0.24333, 0.24, 0.23733, 0.23466, 0.228, 0.23333], "MCCCZ"],
[0xffffcc, 0, [0.3094, 0.3094, 0.30353, 0.31527, 0.31527, 0.32264, 0.32007, 0.31247, 0.32007, 0.3094], [0.23133, 0.23133, 0.2484, 0.23933, 0.23933, 0.23525, 0.23453, 0.2324, 0.22813, 0.23133], "MCCCZ"],
[0xffffcc, 0, [0.3174, 0.3174, 0.31153, 0.32327, 0.32327, 0.33056, 0.32807, 0.32247, 0.32807, 0.3174], [0.23133, 0.23133, 0.2484, 0.23933, 0.23933, 0.23548, 0.23453, 0.2324, 0.22813, 0.23133], "MCCCZ"],
[0xffffcc, 0, [0.3284, 0.3284, 0.32253, 0.33427, 0.33427, 0.34157, 0.33907, 0.33413, 0.33907, 0.3284], [0.231, 0.231, 0.24806, 0.239, 0.239, 0.23511, 0.2342, 0.2324, 0.2278, 0.231], "MCCCZ"],
[0xffffcc, 0, [0.3392, 0.3392, 0.33333, 0.34507, 0.34507, 0.35147, 0.34987, 0.34827, 0.34987, 0.3392], [0.23113, 0.23113, 0.2482, 0.23913, 0.23913, 0.23647, 0.23433, 0.2322, 0.22793, 0.23113], "MCCCZ"],
[0xffffcc, 0, [0.35033, 0.35033, 0.34233, 0.35767, 0.35767, 0.36567, 0.36367, 0.36167, 0.36367, 0.35033], [0.23, 0.23, 0.25067, 0.24, 0.24, 0.23667, 0.234, 0.23133, 0.226, 0.23], "MCCCZ"],
[-1, 0xa5264c, [0.303, 0.303, 0.325, 0.335, 0.335, 0.345, 0.347, 0.349, 0.35433, 0.35433], [0.25867, 0.25867, 0.26333, 0.258, 0.258, 0.256, 0.25666, 0.25733, 0.258, 0.258], "MCCC"],
[-1, 0xa5264c, [0.35967, 0.35967, 0.37967, 0.39967, 0.41136, 0.40967, 0.411, 0.41233, 0.41267, 0.421], [0.244, 0.244, 0.26666, 0.25933, 0.25504, 0.26066, 0.26533, 0.27, 0.277, 0.282], "MCCC"],
[0xffffcc, 0, [0.38833, 0.38833, 0.38167, 0.377, 0.37233, 0.367, 0.36433, 0.36433, 0.36433, 0.37833, 0.37833, 0.39633, 0.397, 0.39767, 0.395, 0.38833], [0.27066, 0.27066, 0.28866, 0.26733, 0.246, 0.24, 0.23533, 0.23533, 0.22667, 0.22733, 0.22733, 0.228, 0.23266, 0.23733, 0.25666, 0.27066], "MCCCCCZ"],
[-1, 0xa5264c, [0.41167, 0.41167, 0.41767, 0.42167], [0.25867, 0.25867, 0.26266, 0.26066], "MC"],
[-1, 0xa5264c, [0.4225, 0.4225, 0.42733, 0.43533], [0.286, 0.286, 0.29416, 0.2955], "MC"],
[0xb2b2b2, -1, [0.29033, 0.29033, 0.32033, 0.32767, 0.32767, 0.34233, 0.32833, 0.32833, 0.307, 0.29367, 0.29367, 0.27433, 0.29033], [0.22466, 0.22466, 0.21933, 0.222, 0.222, 0.222, 0.21867, 0.21867, 0.21867, 0.22066, 0.22066, 0.23, 0.22466], "MCCCCZ"],
[0xffffcc, 0, [0.31367, 0.31367, 0.343, 0.34633, 0.34633, 0.35833, 0.35233, 0.35233, 0.35033, 0.34567, 0.34567, 0.315, 0.30967, 0.30433, 0.31167, 0.31367], [0.38, 0.38, 0.38, 0.37866, 0.37866, 0.32733, 0.31467, 0.31467, 0.31, 0.31933, 0.31933, 0.374, 0.37733, 0.38066, 0.38, 0.38], "MCCCCCZ"],
[0xffffcc, 0, [0.18517, 0.18517, 0.2, 0.221, 0.221, 0.229, 0.23433, 0.23967, 0.23367, 0.22767, 0.22167, 0.197, 0.19367, 0.19033, 0.18517, 0.18517], [0.3845, 0.3845, 0.38166, 0.37733, 0.37733, 0.34, 0.332, 0.324, 0.324, 0.32867, 0.33333, 0.35666, 0.364, 0.37133, 0.3845, 0.3845], "MCCCCCZ"],
[0xffffcc, 0, [0.2216, 0.2216, 0.23127, 0.23295, 0.23463, 0.23095, 0.23095, 0.23095, 0.22928, 0.22728, 0.22527, 0.22049, 0.2216], [0.37697, 0.37697, 0.37439, 0.3707, 0.36701, 0.36151, 0.36151, 0.36151, 0.356, 0.35961, 0.36321, 0.37598, 0.37697], "MCCCCZ"],
[0, -1, [0.22167, 0.22167, 0.22767, 0.23367, 0.23967, 0.2403, 0.245, 0.25267, 0.252, 0.263, 0.2674, 0.27167, 0.27633, 0.281, 0.28633, 0.28833, 0.29033, 0.29833, 0.29833, 0.29833, 0.277, 0.27233, 0.27233, 0.235, 0.22167], [0.37733, 0.37733, 0.36866, 0.36866, 0.36866, 0.36935, 0.36833, 0.36666, 0.37, 0.368, 0.3672, 0.36866, 0.36666, 0.36466, 0.366, 0.36933, 0.37266, 0.37966, 0.37966, 0.37966, 0.37666, 0.37533, 0.37533, 0.37333, 0.37733], "MCCCCCCCCZ"],
[0xffffcc, 0, [0.296, 0.296, 0.28525, 0.28458, 0.28392, 0.29333, 0.29333, 0.29333, 0.29792, 0.29892, 0.29992, 0.29733, 0.296], [0.377, 0.377, 0.37116, 0.36716, 0.36316, 0.357, 0.357, 0.357, 0.34933, 0.35333, 0.35733, 0.37633, 0.377], "MCCCCZ"],
[0xffffcc, 0, [0.23352, 0.23352, 0.2452, 0.24556, 0.24556, 0.24649, 0.24356, 0.23344, 0.23597, 0.23352], [0.36789, 0.36789, 0.34924, 0.36793, 0.36793, 0.37003, 0.37006, 0.37019, 0.37703, 0.36789], "MCCCZ"],
[0xffffcc, 0, [0.24549, 0.24549, 0.25869, 0.25763, 0.25763, 0.25764, 0.25472, 0.24681, 0.24738, 0.24549], [0.36748, 0.36748, 0.34883, 0.36763, 0.36763, 0.36818, 0.36844, 0.36913, 0.37666, 0.36748], "MCCCZ"],
[0xffffcc, 0, [0.25751, 0.25751, 0.27078, 0.26966, 0.26966, 0.26986, 0.2671, 0.26062, 0.26026, 0.25751], [0.36746, 0.36746, 0.34975, 0.36586, 0.36586, 0.36792, 0.36842, 0.36959, 0.37501, 0.36746], "MCCCZ"],
[0xffffcc, 0, [0.26897, 0.26897, 0.28214, 0.28272, 0.28272, 0.28546, 0.28255, 0.27285, 0.27418, 0.26897], [0.36733, 0.36733, 0.34806, 0.36436, 0.36436, 0.36666, 0.36703, 0.36826, 0.37569, 0.36733], "MCCCZ"],
[0xe5e5b2, -1, [0.20868, 0.19592, 0.19158, 0.18808, 0.18808, 0.18808, 0.19867, 0.2195, 0.2195, 0.22096, 0.22341, 0.20868], [0.36145, 0.364, 0.37233, 0.38258, 0.38258, 0.38258, 0.38092, 0.37625, 0.37625, 0.37072, 0.36106, 0.36145], "MLCCCLZ"],
[0xe5e5b2, -1, [0.31713, 0.31435, 0.31216, 0.31107, 0.30605, 0.31296, 0.31484, 0.31484, 0.34246, 0.34559, 0.34559, 0.34647, 0.34761, 0.34761, 0.33079, 0.31713], [0.36922, 0.37339, 0.37632, 0.377, 0.38014, 0.37951, 0.37951, 0.37951, 0.37951, 0.37826, 0.37826, 0.37452, 0.36887, 0.36887, 0.37222, 0.36922], "MCCCCCZ"],
[0xcc7226, -1, [0.40647, 0.45267, 0.4952, 0.49813, 0.50107, 0.4842, 0.4842, 0.4864, 0.47833, 0.46954, 0.46074, 0.43423, 0.405, 0.3786, 0.3478, 0.3456, 0.3434, 0.35367, 0.35587, 0.35807, 0.35367, 0.35367, 0.41073, 0.36027, 0.40647], [0.59946, 0.59286, 0.65226, 0.6684, 0.68453, 0.70433, 0.70433, 0.70947, 0.73293, 0.74833, 0.76373, 0.76211, 0.76373, 0.7652, 0.72633, 0.7234, 0.72047, 0.65666, 0.64713, 0.6376, 0.5936, 0.5936, 0.60876, 0.60606, 0.59946], "MCCCCCCCCZ"],
[0xea8e51, -1, [0.34681, 0.34465, 0.35473, 0.35689, 0.35905, 0.35473, 0.35473, 0.40919, 0.36121, 0.40657, 0.45194, 0.4937, 0.49658, 0.49946, 0.48289, 0.48289, 0.48506, 0.47714, 0.46849, 0.45986, 0.43384, 0.40513, 0.37921, 0.34897, 0.34681], [0.72232, 0.71944, 0.6568, 0.64744, 0.63808, 0.59488, 0.59488, 0.60968, 0.60712, 0.60064, 0.59416, 0.65248, 0.66832, 0.68416, 0.7036, 0.7036, 0.70864, 0.73168, 0.7468, 0.76192, 0.76032, 0.76192, 0.76336, 0.7252, 0.72232], "MCCCCCCCCZ"],
[0xefaa7c, -1, [0.34803, 0.34591, 0.3558, 0.35792, 0.36004, 0.3558, 0.3558, 0.40814, 0.36216, 0.40668, 0.4512, 0.49219, 0.49502, 0.49784, 0.48159, 0.48159, 0.48371, 0.47594, 0.46746, 0.45898, 0.43344, 0.40527, 0.37983, 0.35015, 0.34803], [0.72124, 0.71841, 0.65693, 0.64774, 0.63856, 0.59616, 0.59616, 0.61142, 0.60817, 0.60181, 0.59545, 0.65269, 0.66824, 0.68379, 0.70287, 0.70287, 0.70781, 0.73043, 0.74527, 0.76011, 0.75854, 0.76011, 0.76152, 0.72407, 0.72124], "MCCCCCCCCZ"],
[0xf4c6a8, -1, [0.34924, 0.34716, 0.35687, 0.35895, 0.36103, 0.35687, 0.35687, 0.40593, 0.36311, 0.40679, 0.45047, 0.49068, 0.49346, 0.49623, 0.48028, 0.48028, 0.48236, 0.47474, 0.46642, 0.4581, 0.43304, 0.4054, 0.38044, 0.35132, 0.34924], [0.72016, 0.71739, 0.65706, 0.64805, 0.63904, 0.59744, 0.59744, 0.6125, 0.60922, 0.60298, 0.59675, 0.6529, 0.66816, 0.68341, 0.70213, 0.70213, 0.70699, 0.72917, 0.74373, 0.75829, 0.75676, 0.75829, 0.75968, 0.72293, 0.72016], "MCCCCCCCCZ"],
[0xf9e2d3, -1, [0.35045, 0.34841, 0.35793, 0.35997, 0.36201, 0.35793, 0.35793, 0.40438, 0.36405, 0.40689, 0.44974, 0.48918, 0.4919, 0.49462, 0.47898, 0.47898, 0.48102, 0.47354, 0.46538, 0.45722, 0.43264, 0.40553, 0.38105, 0.35249, 0.35045], [0.71908, 0.71636, 0.6572, 0.64836, 0.63952, 0.59872, 0.59872, 0.61359, 0.61028, 0.60416, 0.59804, 0.65312, 0.66808, 0.68304, 0.7014, 0.7014, 0.70616, 0.72792, 0.7422, 0.75648, 0.75498, 0.75648, 0.75784, 0.7218, 0.71908], "MCCCCCCCCZ"],
[0xffffff, -1, [0.407, 0.449, 0.48767, 0.49034, 0.493, 0.47767, 0.47767, 0.47967, 0.47233, 0.46433, 0.45633, 0.43224, 0.40567, 0.38167, 0.35367, 0.35167, 0.34967, 0.359, 0.361, 0.363, 0.359, 0.359, 0.4015, 0.365, 0.407], [0.60533, 0.59933, 0.65333, 0.668, 0.68267, 0.70067, 0.70067, 0.70533, 0.72667, 0.74067, 0.75467, 0.75319, 0.75467, 0.756, 0.72067, 0.718, 0.71533, 0.65733, 0.64867, 0.64, 0.6, 0.6, 0.61433, 0.61133, 0.60533], "MCCCCCCCCZ"],
[0xcccccc, -1, [0.48434, 0.48434, 0.438, 0.41867, 0.41867, 0.39233, 0.37767, 0.37767, 0.37167, 0.36833, 0.365, 0.48434, 0.48434], [0.662, 0.662, 0.64933, 0.652, 0.652, 0.663, 0.62667, 0.62667, 0.61467, 0.61133, 0.608, 0.662, 0.662], "MCCCCZ"],
[0, -1, [0.49067, 0.49067, 0.44233, 0.42567, 0.42567, 0.39833, 0.38433, 0.38433, 0.37033, 0.365, 0.365, 0.36433, 0.375, 0.39233, 0.39233, 0.417, 0.433, 0.433, 0.43967, 0.43967, 0.43967, 0.475, 0.47767, 0.48034, 0.49134, 0.49067], [0.66567, 0.66567, 0.64533, 0.646, 0.646, 0.65367, 0.62933, 0.62933, 0.614, 0.61133, 0.61133, 0.60867, 0.61533, 0.60667, 0.60667, 0.59067, 0.61733, 0.61733, 0.636, 0.63933, 0.64267, 0.652, 0.65267, 0.65333, 0.66033, 0.66567], "MCCCCLCCCCZ"],
[0x99cc32, -1, [0.41167, 0.40021, 0.38626, 0.38626, 0.38626, 0.40021, 0.41167, 0.42312, 0.43241, 0.43241, 0.43241, 0.42312, 0.41167], [0.60581, 0.60581, 0.61225, 0.62267, 0.63308, 0.64352, 0.64352, 0.64352, 0.63508, 0.62467, 0.61425, 0.60581, 0.60581], "MCCCCZ"],
[0x659900, -1, [0.40563, 0.39755, 0.38907, 0.38919, 0.39176, 0.40236, 0.41167, 0.41883, 0.42514, 0.42887, 0.42887, 0.42001, 0.40563], [0.63362, 0.63241, 0.62991, 0.63027, 0.63797, 0.64352, 0.64352, 0.64352, 0.64022, 0.63521, 0.63521, 0.63576, 0.63362], "MCCCCZ"],
[0xffffff, -1, [0.42567, 0.42567, 0.41833, 0.41833, 0.41833, 0.42433, 0.42567], [0.634, 0.634, 0.63933, 0.63567, 0.63567, 0.62833, 0.634], "MCCZ"],
[0, -1, [0.409, 0.40484, 0.40146, 0.40146, 0.40146, 0.40484, 0.409, 0.41317, 0.41654, 0.41654, 0.41654, 0.41317, 0.409], [0.62046, 0.62046, 0.62383, 0.628, 0.63216, 0.63554, 0.63554, 0.63554, 0.63216, 0.628, 0.62383, 0.62046, 0.62046], "MCCCCZ"],
[0xcc7226, -1, [0.23567, 0.23567, 0.23033, 0.23433, 0.23433, 0.25233, 0.25167, 0.25167, 0.251, 0.249, 0.247, 0.23433, 0.22433, 0.22433, 0.207, 0.20833, 0.20833, 0.20833, 0.19567, 0.193, 0.193, 0.191, 0.189, 0.189, 0.18433, 0.18767, 0.18767, 0.18433, 0.185, 0.19767, 0.19767, 0.201, 0.219, 0.22706, 0.23233, 0.23567], [0.64267, 0.64267, 0.678, 0.68533, 0.68533, 0.702, 0.708, 0.708, 0.738, 0.73933, 0.74067, 0.75067, 0.74, 0.74, 0.71, 0.69933, 0.696, 0.696, 0.69667, 0.69333, 0.69333, 0.68467, 0.684, 0.684, 0.68, 0.67533, 0.67533, 0.67133, 0.66467, 0.658, 0.658, 0.634, 0.62533, 0.62145, 0.63267, 0.64267], "MCCCCCLCCCCLCCZ"],
[0xffffff, -1, [0.234, 0.234, 0.2292, 0.2328, 0.2328, 0.249, 0.2484, 0.2484, 0.2478, 0.246, 0.2442, 0.2328, 0.2238, 0.2238, 0.2082, 0.2094, 0.2094, 0.2094, 0.198, 0.1956, 0.1956, 0.1938, 0.192, 0.192, 0.1878, 0.1908, 0.1908, 0.1878, 0.1884, 0.1998, 0.1998, 0.2028, 0.219, 0.22625, 0.231, 0.234], [0.64573, 0.64573, 0.67753, 0.68413, 0.68413, 0.69913, 0.70453, 0.70453, 0.73153, 0.73273, 0.73393, 0.74293, 0.73333, 0.73333, 0.70633, 0.69673, 0.69373, 0.69373, 0.69433, 0.69133, 0.69133, 0.68353, 0.68293, 0.68293, 0.67933, 0.67513, 0.67513, 0.67153, 0.66553, 0.65953, 0.65953, 0.63793, 0.63013, 0.62664, 0.63673, 0.64573], "MCCCCCLCCCCLCCZ"],
[0xeb955c, -1, [0.24825, 0.24643, 0.23395, 0.2242, 0.2242, 0.2073, 0.2086, 0.2086, 0.2086, 0.19625, 0.19365, 0.19365, 0.1917, 0.18975, 0.18975, 0.1852, 0.18845, 0.18845, 0.1852, 0.18585, 0.1982, 0.1982, 0.20145, 0.219, 0.22686, 0.232, 0.23525, 0.23525, 0.23005, 0.23395, 0.23395, 0.2515, 0.25085, 0.25085, 0.2502, 0.24825], [0.73768, 0.73912, 0.74873, 0.73833, 0.73833, 0.70908, 0.69868, 0.69543, 0.69543, 0.69608, 0.69283, 0.69283, 0.68438, 0.68373, 0.68373, 0.67983, 0.67528, 0.67528, 0.67138, 0.66488, 0.65838, 0.65838, 0.63498, 0.62653, 0.62275, 0.63368, 0.64343, 0.64343, 0.67788, 0.68503, 0.68503, 0.70128, 0.70713, 0.70713, 0.73638, 0.73768], "MCCLCCCCLCCCCCZ"],
[0xf2b892, -1, [0.2475, 0.24587, 0.23357, 0.22407, 0.22407, 0.2076, 0.20887, 0.20887, 0.20887, 0.19683, 0.1943, 0.1943, 0.1924, 0.1905, 0.1905, 0.18607, 0.18923, 0.18923, 0.18607, 0.1867, 0.19873, 0.19873, 0.2019, 0.219, 0.22666, 0.23167, 0.23483, 0.23483, 0.22977, 0.23357, 0.23357, 0.25067, 0.25003, 0.25003, 0.2494, 0.2475], [0.73603, 0.73757, 0.7468, 0.73667, 0.73667, 0.70817, 0.69803, 0.69487, 0.69487, 0.6955, 0.69233, 0.69233, 0.6841, 0.68347, 0.68347, 0.67967, 0.67523, 0.67523, 0.67143, 0.6651, 0.65877, 0.65877, 0.63597, 0.62773, 0.62405, 0.6347, 0.6442, 0.6442, 0.67777, 0.68473, 0.68473, 0.70057, 0.70627, 0.70627, 0.73477, 0.73603], "MCCLCCCCLCCCCCZ"],
[0xf8dcc8, -1, [0.24675, 0.2453, 0.23318, 0.22393, 0.22393, 0.2079, 0.20913, 0.20913, 0.20913, 0.19742, 0.19495, 0.19495, 0.1931, 0.19125, 0.19125, 0.18693, 0.19002, 0.19002, 0.18693, 0.18755, 0.19927, 0.19927, 0.20235, 0.219, 0.22646, 0.23133, 0.23442, 0.23442, 0.22948, 0.23318, 0.23318, 0.24983, 0.24922, 0.24922, 0.2486, 0.24675], [0.73438, 0.73602, 0.74487, 0.735, 0.735, 0.70725, 0.69738, 0.6943, 0.6943, 0.69492, 0.69183, 0.69183, 0.68382, 0.6832, 0.6832, 0.6795, 0.67518, 0.67518, 0.67148, 0.66532, 0.65915, 0.65915, 0.63695, 0.62893, 0.62534, 0.63572, 0.64497, 0.64497, 0.67765, 0.68443, 0.68443, 0.69985, 0.7054, 0.7054, 0.73315, 0.73438], "MCCLCCCCLCCCCCZ"],
[0xffffff, -1, [0.234, 0.234, 0.2292, 0.2328, 0.2328, 0.249, 0.2484, 0.2484, 0.2478, 0.246, 0.24473, 0.2328, 0.2238, 0.2238, 0.2082, 0.2094, 0.2094, 0.2094, 0.198, 0.1956, 0.1956, 0.1938, 0.192, 0.192, 0.1878, 0.1908, 0.1908, 0.1878, 0.1884, 0.1998, 0.1998, 0.2028, 0.219, 0.22625, 0.231, 0.234], [0.6459, 0.6459, 0.67753, 0.68413, 0.68413, 0.69913, 0.70453, 0.70453, 0.73153, 0.73273, 0.73447, 0.74293, 0.73333, 0.73333, 0.70633, 0.69673, 0.69373, 0.69373, 0.69433, 0.69133, 0.69133, 0.68353, 0.68293, 0.68293, 0.67933, 0.67513, 0.67513, 0.67153, 0.66553, 0.65953, 0.65953, 0.63793, 0.63013, 0.62664, 0.6369, 0.6459], "MCCCCCLCCCCLCCZ"],
[0xcccccc, -1, [0.22883, 0.22883, 0.19283, 0.19133, 0.19133, 0.2065, 0.20783, 0.20917, 0.22883, 0.22883], [0.65633, 0.65633, 0.67333, 0.67467, 0.67467, 0.661, 0.661, 0.661, 0.65633, 0.65633], "MCCCZ"],
[0, -1, [0.20033, 0.20033, 0.231, 0.231, 0.231, 0.2306, 0.22167, 0.20767, 0.21367, 0.20033], [0.66667, 0.66667, 0.66067, 0.65333, 0.64848, 0.62612, 0.62867, 0.63267, 0.65667, 0.66667], "MCCCZ"],
[0x99cc32, -1, [0.21433, 0.21433, 0.2293, 0.231, 0.232, 0.2331, 0.224, 0.21641, 0.2127, 0.21433], [0.66033, 0.66033, 0.65788, 0.65333, 0.65067, 0.63682, 0.635, 0.63348, 0.65052, 0.66033], "MCCCZ"],
[0, -1, [0.35766, 0.35683, 0.35902, 0.362, 0.36533, 0.38567, 0.387, 0.38833, 0.41033, 0.41033, 0.41333, 0.42067, 0.42067, 0.42867, 0.43967, 0.43967, 0.44367, 0.44933, 0.44933, 0.47367, 0.49434, 0.49434, 0.52767, 0.51767, 0.51767, 0.51267, 0.518, 0.518, 0.51834, 0.53034, 0.53034, 0.53467, 0.536, 0.536, 0.54934, 0.54367, 0.54367, 0.544, 0.53934, 0.53934, 0.53934, 0.53634, 0.53634, 0.53134, 0.53534, 0.53534, 0.53834, 0.53467, 0.53467, 0.53267, 0.51734, 0.51734, 0.51367, 0.50367, 0.50367, 0.499, 0.49334, 0.49334, 0.489, 0.47967, 0.47967, 0.48434, 0.48833, 0.49234, 0.49634, 0.49934, 0.502, 0.50467, 0.50934, 0.50934, 0.51, 0.50067, 0.50067, 0.5, 0.49734, 0.49734, 0.49234, 0.49034, 0.48867, 0.487, 0.48, 0.48, 0.47734, 0.47533, 0.47533, 0.475, 0.465, 0.465, 0.463, 0.45533, 0.45533, 0.444, 0.448, 0.448, 0.451, 0.46967, 0.46967, 0.47333, 0.46, 0.46, 0.459, 0.46067, 0.46067, 0.46267, 0.474, 0.474, 0.48967, 0.485, 0.485, 0.47467, 0.465, 0.465, 0.46133, 0.43233, 0.43233, 0.42433, 0.42867, 0.422, 0.41533, 0.381, 0.381, 0.36173, 0.35718, 0.3513, 0.3513, 0.36032, 0.35766], [0.58942, 0.59232, 0.5921, 0.593, 0.594, 0.60033, 0.60467, 0.609, 0.60167, 0.60167, 0.60033, 0.596, 0.596, 0.594, 0.59333, 0.59333, 0.59167, 0.587, 0.587, 0.57, 0.582, 0.582, 0.593, 0.62167, 0.62167, 0.63667, 0.64233, 0.64233, 0.64867, 0.638, 0.638, 0.631, 0.62267, 0.62267, 0.604, 0.63367, 0.63367, 0.63533, 0.64133, 0.64333, 0.64533, 0.651, 0.651, 0.65667, 0.66833, 0.66833, 0.69133, 0.68833, 0.68833, 0.69133, 0.67467, 0.67467, 0.669, 0.66633, 0.66633, 0.66333, 0.66567, 0.66567, 0.66633, 0.65467, 0.65467, 0.655, 0.64767, 0.64733, 0.647, 0.65133, 0.65233, 0.65333, 0.64367, 0.64367, 0.63933, 0.63133, 0.63133, 0.62367, 0.62633, 0.62633, 0.62733, 0.621, 0.61333, 0.60567, 0.605, 0.605, 0.59267, 0.59767, 0.59767, 0.607, 0.59733, 0.59733, 0.594, 0.59767, 0.59767, 0.601, 0.60433, 0.60433, 0.608, 0.60433, 0.60433, 0.607, 0.61367, 0.61367, 0.61667, 0.624, 0.624, 0.62933, 0.63867, 0.63867, 0.64067, 0.64333, 0.64333, 0.652, 0.63933, 0.63933, 0.629, 0.604, 0.604, 0.59833, 0.60967, 0.604, 0.59833, 0.61333, 0.61333, 0.61532, 0.58909, 0.59429, 0.59429, 0.58008, 0.58942], "MCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCZ"],
[0, -1, [0.68234, 0.68234, 0.63967, 0.635, 0.635, 0.631, 0.665, 0.665, 0.66567, 0.669, 0.669, 0.66634, 0.69767, 0.743, 0.743, 0.75367, 0.76234, 0.771, 0.79634, 0.79034, 0.79034, 0.79234, 0.78234, 0.78234, 0.76834, 0.75634, 0.75634, 0.745, 0.741, 0.68234], [0.86667, 0.86667, 0.85333, 0.822, 0.822, 0.784, 0.75467, 0.75467, 0.744, 0.73867, 0.73867, 0.73067, 0.74333, 0.75733, 0.75733, 0.76133, 0.776, 0.79067, 0.822, 0.864, 0.864, 0.88267, 0.88333, 0.88333, 0.886, 0.87333, 0.87333, 0.868, 0.86867, 0.86667], "MCCCCLCCCCCLZ"],
[0, -1, [0.77337, 0.77337, 0.77687, 0.7688, 0.7688, 0.75707, 0.7446, 0.7446, 0.7204, 0.71307, 0.71307, 0.70647, 0.71967, 0.71967, 0.72773, 0.73947, 0.7512, 0.77704, 0.77337], [0.86832, 0.86832, 0.8832, 0.87513, 0.87513, 0.8656, 0.8656, 0.8656, 0.86193, 0.83993, 0.83993, 0.7952, 0.78567, 0.78567, 0.7732, 0.7842, 0.7952, 0.84558, 0.86832], "MCCCCCCZ"],
[0x323232, -1, [0.77275, 0.77275, 0.77623, 0.76831, 0.76831, 0.75679, 0.74455, 0.74455, 0.72079, 0.71359, 0.71359, 0.70711, 0.72007, 0.72007, 0.72799, 0.73951, 0.75103, 0.77635, 0.77275], [0.86772, 0.86772, 0.88229, 0.87437, 0.87437, 0.86501, 0.86501, 0.86501, 0.86141, 0.83981, 0.83981, 0.79589, 0.78653, 0.78653, 0.77429, 0.78509, 0.79589, 0.8454, 0.86772], "MCCCCCCZ"],
[0x666666, -1, [0.77212, 0.77212, 0.77559, 0.76782, 0.76782, 0.75651, 0.7445, 0.7445, 0.72118, 0.71411, 0.71411, 0.70775, 0.72047, 0.72047, 0.72824, 0.73955, 0.75086, 0.77566, 0.77212], [0.86712, 0.86712, 0.88139, 0.87361, 0.87361, 0.86443, 0.86443, 0.86443, 0.86089, 0.83969, 0.83969, 0.79659, 0.7874, 0.7874, 0.77539, 0.78599, 0.79659, 0.84522, 0.86712], "MCCCCCCZ"],
[0x999999, -1, [0.7715, 0.7715, 0.77495, 0.76732, 0.76732, 0.75623, 0.74444, 0.74444, 0.72156, 0.71463, 0.71463, 0.70839, 0.72087, 0.72087, 0.7285, 0.73959, 0.75068, 0.77497, 0.7715], [0.86653, 0.86653, 0.88048, 0.87285, 0.87285, 0.86384, 0.86384, 0.86384, 0.86037, 0.83957, 0.83957, 0.79728, 0.78827, 0.78827, 0.77648, 0.78688, 0.79728, 0.84503, 0.86653], "MCCCCCCZ"],
[0xcccccc, -1, [0.77088, 0.77088, 0.77431, 0.76683, 0.76683, 0.75595, 0.74439, 0.74439, 0.72195, 0.71515, 0.71515, 0.70903, 0.72127, 0.72127, 0.72875, 0.73963, 0.75051, 0.77428, 0.77088], [0.86593, 0.86593, 0.87957, 0.87209, 0.87209, 0.86325, 0.86325, 0.86325, 0.85985, 0.83945, 0.83945, 0.79797, 0.78913, 0.78913, 0.77757, 0.78777, 0.79797, 0.84485, 0.86593], "MCCCCCCZ"],
[0xffffff, -1, [0.77025, 0.77025, 0.77367, 0.76634, 0.76634, 0.75567, 0.74434, 0.74434, 0.72234, 0.71567, 0.71567, 0.70967, 0.72167, 0.72167, 0.729, 0.73967, 0.75034, 0.77358, 0.77025], [0.86533, 0.86533, 0.87867, 0.87133, 0.87133, 0.86267, 0.86267, 0.86267, 0.85933, 0.83933, 0.83933, 0.79867, 0.79, 0.79, 0.77867, 0.78867, 0.79867, 0.84467, 0.86533], "MCCCCCCZ"],
[0x992600, -1, [0.41767, 0.41767, 0.38367, 0.37033, 0.37033, 0.313, 0.28833, 0.28833, 0.31767, 0.36367, 0.36367, 0.32767, 0.307, 0.307, 0.279, 0.263, 0.25833, 0.25833, 0.265, 0.29567, 0.29567, 0.33367, 0.35167, 0.35167, 0.31567, 0.299, 0.299, 0.24833, 0.227, 0.227, 0.23367, 0.25833, 0.25833, 0.281, 0.315, 0.315, 0.339, 0.34767, 0.35633, 0.35433, 0.34033, 0.34033, 0.331, 0.30767, 0.30767, 0.23633, 0.219, 0.219, 0.24167, 0.259, 0.259, 0.29633, 0.31033, 0.31033, 0.35167, 0.36433, 0.36433, 0.34567, 0.351, 0.35633, 0.36767, 0.36767, 0.36767, 0.408, 0.414, 0.41767], [0.52667, 0.52667, 0.55867, 0.56, 0.56, 0.56667, 0.53667, 0.53667, 0.57067, 0.56133, 0.56133, 0.56867, 0.56333, 0.56333, 0.56333, 0.54, 0.532, 0.532, 0.55667, 0.56667, 0.56667, 0.57467, 0.56667, 0.56667, 0.578, 0.57467, 0.57467, 0.57867, 0.53467, 0.53467, 0.55867, 0.57067, 0.57067, 0.58533, 0.58067, 0.58067, 0.57533, 0.57133, 0.56733, 0.572, 0.58, 0.58, 0.59667, 0.596, 0.596, 0.59, 0.57, 0.57, 0.58867, 0.59333, 0.59333, 0.60667, 0.60533, 0.60533, 0.60367, 0.61033, 0.61033, 0.602, 0.59667, 0.59133, 0.57867, 0.57667, 0.57467, 0.53783, 0.5305, 0.52667], "MCCCCCLCCCCCCCCCCCCCCCCLZ"],
[0xcccccc, -1, [0.64833, 0.64833, 0.6225, 0.60167, 0.60167, 0.645, 0.65083, 0.65083, 0.65083, 0.64833], [0.20333, 0.20333, 0.26417, 0.28, 0.28, 0.25333, 0.22333, 0.22333, 0.20667, 0.20333], "MCCCZ"],
[0xcccccc, -1, [0.72667, 0.72667, 0.6825, 0.65167, 0.65167, 0.72417, 0.7325, 0.73333, 0.72833, 0.72833, 0.7275, 0.72667], [0.19083, 0.19083, 0.2825, 0.3225, 0.3225, 0.26, 0.21583, 0.20667, 0.21083, 0.21083, 0.19583, 0.19083], "MCCLLCZ"],
[0xcccccc, -1, [0.82083, 0.82083, 0.71667, 0.71417, 0.71417, 0.815, 0.82, 0.82, 0.81667, 0.82083], [0.27167, 0.27167, 0.37083, 0.375, 0.375, 0.265, 0.2525, 0.2525, 0.2675, 0.27167], "MCCCZ"],
[0xcccccc, -1, [0.50667, 0.50667, 0.53917, 0.57083, 0.57083, 0.59583, 0.595, 0.595, 0.58833, 0.55833, 0.55833, 0.52667, 0.50667], [0.19917, 0.19917, 0.28583, 0.24833, 0.24833, 0.23167, 0.22667, 0.22667, 0.2375, 0.23667, 0.23667, 0.24167, 0.19917], "MCCCCZ"],
[0xcccccc, -1, [0.82417, 0.82417, 0.74917, 0.73667, 0.71699, 0.81917, 0.8275, 0.8275, 0.83083, 0.82417], [0.41167, 0.41167, 0.45917, 0.46167, 0.4656, 0.41333, 0.39583, 0.39583, 0.4, 0.41167], "MCCCZ"],
[0, -1, [0.573, 0.573, 0.607, 0.61834, 0.62567, 0.655, 0.661, 0.661, 0.685, 0.68367, 0.68234, 0.705, 0.705, 0.705, 0.70367, 0.71567, 0.71567, 0.71167, 0.72567, 0.72567, 0.70811, 0.74567, 0.755, 0.74767, 0.74767, 0.74767, 0.70434, 0.74034, 0.74034, 0.74367, 0.74167, 0.73967, 0.73634, 0.72834, 0.72034, 0.729, 0.73834, 0.73834, 0.71967, 0.74167, 0.74167, 0.73567, 0.72834, 0.72834, 0.719, 0.74434, 0.74434, 0.737, 0.73167, 0.73167, 0.71234, 0.72434, 0.73167, 0.73167, 0.72034, 0.731, 0.74167, 0.74167, 0.74167, 0.74167, 0.70634, 0.74034, 0.74034, 0.72674, 0.721, 0.721, 0.68967, 0.71367, 0.72167, 0.72167, 0.707, 0.69367, 0.68034, 0.69767, 0.70834, 0.719, 0.745, 0.745, 0.745, 0.76634, 0.773, 0.773, 0.73967, 0.74967, 0.74967, 0.77367, 0.76167, 0.76167, 0.75167, 0.75967, 0.75967, 0.7289, 0.75367, 0.765, 0.765, 0.72434, 0.743, 0.743, 0.77167, 0.775, 0.77834, 0.78567, 0.78567, 0.77834, 0.787, 0.787, 0.76834, 0.77834, 0.78767, 0.78767, 0.75367, 0.77634, 0.77634, 0.749, 0.76367, 0.76367, 0.761, 0.76167, 0.76234, 0.76434, 0.757, 0.74967, 0.767, 0.77034, 0.77367, 0.77967, 0.765, 0.75034, 0.75767, 0.761, 0.76434, 0.77434, 0.773, 0.773, 0.771, 0.76567, 0.76567, 0.741, 0.74367, 0.74367, 0.74167, 0.73634, 0.73634, 0.731, 0.731, 0.731, 0.72567, 0.721, 0.71634, 0.71034, 0.70567, 0.701, 0.69234, 0.69034, 0.69034, 0.67034, 0.66234, 0.643, 0.643, 0.64234, 0.64034, 0.64034, 0.59034, 0.573], [0.234, 0.234, 0.23733, 0.24867, 0.24266, 0.30666, 0.298, 0.298, 0.32266, 0.336, 0.34933, 0.326, 0.326, 0.326, 0.34533, 0.334, 0.334, 0.36, 0.34666, 0.34666, 0.39693, 0.354, 0.34333, 0.35466, 0.35466, 0.35466, 0.43466, 0.41066, 0.41066, 0.44866, 0.456, 0.46333, 0.50067, 0.50933, 0.518, 0.52067, 0.512, 0.512, 0.552, 0.532, 0.532, 0.55733, 0.562, 0.562, 0.59067, 0.57267, 0.57267, 0.59333, 0.59867, 0.59867, 0.64467, 0.63667, 0.63067, 0.63067, 0.654, 0.64667, 0.63933, 0.64, 0.64, 0.64, 0.69533, 0.666, 0.666, 0.6892, 0.70067, 0.70067, 0.73467, 0.724, 0.72133, 0.72133, 0.738, 0.74067, 0.74333, 0.754, 0.75067, 0.74733, 0.73467, 0.73467, 0.73467, 0.70333, 0.70267, 0.70267, 0.71533, 0.702, 0.702, 0.67867, 0.67933, 0.67933, 0.66733, 0.65267, 0.65267, 0.68332, 0.64067, 0.61333, 0.61333, 0.65467, 0.618, 0.618, 0.57867, 0.578, 0.57733, 0.56267, 0.56267, 0.566, 0.55133, 0.55133, 0.57133, 0.54933, 0.52533, 0.52533, 0.562, 0.51267, 0.51267, 0.52133, 0.49267, 0.49267, 0.466, 0.45733, 0.44866, 0.40133, 0.388, 0.37466, 0.34266, 0.336, 0.32933, 0.31133, 0.32666, 0.342, 0.33266, 0.318, 0.30333, 0.27733, 0.268, 0.268, 0.266, 0.272, 0.272, 0.31, 0.286, 0.286, 0.27266, 0.258, 0.258, 0.24, 0.25467, 0.25467, 0.28266, 0.27, 0.25733, 0.24733, 0.24333, 0.23933, 0.27733, 0.26, 0.26, 0.28066, 0.25333, 0.226, 0.226, 0.24666, 0.23667, 0.23667, 0.22667, 0.234], "MCLLLCCCCCCCCCCCCCCCLCCCCCLCCCCCCCCLCCCLLCLCCCCCCCCCCCCCCCCLCCZ"],
[0, -1, [0.51567, 0.51567, 0.49634, 0.48967, 0.483, 0.53567, 0.60433, 0.60433, 0.61233, 0.61834, 0.61834, 0.62367, 0.619, 0.619, 0.60433, 0.623, 0.623, 0.65367, 0.64434, 0.64434, 0.66234, 0.66634, 0.67034, 0.66834, 0.66834, 0.66834, 0.65767, 0.64767, 0.64767, 0.639, 0.63434, 0.62967, 0.62567, 0.633, 0.633, 0.62634, 0.62767, 0.629, 0.635, 0.63767, 0.64034, 0.66767, 0.679, 0.679, 0.66367, 0.65867, 0.65367, 0.60933, 0.59933, 0.59933, 0.57133, 0.59133, 0.59133, 0.651, 0.68134, 0.68134, 0.66934, 0.63867, 0.63867, 0.60167, 0.543, 0.543, 0.51334, 0.50034, 0.50034, 0.496, 0.495, 0.494, 0.47433, 0.42833, 0.42833, 0.4, 0.38567, 0.38567, 0.36033, 0.35433, 0.35433, 0.32333, 0.32, 0.31667, 0.29767, 0.29633, 0.29633, 0.33733, 0.34133, 0.34533, 0.37433, 0.37833, 0.38233, 0.39633, 0.38033, 0.38033, 0.50634, 0.50767, 0.509, 0.51567, 0.51567], [0.82867, 0.82867, 0.842, 0.84133, 0.84067, 0.856, 0.81, 0.81, 0.80533, 0.806, 0.806, 0.802, 0.796, 0.796, 0.78, 0.76133, 0.76133, 0.75, 0.76467, 0.76467, 0.758, 0.75133, 0.74467, 0.75133, 0.75133, 0.75133, 0.76333, 0.772, 0.772, 0.77533, 0.78933, 0.80333, 0.82, 0.82533, 0.82533, 0.818, 0.82467, 0.83133, 0.83733, 0.838, 0.83867, 0.865, 0.86567, 0.86567, 0.86333, 0.865, 0.86667, 0.88567, 0.88767, 0.88767, 0.89867, 0.89533, 0.89533, 0.889, 0.867, 0.867, 0.881, 0.89267, 0.89267, 0.91367, 0.90533, 0.90533, 0.9, 0.897, 0.897, 0.898, 0.89867, 0.89933, 0.91433, 0.90267, 0.90267, 0.895, 0.887, 0.887, 0.885, 0.87967, 0.87967, 0.85533, 0.854, 0.85267, 0.84, 0.83933, 0.83933, 0.85033, 0.85433, 0.85833, 0.86267, 0.86033, 0.858, 0.859, 0.858, 0.858, 0.83333, 0.83, 0.82667, 0.82867, 0.82867], "MCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCZ"],
[0xcc7226, -1, [0.63467, 0.63467, 0.61767, 0.61434, 0.611, 0.59034, 0.58333, 0.57633, 0.556, 0.51034, 0.51034, 0.50934, 0.51534, 0.51534, 0.526, 0.52667, 0.52667, 0.56034, 0.57234, 0.57234, 0.58767, 0.598, 0.598, 0.61667, 0.622, 0.622, 0.63667, 0.63734, 0.63734, 0.647, 0.644, 0.644, 0.64467, 0.63467], [0.844, 0.844, 0.85633, 0.85633, 0.85633, 0.87333, 0.87267, 0.872, 0.88867, 0.875, 0.875, 0.87833, 0.87967, 0.87967, 0.88333, 0.88433, 0.88433, 0.89133, 0.88533, 0.88533, 0.881, 0.87067, 0.87067, 0.86533, 0.867, 0.867, 0.86333, 0.86033, 0.86033, 0.85533, 0.851, 0.851, 0.84833, 0.844], "MCCCCCCCCCCCZ"],
[0xcc7226, -1, [0.61388, 0.61523, 0.61693, 0.61793, 0.61832, 0.61784, 0.61732, 0.61558, 0.61382, 0.61192, 0.61125, 0.61018, 0.60926, 0.60653, 0.60349, 0.60067, 0.59736, 0.59343, 0.58996, 0.58986, 0.58949, 0.58937, 0.58429, 0.57805, 0.574, 0.56996, 0.56603, 0.56198, 0.55895, 0.5566, 0.5539, 0.55161, 0.54918, 0.54662, 0.54352, 0.54046, 0.53731, 0.53716, 0.53683, 0.5367, 0.53616, 0.53567, 0.53539, 0.53259, 0.53011, 0.52734, 0.52537, 0.52249, 0.51992, 0.51498, 0.50972, 0.50467, 0.51152, 0.51934, 0.52611, 0.52998, 0.53442, 0.5387, 0.53952, 0.54067, 0.54134, 0.54156, 0.54186, 0.54196, 0.54604, 0.5499, 0.55404, 0.5546, 0.55549, 0.55591, 0.55844, 0.56168, 0.564, 0.56683, 0.56982, 0.5727, 0.57283, 0.57322, 0.57328, 0.57516, 0.57708, 0.57857, 0.57913, 0.58024, 0.58075, 0.58262, 0.58405, 0.58602, 0.5862, 0.58651, 0.58663, 0.58851, 0.59024, 0.59133, 0.59156, 0.59184, 0.59197, 0.59368, 0.59477, 0.5966, 0.59741, 0.59843, 0.59939, 0.60338, 0.60645, 0.6101, 0.61137, 0.61283, 0.61388], [0.84752, 0.84646, 0.8463, 0.84495, 0.84443, 0.84388, 0.84372, 0.84319, 0.84414, 0.84316, 0.84282, 0.84312, 0.84337, 0.8441, 0.84415, 0.843, 0.84488, 0.84391, 0.84558, 0.84562, 0.84504, 0.84509, 0.847, 0.84653, 0.85033, 0.85102, 0.85179, 0.85292, 0.85377, 0.85542, 0.85681, 0.85799, 0.85886, 0.85948, 0.86023, 0.86004, 0.86091, 0.86095, 0.86038, 0.86042, 0.8606, 0.86157, 0.86149, 0.86062, 0.86224, 0.86167, 0.86371, 0.8633, 0.86404, 0.86545, 0.86333, 0.865, 0.86807, 0.86602, 0.86946, 0.87144, 0.8696, 0.87087, 0.87111, 0.87145, 0.87033, 0.87056, 0.87096, 0.87091, 0.86898, 0.86686, 0.86508, 0.86484, 0.86522, 0.86489, 0.86286, 0.86303, 0.861, 0.86184, 0.8612, 0.86224, 0.86229, 0.86172, 0.86176, 0.863, 0.86255, 0.86204, 0.86185, 0.86144, 0.86132, 0.86086, 0.86006, 0.85975, 0.85972, 0.86029, 0.86024, 0.85951, 0.85961, 0.85767, 0.85789, 0.85829, 0.85824, 0.85768, 0.85642, 0.85602, 0.85584, 0.8548, 0.85451, 0.85329, 0.85074, 0.84925, 0.84873, 0.84834, 0.84752], "MCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCZ"],
[0xcc7226, -1, [0.48616, 0.48196, 0.47802, 0.47395, 0.47365, 0.47304, 0.47271, 0.47103, 0.46955, 0.46791, 0.46702, 0.46565, 0.4648, 0.46056, 0.45618, 0.452, 0.45314, 0.45501, 0.456, 0.45632, 0.45672, 0.45731, 0.46011, 0.46319, 0.46598, 0.46882, 0.47169, 0.47465, 0.47516, 0.47549, 0.47603, 0.47974, 0.48372, 0.48726, 0.48991, 0.49252, 0.49459, 0.49501, 0.49447, 0.494, 0.49465, 0.4951, 0.49529, 0.49543, 0.49543, 0.49529, 0.4951, 0.49464, 0.49401, 0.49167, 0.49462, 0.49346, 0.49133, 0.49258, 0.49134, 0.49087, 0.49048, 0.49067, 0.49106, 0.49006, 0.48978, 0.48913, 0.48764, 0.48616], [0.87123, 0.87411, 0.87595, 0.87893, 0.87915, 0.87886, 0.87907, 0.88008, 0.88105, 0.88221, 0.88285, 0.88283, 0.88326, 0.88541, 0.88634, 0.88833, 0.8894, 0.88899, 0.89033, 0.88987, 0.88939, 0.88971, 0.89122, 0.89147, 0.89132, 0.89117, 0.89066, 0.89021, 0.89013, 0.88926, 0.8891, 0.88799, 0.88887, 0.88747, 0.88642, 0.88505, 0.88292, 0.88249, 0.88199, 0.88167, 0.88185, 0.8815, 0.88098, 0.88058, 0.88008, 0.87968, 0.87916, 0.879, 0.87891, 0.87859, 0.88089, 0.88015, 0.87879, 0.87645, 0.87433, 0.87466, 0.87502, 0.87567, 0.8748, 0.87431, 0.87379, 0.87258, 0.87022, 0.87123], "MCCCCCCCCCCCCCCCCCCCCCZ"],
[0xcc7226, -1, [0.432, 0.42674, 0.42166, 0.41663, 0.41652, 0.41615, 0.41604, 0.41376, 0.41223, 0.41044, 0.40892, 0.40616, 0.40403, 0.40349, 0.40315, 0.40265, 0.40062, 0.39908, 0.39733, 0.40123, 0.40524, 0.40935, 0.40954, 0.40978, 0.41, 0.41023, 0.41044, 0.41067, 0.41099, 0.4115, 0.41192, 0.41284, 0.41376, 0.41466, 0.4149, 0.41511, 0.41533, 0.41556, 0.41578, 0.416, 0.41623, 0.41644, 0.41667, 0.41689, 0.41711, 0.41733, 0.41849, 0.41996, 0.42133, 0.42306, 0.42351, 0.4253, 0.43316, 0.44005, 0.44732, 0.44783, 0.44818, 0.448, 0.44845, 0.44897, 0.44928, 0.45105, 0.45278, 0.45394, 0.4543, 0.45376, 0.45337, 0.44598, 0.43931, 0.432], [0.85898, 0.86031, 0.86012, 0.86224, 0.86229, 0.86171, 0.86176, 0.86275, 0.86436, 0.8661, 0.86758, 0.86694, 0.86775, 0.86795, 0.86881, 0.86887, 0.86913, 0.87071, 0.87167, 0.87299, 0.87294, 0.87358, 0.87361, 0.87308, 0.87308, 0.87308, 0.87344, 0.87367, 0.8732, 0.87266, 0.87308, 0.87398, 0.87366, 0.8736, 0.87358, 0.87308, 0.87308, 0.87308, 0.87359, 0.87359, 0.87359, 0.87308, 0.87308, 0.87308, 0.87344, 0.87367, 0.87236, 0.87329, 0.87299, 0.87261, 0.8707, 0.8702, 0.86803, 0.86445, 0.86097, 0.86073, 0.86032, 0.85967, 0.85967, 0.85981, 0.85959, 0.85837, 0.85746, 0.85563, 0.85506, 0.85439, 0.85447, 0.85613, 0.85714, 0.85898], "MCCCCCCCCCCCCCCCCCCCCCCCZ"],
[0xcc7226, -1, [0.4089, 0.40624, 0.40527, 0.40339, 0.40303, 0.40349, 0.40402, 0.40495, 0.40586, 0.40654, 0.40945, 0.412, 0.41533, 0.41865, 0.42575, 0.42576, 0.42576, 0.42338, 0.42267, 0.41858, 0.4146, 0.41063, 0.4096, 0.41016, 0.4089], [0.7853, 0.78733, 0.79072, 0.7937, 0.79427, 0.79479, 0.79494, 0.7952, 0.79441, 0.79407, 0.79264, 0.79056, 0.79033, 0.78661, 0.78597, 0.78033, 0.7789, 0.78043, 0.779, 0.78067, 0.7805, 0.78294, 0.78358, 0.78434, 0.7853], "MCCCCCCCCZ"],
[0xcc7226, -1, [0.363, 0.36322, 0.37494, 0.37492, 0.37484, 0.36196, 0.36135, 0.36108, 0.34856, 0.34833, 0.34878, 0.36256, 0.363], [0.87293, 0.87292, 0.87254, 0.87235, 0.87183, 0.87008, 0.87037, 0.87049, 0.86644, 0.86667, 0.86689, 0.87293, 0.87293], "MCCCCZ"],
[0, -1, [0.38867, 0.38867, 0.364, 0.35667, 0.34933, 0.31833, 0.31333, 0.31333, 0.291, 0.26267, 0.26267, 0.27533, 0.279, 0.279, 0.30167, 0.30133, 0.30133, 0.32167, 0.32067, 0.32067, 0.36133, 0.358, 0.358, 0.394, 0.39233, 0.39233, 0.42367, 0.419, 0.419, 0.40933, 0.42, 0.42, 0.41433, 0.40533, 0.39633, 0.40133, 0.393, 0.393, 0.38867, 0.381, 0.381, 0.37167, 0.357, 0.357, 0.306, 0.30267, 0.30267, 0.29667, 0.29267, 0.29267, 0.283, 0.278, 0.278, 0.25667, 0.25467, 0.25467, 0.249, 0.24767, 0.24767, 0.25833, 0.26167, 0.26167, 0.285, 0.294, 0.294, 0.30133, 0.30267, 0.30267, 0.32667, 0.33367, 0.33367, 0.349, 0.353, 0.353, 0.36267, 0.372, 0.372, 0.37733, 0.376, 0.376, 0.37867, 0.38033, 0.38033, 0.386, 0.394, 0.394, 0.40067, 0.39733, 0.39733, 0.39, 0.37033, 0.37033, 0.34967, 0.32233, 0.32233, 0.27267, 0.25733, 0.25733, 0.24667, 0.23767, 0.23767, 0.228, 0.218, 0.218, 0.23433, 0.24933, 0.24933, 0.256, 0.24967, 0.24967, 0.24367, 0.24633, 0.24633, 0.24533, 0.244, 0.244, 0.231, 0.231, 0.231, 0.233, 0.23367, 0.23433, 0.232, 0.23833, 0.24467, 0.24933, 0.25067, 0.252, 0.24733, 0.247, 0.24667, 0.23967, 0.241, 0.241, 0.24267, 0.244, 0.244, 0.243, 0.244, 0.244, 0.24533, 0.24767, 0.25, 0.25333, 0.254, 0.25467, 0.25467, 0.257, 0.26267, 0.26267, 0.258, 0.26167, 0.26167, 0.26, 0.263, 0.263, 0.27467, 0.27733, 0.28, 0.27767, 0.27767, 0.27767, 0.28767, 0.278, 0.278, 0.27133, 0.26633, 0.26633, 0.25367, 0.26033, 0.267, 0.28367, 0.29, 0.29133, 0.31, 0.308, 0.308, 0.30767, 0.31467, 0.32167, 0.33133, 0.33367, 0.336, 0.34167, 0.341, 0.34033, 0.34, 0.34, 0.34, 0.34833, 0.34733, 0.34633, 0.33267, 0.33033, 0.34767, 0.35367, 0.35367, 0.35967, 0.36, 0.36033, 0.368, 0.37, 0.372, 0.37533, 0.375, 0.37467, 0.38733, 0.38733, 0.38733, 0.39267, 0.395, 0.39733, 0.40433, 0.40433, 0.446, 0.51867, 0.49034, 0.38867], [0.85667, 0.85667, 0.85367, 0.85167, 0.84967, 0.837, 0.83367, 0.83367, 0.82467, 0.79133, 0.79133, 0.797, 0.80167, 0.80167, 0.82267, 0.81833, 0.81833, 0.83267, 0.829, 0.829, 0.84767, 0.84233, 0.84233, 0.85, 0.84667, 0.84667, 0.83933, 0.839, 0.839, 0.837, 0.831, 0.831, 0.82367, 0.83033, 0.837, 0.83333, 0.83167, 0.83167, 0.83033, 0.837, 0.837, 0.84467, 0.83867, 0.83867, 0.81767, 0.81667, 0.81667, 0.812, 0.806, 0.806, 0.79867, 0.79633, 0.79633, 0.777, 0.77467, 0.77467, 0.766, 0.76533, 0.76533, 0.77167, 0.775, 0.775, 0.79167, 0.793, 0.793, 0.798, 0.80033, 0.80033, 0.81567, 0.81567, 0.81567, 0.807, 0.81867, 0.81867, 0.82167, 0.81967, 0.81967, 0.824, 0.82767, 0.82767, 0.83067, 0.82433, 0.82433, 0.81833, 0.82167, 0.82167, 0.822, 0.818, 0.818, 0.81167, 0.81133, 0.81133, 0.81033, 0.79767, 0.79767, 0.78033, 0.763, 0.763, 0.74833, 0.74633, 0.74633, 0.745, 0.73267, 0.73267, 0.74233, 0.74233, 0.74233, 0.74633, 0.74033, 0.74033, 0.72767, 0.71867, 0.71867, 0.71, 0.70733, 0.70733, 0.686, 0.682, 0.678, 0.66167, 0.66067, 0.65967, 0.66333, 0.65933, 0.65533, 0.65267, 0.648, 0.64333, 0.657, 0.66, 0.663, 0.675, 0.679, 0.679, 0.67733, 0.675, 0.675, 0.676, 0.682, 0.682, 0.69067, 0.696, 0.70133, 0.70767, 0.709, 0.71033, 0.72, 0.71567, 0.71133, 0.71133, 0.71567, 0.71933, 0.71933, 0.72867, 0.733, 0.733, 0.747, 0.74867, 0.75033, 0.74967, 0.74967, 0.74967, 0.75667, 0.754, 0.754, 0.75133, 0.75133, 0.75133, 0.748, 0.755, 0.762, 0.771, 0.77067, 0.768, 0.772, 0.77067, 0.77067, 0.771, 0.77167, 0.77233, 0.77, 0.773, 0.776, 0.77767, 0.77533, 0.773, 0.76967, 0.76967, 0.76967, 0.77933, 0.77567, 0.772, 0.76333, 0.753, 0.76667, 0.77167, 0.77167, 0.768, 0.77067, 0.77333, 0.783, 0.78267, 0.78233, 0.787, 0.78267, 0.77833, 0.76933, 0.76933, 0.76933, 0.77233, 0.77, 0.76767, 0.803, 0.803, 0.82067, 0.82633, 0.83767, 0.85667], "MCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCLCCCCCCCCLLLCCCCCCLLCCCCCCLLLLZ"],
[-1, 0x4c0000, [0.419, 0.419, 0.394, 0.38, 0.38, 0.35767, 0.31667], [0.525, 0.525, 0.553, 0.55733, 0.55733, 0.56867, 0.55567], "MCC"],
[-1, 0x4c0000, [0.37467, 0.37467, 0.33267, 0.307, 0.307, 0.27633, 0.26233], [0.55967, 0.55967, 0.573, 0.566, 0.566, 0.56267, 0.53967], "MCC"],
[-1, 0x4c0000, [0.36867, 0.36867, 0.34033, 0.31567, 0.31567, 0.288, 0.26033, 0.26033, 0.24, 0.231], [0.56167, 0.56167, 0.57367, 0.57733, 0.57733, 0.58167, 0.56967, 0.56967, 0.55967, 0.54267], "MCCC"],
[-1, 0x4c0000, [0.37033, 0.37033, 0.34467, 0.343, 0.343, 0.33133, 0.30967, 0.30967, 0.274, 0.24533], [0.561, 0.561, 0.57933, 0.58167, 0.58167, 0.6, 0.60067, 0.60067, 0.59933, 0.586], "MCCC"],
[0, -1, [0.36816, 0.37073, 0.41567, 0.41567, 0.47433, 0.42767, 0.42767, 0.415, 0.39967, 0.39967, 0.39767, 0.423, 0.423, 0.42967, 0.45233, 0.45233, 0.441, 0.449, 0.449, 0.45367, 0.48767, 0.48767, 0.49367, 0.50034, 0.50034, 0.52367, 0.513, 0.513, 0.517, 0.52634, 0.52634, 0.545, 0.51767, 0.51767, 0.46767, 0.45633, 0.45633, 0.45233, 0.467, 0.467, 0.481, 0.455, 0.455, 0.459, 0.47433, 0.47433, 0.49167, 0.495, 0.495, 0.525, 0.54234, 0.54234, 0.54567, 0.53634, 0.539, 0.54167, 0.54967, 0.54967, 0.54567, 0.54634, 0.54634, 0.57434, 0.55833, 0.55833, 0.53234, 0.557, 0.557, 0.56234, 0.577, 0.577, 0.57234, 0.56967, 0.56967, 0.57767, 0.573, 0.573, 0.563, 0.561, 0.561, 0.57234, 0.55567, 0.55567, 0.56167, 0.55367, 0.55367, 0.54567, 0.535, 0.535, 0.539, 0.52167, 0.52167, 0.50767, 0.51234, 0.51234, 0.499, 0.495, 0.495, 0.49367, 0.48967, 0.49834, 0.507, 0.50567, 0.50567, 0.49767, 0.569, 0.569, 0.57567, 0.577, 0.577, 0.58034, 0.595, 0.595, 0.60767, 0.60833, 0.60833, 0.61034, 0.60767, 0.60767, 0.61767, 0.59433, 0.59433, 0.57767, 0.601, 0.601, 0.60567, 0.62367, 0.62367, 0.62967, 0.627, 0.641, 0.641, 0.64567, 0.65567, 0.65567, 0.66167, 0.68234, 0.68234, 0.689, 0.691, 0.691, 0.697, 0.691, 0.691, 0.697, 0.713, 0.713, 0.71767, 0.70234, 0.709, 0.71567, 0.723, 0.723, 0.72434, 0.70767, 0.70767, 0.70034, 0.69167, 0.69167, 0.70367, 0.687, 0.687, 0.687, 0.697, 0.697, 0.69567, 0.697, 0.697, 0.70167, 0.715, 0.70367, 0.69234, 0.705, 0.705, 0.71234, 0.68967, 0.68967, 0.681, 0.68167, 0.68167, 0.695, 0.671, 0.667, 0.663, 0.679, 0.679, 0.701, 0.68167, 0.68167, 0.649, 0.667, 0.667, 0.677, 0.67434, 0.67434, 0.66567, 0.64967, 0.64967, 0.643, 0.649, 0.649, 0.677, 0.629, 0.629, 0.64234, 0.61233, 0.61233, 0.609, 0.60367, 0.60367, 0.59367, 0.58567, 0.58567, 0.585, 0.577, 0.577, 0.55767, 0.54833, 0.54833, 0.52367, 0.515, 0.515, 0.427, 0.383, 0.396, 0.396, 0.40667, 0.422, 0.422, 0.42967, 0.449, 0.449, 0.483, 0.47867, 0.47867, 0.47467, 0.44767, 0.44733, 0.447, 0.43233, 0.43233, 0.42733, 0.42, 0.42, 0.41467, 0.441, 0.441, 0.439, 0.45133, 0.45133, 0.48034, 0.498, 0.498, 0.516, 0.51634, 0.51634, 0.521, 0.50134, 0.50134, 0.502, 0.51567, 0.51567, 0.518, 0.51934, 0.51934, 0.51967, 0.526, 0.526, 0.53067, 0.53667, 0.53667, 0.54434, 0.54667, 0.53667, 0.53667, 0.53634, 0.53767, 0.52834, 0.519, 0.52467, 0.52467, 0.52234, 0.537, 0.537, 0.54134, 0.547, 0.547, 0.55, 0.554, 0.554, 0.55667, 0.567, 0.567, 0.56934, 0.583, 0.583, 0.58467, 0.58534, 0.591, 0.59667, 0.55567, 0.55567, 0.56634, 0.55267, 0.55267, 0.549, 0.568, 0.57167, 0.57534, 0.577, 0.57434, 0.57167, 0.56867, 0.571, 0.57333, 0.595, 0.60067, 0.60633, 0.61634, 0.62667, 0.637, 0.63567, 0.63567, 0.635, 0.645, 0.645, 0.64834, 0.64134, 0.64134, 0.638, 0.64034, 0.64034, 0.642, 0.65334, 0.65334, 0.65034, 0.65634, 0.65634, 0.665, 0.65434, 0.65434, 0.64434, 0.656, 0.656, 0.658, 0.643, 0.643, 0.62767, 0.647, 0.647, 0.66134, 0.64234, 0.64234, 0.63467, 0.64, 0.64, 0.64534, 0.674, 0.674, 0.67467, 0.66967, 0.66967, 0.67034, 0.66667, 0.66667, 0.66467, 0.66367, 0.66367, 0.65667, 0.64434, 0.64434, 0.64134, 0.62434, 0.62434, 0.621, 0.591, 0.591, 0.58, 0.58333, 0.58333, 0.58267, 0.59067, 0.59067, 0.60234, 0.598, 0.598, 0.60467, 0.586, 0.58633, 0.58667, 0.59633, 0.59633, 0.60967, 0.60234, 0.60234, 0.60133, 0.60633, 0.60633, 0.62567, 0.60633, 0.60633, 0.58833, 0.58667, 0.58667, 0.60767, 0.594, 0.59433, 0.59467, 0.59667, 0.59667, 0.59333, 0.58833, 0.58833, 0.592, 0.60433, 0.60433, 0.609, 0.62367, 0.62, 0.61634, 0.60333, 0.60333, 0.59034, 0.59133, 0.59133, 0.58867, 0.58734, 0.58734, 0.58613, 0.57133, 0.57133, 0.55267, 0.568, 0.568, 0.578, 0.56167, 0.56167, 0.54334, 0.557, 0.557, 0.575, 0.57, 0.57, 0.553, 0.574, 0.574, 0.574, 0.57267, 0.573, 0.57333, 0.57833, 0.57967, 0.581, 0.57434, 0.57434, 0.57534, 0.54967, 0.54967, 0.54967, 0.54967, 0.547, 0.54434, 0.52567, 0.516, 0.50634, 0.495, 0.495, 0.495, 0.48833, 0.47567, 0.463, 0.44967, 0.44967, 0.44233, 0.45667, 0.457, 0.45733, 0.46667, 0.45333, 0.417, 0.399, 0.399, 0.39567, 0.39133, 0.39133, 0.37467, 0.39367, 0.39367, 0.39567, 0.39333, 0.39333, 0.392, 0.379, 0.379, 0.37432, 0.37204, 0.36816], [0.57599, 0.57355, 0.52533, 0.52533, 0.46433, 0.52133, 0.52133, 0.52933, 0.56067, 0.56067, 0.56533, 0.54867, 0.54867, 0.54733, 0.51533, 0.51533, 0.51933, 0.50733, 0.50733, 0.504, 0.478, 0.478, 0.47133, 0.46866, 0.46866, 0.47733, 0.45533, 0.45533, 0.444, 0.46333, 0.46333, 0.49133, 0.48733, 0.48733, 0.48266, 0.50933, 0.50933, 0.51333, 0.50933, 0.50933, 0.506, 0.53, 0.53, 0.53, 0.51867, 0.51867, 0.50333, 0.50667, 0.50667, 0.52133, 0.50867, 0.50867, 0.506, 0.49467, 0.486, 0.47733, 0.45667, 0.45667, 0.454, 0.436, 0.436, 0.39733, 0.40066, 0.40066, 0.40133, 0.38867, 0.38867, 0.38533, 0.37333, 0.37333, 0.37533, 0.36666, 0.36666, 0.36, 0.352, 0.352, 0.35, 0.34333, 0.34333, 0.33, 0.32933, 0.32933, 0.322, 0.302, 0.302, 0.302, 0.29266, 0.29266, 0.28466, 0.27533, 0.27533, 0.27266, 0.26133, 0.26133, 0.25133, 0.22466, 0.22466, 0.20733, 0.202, 0.20533, 0.20866, 0.22933, 0.22933, 0.25533, 0.282, 0.282, 0.28466, 0.29333, 0.29333, 0.29266, 0.28, 0.28, 0.26133, 0.27666, 0.27666, 0.28266, 0.29266, 0.29266, 0.32867, 0.33933, 0.33933, 0.39533, 0.38133, 0.38133, 0.372, 0.36333, 0.36333, 0.36733, 0.37533, 0.38533, 0.38533, 0.37466, 0.388, 0.388, 0.42866, 0.40466, 0.40466, 0.40266, 0.414, 0.414, 0.43133, 0.454, 0.454, 0.45466, 0.44466, 0.44466, 0.45066, 0.47866, 0.47466, 0.47066, 0.468, 0.468, 0.47133, 0.492, 0.492, 0.49667, 0.53067, 0.53067, 0.52467, 0.55, 0.55, 0.55533, 0.574, 0.574, 0.58533, 0.58467, 0.58467, 0.58267, 0.58, 0.59067, 0.60133, 0.60933, 0.60933, 0.614, 0.61333, 0.61333, 0.62067, 0.62733, 0.62733, 0.624, 0.648, 0.654, 0.66, 0.66867, 0.66867, 0.67467, 0.68, 0.68, 0.67933, 0.69733, 0.69733, 0.69667, 0.70067, 0.70067, 0.70267, 0.71333, 0.71333, 0.71933, 0.718, 0.718, 0.716, 0.73467, 0.73467, 0.73467, 0.752, 0.752, 0.75467, 0.76733, 0.76733, 0.776, 0.78733, 0.78733, 0.79467, 0.80267, 0.80267, 0.82533, 0.82467, 0.82467, 0.83067, 0.82933, 0.82933, 0.822, 0.80067, 0.76567, 0.76567, 0.75167, 0.758, 0.758, 0.76833, 0.76467, 0.76467, 0.75933, 0.76533, 0.76533, 0.773, 0.78333, 0.78433, 0.78533, 0.791, 0.791, 0.793, 0.80833, 0.80833, 0.814, 0.80433, 0.80433, 0.80267, 0.796, 0.796, 0.79767, 0.77967, 0.77967, 0.752, 0.76567, 0.76567, 0.78133, 0.81667, 0.81667, 0.82, 0.809, 0.809, 0.81233, 0.80267, 0.80267, 0.79867, 0.78533, 0.78533, 0.76367, 0.776, 0.776, 0.76033, 0.756, 0.74333, 0.74333, 0.73867, 0.739, 0.72633, 0.71367, 0.70633, 0.70633, 0.69533, 0.696, 0.696, 0.69233, 0.69233, 0.69233, 0.689, 0.69, 0.69, 0.69633, 0.693, 0.693, 0.697, 0.69767, 0.69767, 0.702, 0.70467, 0.70567, 0.70667, 0.77833, 0.77833, 0.77967, 0.80033, 0.80033, 0.81133, 0.787, 0.78467, 0.78233, 0.77867, 0.779, 0.77933, 0.77567, 0.77533, 0.775, 0.75, 0.733, 0.716, 0.70933, 0.69933, 0.68933, 0.649, 0.649, 0.63433, 0.61667, 0.61667, 0.61033, 0.58, 0.58, 0.57633, 0.575, 0.575, 0.573, 0.551, 0.551, 0.55133, 0.54533, 0.54533, 0.53533, 0.54033, 0.54033, 0.543, 0.52667, 0.52667, 0.52367, 0.53133, 0.53133, 0.53233, 0.52033, 0.52033, 0.50833, 0.51567, 0.51567, 0.51867, 0.50733, 0.50733, 0.50467, 0.493, 0.493, 0.48666, 0.47833, 0.47833, 0.47167, 0.466, 0.466, 0.45233, 0.451, 0.451, 0.45066, 0.42766, 0.42766, 0.42333, 0.40333, 0.40333, 0.39166, 0.40366, 0.40366, 0.398, 0.40366, 0.40366, 0.40733, 0.41733, 0.41733, 0.42166, 0.43966, 0.43966, 0.442, 0.44666, 0.44866, 0.45066, 0.453, 0.453, 0.45633, 0.46033, 0.46033, 0.467, 0.47633, 0.47633, 0.47766, 0.50467, 0.50467, 0.51733, 0.527, 0.527, 0.54067, 0.56133, 0.56733, 0.57333, 0.60933, 0.60933, 0.61967, 0.64233, 0.64233, 0.651, 0.672, 0.672, 0.679, 0.687, 0.692, 0.697, 0.694, 0.694, 0.69633, 0.68767, 0.68767, 0.686, 0.67767, 0.67767, 0.66444, 0.654, 0.654, 0.64367, 0.637, 0.637, 0.626, 0.62567, 0.62567, 0.62867, 0.61167, 0.61167, 0.59033, 0.58567, 0.58567, 0.584, 0.56867, 0.56867, 0.56867, 0.572, 0.569, 0.566, 0.559, 0.55567, 0.55233, 0.552, 0.552, 0.536, 0.543, 0.543, 0.543, 0.543, 0.54267, 0.54233, 0.54367, 0.54733, 0.551, 0.551, 0.551, 0.551, 0.548, 0.54833, 0.54867, 0.544, 0.544, 0.54467, 0.552, 0.55167, 0.55133, 0.56067, 0.55967, 0.55694, 0.574, 0.574, 0.57633, 0.581, 0.581, 0.58433, 0.56033, 0.56033, 0.558, 0.55633, 0.55633, 0.559, 0.568, 0.568, 0.56962, 0.57184, 0.57599], "MCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCLCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCLCCCCCCCCCCCCCCCCLCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCZ"],
[0x4c0000, -1, [0.32833, 0.32833, 0.34767, 0.352, 0.35633, 0.37967, 0.37967, 0.37967, 0.37067, 0.36633, 0.362, 0.344, 0.344, 0.344, 0.33767, 0.32833], [0.59533, 0.59533, 0.586, 0.58133, 0.57667, 0.55767, 0.55767, 0.55767, 0.561, 0.564, 0.567, 0.58067, 0.58067, 0.58067, 0.59067, 0.59533], "MCCCCCZ"],
[0x99cc32, -1, [0.23165, 0.23221, 0.23134, 0.231, 0.2293, 0.21433, 0.21433, 0.21395, 0.21386, 0.21404, 0.21404, 0.22215, 0.23165], [0.64733, 0.64758, 0.6521, 0.653, 0.65754, 0.66, 0.66, 0.65772, 0.65506, 0.65234, 0.65234, 0.64314, 0.64733], "MCCCCZ"],
[0x659900, -1, [0.23165, 0.2309, 0.23163, 0.23133, 0.22963, 0.21433, 0.21433, 0.21395, 0.21386, 0.21404, 0.21404, 0.22115, 0.23165], [0.64766, 0.6474, 0.65222, 0.653, 0.65754, 0.66017, 0.66017, 0.65789, 0.65523, 0.6525, 0.6525, 0.64397, 0.64766], "MCCCCZ"],
[0, -1, [0.22433, 0.22329, 0.22245, 0.22245, 0.22245, 0.22329, 0.22433, 0.22538, 0.22622, 0.22622, 0.22622, 0.22538, 0.22433], [0.64742, 0.64742, 0.64932, 0.65167, 0.65401, 0.65591, 0.65591, 0.65591, 0.65401, 0.65167, 0.64932, 0.64742, 0.64742], "MCCCCZ"],
[0, -1, [0.22433], [0.65167], "MZ"],
[0, -1, [0.14833, 0.14833, 0.139, 0.18033, 0.18033, 0.20367, 0.20767, 0.20967, 0.22361, 0.22833, 0.23967, 0.25367, 0.25367, 0.25367, 0.26133, 0.266, 0.27067, 0.26533, 0.26533, 0.26533, 0.25433, 0.255, 0.255, 0.24633, 0.21967, 0.21967, 0.19275, 0.195, 0.195, 0.20967, 0.21367, 0.21367, 0.23167, 0.21833, 0.207, 0.207, 0.20723, 0.19033, 0.174, 0.15683, 0.15683, 0.15683, 0.13025, 0.14833], [0.484, 0.484, 0.46733, 0.47733, 0.47733, 0.47933, 0.48333, 0.482, 0.47711, 0.476, 0.47333, 0.49, 0.49, 0.49, 0.5075, 0.5075, 0.5075, 0.50483, 0.50483, 0.50483, 0.488, 0.48533, 0.48533, 0.452, 0.45066, 0.45066, 0.44908, 0.43933, 0.43933, 0.44333, 0.43933, 0.43933, 0.44, 0.42933, 0.41, 0.41, 0.40347, 0.40933, 0.415, 0.4365, 0.4365, 0.4365, 0.46075, 0.484], "MCCCCCCCCCCCLCCCZ"],
[0xe59999, -1, [0.14633, 0.14633, 0.143, 0.20433, 0.20433, 0.21167, 0.21567, 0.21967, 0.23967, 0.243, 0.243, 0.231, 0.21167, 0.21167, 0.18967, 0.19033, 0.19033, 0.197, 0.205, 0.205, 0.20967, 0.209, 0.20833, 0.20367, 0.20033, 0.197, 0.19167, 0.189, 0.18633, 0.17233, 0.165, 0.15767, 0.14367, 0.14433, 0.145, 0.14633, 0.14633], [0.47733, 0.47733, 0.46133, 0.47866, 0.47866, 0.47866, 0.47733, 0.476, 0.47133, 0.47333, 0.47333, 0.45066, 0.45333, 0.45333, 0.45066, 0.44266, 0.44266, 0.43066, 0.42666, 0.42666, 0.42266, 0.41733, 0.412, 0.40933, 0.408, 0.40666, 0.412, 0.412, 0.412, 0.42266, 0.43066, 0.43866, 0.45867, 0.46333, 0.468, 0.47733, 0.47733], "MCCCCCCCCCCCCZ"],
[0xb26565, -1, [0.15167, 0.156, 0.16133, 0.165, 0.17233, 0.18633, 0.189, 0.19167, 0.197, 0.20033, 0.20367, 0.20833, 0.209, 0.20967, 0.205, 0.205, 0.19989, 0.19532, 0.19269, 0.19269, 0.193, 0.18433, 0.17567, 0.167, 0.16433, 0.16167, 0.15767, 0.16033, 0.163, 0.167, 0.16967, 0.17233, 0.17167, 0.16767, 0.16367, 0.159, 0.15167], [0.44825, 0.44166, 0.43466, 0.43066, 0.42266, 0.412, 0.412, 0.412, 0.40666, 0.408, 0.40933, 0.412, 0.41733, 0.42266, 0.42666, 0.42666, 0.42922, 0.43504, 0.43891, 0.43891, 0.43466, 0.436, 0.43733, 0.442, 0.44733, 0.45266, 0.45667, 0.45066, 0.44466, 0.43866, 0.438, 0.43733, 0.43533, 0.436, 0.43666, 0.43733, 0.446], "MCCCCCCCCCCCCZ"],
[0x992600, -1, [0.14733, 0.14733, 0.15033, 0.15233, 0.15233, 0.151, 0.155, 0.159, 0.16233, 0.16733, 0.17233, 0.17267, 0.17933, 0.186, 0.196, 0.20067, 0.20533, 0.205, 0.205, 0.205, 0.21633, 0.239, 0.239, 0.212, 0.23833, 0.23833, 0.23033, 0.23583, 0.2395, 0.23867, 0.22033, 0.22033, 0.212, 0.20333, 0.19467, 0.17467, 0.17267, 0.17067, 0.16533, 0.162, 0.15867, 0.154, 0.15333, 0.15333, 0.15133, 0.149, 0.14667, 0.14633, 0.14633, 0.14633, 0.143, 0.14333, 0.14333, 0.14467, 0.144, 0.14733], [0.48333, 0.48333, 0.506, 0.51267, 0.51267, 0.524, 0.531, 0.538, 0.54833, 0.55733, 0.56633, 0.573, 0.57567, 0.57833, 0.59267, 0.59433, 0.596, 0.59467, 0.59467, 0.59467, 0.61933, 0.61267, 0.61267, 0.61733, 0.633, 0.633, 0.63117, 0.64283, 0.65061, 0.63933, 0.61967, 0.61967, 0.60533, 0.60033, 0.59533, 0.58367, 0.57733, 0.571, 0.56133, 0.55867, 0.556, 0.549, 0.54333, 0.54333, 0.53667, 0.53467, 0.53267, 0.52733, 0.524, 0.52067, 0.516, 0.512, 0.512, 0.48033, 0.477, 0.48333], "MCCCCCCCCCCCCCCCCCCLZ"],
[0xffffff, -1, [0.133, 0.133, 0.12967, 0.12233, 0.12233, 0.1345, 0.1345, 0.1345, 0.13633, 0.13417, 0.132, 0.1305, 0.1305, 0.133], [0.47566, 0.47566, 0.478, 0.468, 0.468, 0.413, 0.41066, 0.41066, 0.41416, 0.42616, 0.43816, 0.45933, 0.45933, 0.47566], "MCCCCLZ"],
[0x992600, -1, [0.169, 0.169, 0.13967, 0.14033, 0.139, 0.139, 0.137, 0.135, 0.133, 0.13967, 0.13433, 0.13433, 0.111, 0.12433, 0.12433, 0.12683, 0.12183, 0.12183, 0.11417, 0.116, 0.116, 0.11633, 0.1195, 0.1195, 0.13383, 0.13833, 0.13833, 0.14133, 0.167, 0.167, 0.1765, 0.169], [0.57667, 0.57667, 0.57133, 0.52267, 0.48133, 0.48133, 0.524, 0.52667, 0.52933, 0.548, 0.538, 0.538, 0.51467, 0.47933, 0.47933, 0.47383, 0.4815, 0.4815, 0.5025, 0.51317, 0.51317, 0.51683, 0.5215, 0.5215, 0.541, 0.54483, 0.54483, 0.56883, 0.5775, 0.5775, 0.58133, 0.57667], "MCLCCCCCCCCCZ"],
[0, -1, [0.40133, 0.40243, 0.40242, 0.40338, 0.4053, 0.40557, 0.40644, 0.40789, 0.40821, 0.40918, 0.40964, 0.40968, 0.40916, 0.4072, 0.40603, 0.40363, 0.40318, 0.40275, 0.40241, 0.40164, 0.39954, 0.39811, 0.39763, 0.39851, 0.39731, 0.39582, 0.3934, 0.39302, 0.39204, 0.39372, 0.39533, 0.39403, 0.39459, 0.39484, 0.39602, 0.39403, 0.39276, 0.39272, 0.39329, 0.39324, 0.39121, 0.38879, 0.38576, 0.38449, 0.38304, 0.38221, 0.3816, 0.38092, 0.38133, 0.37561, 0.37186, 0.36751, 0.36674, 0.36722, 0.36814, 0.3695, 0.37109, 0.37187, 0.37252, 0.37311, 0.37393, 0.37415, 0.37385, 0.37408, 0.37846, 0.38124, 0.38533, 0.38858, 0.39114, 0.39406, 0.39457, 0.39544, 0.39593, 0.39885, 0.39885, 0.39902, 0.39911, 0.39943, 0.40133], [0.687, 0.68759, 0.68921, 0.6895, 0.69007, 0.69185, 0.69315, 0.69535, 0.69791, 0.70039, 0.70156, 0.70315, 0.70425, 0.70838, 0.71247, 0.71651, 0.71726, 0.71858, 0.71958, 0.72189, 0.72354, 0.72574, 0.72647, 0.72802, 0.72816, 0.72833, 0.7293, 0.72759, 0.72328, 0.71907, 0.715, 0.71385, 0.71231, 0.71103, 0.70502, 0.69944, 0.69365, 0.69347, 0.69314, 0.69304, 0.68856, 0.68449, 0.68052, 0.67887, 0.67734, 0.67557, 0.67426, 0.67265, 0.671, 0.66637, 0.65996, 0.65355, 0.65242, 0.6504, 0.64995, 0.64929, 0.651, 0.6524, 0.65357, 0.65465, 0.65572, 0.65602, 0.65671, 0.65692, 0.66083, 0.66572, 0.66967, 0.67022, 0.67188, 0.67357, 0.67386, 0.67345, 0.67378, 0.67574, 0.67913, 0.68231, 0.68379, 0.68598, 0.687], "MCCCCCCCCCCCCCCCCCCCCCCCCCCZ"],
[0, -1, [0.3866, 0.3868, 0.38655, 0.38673, 0.387, 0.38767, 0.38794, 0.38812, 0.38785, 0.38808, 0.39196, 0.39239, 0.39067, 0.39237, 0.39248, 0.39177, 0.39034, 0.39002, 0.3884, 0.38706, 0.38443, 0.38215, 0.38144, 0.38091, 0.38137, 0.38148, 0.38196, 0.38191, 0.38173, 0.38082, 0.38081, 0.38076, 0.37917, 0.38022, 0.38151, 0.38287, 0.384, 0.38194, 0.38366, 0.38074, 0.38052, 0.38053, 0.38074, 0.38122, 0.3819, 0.38271, 0.38304, 0.38362, 0.38396, 0.38492, 0.38558, 0.3866], [0.69444, 0.69457, 0.69528, 0.69564, 0.69618, 0.69649, 0.69703, 0.69739, 0.69802, 0.69826, 0.70234, 0.70738, 0.71233, 0.71337, 0.71547, 0.71688, 0.71972, 0.72292, 0.7255, 0.72761, 0.72968, 0.72751, 0.72685, 0.72554, 0.72435, 0.72408, 0.72383, 0.7237, 0.72316, 0.72278, 0.72233, 0.71984, 0.71732, 0.7151, 0.71239, 0.70929, 0.70633, 0.70281, 0.69872, 0.6956, 0.69535, 0.69471, 0.69437, 0.69356, 0.69289, 0.6924, 0.6922, 0.6922, 0.6924, 0.693, 0.69382, 0.69444], "MCCCCCCCCCCCCCCCCCZ"],
[0, -1, [0.49128, 0.4936, 0.49408, 0.49067, 0.49159, 0.49717, 0.50067, 0.50049, 0.50086, 0.50134, 0.5031, 0.50423, 0.506, 0.50672, 0.50946, 0.51053, 0.5134, 0.51242, 0.50811, 0.50778, 0.50814, 0.50793, 0.50667, 0.50322, 0.5, 0.49804, 0.49694, 0.494, 0.49131, 0.49015, 0.48792, 0.4857, 0.48475, 0.48482, 0.48483, 0.48558, 0.4853, 0.48518, 0.48474, 0.48474, 0.48474, 0.48511, 0.48534, 0.48381, 0.48143, 0.48094, 0.47935, 0.48364, 0.48642, 0.4874, 0.48618, 0.48491, 0.48418, 0.48436, 0.48482, 0.48608, 0.48873, 0.49128], [0.71163, 0.70864, 0.70398, 0.70167, 0.69615, 0.69945, 0.70033, 0.70098, 0.70155, 0.70156, 0.70159, 0.70331, 0.703, 0.7056, 0.70677, 0.70906, 0.71522, 0.72234, 0.72776, 0.72818, 0.72904, 0.72964, 0.73333, 0.73385, 0.735, 0.74146, 0.74818, 0.75433, 0.75475, 0.75765, 0.75885, 0.76005, 0.75742, 0.75567, 0.75533, 0.75496, 0.75435, 0.75408, 0.75389, 0.75367, 0.75344, 0.75322, 0.753, 0.75163, 0.75084, 0.74893, 0.74275, 0.73754, 0.7322, 0.73031, 0.72819, 0.72618, 0.72502, 0.72314, 0.72172, 0.71783, 0.71493, 0.71163], "MCCCCCCCCCCCCCCCCCCCZ"],
[0, -1, [0.42935, 0.42687, 0.42119, 0.42605, 0.42637, 0.42699, 0.42728, 0.43064, 0.43398, 0.43804, 0.43825, 0.43871, 0.43932, 0.44201, 0.44536, 0.44733, 0.45361, 0.45955, 0.4652, 0.46714, 0.46926, 0.47126, 0.47355, 0.47556, 0.47741, 0.47763, 0.47822, 0.47867, 0.4786, 0.4802, 0.48062, 0.48077, 0.48052, 0.48074, 0.4843, 0.48584, 0.48389, 0.48342, 0.483, 0.48206, 0.48025, 0.47841, 0.47667, 0.4764, 0.47519, 0.47454, 0.47311, 0.47089, 0.46946, 0.46719, 0.46512, 0.46275, 0.46223, 0.46093, 0.46067, 0.46044, 0.4602, 0.46002, 0.45684, 0.45474, 0.45257, 0.4524, 0.45166, 0.4514, 0.4498, 0.44913, 0.44729, 0.44696, 0.44637, 0.44605, 0.44497, 0.44436, 0.44329, 0.44274, 0.44208, 0.44213, 0.44254, 0.44362, 0.44267, 0.44613, 0.45032, 0.45333, 0.45336, 0.45451, 0.45432, 0.45431, 0.45383, 0.45362, 0.4531, 0.45461, 0.4532, 0.45086, 0.44871, 0.44733, 0.44427, 0.44083, 0.4382, 0.43652, 0.43556, 0.43422, 0.43258, 0.43314, 0.43326, 0.43327, 0.43274, 0.43274, 0.43274, 0.43311, 0.43333, 0.43216, 0.4317, 0.43, 0.43051, 0.42938, 0.42793, 0.4246, 0.42181, 0.41866, 0.4178, 0.41702, 0.41594, 0.41522, 0.41405, 0.41344, 0.4123, 0.41132, 0.41005, 0.40736, 0.40523, 0.40276, 0.40028, 0.39869, 0.39689, 0.39532, 0.3951, 0.39746, 0.40054, 0.40271, 0.40599, 0.40651, 0.40685, 0.40667, 0.40732, 0.40768, 0.408, 0.40943, 0.41137, 0.41293, 0.41455, 0.41746, 0.41915, 0.4217, 0.42077, 0.424, 0.42302, 0.42201, 0.42145, 0.42098, 0.42264, 0.42466, 0.42676, 0.42719, 0.428, 0.42844, 0.42923, 0.42916, 0.4284, 0.42669, 0.42592, 0.42582, 0.42532, 0.42467, 0.42389, 0.41713, 0.41289, 0.41222, 0.41221, 0.4129, 0.41523, 0.4185, 0.42133, 0.42169, 0.42312, 0.42534, 0.42577, 0.42616, 0.4267, 0.42728, 0.42812, 0.42859, 0.43147, 0.43382, 0.43672, 0.43704, 0.43761, 0.43797, 0.43851, 0.43882, 0.43936, 0.43994, 0.44034, 0.44067, 0.43959, 0.43958, 0.43861, 0.43732, 0.43639, 0.43526, 0.43478, 0.43368, 0.4335, 0.43251, 0.43054, 0.42935], [0.68098, 0.6779, 0.67362, 0.6704, 0.67019, 0.67018, 0.67041, 0.67302, 0.67452, 0.67549, 0.67554, 0.67476, 0.67503, 0.6762, 0.6761, 0.67833, 0.67796, 0.67982, 0.682, 0.68275, 0.68368, 0.68452, 0.68548, 0.68702, 0.68892, 0.68915, 0.689, 0.689, 0.69044, 0.69065, 0.69169, 0.69207, 0.69273, 0.6929, 0.69562, 0.69898, 0.70294, 0.70391, 0.70494, 0.70573, 0.70725, 0.70583, 0.70633, 0.70529, 0.70557, 0.70524, 0.70451, 0.70549, 0.70476, 0.7036, 0.70332, 0.70269, 0.70255, 0.70271, 0.70167, 0.70189, 0.70228, 0.70224, 0.70158, 0.70123, 0.69841, 0.69818, 0.69848, 0.69826, 0.69691, 0.69486, 0.69374, 0.69353, 0.69381, 0.6936, 0.69288, 0.69181, 0.69106, 0.69068, 0.69122, 0.69165, 0.6949, 0.69787, 0.701, 0.7052, 0.70845, 0.713, 0.71661, 0.72019, 0.72365, 0.72397, 0.72519, 0.72577, 0.72719, 0.72897, 0.73018, 0.73218, 0.73072, 0.72833, 0.72768, 0.72653, 0.72858, 0.7299, 0.73141, 0.73317, 0.73534, 0.73773, 0.74034, 0.74055, 0.74078, 0.741, 0.74122, 0.74144, 0.74167, 0.74271, 0.74447, 0.745, 0.74682, 0.74829, 0.7488, 0.74995, 0.74676, 0.74664, 0.74661, 0.74834, 0.74888, 0.74924, 0.7493, 0.74885, 0.74801, 0.74782, 0.74748, 0.74675, 0.74493, 0.7435, 0.74208, 0.73968, 0.73748, 0.73556, 0.73154, 0.7307, 0.72962, 0.73413, 0.73358, 0.7335, 0.73298, 0.73233, 0.73215, 0.73253, 0.733, 0.7313, 0.73074, 0.72928, 0.72778, 0.72848, 0.72681, 0.72431, 0.71984, 0.71767, 0.71548, 0.71335, 0.71097, 0.70896, 0.70697, 0.70712, 0.70728, 0.70854, 0.71033, 0.70989, 0.70939, 0.70903, 0.70506, 0.70173, 0.69768, 0.69715, 0.69682, 0.697, 0.69008, 0.6861, 0.68082, 0.67998, 0.6779, 0.67723, 0.67492, 0.6775, 0.67833, 0.68034, 0.68191, 0.68182, 0.6818, 0.68271, 0.68292, 0.68315, 0.68278, 0.68311, 0.6851, 0.68694, 0.68893, 0.68914, 0.68888, 0.68906, 0.68933, 0.68998, 0.69028, 0.69062, 0.69013, 0.68967, 0.68908, 0.68749, 0.68715, 0.68671, 0.68585, 0.68511, 0.68478, 0.6852, 0.6849, 0.68319, 0.68246, 0.68098], "MCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCZ"],
[0, -1, [0.337, 0.337, 0.3216, 0.303, 0.303, 0.299, 0.295, 0.291, 0.27233, 0.269, 0.25167, 0.25167, 0.27633, 0.28167, 0.28167, 0.295, 0.28967, 0.28967, 0.26633, 0.26833, 0.26833, 0.259, 0.25767, 0.25767, 0.28433, 0.28833, 0.29233, 0.29433, 0.29233, 0.29033, 0.28967, 0.285, 0.285, 0.29833, 0.297, 0.297, 0.30233, 0.30633, 0.30433, 0.31167, 0.31167, 0.30767, 0.31033, 0.31033, 0.307, 0.31367, 0.32033, 0.33633, 0.33633, 0.33633, 0.32767, 0.31233, 0.30567, 0.30367, 0.30367, 0.29767, 0.305, 0.31233, 0.31167, 0.31167, 0.31167, 0.32233, 0.325, 0.325, 0.347, 0.349, 0.349, 0.36033, 0.345, 0.345, 0.32033, 0.32233, 0.33433, 0.34033, 0.33767, 0.33767], [0.76333, 0.76333, 0.76812, 0.72533, 0.72533, 0.71667, 0.71333, 0.71, 0.704, 0.69733, 0.67067, 0.67067, 0.69733, 0.70133, 0.70133, 0.71533, 0.704, 0.704, 0.686, 0.67067, 0.67067, 0.64667, 0.64333, 0.64333, 0.69667, 0.69867, 0.70067, 0.70067, 0.69467, 0.68867, 0.66133, 0.658, 0.658, 0.692, 0.69733, 0.69733, 0.70333, 0.69467, 0.668, 0.648, 0.648, 0.66667, 0.69267, 0.69267, 0.71, 0.70067, 0.69133, 0.68133, 0.67333, 0.67333, 0.70267, 0.71067, 0.70067, 0.704, 0.704, 0.70533, 0.71667, 0.728, 0.72933, 0.72933, 0.72933, 0.71733, 0.71733, 0.71733, 0.73, 0.68933, 0.68933, 0.71333, 0.72467, 0.72467, 0.728, 0.73667, 0.75733, 0.766, 0.76133, 0.76133], "MCCCLCCCCCCCCCLLCCCCLLCCCCCCLCZ"],
[0, -1, [0.30367, 0.30367, 0.28233, 0.277, 0.265, 0.265, 0.29367, 0.30033, 0.307, 0.30367, 0.30367], [0.736, 0.736, 0.736, 0.72733, 0.71133, 0.71133, 0.728, 0.73, 0.732, 0.736, 0.736], "MCLCCZ"],
[0, -1, [0.237, 0.237, 0.23367, 0.233, 0.23233, 0.22833, 0.22967, 0.231, 0.23433, 0.23433, 0.23433, 0.237, 0.23833, 0.23967, 0.24233, 0.237], [0.72533, 0.72533, 0.72333, 0.71867, 0.714, 0.71333, 0.70867, 0.704, 0.7, 0.70667, 0.71333, 0.71667, 0.71867, 0.72067, 0.728, 0.72533], "MCCCCCZ"],
[0, -1, [0.22233, 0.22233, 0.20833, 0.203, 0.19767, 0.19842, 0.19033, 0.18059, 0.18233, 0.18233, 0.17567, 0.17567, 0.17367, 0.187, 0.19351, 0.19567, 0.193, 0.19033, 0.20233, 0.19767, 0.193, 0.217, 0.213, 0.22233], [0.62333, 0.62333, 0.63, 0.636, 0.642, 0.63339, 0.634, 0.63474, 0.66133, 0.66133, 0.64867, 0.64867, 0.62467, 0.62867, 0.63062, 0.628, 0.62667, 0.62533, 0.62467, 0.622, 0.61933, 0.628, 0.61067, 0.62333], "MCCCLCCCCLZ"],
[0, -1, [0.20133, 0.20133, 0.17567, 0.16967, 0.16967, 0.16167, 0.16533, 0.169, 0.171, 0.171, 0.171, 0.18, 0.179, 0.178, 0.174, 0.174, 0.174, 0.191, 0.20133], [0.59933, 0.59933, 0.592, 0.608, 0.608, 0.604, 0.599, 0.594, 0.59333, 0.59333, 0.59333, 0.59133, 0.59, 0.58867, 0.583, 0.583, 0.583, 0.593, 0.59933], "MCCCCCCZ"],
[0xffffff, -1, [0.582, 0.58129, 0.57851, 0.57534, 0.57214, 0.56781, 0.56467, 0.56388, 0.56258, 0.56201, 0.56123, 0.56168, 0.56111, 0.56021, 0.55902, 0.55934, 0.56235, 0.56334, 0.56255, 0.56243, 0.56172, 0.56205, 0.56236, 0.56289, 0.56333, 0.56311, 0.56287, 0.56268, 0.56167, 0.56187, 0.56209, 0.56307, 0.56642, 0.56867, 0.5691, 0.56994, 0.57067, 0.57058, 0.57126, 0.5716, 0.57246, 0.57517, 0.5765, 0.5783, 0.58007, 0.58186, 0.58488, 0.58773, 0.58973, 0.59068, 0.59108, 0.59099, 0.59092, 0.58862, 0.58805, 0.58698, 0.59003, 0.59129, 0.59162, 0.59118, 0.59065, 0.58997, 0.58866, 0.58889, 0.59051, 0.58592, 0.582], [0.469, 0.46544, 0.46411, 0.463, 0.4646, 0.46977, 0.46633, 0.46713, 0.46723, 0.46833, 0.46983, 0.47158, 0.4729, 0.47502, 0.4773, 0.47966, 0.48085, 0.48403, 0.48697, 0.4874, 0.48772, 0.4883, 0.48884, 0.48922, 0.48967, 0.48944, 0.48905, 0.48909, 0.48926, 0.49032, 0.49097, 0.49388, 0.49432, 0.49233, 0.49328, 0.49295, 0.493, 0.49397, 0.49484, 0.49554, 0.49737, 0.49553, 0.49655, 0.49792, 0.49909, 0.49808, 0.4964, 0.49438, 0.49148, 0.49008, 0.48795, 0.48635, 0.48528, 0.48586, 0.48431, 0.4814, 0.48054, 0.4783, 0.47772, 0.47722, 0.47705, 0.47684, 0.47716, 0.4764, 0.47108, 0.46995, 0.469], "MCCCCCCCCCCCCCCCCCCCCCCZ"],
[0xffffff, -1, [0.566, 0.56599, 0.56332, 0.56534, 0.56556, 0.56578, 0.566, 0.56623, 0.56644, 0.56667, 0.56916, 0.57525, 0.57501, 0.57497, 0.57316, 0.57467, 0.57165, 0.57156, 0.57, 0.56794, 0.56592, 0.564, 0.56459, 0.56439, 0.56576, 0.56648, 0.566, 0.566], [0.43633, 0.43923, 0.44216, 0.445, 0.44478, 0.44441, 0.44441, 0.44441, 0.44478, 0.445, 0.4413, 0.43976, 0.43502, 0.43427, 0.43274, 0.43166, 0.42942, 0.42547, 0.42233, 0.42281, 0.42341, 0.42433, 0.42681, 0.42962, 0.43182, 0.43298, 0.43478, 0.43633], "MCCCCCCCCCZ"],
[0xcccccc, -1, [0.289, 0.289, 0.2609, 0.28433, 0.299, 0.31567, 0.31567, 0.31567, 0.333, 0.339, 0.345, 0.37033, 0.37567, 0.381, 0.397, 0.40833, 0.41967, 0.433, 0.433, 0.433, 0.40567, 0.39967, 0.39367, 0.38167, 0.37167, 0.37167, 0.347, 0.34167, 0.33633, 0.319, 0.31633, 0.31367, 0.317, 0.319, 0.321, 0.31767, 0.30433, 0.291, 0.289, 0.289], [0.45133, 0.45133, 0.43444, 0.46, 0.476, 0.48533, 0.48533, 0.48533, 0.49267, 0.49467, 0.49667, 0.50533, 0.506, 0.50667, 0.51333, 0.50667, 0.5, 0.49267, 0.49267, 0.49267, 0.50667, 0.50267, 0.49867, 0.49933, 0.494, 0.494, 0.48666, 0.48333, 0.48, 0.46066, 0.462, 0.46333, 0.464, 0.46866, 0.47333, 0.476, 0.46533, 0.45466, 0.45133, 0.45133], "MCCCCCCCCCCCCCZ"],
[0, -1, [0.30134, 0.30134, 0.30369, 0.31782, 0.31782, 0.33153, 0.33607, 0.33607, 0.34963, 0.35182, 0.38262, 0.40715, 0.40806, 0.40897, 0.44168, 0.44769, 0.44834, 0.43064, 0.41448, 0.40069, 0.36469, 0.34651, 0.34155, 0.32665, 0.32243, 0.31821, 0.30134, 0.30134], [0.46128, 0.46128, 0.48301, 0.48024, 0.48024, 0.48718, 0.49046, 0.49046, 0.4933, 0.49433, 0.50883, 0.5013, 0.50316, 0.50503, 0.49317, 0.48636, 0.48562, 0.49569, 0.49884, 0.50153, 0.49843, 0.4892, 0.48668, 0.47704, 0.47723, 0.47742, 0.46128, 0.46128], "MCCCCCCCCCZ"],
[0xcccccc, -1, [0.295, 0.295, 0.26967, 0.29767, 0.29767, 0.32767, 0.33433, 0.33433, 0.357, 0.36167, 0.36633, 0.41633, 0.417, 0.41767, 0.427, 0.42967, 0.43233, 0.431, 0.42633, 0.42167, 0.36967, 0.359, 0.34833, 0.329, 0.321, 0.313, 0.295, 0.295], [0.41866, 0.41866, 0.42266, 0.42533, 0.42533, 0.42866, 0.43733, 0.43733, 0.45266, 0.45333, 0.454, 0.466, 0.47, 0.474, 0.474, 0.47266, 0.47133, 0.46933, 0.468, 0.46667, 0.43933, 0.43733, 0.43533, 0.42266, 0.42066, 0.41866, 0.41866, 0.41866], "MCCCCCCCCCZ"],
[0, -1, [0.32753, 0.32753, 0.31323, 0.32756, 0.32756, 0.34226, 0.34567, 0.34567, 0.35728, 0.35967, 0.36206, 0.38532, 0.38566, 0.38601, 0.44278, 0.45015, 0.455, 0.4385, 0.42244, 0.42018, 0.36376, 0.3583, 0.35284, 0.34294, 0.33884, 0.33475, 0.32753, 0.32753], [0.43099, 0.43099, 0.43238, 0.43374, 0.43374, 0.43945, 0.44389, 0.44389, 0.45174, 0.45208, 0.45242, 0.45856, 0.46061, 0.46266, 0.48363, 0.47831, 0.47481, 0.4776, 0.47026, 0.46922, 0.44491, 0.44389, 0.44286, 0.43638, 0.43535, 0.43433, 0.43099, 0.43099], "MCCCCCCCCCZ"],
[0, -1, [0.31433, 0.31433, 0.323, 0.321, 0.319, 0.315, 0.315, 0.31433], [0.42733, 0.42733, 0.428, 0.42533, 0.42266, 0.424, 0.424, 0.42733], "MCCLZ"],
[0, -1, [0.30233, 0.30233, 0.311, 0.309, 0.307, 0.303, 0.303, 0.30233], [0.42466, 0.42466, 0.42533, 0.42266, 0.42, 0.42133, 0.42133, 0.42466], "MCCLZ"],
[0, -1, [0.285, 0.285, 0.29367, 0.29167, 0.28967, 0.28567, 0.28567, 0.285], [0.422, 0.422, 0.42266, 0.42, 0.41733, 0.41866, 0.41866, 0.422], "MCCLZ"],
[0, -1, [0.27233, 0.27233, 0.281, 0.279, 0.277, 0.273, 0.273, 0.27233], [0.42066, 0.42066, 0.42133, 0.41866, 0.416, 0.41733, 0.41733, 0.42066], "MCCLZ"],
[0, -1, [0.33633, 0.33633, 0.34367, 0.34167, 0.33967, 0.33433, 0.33433, 0.33633], [0.48666, 0.48666, 0.48666, 0.484, 0.48133, 0.482, 0.482, 0.48666], "MCCLZ"],
[0, -1, [0.31967, 0.31967, 0.33051, 0.32633, 0.32433, 0.32033, 0.32033, 0.31967], [0.47733, 0.47733, 0.4809, 0.47533, 0.47266, 0.474, 0.474, 0.47733], "MCCLZ"],
[0, -1, [0.301, 0.301, 0.30967, 0.30767, 0.30567, 0.30167, 0.30167, 0.301], [0.46933, 0.46933, 0.47, 0.46733, 0.46466, 0.466, 0.466, 0.46933], "MCCLZ"],
[0, -1, [0.28833, 0.28833, 0.297, 0.295, 0.293, 0.289, 0.289, 0.28833], [0.45933, 0.45933, 0.46, 0.45733, 0.45466, 0.456, 0.456, 0.45933], "MCCLZ"],
[0, -1, [0.277, 0.277, 0.28567, 0.28367, 0.28167, 0.27767, 0.27767, 0.277], [0.45133, 0.45133, 0.452, 0.44933, 0.44666, 0.448, 0.448, 0.45133], "MCCLZ"],
[0, -1, [0.34214, 0.34214, 0.35367, 0.35101, 0.34835, 0.34302, 0.34302, 0.34214], [0.44067, 0.44067, 0.44156, 0.43801, 0.43446, 0.43623, 0.43623, 0.44067], "MCCLZ"],
[0, -1, [0.35947, 0.35947, 0.371, 0.36834, 0.36568, 0.36036, 0.36036, 0.35947], [0.44867, 0.44867, 0.44956, 0.44601, 0.44246, 0.44423, 0.44423, 0.44867], "MCCLZ"],
[0, -1, [0.37747, 0.37747, 0.389, 0.38634, 0.38368, 0.37836, 0.37836, 0.37747], [0.456, 0.456, 0.45689, 0.45334, 0.44979, 0.45157, 0.45157, 0.456], "MCCLZ"],
[0, -1, [0.3948, 0.3948, 0.40634, 0.40368, 0.40102, 0.39569, 0.39569, 0.3948], [0.464, 0.464, 0.46489, 0.46134, 0.45779, 0.45957, 0.45957, 0.464], "MCCLZ"],
[0, -1, [0.3488, 0.3488, 0.36034, 0.35768, 0.35502, 0.34836, 0.34836, 0.3488], [0.494, 0.494, 0.49489, 0.49134, 0.48779, 0.48823, 0.48823, 0.494], "MCCLZ"],
[0, -1, [0.36547, 0.36547, 0.377, 0.37434, 0.37168, 0.36436, 0.36436, 0.36547], [0.49934, 0.49934, 0.50022, 0.49668, 0.49312, 0.49357, 0.49357, 0.49934], "MCCLZ"],
[0, -1, [0.32767, 0.32767, 0.33633, 0.33433, 0.33233, 0.32833, 0.32833, 0.32767], [0.43266, 0.43266, 0.43333, 0.43066, 0.428, 0.42933, 0.42933, 0.43266], "MCCLZ"],
[0x992600, -1, [0.20567, 0.20567, 0.19833, 0.19767, 0.19767, 0.199, 0.201, 0.203, 0.20567, 0.20567], [0.598, 0.598, 0.58333, 0.578, 0.578, 0.59267, 0.596, 0.59933, 0.598, 0.598], "MCCCZ"],
[0x992600, -1, [0.175, 0.175, 0.16967, 0.17033, 0.17033, 0.16833, 0.169, 0.16967, 0.175, 0.175], [0.57467, 0.57467, 0.55067, 0.546, 0.546, 0.56533, 0.56733, 0.56933, 0.57467, 0.57467], "MCCCZ"],
[0xcccccc, -1, [0.20967, 0.20933, 0.20567, 0.20567, 0.22933, 0.23033, 0.23033, 0.23167, 0.20967], [0.699, 0.69367, 0.69333, 0.69333, 0.67233, 0.65967, 0.65967, 0.67333, 0.699], "MLLCCZ"],
[0, -1, [0.21631, 0.21559, 0.21595, 0.21527, 0.21393, 0.21744, 0.21706, 0.21642, 0.21673, 0.21653, 0.21643, 0.21741, 0.21805, 0.22046, 0.21826, 0.22099, 0.2215, 0.22212, 0.2226, 0.2237, 0.22567, 0.22725, 0.22778, 0.22745, 0.2284, 0.22959, 0.23166, 0.23158, 0.23139, 0.22918, 0.22704, 0.2278, 0.22703, 0.22656, 0.22433, 0.22465, 0.22436, 0.22435, 0.22384, 0.22385, 0.22444, 0.22541, 0.22688, 0.22749, 0.22827, 0.22852, 0.22871, 0.22888, 0.2282, 0.23159, 0.22941, 0.23136, 0.2317, 0.23452, 0.23375, 0.22956, 0.22938, 0.22908, 0.22884, 0.22829, 0.22789, 0.22779, 0.2275, 0.22737, 0.22485, 0.22711, 0.22483, 0.22245, 0.22084, 0.21897, 0.21864, 0.22054, 0.22024, 0.21842, 0.2165, 0.21706, 0.21732, 0.21755, 0.21631], [0.69689, 0.69758, 0.69883, 0.69926, 0.70012, 0.70022, 0.70141, 0.70342, 0.70345, 0.70558, 0.70658, 0.70914, 0.70988, 0.71263, 0.71752, 0.7201, 0.72058, 0.7215, 0.7222, 0.7238, 0.72462, 0.726, 0.72646, 0.72784, 0.72768, 0.72749, 0.72771, 0.72631, 0.72277, 0.71991, 0.71713, 0.71595, 0.7149, 0.71397, 0.7096, 0.70486, 0.70013, 0.69999, 0.69986, 0.69977, 0.69588, 0.69222, 0.6885, 0.68695, 0.68546, 0.68391, 0.68277, 0.68138, 0.68021, 0.67539, 0.67097, 0.66509, 0.66404, 0.66086, 0.66112, 0.66258, 0.66324, 0.66449, 0.66553, 0.66783, 0.66883, 0.6691, 0.67224, 0.67245, 0.67642, 0.67612, 0.68005, 0.68119, 0.68303, 0.68496, 0.6853, 0.68651, 0.68686, 0.68901, 0.69029, 0.69278, 0.69393, 0.69569, 0.69689], "MCCCCCCCCCCCCCCCCCCCCCCCCCCZ"],
[0, -1, [0.21033, 0.21033, 0.211, 0.215, 0.219, 0.217, 0.21167, 0.20633, 0.20833, 0.20833, 0.20833, 0.20367, 0.20767, 0.21167, 0.21767, 0.215, 0.21233, 0.19967, 0.19967, 0.19967, 0.198, 0.198, 0.198, 0.19983, 0.20783, 0.20783, 0.21017, 0.21033], [0.694, 0.694, 0.68267, 0.68, 0.67733, 0.67867, 0.68067, 0.68267, 0.684, 0.684, 0.684, 0.68333, 0.68, 0.67667, 0.67267, 0.67267, 0.67267, 0.67933, 0.684, 0.68867, 0.6955, 0.6955, 0.6955, 0.69683, 0.69667, 0.69667, 0.6955, 0.694], "MCCCCCCCCCZ"],
[0xffffff, 0, [0.209, 0.209, 0.1948, 0.164, 0.164, 0.17905, 0.21033, 0.2275, 0.209, 0.209], [0.663, 0.663, 0.66765, 0.662, 0.662, 0.66546, 0.66167, 0.65958, 0.663, 0.663], "MCCCZ"],
[0xffffff, 0, [0.2125, 0.2125, 0.19875, 0.16758, 0.16758, 0.18288, 0.21371, 0.23063, 0.2125, 0.2125], [0.66312, 0.66312, 0.66898, 0.66601, 0.66601, 0.66816, 0.66168, 0.65812, 0.66312, 0.66312], "MCCCZ"],
[0xffffff, 0, [0.21548, 0.21548, 0.20221, 0.1709, 0.1709, 0.18631, 0.21658, 0.23319, 0.21548, 0.21548], [0.66296, 0.66296, 0.66983, 0.66919, 0.66919, 0.6702, 0.66143, 0.65662, 0.66296, 0.66296], "MCCCZ"],
[0xffffff, 0, [0.21759, 0.21759, 0.20622, 0.1781, 0.1781, 0.192, 0.21846, 0.23299, 0.21759, 0.21759], [0.66259, 0.66259, 0.66977, 0.6716, 0.6716, 0.67132, 0.66113, 0.65555, 0.66259, 0.66259], "MCCCZ"],
[0xffffff, 0, [0.40973, 0.40973, 0.40818, 0.40854, 0.4089, 0.45591, 0.46194, 0.46194, 0.41152, 0.40973], [0.64506, 0.64506, 0.64399, 0.64596, 0.64793, 0.66988, 0.66946, 0.66946, 0.64745, 0.64506], "MCCCZ"],
[0xffffff, 0, [0.40408, 0.40408, 0.40262, 0.40282, 0.40302, 0.44816, 0.4542, 0.4542, 0.40567, 0.40408], [0.644, 0.644, 0.64281, 0.6448, 0.64679, 0.67236, 0.67242, 0.67242, 0.64652, 0.644], "MCCCZ"],
[0xffffff, 0, [0.3986, 0.3986, 0.39722, 0.39729, 0.39736, 0.43039, 0.44674, 0.44674, 0.41669, 0.3986], [0.64171, 0.64171, 0.64042, 0.64242, 0.64442, 0.67025, 0.67337, 0.67337, 0.65983, 0.64171], "MCCCZ"],
[0xffffff, 0, [0.39381, 0.39381, 0.39256, 0.39263, 0.39269, 0.42242, 0.43713, 0.43713, 0.41009, 0.39381], [0.6386, 0.6386, 0.63745, 0.63925, 0.64104, 0.66429, 0.6671, 0.6671, 0.65491, 0.6386], "MCCCZ"],
[0xcccccc, -1, [0.341, 0.341, 0.31567, 0.34367, 0.34367, 0.37367, 0.38033, 0.38033, 0.403, 0.40767, 0.41233, 0.43967, 0.44033, 0.441, 0.451, 0.45367, 0.45633, 0.45633, 0.45167, 0.447, 0.41567, 0.405, 0.39433, 0.375, 0.367, 0.359, 0.341, 0.341], [0.392, 0.392, 0.396, 0.39867, 0.39867, 0.402, 0.41066, 0.41066, 0.426, 0.42666, 0.42733, 0.43333, 0.43733, 0.44133, 0.44533, 0.444, 0.44266, 0.42733, 0.426, 0.42466, 0.41266, 0.41066, 0.40866, 0.396, 0.394, 0.392, 0.392, 0.392], "MCCCCCCCCCZ"],
[0, -1, [0.46267, 0.46267, 0.45767, 0.45567, 0.45567, 0.445, 0.42133, 0.42133, 0.383, 0.37, 0.37, 0.34767, 0.33533, 0.33533, 0.32367, 0.334, 0.334, 0.36767, 0.37333, 0.37333, 0.39933, 0.40433, 0.40933, 0.43967, 0.44333, 0.447, 0.46333, 0.46267], [0.45433, 0.45433, 0.45167, 0.44733, 0.44733, 0.42966, 0.42433, 0.42433, 0.40933, 0.406, 0.406, 0.39766, 0.399, 0.399, 0.39867, 0.396, 0.396, 0.39933, 0.40233, 0.40233, 0.411, 0.41533, 0.41966, 0.428, 0.43133, 0.43466, 0.44866, 0.45433], "MCCCCCCCCCZ"],
[0, -1, [0.3648, 0.3648, 0.37352, 0.3716, 0.36968, 0.3656, 0.3656, 0.3648], [0.40182, 0.40182, 0.40219, 0.39961, 0.39703, 0.39848, 0.39848, 0.40182], "MCCLZ"],
[0, -1, [0.3528, 0.3528, 0.36151, 0.35959, 0.35767, 0.35359, 0.35359, 0.3528], [0.39956, 0.39956, 0.39994, 0.39736, 0.39477, 0.39623, 0.39623, 0.39956], "MCCLZ"],
[0, -1, [0.33542, 0.33542, 0.34413, 0.34221, 0.34029, 0.33621, 0.33621, 0.33542], [0.39748, 0.39748, 0.39786, 0.39528, 0.39269, 0.39415, 0.39415, 0.39748], "MCCLZ"],
[0, -1, [0.3227, 0.3227, 0.33141, 0.32949, 0.32757, 0.32349, 0.32349, 0.3227], [0.39658, 0.39658, 0.39695, 0.39437, 0.39179, 0.39324, 0.39324, 0.39658], "MCCLZ"],
[0, -1, [0.39236, 0.39236, 0.40396, 0.4014, 0.39884, 0.39341, 0.39341, 0.39236], [0.41414, 0.41414, 0.41465, 0.41121, 0.40777, 0.40971, 0.40971, 0.41414], "MCCLZ"],
[0, -1, [0.40955, 0.40955, 0.41948, 0.41859, 0.41814, 0.4106, 0.4106, 0.40955], [0.42152, 0.42152, 0.42702, 0.41859, 0.41432, 0.41708, 0.41708, 0.42152], "MCCLZ"],
[0, -1, [0.42477, 0.42477, 0.4367, 0.43381, 0.43252, 0.42582, 0.42582, 0.42477], [0.42621, 0.42621, 0.43238, 0.42328, 0.41919, 0.42178, 0.42178, 0.42621], "MCCLZ"],
[0, -1, [0.44063, 0.44063, 0.44789, 0.44967, 0.45034, 0.44168, 0.44168, 0.44063], [0.43425, 0.43425, 0.44242, 0.43132, 0.42709, 0.42982, 0.42982, 0.43425], "MCCLZ"],
[0, -1, [0.37806, 0.37806, 0.38677, 0.38485, 0.38293, 0.37885, 0.37885, 0.37806], [0.40667, 0.40667, 0.40705, 0.40447, 0.40188, 0.40334, 0.40334, 0.40667], "MCCLZ"],
[0xffffff, 0, [0.43739, 0.43739, 0.43618, 0.43618, 0.43618, 0.46532, 0.48006, 0.48006, 0.45322, 0.43739], [0.60899, 0.60899, 0.60789, 0.60967, 0.61146, 0.63356, 0.63587, 0.63587, 0.62465, 0.60899], "MCCCZ"],
[0, -1, [0.44233, 0.44233, 0.479, 0.49434, 0.49434, 0.50967, 0.503, 0.503, 0.49767, 0.49234, 0.49234, 0.49767, 0.479, 0.479, 0.465, 0.47567, 0.47567, 0.481, 0.48167, 0.48234, 0.469, 0.44033, 0.41167, 0.44233, 0.44233], [0.50267, 0.50267, 0.46533, 0.45933, 0.45933, 0.44066, 0.39733, 0.39733, 0.38466, 0.41933, 0.41933, 0.46133, 0.43466, 0.43466, 0.45117, 0.45066, 0.45066, 0.44733, 0.45, 0.45266, 0.47533, 0.49933, 0.52333, 0.50267, 0.50267], "MCCCCCCCCZ"],
[0xffffff, 0, [0.345, 0.345, 0.34467, 0.34767, 0.35067, 0.50767, 0.56034, 0.56034, 0.485, 0.345], [0.438, 0.438, 0.441, 0.43966, 0.43833, 0.428, 0.388, 0.388, 0.42666, 0.438], "MCCCZ"],
[0xffffff, 0, [0.36233, 0.36233, 0.362, 0.365, 0.368, 0.59567, 0.635, 0.635, 0.59833, 0.36233], [0.44533, 0.44533, 0.44833, 0.447, 0.44566, 0.44733, 0.39333, 0.39333, 0.43533, 0.44533], "MCCCZ"],
[0xffffff, 0, [0.38167, 0.38167, 0.38133, 0.38433, 0.38733, 0.67634, 0.71567, 0.71567, 0.69967, 0.38167], [0.452, 0.452, 0.455, 0.45367, 0.45233, 0.474, 0.42, 0.42, 0.46266, 0.452], "MCCCZ"],
[0xffffff, 0, [0.39833, 0.39833, 0.398, 0.401, 0.404, 0.60767, 0.647, 0.647, 0.62467, 0.39833], [0.46, 0.46, 0.463, 0.46167, 0.46033, 0.52467, 0.47066, 0.47066, 0.51167, 0.46], "MCCCZ"],
[0xffffff, 0, [0.30167, 0.30167, 0.30133, 0.30433, 0.30733, 0.337, 0.34033, 0.34033, 0.32833, 0.30167], [0.422, 0.422, 0.425, 0.42366, 0.42233, 0.41866, 0.354, 0.354, 0.424, 0.422], "MCCCZ"],
[0xffffff, 0, [0.287, 0.287, 0.28667, 0.28967, 0.29267, 0.31633, 0.31167, 0.31167, 0.31367, 0.287], [0.41933, 0.41933, 0.42233, 0.421, 0.41966, 0.42733, 0.36266, 0.36266, 0.42133, 0.41933], "MCCCZ"],
[0xffffff, 0, [0.27367, 0.27367, 0.27333, 0.27633, 0.27933, 0.305, 0.28433, 0.28433, 0.30033, 0.27367], [0.41866, 0.41866, 0.42166, 0.42033, 0.419, 0.418, 0.38066, 0.38066, 0.42066, 0.41866], "MCCCZ"],
[0xffffff, 0, [0.35254, 0.35254, 0.3518, 0.35438, 0.38116, 0.43524, 0.52804, 0.52804, 0.4642, 0.35254], [0.49256, 0.49256, 0.48923, 0.49126, 0.5123, 0.61047, 0.61986, 0.61986, 0.64004, 0.49256], "MCCCZ"],
[0xffffff, 0, [0.37121, 0.37121, 0.36894, 0.37205, 0.37516, 0.56257, 0.62804, 0.62804, 0.58286, 0.37121], [0.49556, 0.49556, 0.49755, 0.49859, 0.49963, 0.62047, 0.60719, 0.60719, 0.61971, 0.49556], "MCCCZ"],
[0xffffff, 0, [0.33648, 0.33648, 0.33563, 0.33848, 0.35344, 0.35946, 0.43209, 0.43209, 0.37807, 0.33648], [0.48539, 0.48539, 0.48272, 0.48435, 0.49288, 0.58824, 0.59033, 0.59033, 0.61485, 0.48539], "MCCCZ"],
[0xffffff, 0, [0.30327, 0.30327, 0.30148, 0.30473, 0.32181, 0.35748, 0.43155, 0.43155, 0.37827, 0.30327], [0.46701, 0.46701, 0.46487, 0.46529, 0.46751, 0.5363, 0.52783, 0.52783, 0.55174, 0.46701], "MCCCZ"],
[0xffffff, 0, [0.322, 0.322, 0.32065, 0.32376, 0.3401, 0.3619, 0.43622, 0.43622, 0.37934, 0.322], [0.47722, 0.47722, 0.47477, 0.47582, 0.48127, 0.55562, 0.56154, 0.56154, 0.57478, 0.47722], "MCCCZ"],
[0xffffff, 0, [0.29154, 0.29154, 0.29008, 0.29272, 0.30655, 0.33545, 0.39544, 0.39544, 0.35228, 0.29154], [0.45848, 0.45848, 0.45674, 0.45709, 0.45889, 0.5146, 0.50774, 0.50774, 0.52711, 0.45848], "MCCCZ"],
[0xffffff, 0, [0.27887, 0.27887, 0.2772, 0.27985, 0.2868, 0.33786, 0.36864, 0.36864, 0.34861, 0.27887], [0.44863, 0.44863, 0.44689, 0.44709, 0.4476, 0.50273, 0.4769, 0.4769, 0.5081, 0.44863], "MCCCZ"],
[0xffffff, 0, [0.39476, 0.39476, 0.39276, 0.39598, 0.3992, 0.60037, 0.66364, 0.66364, 0.6204, 0.39476], [0.50184, 0.50184, 0.50409, 0.50474, 0.50538, 0.60156, 0.58012, 0.58012, 0.59824, 0.50184], "MCCCZ"],
[0xffffff, 0, [0.339, 0.339, 0.33867, 0.34167, 0.34467, 0.37033, 0.34967, 0.34967, 0.36567, 0.339], [0.39466, 0.39466, 0.39766, 0.39633, 0.395, 0.394, 0.35666, 0.35666, 0.39666, 0.39466], "MCCCZ"],
[0xffffff, 0, [0.35633, 0.35633, 0.356, 0.359, 0.362, 0.39167, 0.395, 0.395, 0.383, 0.35633], [0.39733, 0.39733, 0.40033, 0.399, 0.39766, 0.394, 0.32933, 0.32933, 0.39933, 0.39733], "MCCCZ"],
[0xffffff, 0, [0.36767, 0.36767, 0.36733, 0.37033, 0.37333, 0.41433, 0.45367, 0.45367, 0.39433, 0.36767], [0.4, 0.4, 0.403, 0.40166, 0.40033, 0.39466, 0.34066, 0.34066, 0.402, 0.4], "MCCCZ"],
[0xffffff, 0, [0.38037, 0.38037, 0.37965, 0.3828, 0.38595, 0.42, 0.4835, 0.4835, 0.40654, 0.38037], [0.40338, 0.40338, 0.40631, 0.40539, 0.40446, 0.40763, 0.35067, 0.35067, 0.40891, 0.40338], "MCCCZ"],
[0xffffff, 0, [0.39771, 0.39771, 0.39698, 0.40013, 0.40328, 0.46134, 0.5475, 0.5475, 0.42387, 0.39771], [0.41071, 0.41071, 0.41364, 0.41272, 0.4118, 0.4043, 0.346, 0.346, 0.41624, 0.41071], "MCCCZ"],
[0xffffff, 0, [0.33033, 0.33033, 0.33, 0.333, 0.336, 0.425, 0.47567, 0.47567, 0.4175, 0.33033], [0.43, 0.43, 0.433, 0.43166, 0.43033, 0.426, 0.38133, 0.38133, 0.42262, 0.43], "MCCCZ"],
[0xffffff, 0, [0.31367, 0.31367, 0.31333, 0.31633, 0.31933, 0.36033, 0.39967, 0.39967, 0.34033, 0.31367], [0.42333, 0.42333, 0.42633, 0.425, 0.42366, 0.418, 0.364, 0.364, 0.42533, 0.42333], "MCCCZ"],
[0xffffff, 0, [0.41584, 0.41584, 0.4149, 0.41811, 0.42132, 0.47977, 0.56997, 0.56997, 0.44053, 0.41584], [0.4184, 0.4184, 0.42126, 0.42058, 0.41989, 0.41666, 0.36484, 0.36484, 0.42216, 0.4184], "MCCCZ"],
[0xffffff, 0, [0.42984, 0.42984, 0.4289, 0.43211, 0.43532, 0.49377, 0.58397, 0.58397, 0.45553, 0.42984], [0.4224, 0.4224, 0.42526, 0.42458, 0.42389, 0.42066, 0.36884, 0.36884, 0.42683, 0.4224], "MCCCZ"],
[0xffffff, 0, [0.44584, 0.44584, 0.4449, 0.44811, 0.45132, 0.5231, 0.67264, 0.67264, 0.47153, 0.44584], [0.43073, 0.43073, 0.4336, 0.43291, 0.43222, 0.42499, 0.36784, 0.36784, 0.43516, 0.43073], "MCCCZ"],
[0, -1, [0.26033, 0.26033, 0.269, 0.267, 0.265, 0.261, 0.261, 0.26033], [0.41933, 0.41933, 0.42, 0.41733, 0.41466, 0.416, 0.416, 0.41933], "MCCLZ"],
[0, -1, [0.31167, 0.31167, 0.32033, 0.31833, 0.31633, 0.31233, 0.31233, 0.31167], [0.396, 0.396, 0.39666, 0.394, 0.39133, 0.39266, 0.39266, 0.396], "MCCLZ"],
[0, -1, [0.297, 0.297, 0.30567, 0.30367, 0.30167, 0.29767, 0.29767, 0.297], [0.39666, 0.39666, 0.39733, 0.39466, 0.392, 0.39333, 0.39333, 0.39666], "MCCLZ"],
[0, -1, [0.13805, 0.13805, 0.14646, 0.1437, 0.14094, 0.13759, 0.13759, 0.13805], [0.41636, 0.41636, 0.41416, 0.41229, 0.41043, 0.413, 0.413, 0.41636], "MCCLZ"],
[0, -1, [0.14139, 0.14139, 0.14979, 0.14703, 0.14427, 0.14092, 0.14092, 0.14139], [0.43236, 0.43236, 0.43016, 0.42829, 0.42643, 0.429, 0.429, 0.43236], "MCCLZ"],
[0, -1, [0.12938, 0.12938, 0.13779, 0.13503, 0.13227, 0.12892, 0.12892, 0.12938], [0.4397, 0.4397, 0.43749, 0.43563, 0.43376, 0.43633, 0.43633, 0.4397], "MCCLZ"],
[0xcccccc, -1, [0.26233, 0.26233, 0.25967, 0.253, 0.24967, 0.231, 0.22167, 0.22167, 0.24233, 0.26233], [0.31467, 0.31467, 0.31467, 0.31133, 0.31133, 0.30533, 0.28866, 0.28866, 0.30467, 0.31467], "MCCCZ"],
[0xcccccc, -1, [0.40853, 0.40876, 0.40885, 0.40934, 0.41044, 0.41249, 0.41228, 0.41087, 0.40941, 0.40252, 0.40146, 0.39906, 0.39893, 0.39872, 0.39853, 0.39904, 0.39954, 0.40314, 0.40467, 0.40623, 0.40695, 0.40853], [0.16026, 0.15982, 0.15913, 0.1591, 0.15903, 0.15853, 0.15959, 0.16677, 0.17499, 0.178, 0.17847, 0.17778, 0.17631, 0.17377, 0.17152, 0.16908, 0.16671, 0.16669, 0.169, 0.16621, 0.16314, 0.16026], "MCCCCCCCZ"],
[0xcccccc, -1, [0.39006, 0.39131, 0.3911, 0.39346, 0.39469, 0.39777, 0.39708, 0.39574, 0.39509, 0.39278, 0.39244, 0.39284, 0.39258, 0.39159, 0.38968, 0.38733, 0.38548, 0.38739, 0.38992, 0.39015, 0.38988, 0.39006], [0.15236, 0.15001, 0.14691, 0.14597, 0.14548, 0.14711, 0.1489, 0.15233, 0.15607, 0.15908, 0.15952, 0.16041, 0.16095, 0.16299, 0.16421, 0.16367, 0.15999, 0.15645, 0.1536, 0.15334, 0.15272, 0.15236], "MCCCCCCCZ"],
[0xcccccc, -1, [0.32406, 0.32388, 0.32382, 0.32409, 0.32498, 0.3263, 0.32574, 0.32516, 0.3233, 0.32221, 0.32032, 0.32024, 0.31909, 0.31876, 0.31885, 0.31811, 0.31732, 0.31659, 0.31675, 0.31684, 0.31653, 0.3169, 0.31793, 0.32302, 0.32313, 0.32323, 0.32445, 0.32406], [0.16101, 0.16164, 0.16242, 0.16295, 0.16467, 0.16654, 0.16824, 0.17, 0.1697, 0.16875, 0.1671, 0.16405, 0.16179, 0.16115, 0.16019, 0.15954, 0.15884, 0.1563, 0.15534, 0.15482, 0.13831, 0.13875, 0.13997, 0.15619, 0.15768, 0.1589, 0.15967, 0.16101], "MCCCCCCCCCZ"],
[0xcccccc, -1, [0.28133, 0.28572, 0.29037, 0.28963, 0.28944, 0.28648, 0.28617, 0.28483, 0.28144, 0.27716, 0.2735, 0.27039, 0.27, 0.27616, 0.27992, 0.28133], [0.17234, 0.1765, 0.18144, 0.18762, 0.18925, 0.18837, 0.18696, 0.18092, 0.17648, 0.1725, 0.16909, 0.15851, 0.15766, 0.16642, 0.171, 0.17234], "MCCCCCZ"],
[0xcccccc, -1, [0.25871, 0.25958, 0.25908, 0.25944, 0.26102, 0.26319, 0.26322, 0.26323, 0.2626, 0.26204, 0.26158, 0.26101, 0.26083, 0.25744, 0.25511, 0.25271, 0.2524, 0.25049, 0.25102, 0.25142, 0.25428, 0.25463, 0.25674, 0.25676, 0.25871], [0.18228, 0.18289, 0.18371, 0.18426, 0.18669, 0.18877, 0.19167, 0.19213, 0.19264, 0.19227, 0.19196, 0.19175, 0.19153, 0.18745, 0.183, 0.17831, 0.17772, 0.17023, 0.17004, 0.1699, 0.17687, 0.17706, 0.1782, 0.1809, 0.18228], "MCCCCCCCCZ"],
[0xcccccc, -1, [0.2866, 0.28734, 0.28996, 0.28977, 0.28958, 0.29036, 0.28884, 0.28674, 0.28099, 0.28054, 0.2805, 0.28546, 0.2866], [0.1497, 0.15115, 0.15315, 0.15464, 0.15618, 0.15859, 0.15744, 0.15586, 0.15361, 0.14393, 0.14298, 0.14747, 0.1497], "MCCCCZ"],
[0xcccccc, -1, [0.31067, 0.31133, 0.31251, 0.31328, 0.31436, 0.31538, 0.31586, 0.31748, 0.32043, 0.32067, 0.31818, 0.31705, 0.316, 0.3138, 0.31213, 0.30996, 0.30985, 0.30949, 0.30937, 0.30741, 0.30627, 0.30461, 0.30432, 0.30365, 0.3034, 0.30232, 0.3007, 0.30023, 0.29838, 0.29314, 0.29, 0.29063, 0.29752, 0.29833, 0.29973, 0.29992, 0.30205, 0.30213, 0.30244, 0.30267, 0.30299, 0.30335, 0.304, 0.304, 0.30378, 0.30411, 0.30617, 0.30603, 0.30733, 0.3081, 0.30986, 0.31067], [0.17833, 0.17944, 0.17866, 0.17909, 0.17972, 0.18064, 0.18173, 0.18531, 0.18834, 0.19233, 0.19467, 0.19127, 0.18966, 0.19241, 0.18929, 0.18842, 0.18837, 0.18895, 0.18891, 0.18817, 0.18637, 0.18507, 0.18485, 0.18515, 0.18492, 0.18392, 0.18337, 0.18224, 0.17773, 0.17424, 0.16167, 0.16015, 0.17274, 0.1739, 0.17589, 0.17113, 0.17224, 0.17228, 0.17189, 0.17167, 0.17213, 0.17251, 0.17233, 0.173, 0.17393, 0.17419, 0.17582, 0.1776, 0.17966, 0.17835, 0.17955, 0.17833], "MCCCCCCCCCCCCCCCCCZ"],
[0xcccccc, -1, [0.41033, 0.41033, 0.42267, 0.41533, 0.41533, 0.43433, 0.42667, 0.42667, 0.426, 0.41933, 0.41933, 0.41267, 0.41033], [0.08766, 0.08766, 0.12167, 0.14033, 0.14033, 0.10433, 0.08566, 0.08566, 0.103, 0.11133, 0.11133, 0.09, 0.08766], "MCCCCZ"],
[0xcccccc, -1, [0.38567, 0.38567, 0.39467, 0.38133, 0.38133, 0.38, 0.36867, 0.36867, 0.39233, 0.38567], [0.092, 0.092, 0.10667, 0.13733, 0.13733, 0.10333, 0.085, 0.085, 0.11867, 0.092], "MCCCZ"],
[0xcccccc, -1, [0.369, 0.369, 0.36867, 0.36933, 0.36933, 0.363, 0.346, 0.346, 0.37, 0.369], [0.09533, 0.09533, 0.12867, 0.13367, 0.13367, 0.106, 0.09, 0.09, 0.11, 0.09533], "MCCCZ"],
[0xcccccc, -1, [0.353, 0.353, 0.363, 0.34633, 0.34633, 0.357, 0.349, 0.349, 0.35333, 0.353], [0.14867, 0.14867, 0.126, 0.09533, 0.09533, 0.11566, 0.12733, 0.12733, 0.133, 0.14867], "MCCCZ"],
[0xcccccc, -1, [0.321, 0.321, 0.31933, 0.32233, 0.32233, 0.32267, 0.322, 0.322, 0.32867, 0.329, 0.329, 0.33133, 0.336, 0.336, 0.342, 0.34167, 0.34167, 0.325, 0.321], [0.096, 0.096, 0.122, 0.12566, 0.12566, 0.13633, 0.138, 0.138, 0.14833, 0.136, 0.136, 0.123, 0.11533, 0.11533, 0.10633, 0.09566, 0.09566, 0.146, 0.096], "MCCCCCCZ"],
[0xcccccc, -1, [0.315, 0.315, 0.304, 0.301, 0.301, 0.29867, 0.305, 0.305, 0.312, 0.315], [0.142, 0.142, 0.124, 0.09233, 0.09233, 0.10266, 0.12667, 0.12667, 0.15233, 0.142], "MCCCZ"],
[0xcccccc, -1, [0.27867, 0.27867, 0.287, 0.28933, 0.28933, 0.29533, 0.28467, 0.28467, 0.285, 0.27133, 0.27133, 0.27933, 0.27867], [0.109, 0.109, 0.118, 0.12633, 0.12633, 0.15266, 0.13833, 0.13833, 0.125, 0.11266, 0.11266, 0.11667, 0.109], "MCCCCZ"],
[0xcccccc, -1, [0.269, 0.269, 0.27467, 0.276, 0.276, 0.279, 0.27433, 0.27433, 0.25967, 0.253, 0.253, 0.26633, 0.269], [0.11733, 0.11733, 0.14633, 0.14766, 0.14766, 0.15333, 0.148, 0.148, 0.116, 0.105, 0.105, 0.12033, 0.11733], "MCCCCZ"],
[0xcccccc, -1, [0.25933, 0.25933, 0.27867, 0.24233, 0.24233, 0.26067, 0.25933], [0.145, 0.145, 0.18233, 0.13933, 0.13933, 0.15566, 0.145], "MCCZ"],
[0xcccccc, -1, [0.23367, 0.23367, 0.24167, 0.246, 0.246, 0.25967, 0.24867, 0.24867, 0.23867, 0.23967, 0.23967, 0.23867, 0.23367], [0.16933, 0.16933, 0.20066, 0.20033, 0.20033, 0.21533, 0.19766, 0.19766, 0.18167, 0.16533, 0.16533, 0.18133, 0.16933], "MCCCCZ"],
[0xcccccc, -1, [0.78417, 0.78417, 0.76417, 0.76, 0.76, 0.7825, 0.7825, 0.7825, 0.78667, 0.78417], [0.18833, 0.18833, 0.205, 0.21083, 0.21083, 0.18, 0.16833, 0.16833, 0.18083, 0.18833], "MCCCZ"],
[0xcccccc, -1, [0.79333, 0.79333, 0.75833, 0.7525, 0.7525, 0.79667, 0.79667, 0.79667, 0.7975, 0.79333], [0.225, 0.225, 0.25, 0.2625, 0.2625, 0.21333, 0.20583, 0.20583, 0.22083, 0.225], "MCCCZ"],
[0xcccccc, -1, [0.82167, 0.82167, 0.80167, 0.79917, 0.79917, 0.81667, 0.82083, 0.82083, 0.81833, 0.82167], [0.48167, 0.48167, 0.495, 0.49167, 0.49167, 0.48083, 0.46667, 0.46667, 0.48167, 0.48167], "MCCCZ"],
[0xcccccc, -1, [0.83583, 0.80667, 0.80667, 0.83833, 0.83917, 0.83583], [0.3475, 0.3675, 0.3675, 0.33917, 0.3325, 0.3475], "MLCLZ"],
[-1, 0, [0.18458, 0.22125], [0.385, 0.37708], "ML"],
[-1, 0, [0.26833, 0.26833, 0.2675, 0.2525], [0.115, 0.115, 0.12083, 0.10333], "MC"],
[-1, 0, [0.2775, 0.2775, 0.28083, 0.27], [0.10667, 0.10667, 0.1175, 0.11], "MC"],
[-1, 0, [0.3675, 0.3675, 0.37, 0.35083], [0.0925, 0.0925, 0.11083, 0.08917], "MC"] ]
function getcolorind(rgbmask)
r = ((rgbmask >> 16) & 0xff) / 255
g = ((rgbmask >> 8) & 0xff) / 255
b = ((rgbmask >> 0) & 0xff) / 255
Int(inqcolorfromrgb(r, g, b))
end
setviewport(0, 1, 0, 1)
setborderwidth(0.5)
for (fill, stroke, x, y, codes) in paths
if fill != -1
setfillcolorind(getcolorind(fill))
op = 'f'
end
if stroke != -1
setbordercolorind(getcolorind(stroke))
op = 'S'
end
if fill != -1 && stroke != -1
op = 'F'
end
path(x, y, codes * op)
end
updatews()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 422 | using GR
using LaTeXStrings
setscientificformat(3)
R, r = 2, 1/2
θ = φ = LinRange(0, 2π, 200)
f(θ, φ) = ((R + r*cos(θ)) * cos(φ), (R + r*cos(θ)) * sin(φ), r * sin(θ))
x, y, z = [[v[i] for v in f.(θ, φ')] for i in 1:3]
surface(x, y, z, xlabel=L"x(\theta,\varphi) = R + r \cos \theta \cos \varphi", ylabel=L"y(\theta,\varphi) = R + r \cos \theta \sin \varphi", zlabel=L"z(\theta,\varphi) = r \sin \theta", title="Torus")
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1508 | ENV["GKS_DOUBLE_BUF"] = "True"
using GR
using BenchmarkTools
const GDP_DRAW_TRIANGLES = 4
function points_from_image(img, npts)
w, h = size(img)
xpts = Float64[]
ypts = Float64[]
cols = Int32[]
for i in 1:npts
x = rand() * w
y = rand() * h
c = img[Int64(floor(x)) + 1, Int64(floor(h-y)) + 1] & 0xffffff
r = ( c & 0xff) / 255.0
g = ((c >> 8 ) & 0xff) / 255.0
b = ((c >> 16) & 0xff) / 255.0
if 0.2989 * r + 0.5870 * g + 0.1140 * b > 0.8
if rand() < 0.1
push!(xpts, x)
push!(ypts, y)
push!(cols, 0xc0c0c0)
end
continue
end
push!(xpts, x)
push!(ypts, y)
push!(cols, c)
end
xpts, ypts, cols
end
w, h, img = readimage("julia_logo.png")
x, y, cols = points_from_image(img, 100_000)
setwsviewport(0, 0.24, 0, 0.16)
setwswindow(0, 1, 0, 2/3)
setviewport(0, 1, 0, 2/3)
setwindow(0, w, 0, h)
setmarkersize(2/3)
setborderwidth(0.2)
setmarkertype(GR.MARKERTYPE_SOLID_CIRCLE)
settransparency(0.5)
polymarker(x, y)
n, tri = delaunay(x, y)
@show n
tri = convert(Matrix{Int32}, tri)
color = zeros(Int32, n)
for i in 1:n
color[i] = cols[tri[i,1]]
end
attributes = vec(hcat(tri, color)')
setlinewidth(0.4)
# Draw a vector of triangles for given vertices x and y using point indices and color triplets
# x: x coordinates
# y: y coordinates
# attributes: indices of the triangle points (i₁₁, i₁₂, i₁₃, rrggbb₁, i₂₁, i₂₂, i₂₃, rrggbb₂, ...)
@time gdp(x, y, GDP_DRAW_TRIANGLES, attributes)
updatews()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1272 | download("https://gr-framework.org/downloads/gr-fonts.tgz", "fonts.tgz")
run(`tar xzf fonts.tgz`)
rm("fonts.tgz")
ENV["GKS_FONT_DIRS"] = joinpath(pwd(), "fonts", "urw-base35")
const fonts = ("Times Roman", "Times Italic", "Times Bold", "Times Bold Italic", "Helvetica", "Helvetica Oblique", "Helvetica Bold", "Helvetica Bold Oblique", "Courier", "Courier Oblique", "Courier Bold", "Courier Bold Oblique", "Bookman Light", "Bookman Light Italic", "Bookman Demi", "Bookman Demi Italic", "New Century Schoolbook Roman", "New Century Schoolbook Italic", "New Century Schoolbook Bold", "New Century Schoolbook Bold Italic", "Avantgarde Book", "Avantgarde Book Oblique", "Avantgarde Demi", "Avantgarde Demi Oblique", "Palatino Roman", "Palatino Italic", "Palatino Bold", "Palatino Bold Italic", "Zapf Chancery Medium Italica", "Zapf Dingbats")
using GR
selntran(0)
setcharheight(0.018)
settextalign(GR.TEXT_HALIGN_CENTER, GR.TEXT_VALIGN_HALF)
y = 0.95
for fontname in fonts
global y
font = loadfont(fontname)
settextfontprec(font, GR.TEXT_PRECISION_OUTLINE)
text(0.5, y, "The quick brown fox jumps over the lazy dog")
tbx, tby = inqtext(0.5, y, "The quick brown fox jumps over the lazy dog")
fillrect(tbx[1], tbx[2], tby[1], tby[3])
y -= 0.03
end
updatews()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 843 | ENV["GKS_ENCODING"] = "utf8"
import GR
GR.selntran(0)
GR.setcharheight(0.024)
function main()
GR.settextfontprec(2, 0)
y = 0
for i in 0:1
GR.text(0.05, 0.85-y, " !\"#\$\$%&'()*+,-./")
GR.text(0.05, 0.80-y, "0123456789:;<=>?")
GR.text(0.05, 0.75-y, "@ABCDEFGHIJKLMNO")
GR.text(0.05, 0.70-y, "PQRSTUVWXYZ[\\]^_")
GR.text(0.05, 0.65-y, "`abcdefghijklmno")
GR.text(0.05, 0.60-y, "pqrstuvwxyz{|}~ ")
GR.text(0.5, 0.85-y, " ¡¢£¤¥¦§¨©ª«¬®¯")
GR.text(0.5, 0.80-y, "°±²³´µ¶·¸¹º»¼½¾¿")
GR.text(0.5, 0.75-y, "ÀÁÂÃÄÅÆÇÈÉÊËÌÍÎÏ")
GR.text(0.5, 0.70-y, "ÐÑÒÓÔÕÖרÙÚÛÜÝÞß")
GR.text(0.5, 0.65-y, "àáâãäåæçèéêëìíîï")
GR.text(0.5, 0.60-y, "ðñòóôõö÷øùúûüýþÿ")
GR.settextfontprec(233, 3)
y = 0.4
end
GR.updatews()
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1297 | using GR
include("noise.jl")
const n = 100000
const radius = 200
const width, height = 500, 500
const length = 50
const scale = 0.005
function main()
T = rand(n) * 2pi
R = sqrt.(rand(n))
X = R .* cos.(T)
Y = R .* sin.(T)
intensity = (1.001 .- sqrt.(X.^2 .+ Y.^2)) .^ 0.75
X = X .* radius .+ div(width, 2)
Y = Y .* radius .+ div(height, 2)
w, h, I = readimage("julia-logo.png")
P = zeros(UInt32, width, height)
settextfontprec(128, 0)
settextalign(2, 3)
setcharheight(0.036)
for time in 0:0.004:1
P .= 0xffffffff
cos_t = 1.5 * cos(2pi * time)
sin_t = 1.5 * sin(2pi * time)
for i in 1:n
x, y = X[i], Y[i]
dx = noise4(scale * x, scale * y, cos_t, sin_t, 2)
dx *= intensity[i] * length
dy = noise4(100 + scale * x, 200 + scale * y, cos_t, sin_t, 2)
dy *= intensity[i] * length
P[round(Int, x + dx), round(Int, y + dy)] = I[round(Int, x), round(Int, y)]
end
clearws()
setviewport(0, 1, 0, 1)
drawimage(0, 1, 0, 1, width, height, P)
textext(0.5, -0.2 + 1.5*time, "Julia Noise\\n\\nMade with GR.jl\\n\\nOriginal idea by Necessary Disorder")
updatews()
end
end
main()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 8212 | #=
# Perlin noise ported from: https://github.com/caseman/noise
# with the license:
Copyright (c) 2008 Casey Duncan
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
=#
const F2 = 0.5 * (sqrt(3.0) - 1.0)
const G2 = (3.0 - sqrt(3.0)) / 6.0
const F4 = (sqrt(5.0) - 1.0) / 4.0
const G4 = (5.0 - sqrt(5.0)) / 20.0
const SIMPLEX = [
(0, 1, 2, 3), (0, 1, 3, 2), (0, 0, 0, 0), (0, 2, 3, 1), (0, 0, 0, 0),
(0, 0, 0, 0), (0, 0, 0, 0), (1, 2, 3, 0), (0, 2, 1, 3), (0, 0, 0, 0),
(0, 3, 1, 2), (0, 3, 2, 1), (0, 0, 0, 0), (0, 0, 0, 0), (0, 0, 0, 0),
(1, 3, 2, 0), (0, 0, 0, 0), (0, 0, 0, 0), (0, 0, 0, 0), (0, 0, 0, 0),
(0, 0, 0, 0), (0, 0, 0, 0), (0, 0, 0, 0), (0, 0, 0, 0), (1, 2, 0, 3),
(0, 0, 0, 0), (1, 3, 0, 2), (0, 0, 0, 0), (0, 0, 0, 0), (0, 0, 0, 0),
(2, 3, 0, 1), (2, 3, 1, 0), (1, 0, 2, 3), (1, 0, 3, 2), (0, 0, 0, 0),
(0, 0, 0, 0), (0, 0, 0, 0), (2, 0, 3, 1), (0, 0, 0, 0), (2, 1, 3, 0),
(0, 0, 0, 0), (0, 0, 0, 0), (0, 0, 0, 0), (0, 0, 0, 0), (0, 0, 0, 0),
(0, 0, 0, 0), (0, 0, 0, 0), (0, 0, 0, 0), (2, 0, 1, 3), (0, 0, 0, 0),
(0, 0, 0, 0), (0, 0, 0, 0), (3, 0, 1, 2), (3, 0, 2, 1), (0, 0, 0, 0),
(3, 1, 2, 0), (2, 1, 0, 3), (0, 0, 0, 0), (0, 0, 0, 0), (0, 0, 0, 0),
(3, 1, 0, 2), (0, 0, 0, 0), (3, 2, 0, 1), (3, 2, 1, 0)
]
const GRAD4 = NTuple{4, Float32}[
(0, 1, 1, 1), (0, 1, 1, -1), (0, 1, -1, 1), (0, 1, -1, -1),
(0, -1, 1, 1), (0, -1, 1, -1), (0, -1, -1, 1), (0, -1, -1, -1),
(1, 0, 1, 1), (1, 0, 1, -1), (1, 0, -1, 1), (1, 0, -1, -1),
(-1, 0, 1, 1), (-1, 0, 1, -1), (-1, 0, -1, 1), (-1, 0, -1, -1),
(1, 1, 0, 1), (1, 1, 0, -1), (1, -1, 0, 1), (1, -1, 0, -1),
(-1, 1, 0, 1), (-1, 1, 0, -1), (-1, -1, 0, 1), (-1, -1, 0, -1),
(1, 1, 1, 0), (1, 1, -1, 0), (1, -1, 1, 0), (1, -1, -1, 0),
(-1, 1, 1, 0), (-1, 1, -1, 0), (-1, -1, 1, 0), (-1, -1, -1, 0)
]
const PERM = [
151, 160, 137, 91, 90, 15, 131, 13, 201, 95, 96, 53, 194, 233, 7, 225, 140,
36, 103, 30, 69, 142, 8, 99, 37, 240, 21, 10, 23, 190, 6, 148, 247, 120,
234, 75, 0, 26, 197, 62, 94, 252, 219, 203, 117, 35, 11, 32, 57, 177, 33,
88, 237, 149, 56, 87, 174, 20, 125, 136, 171, 168, 68, 175, 74, 165, 71,
134, 139, 48, 27, 166, 77, 146, 158, 231, 83, 111, 229, 122, 60, 211, 133,
230, 220, 105, 92, 41, 55, 46, 245, 40, 244, 102, 143, 54, 65, 25, 63, 161,
1, 216, 80, 73, 209, 76, 132, 187, 208, 89, 18, 169, 200, 196, 135, 130,
116, 188, 159, 86, 164, 100, 109, 198, 173, 186, 3, 64, 52, 217, 226, 250,
124, 123, 5, 202, 38, 147, 118, 126, 255, 82, 85, 212, 207, 206, 59, 227,
47, 16, 58, 17, 182, 189, 28, 42, 223, 183, 170, 213, 119, 248, 152, 2, 44,
154, 163, 70, 221, 153, 101, 155, 167, 43, 172, 9, 129, 22, 39, 253, 19, 98,
108, 110, 79, 113, 224, 232, 178, 185, 112, 104, 218, 246, 97, 228, 251, 34,
242, 193, 238, 210, 144, 12, 191, 179, 162, 241, 81, 51, 145, 235, 249, 14,
239, 107, 49, 192, 214, 31, 181, 199, 106, 157, 184, 84, 204, 176, 115, 121,
50, 45, 127, 4, 150, 254, 138, 236, 205, 93, 222, 114, 67, 29, 24, 72, 243,
141, 128, 195, 78, 66, 215, 61, 156, 180, 151, 160, 137, 91, 90, 15, 131,
13, 201, 95, 96, 53, 194, 233, 7, 225, 140, 36, 103, 30, 69, 142, 8, 99, 37,
240, 21, 10, 23, 190, 6, 148, 247, 120, 234, 75, 0, 26, 197, 62, 94, 252,
219, 203, 117, 35, 11, 32, 57, 177, 33, 88, 237, 149, 56, 87, 174, 20, 125,
136, 171, 168, 68, 175, 74, 165, 71, 134, 139, 48, 27, 166, 77, 146, 158,
231, 83, 111, 229, 122, 60, 211, 133, 230, 220, 105, 92, 41, 55, 46, 245,
40, 244, 102, 143, 54, 65, 25, 63, 161, 1, 216, 80, 73, 209, 76, 132, 187,
208, 89, 18, 169, 200, 196, 135, 130, 116, 188, 159, 86, 164, 100, 109, 198,
173, 186, 3, 64, 52, 217, 226, 250, 124, 123, 5, 202, 38, 147, 118, 126,
255, 82, 85, 212, 207, 206, 59, 227, 47, 16, 58, 17, 182, 189, 28, 42, 223,
183, 170, 213, 119, 248, 152, 2, 44, 154, 163, 70, 221, 153, 101, 155, 167,
43, 172, 9, 129, 22, 39, 253, 19, 98, 108, 110, 79, 113, 224, 232, 178, 185,
112, 104, 218, 246, 97, 228, 251, 34, 242, 193, 238, 210, 144, 12, 191, 179,
162, 241, 81, 51, 145, 235, 249, 14, 239, 107, 49, 192, 214, 31, 181, 199,
106, 157, 184, 84, 204, 176, 115, 121, 50, 45, 127, 4, 150, 254, 138, 236,
205, 93, 222, 114, 67, 29, 24, 72, 243, 141, 128, 195, 78, 66, 215, 61, 156,
180
]
dot4(v1, x, y, z, w) = ((v1)[1]*(x) + (v1)[2]*(y) + (v1)[3]*(z) + (v1)[4]*(w))
function noise4(x, y, z, w)
s = (x + y + z + w) * F4
i = floor(x + s)
j = floor(y + s)
k = floor(z + s)
l = floor(w + s)
t = (i + j + k + l) * G4
x0 = x - (i - t)
y0 = y - (j - t)
z0 = z - (k - t)
w0 = w - (l - t)
c = (x0 > y0)*32 + (x0 > z0)*16 + (y0 > z0)*8 + (x0 > w0)*4 + (y0 > w0)*2 + (z0 > w0)
c += 1
i1 = SIMPLEX[c][1] >= 3
j1 = SIMPLEX[c][2] >= 3
k1 = SIMPLEX[c][3] >= 3
l1 = SIMPLEX[c][4] >= 3
i2 = SIMPLEX[c][1] >= 2
j2 = SIMPLEX[c][2] >= 2
k2 = SIMPLEX[c][3] >= 2
l2 = SIMPLEX[c][4] >= 2
i3 = SIMPLEX[c][1] >= 1
j3 = SIMPLEX[c][2] >= 1
k3 = SIMPLEX[c][3] >= 1
l3 = SIMPLEX[c][4] >= 1
x1 = x0 - i1 + G4
y1 = y0 - j1 + G4
z1 = z0 - k1 + G4
w1 = w0 - l1 + G4
x2 = x0 - i2 + 2.0*G4
y2 = y0 - j2 + 2.0*G4
z2 = z0 - k2 + 2.0*G4
w2 = w0 - l2 + 2.0*G4
x3 = x0 - i3 + 3.0*G4
y3 = y0 - j3 + 3.0*G4
z3 = z0 - k3 + 3.0*G4
w3 = w0 - l3 + 3.0*G4
x4 = x0 - 1.0 + 4.0*G4
y4 = y0 - 1.0 + 4.0*G4
z4 = z0 - 1.0 + 4.0*G4
w4 = w0 - 1.0 + 4.0*G4
I = Int(i) & 255
J = Int(j) & 255
K = Int(k) & 255
L = Int(l) & 255
gi0 = PERM[1 + I + PERM[1 + J + PERM[1 + K + PERM[1 + L]]]] & 0x1f
gi1 = PERM[1 + I + i1 + PERM[1 + J + j1 + PERM[1 + K + k1 + PERM[1 + L + l1]]]] & 0x1f
gi2 = PERM[1 + I + i2 + PERM[1 + J + j2 + PERM[1 + K + k2 + PERM[1 + L + l2]]]] & 0x1f
gi3 = PERM[1 + I + i3 + PERM[1 + J + j3 + PERM[1 + K + k3 + PERM[1 + L + l3]]]] & 0x1f
gi4 = PERM[1 + I + 1 + PERM[1 + J + 1 + PERM[1 + K + 1 + PERM[1 + L + 1]]]] & 0x1f
t0 = 0.6 - x0*x0 - y0*y0 - z0*z0 - w0*w0
noise = zeros(5)
if t0 >= 0.0
t0 *= t0
noise[1] = t0 * t0 * dot4(GRAD4[gi0 + 1], x0, y0, z0, w0)
end
t1 = 0.6 - x1*x1 - y1*y1 - z1*z1 - w1*w1
if t1 >= 0.0
t1 *= t1
noise[2]= t1 * t1 * dot4(GRAD4[gi1 + 1], x1, y1, z1, w1)
end
t2 = 0.6 - x2*x2 - y2*y2 - z2*z2 - w2*w2
if t2 >= 0.0
t2 *= t2
noise[3] = t2 * t2 * dot4(GRAD4[gi2 + 1], x2, y2, z2, w2)
end
t3 = 0.6 - x3*x3 - y3*y3 - z3*z3 - w3*w3
if t3 >= 0.0
t3 *= t3
noise[4] = t3 * t3 * dot4(GRAD4[gi3 + 1], x3, y3, z3, w3)
end
t4 = 0.6 - x4*x4 - y4*y4 - z4*z4 - w4*w4
if t4 >= 0.0
t4 *= t4
noise[5] = t4 * t4 * dot4(GRAD4[gi4 + 1], x4, y4, z4, w4)
end
return 27.0 * sum(noise)
end
function noise4(x, y, z, w, octaves, persistence = 0.5, lacunarity = 2)
freq = 1.0
amp = 1.0
max = 1.0
total = noise4(x, y, z, w)
for i = 1:(octaves-1)
freq *= lacunarity
amp *= persistence
max += amp
total += noise4(x * freq, y * freq, z * freq, w * freq) * amp
end
return total / max
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 140741 | """
GR is a universal framework for cross-platform visualization applications.
It offers developers a compact, portable and consistent graphics library
for their programs. Applications range from publication quality 2D graphs
to the representation of complex 3D scenes.
See https://gr-framework.org/julia.html for full documentation.
Basic usage:
```julia
using GR
GR.init() # optional
plot(
[0, 0.2, 0.4, 0.6, 0.8, 1.0],
[0.3, 0.5, 0.4, 0.2, 0.6, 0.7]
)
# GR.show() # Use if in a Jupyter Notebook
```
"""
module GR
@static if isdefined(Base, :Experimental) &&
isdefined(Base.Experimental, Symbol("@optlevel"))
Base.Experimental.@optlevel 1
end
if haskey(ENV, "WAYLAND_DISPLAY") || get(ENV, "XDG_SESSION_TYPE", "") == "wayland"
# loading this automatially on Wayland ensures that applications run natively on Wayland without user intervention
using Qt6Wayland_jll
end
import Base64
import Libdl
export
init,
initgr,
opengks,
closegks,
inqdspsize,
openws,
closews,
activatews,
deactivatews,
clearws,
updatews,
polyline,
polymarker,
text,
inqtext,
textx,
inqtextx,
fillarea,
cellarray,
nonuniformcellarray,
polarcellarray,
nonuniformpolarcellarray,
gdp,
path,
spline,
gridit,
setlinetype,
setlinewidth,
setlinecolorind,
setmarkertype,
setmarkersize,
setmarkercolorind,
settextfontprec,
setcharexpan,
setcharspace,
settextcolorind,
setcharheight,
inqcharheight,
setcharup,
settextpath,
settextalign,
setfillintstyle,
setfillstyle,
setfillcolorind,
setcolorrep,
setscale,
inqscale,
setwindow,
inqwindow,
setviewport,
inqviewport,
selntran,
setclip,
setwswindow,
setwsviewport,
createseg,
copyseg,
redrawseg,
setsegtran,
closeseg,
samplelocator,
emergencyclosegks,
updategks,
setspace,
textext,
inqtextext,
axes2d, # to avoid WARNING: both GR and Base export "axes"
axeslbl,
grid,
grid3d,
verrorbars,
herrorbars,
polyline3d,
polymarker3d,
axes3d,
settitles3d,
titles3d,
surface,
volume,
contour,
hexbin,
setcolormap,
colorbar,
inqcolor,
inqcolorfromrgb,
hsvtorgb,
tick,
validaterange,
adjustlimits,
adjustrange,
beginprint,
beginprintext,
endprint,
ndctowc,
wctondc,
wc3towc,
drawrect,
fillrect,
drawarc,
fillarc,
drawpath,
setarrowstyle,
setarrowsize,
drawarrow,
readimage,
drawimage,
importgraphics,
setshadow,
settransparency,
setcoordxform,
begingraphics,
endgraphics,
getgraphics,
drawgraphics,
startlistener,
mathtex,
inqmathtex,
selectcontext,
destroycontext,
delaunay,
interp2,
trisurface,
tricontour,
# gradient, # deprecated, but still in Base
quiver,
reducepoints,
version,
check_for_updates,
openmeta,
sendmeta,
sendmetaref,
closemeta,
grplot,
shadepoints,
shadelines,
setcolormapfromrgb,
setborderwidth,
setbordercolorind,
setprojectiontype,
setperspectiveprojection,
setorthographicprojection,
settransformationparameters,
setresamplemethod,
setwindow3d,
setspace3d,
text3d,
inqtext3d,
settextencoding,
inqtextencoding,
loadfont,
inqvpsize,
setpicturesizeforvolume,
inqtransformationparameters,
polygonmesh3d,
setscientificformat,
setresizebehaviour,
inqprojectiontype,
setmathfont,
inqmathfont,
setclipregion,
inqclipregion,
# Convenience functions
jlgr,
colormap,
figure,
kvs,
gcf,
hold,
usecolorscheme,
subplot,
plot,
oplot,
stairs,
scatter,
stem,
barplot,
histogram,
polarhistogram,
contourf,
heatmap,
polarheatmap,
wireframe,
plot3,
scatter3,
redraw,
title,
xlabel,
ylabel,
drawgrid,
xticks,
yticks,
zticks,
xticklabels,
yticklabels,
legend,
xlim,
ylim,
savefig,
meshgrid,
peaks,
imshow,
isosurface,
cart2sph,
sph2cart,
polar,
trisurf,
tricont,
shade,
panzoom,
setpanzoom,
libGR3,
gr3,
libGRM,
isinline,
inline,
displayname,
mainloop,
axis,
drawaxis,
drawaxes,
GRAxis,
GRTick,
GRTickLabel
const ENCODING_LATIN1 = 300
const ENCODING_UTF8 = 301
const display_name = Ref("")
const mime_type = Ref("")
const file_path = Ref("")
const figure_count = Ref(-1)
const send_c = Ref(C_NULL)
const recv_c = Ref(C_NULL)
const text_encoding = Ref(ENCODING_UTF8)
const check_env = Ref(true)
isijulia() = isdefined(Main, :IJulia) && Main.IJulia isa Module && isdefined(Main.IJulia, :clear_output)
isatom() = isdefined(Main, :Atom) && Main.Atom isa Module && Main.Atom.isconnected() && (isdefined(Main.Atom, :PlotPaneEnabled) ? Main.Atom.PlotPaneEnabled[] : true)
ispluto() = isdefined(Main, :PlutoRunner) && Main.PlutoRunner isa Module
isvscode() = isdefined(Main, :VSCodeServer) && Main.VSCodeServer isa Module && (isdefined(Main.VSCodeServer, :PLOT_PANE_ENABLED) ? Main.VSCodeServer.PLOT_PANE_ENABLED[] : true)
setraw!(raw) = ccall(:jl_tty_set_mode, Int32, (Ptr{Cvoid}, Int32), stdin.handle, raw)
include("preferences.jl")
# Load function pointer caching mechanism
include("funcptrs.jl")
"""
function set_callback()
callback_c = @cfunction(callback, Cstring, (Cstring, ))
ccall(libGR_ptr(:gr_setcallback),
Nothing,
(Ptr{Cvoid}, ),
callback_c)
end
"""
"""
init(always::Bool = false)
Initialize GR's environmental variables before plotting and ensure that the
binary shared libraries are loaded. Initialization usually only needs to be
done once, but reinitialized may be required when settings change.
The `always` argument is true if initialization should be forced in the
current and subsequent calls. It is `false` by default so that
initialization only is done once.
# Extended Help
Environmental variables which influence `init`:
GRDISPLAY - if "js" or "pluto", javascript support is initialized
GKS_NO_GUI - no initialization is done
GKS_IGNORE_ENCODING - Force use of UTF-8 for font encoding, ignore GKS_ENCODING
Environmental variables set by `init`:
GKS_FONTPATH - path to GR fonts, often the same as GRDIR
GKS_USE_CAIRO_PNG
GKSwstype - Graphics workstation type, see help for `openws`
GKS_QT - Command to start QT backend via gksqt executable
GKS_ENCODING - Sets the text encoding (e.g. Latin1 or UTF-8)
"""
function init(always::Bool = false)
if !libs_loaded[]
load_libs(always)
return
end
if check_env[] || always
haskey(ENV, "GKS_FONTPATH") || get!(ENV, "GKS_FONTPATH", GRPreferences.grdir[])
ENV["GKS_USE_CAIRO_PNG"] = "true"
if "GRDISPLAY" in keys(ENV)
display_name[] = ENV["GRDISPLAY"]
if display_name[] == "js" || display_name[] == "pluto" || display_name[] == "js-server"
send_c[], recv_c[] = js.initjs()
elseif display_name[] == "plot" || display_name[] == "edit"
ENV["GR_PLOT"] = if Sys.iswindows()
"set PATH=$(GRPreferences.libpath[]) & \"$(GRPreferences.grplot[])\" --listen"
else
key = Sys.isapple() ? "DYLD_FALLBACK_LIBRARY_PATH" : "LD_LIBRARY_PATH"
"env $key=$(GRPreferences.libpath[]) $(GRPreferences.grplot[]) --listen"
end
GR.startlistener()
ENV["GKS_WSTYPE"] = "nul"
end
@debug "Found GRDISPLAY in ENV" display_name[]
elseif "GKS_NO_GUI" in keys(ENV)
@debug "Found GKS_NO_GUI in ENV, returning"
return
elseif "GKS_WSTYPE" in keys(ENV)
mime_type[] = ""
@debug "Force user-defined output type" ENV["GKS_WSTYPE"]
elseif isijulia() || ispluto() || isvscode() || isatom()
mime_type[] = "svg"
file_path[] = tempname() * ".svg"
ENV["GKSwstype"] = "svg"
ENV["GKS_FILEPATH"] = file_path[]
@debug "Found an embedded environment" mime_type[] file_path[] ENV["GKSwstype"] ENV["GKS_FILEPATH"]
else
default_wstype = haskey(ENV, "DISPLAY") ? "gksqt" : ""
haskey(ENV, "GKSwstype") || get!(ENV, "GKSwstype", default_wstype)
if !haskey(ENV, "GKS_QT")
ENV["GKS_QT"] = if Sys.iswindows()
"set PATH=$(GRPreferences.libpath[]) & \"$(GRPreferences.gksqt[])\""
else
key = Sys.isapple() ? "DYLD_FALLBACK_LIBRARY_PATH" : "LD_LIBRARY_PATH"
"env $key=$(GRPreferences.libpath[]) $(GRPreferences.gksqt[])"
end
end
@debug "Artifacts setup" ENV["GKSwstype"] ENV["GKS_QT"]
end
if "GKS_IGNORE_ENCODING" in keys(ENV)
text_encoding[] = ENCODING_UTF8
@debug "Found GKS_IGNORE_ENCODING in ENV" text_encoding[]
elseif "GKS_ENCODING" in keys(ENV)
text_encoding[] = if (enc = ENV["GKS_ENCODING"]) == "latin1" || enc == "latin-1"
ENCODING_LATIN1
else
ENCODING_UTF8
end
@debug "Found GKS_ENCODING in ENV" text_encoding[]
else
ENV["GKS_ENCODING"] = "utf8"
@debug "Default GKS_ENCODING" ENV["GKS_ENCODING"]
end
check_env[] = always
end
end
function initgr()
ccall( libGR_ptr(:gr_initgr),
Nothing,
()
)
end
function opengks()
ccall( libGR_ptr(:gr_opengks),
Nothing,
()
)
end
function closegks()
ccall( libGR_ptr(:gr_closegks),
Nothing,
()
)
end
# (Information taken from <https://www.iterm2.com/utilities/imgcat>.)
# tmux requires unrecognized OSC sequences to be wrapped with DCS
# tmux; <sequence> ST, and for all ESCs in <sequence> to be replaced
# with ESC ESC. It only accepts ESC backslash for ST. We use TERM
# instead of TMUX because TERM gets passed through ssh.
function osc_seq()
if startswith(get(ENV, "TERM", ""), "screen")
"\033Ptmux;\033\033]"
else
"\033]"
end
end
function st_seq()
if startswith(get(ENV, "TERM", ""), "screen")
"\a\033\\"
else
"\a"
end
end
function is_dark_mode()
try
setraw!(true)
print(stdin, "\033]11;?\033\\")
resp = read(stdin, 24)
setraw!(false)
bg = String(resp)[10:23]
red, green, blue = parse.(Int, rsplit(bg, '/'), base=16) ./ 0xffff
return 0.3 * red + 0.59 * green + 0.11 * blue < 0.5
catch e
setraw!(false)
return false
end
end
function inqdspsize()
mwidth = Cdouble[0]
mheight = Cdouble[0]
width = Cint[0]
height = Cint[0]
ccall( libGR_ptr(:gr_inqdspsize),
Nothing,
(Ptr{Cdouble}, Ptr{Cdouble}, Ptr{Cint}, Ptr{Cint}),
mwidth, mheight, width, height)
return mwidth[1], mheight[1], width[1], height[1]
end
"""
openws(workstation_id::Int, connection, workstation_type::Int)
Open a graphical workstation.
**Parameters:**
`workstation_id` :
A workstation identifier.
`connection` :
A connection identifier.
`workstation_type` :
The desired workstation type.
Available workstation types:
+-------------+------------------------------------------------------+
| 5|Workstation Independent Segment Storage |
+-------------+------------------------------------------------------+
| 7, 8|Computer Graphics Metafile (CGM binary, clear text) |
+-------------+------------------------------------------------------+
| 41|Windows GDI |
+-------------+------------------------------------------------------+
| 51|Mac Quickdraw |
+-------------+------------------------------------------------------+
| 61 - 64|PostScript (b/w, color) |
+-------------+------------------------------------------------------+
| 101, 102|Portable Document Format (plain, compressed) |
+-------------+------------------------------------------------------+
| 210 - 213|X Windows |
+-------------+------------------------------------------------------+
| 214|Sun Raster file (RF) |
+-------------+------------------------------------------------------+
| 215, 218|Graphics Interchange Format (GIF87, GIF89) |
+-------------+------------------------------------------------------+
| 216|Motif User Interface Language (UIL) |
+-------------+------------------------------------------------------+
| 320|Windows Bitmap (BMP) |
+-------------+------------------------------------------------------+
| 321|JPEG image file |
+-------------+------------------------------------------------------+
| 322|Portable Network Graphics file (PNG) |
+-------------+------------------------------------------------------+
| 323|Tagged Image File Format (TIFF) |
+-------------+------------------------------------------------------+
| 370|Xfig vector graphics file |
+-------------+------------------------------------------------------+
| 371|Gtk |
+-------------+------------------------------------------------------+
| 380|wxWidgets |
+-------------+------------------------------------------------------+
| 381|Qt4 |
+-------------+------------------------------------------------------+
| 382|Scaleable Vector Graphics (SVG) |
+-------------+------------------------------------------------------+
| 390|Windows Metafile |
+-------------+------------------------------------------------------+
| 400|Quartz |
+-------------+------------------------------------------------------+
| 410|Socket driver |
+-------------+------------------------------------------------------+
| 415|0MQ driver |
+-------------+------------------------------------------------------+
| 420|OpenGL |
+-------------+------------------------------------------------------+
| 430|HTML5 Canvas |
+-------------+------------------------------------------------------+
"""
function openws(workstation_id::Int, connection, workstation_type::Int)
ccall( libGR_ptr(:gr_openws),
Nothing,
(Int32, Ptr{Cchar}, Int32),
workstation_id, connection, workstation_type)
end
"""
closews(workstation_id::Int)
Close the specified workstation.
**Parameters:**
`workstation_id` :
A workstation identifier.
"""
function closews(workstation_id::Int)
ccall( libGR_ptr(:gr_closews),
Nothing,
(Int32, ),
workstation_id)
end
"""
activatews(workstation_id::Int)
Activate the specified workstation.
**Parameters:**
`workstation_id` :
A workstation identifier.
"""
function activatews(workstation_id::Int)
ccall( libGR_ptr(:gr_activatews),
Nothing,
(Int32, ),
workstation_id)
end
"""
deactivatews(workstation_id::Int)
Deactivate the specified workstation.
**Parameters:**
`workstation_id` :
A workstation identifier.
"""
function deactivatews(workstation_id::Int)
ccall( libGR_ptr(:gr_deactivatews),
Nothing,
(Int32, ),
workstation_id)
end
function clearws()
ccall( libGR_ptr(:gr_clearws),
Nothing,
()
)
end
function updatews()
ccall( libGR_ptr(:gr_updatews),
Nothing,
()
)
end
"""
polyline(x, y)
Draw a polyline using the current line attributes, starting from the
first data point and ending at the last data point.
**Parameters:**
`x` :
A list containing the X coordinates
`y` :
A list containing the Y coordinates
The values for `x` and `y` are in world coordinates. The attributes that
control the appearance of a polyline are linetype, linewidth and color
index.
"""
function polyline(x, y)
@assert length(x) == length(y)
n = length(x)
ccall( libGR_ptr(:gr_polyline),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}),
n, convert(Vector{Float64}, x), convert(Vector{Float64}, y))
end
"""
polymarker(x, y)
Draw marker symbols centered at the given data points.
**Parameters:**
`x` :
A list containing the X coordinates
`y` :
A list containing the Y coordinates
The values for `x` and `y` are in world coordinates. The attributes that
control the appearance of a polymarker are marker type, marker size
scale factor and color index.
"""
function polymarker(x, y)
@assert length(x) == length(y)
n = length(x)
ccall( libGR_ptr(:gr_polymarker),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}),
n, convert(Vector{Float64}, x), convert(Vector{Float64}, y))
end
function latin1(string)
if text_encoding[] == ENCODING_UTF8
# add null character '\0' for SubString types (see GR.jl SubString issue #336)
if typeof(string) == SubString{String}
return string * "\0"
else
return string
end
end
b = unsafe_wrap(Array{UInt8,1}, pointer(string), sizeof(string))
s = zeros(UInt8, sizeof(string) * 2)
len = 0
mask = 0
for c in b
if mask == -1
mask = 0
continue
end
if c == 0xce || c == 0xcf
len += 1
s[len] = 0x3f
mask = -1
continue
end
if c != 0xc2 && c != 0xc3
len += 1
s[len] = c | mask
end
if c == 0xc3
mask = 0x40
else
mask = 0
end
end
return s[1:len]
end
"""
text(x::Real, y::Real, string)
Draw a text at position `x`, `y` using the current text attributes.
**Parameters:**
`x` :
The X coordinate of starting position of the text string
`y` :
The Y coordinate of starting position of the text string
`string` :
The text to be drawn
The values for `x` and `y` are in normalized device coordinates.
The attributes that control the appearance of text are text font and precision,
character expansion factor, character spacing, text color index, character
height, character up vector, text path and text alignment.
"""
function text(x::Real, y::Real, string)
ccall( libGR_ptr(:gr_text),
Nothing,
(Float64, Float64, Ptr{UInt8}),
x, y, latin1(string))
end
function inqtext(x, y, string)
tbx = Cdouble[0, 0, 0, 0]
tby = Cdouble[0, 0, 0, 0]
ccall( libGR_ptr(:gr_inqtext),
Nothing,
(Float64, Float64, Ptr{UInt8}, Ptr{Cdouble}, Ptr{Cdouble}),
x, y, latin1(string), tbx, tby)
return tbx, tby
end
function textx(x::Real, y::Real, string, opts::UInt32)
ccall( libGR_ptr(:gr_textx),
Nothing,
(Float64, Float64, Ptr{UInt8}, UInt32),
x, y, latin1(string), opts)
end
function inqtextx(x, y, string, opts::UInt32)
tbx = Cdouble[0, 0, 0, 0]
tby = Cdouble[0, 0, 0, 0]
ccall( libGR_ptr(:gr_inqtextx),
Nothing,
(Float64, Float64, Ptr{UInt8}, UInt32, Ptr{Cdouble}, Ptr{Cdouble}),
x, y, latin1(string), opts, tbx, tby)
return tbx, tby
end
"""
fillarea(x, y)
Allows you to specify a polygonal shape of an area to be filled.
**Parameters:**
`x` :
A list containing the X coordinates
`y` :
A list containing the Y coordinates
The attributes that control the appearance of fill areas are fill area interior
style, fill area style index and fill area color index.
"""
function fillarea(x, y)
@assert length(x) == length(y)
n = length(x)
ccall( libGR_ptr(:gr_fillarea),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}),
n, convert(Vector{Float64}, x), convert(Vector{Float64}, y))
end
"""
cellarray(xmin::Real, xmax::Real, ymin::Real, ymax::Real, dimx::Int, dimy::Int, color)
Display rasterlike images in a device-independent manner. The cell array
function partitions a rectangle given by two corner points into DIMX X DIMY
cells, each of them colored individually by the corresponding color index
of the given cell array.
**Parameters:**
`xmin`, `ymin` :
Lower left point of the rectangle
`xmax`, `ymax` :
Upper right point of the rectangle
`dimx`, `dimy` :
X and Y dimension of the color index array
`color` :
Color index array
The values for `xmin`, `xmax`, `ymin` and `ymax` are in world coordinates.
"""
function cellarray(xmin::Real, xmax::Real, ymin::Real, ymax::Real, dimx::Int, dimy::Int, color)
if ndims(color) == 2
color = reshape(color, dimx * dimy)
end
ccall( libGR_ptr(:gr_cellarray),
Nothing,
(Float64, Float64, Float64, Float64, Int32, Int32, Int32, Int32, Int32, Int32, Ptr{Int32}),
xmin, xmax, ymin, ymax, dimx, dimy, 1, 1, dimx, dimy, convert(Vector{Int32}, color))
end
"""
nonuniformcellarray(x, y, dimx::Int, dimy::Int, color)
Display a two dimensional color index array with nonuniform cell sizes.
**Parameters:**
`x`, `y` :
X and Y coordinates of the cell edges
`dimx`, `dimy` :
X and Y dimension of the color index array
`color` :
Color index array
The values for `x` and `y` are in world coordinates. `x` must contain `dimx` + 1 elements
and `y` must contain `dimy` + 1 elements. The elements i and i+1 are respectively the edges
of the i-th cell in X and Y direction.
"""
function nonuniformcellarray(x, y, dimx::Int, dimy::Int, color)
@assert dimx <= length(x) <= dimx+1 && dimy <= length(y) <= dimy+1
if ndims(color) == 2
color = reshape(color, dimx * dimy)
end
nx = dimx == length(x) ? -dimx : dimx
ny = dimy == length(y) ? -dimy : dimy
ccall( libGR_ptr(:gr_nonuniformcellarray),
Nothing,
(Ptr{Float64}, Ptr{Float64}, Int32, Int32, Int32, Int32, Int32, Int32, Ptr{Int32}),
convert(Vector{Float64}, x), convert(Vector{Float64}, y), nx, ny, 1, 1, dimx, dimy, convert(Vector{Int32}, color))
end
"""
polarcellarray(xorg::Real, yorg::Real, phimin::Real, phimax::Real, rmin::Real, rmax::Real, imphi::Int, dimr::Int, color)
Display a two dimensional color index array mapped to a disk using polar
coordinates.
**Parameters:**
`xorg` :
X coordinate of the disk center in world coordinates
`yorg` :
Y coordinate of the disk center in world coordinates
`phimin` :
start angle of the disk sector in degrees
`phimax` :
end angle of the disk sector in degrees
`rmin` :
inner radius of the punctured disk in world coordinates
`rmax` :
outer radius of the punctured disk in world coordinates
`dimiphi`, `dimr` :
Phi (X) and iR (Y) dimension of the color index array
`color` :
Color index array
The two dimensional color index array is mapped to the resulting image by
interpreting the X-axis of the array as the angle and the Y-axis as the radius.
The center point of the resulting disk is located at `xorg`, `yorg` and the
radius of the disk is `rmax`.
"""
function polarcellarray(xorg::Real, yorg::Real, phimin::Real, phimax::Real, rmin::Real, rmax::Real,
dimphi::Int, dimr::Int, color)
if ndims(color) == 2
color = reshape(color, dimphi * dimr)
end
ccall( libGR_ptr(:gr_polarcellarray),
Nothing,
(Float64, Float64, Float64, Float64, Float64, Float64, Int32, Int32, Int32, Int32, Int32, Int32, Ptr{Int32}),
xorg, yorg, phimin, phimax, rmin, rmax, dimphi, dimr, 1, 1, dimphi, dimr, convert(Vector{Int32}, color))
end
"""
nonuniformpolarcellarray(x, y, dimx::Int, dimy::Int, color)
Display a two dimensional color index array mapped to a disk using nonuniform
polar coordinates.
**Parameters:**
`x`, `y` :
X and Y coordinates of the cell edges
`dimx`, `dimy` :
X and Y dimension of the color index array
`color` :
Color index array
The two dimensional color index array is mapped to the resulting image by
interpreting the X-axis of the array as the angle and the Y-axis as the radius.
"""
function nonuniformpolarcellarray(x, y, dimx::Int, dimy::Int, color)
@assert dimx <= length(x) <= dimx+1 && dimy <= length(y) <= dimy+1
if ndims(color) == 2
color = reshape(color, dimx * dimy)
end
nx = dimx == length(x) ? -dimx : dimx
ny = dimy == length(y) ? -dimy : dimy
ccall( libGR_ptr(:gr_nonuniformpolarcellarray),
Nothing,
(Float64, Float64, Ptr{Float64}, Ptr{Float64}, Int32, Int32, Int32, Int32, Int32, Int32, Ptr{Int32}),
0, 0, convert(Vector{Float64}, x), convert(Vector{Float64}, y), nx, ny, 1, 1, dimx, dimy, convert(Vector{Int32}, color))
end
"""
gdp(x, y, primid, datrec)
Generates a generalized drawing primitive (GDP) of the type you specify,
using specified points and any additional information contained in a data
record.
**Parameters:**
`x` :
A list containing the X coordinates
`y` :
A list containing the Y coordinates
`primid` :
Primitive identifier
`datrec` :
Primitive data record
"""
function gdp(x, y, primid, datrec)
@assert length(x) == length(y)
n = length(x)
ldr = length(datrec)
ccall( libGR_ptr(:gr_gdp),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Int32, Int32, Ptr{Int32}),
n, convert(Vector{Float64}, x), convert(Vector{Float64}, y),
primid, ldr, convert(Vector{Int32}, datrec))
end
"""
path(x, y, codes)
Draw paths using the given vertices and path codes.
**Parameters:**
`x` :
A list containing the X coordinates
`y` :
A list containing the Y coordinates
`codes` :
A list containing the path codes
The values for `x` and `y` are in world coordinates.
The `codes` describe several path primitives that can be used to create compound paths.
The following path codes are recognized:
+----------+---------------------------------+-------------------+-------------------+
| **Code** | **Description** | **x** | **y** |
+----------+---------------------------------+-------------------+-------------------+
| M, m | move | x | y |
+----------+---------------------------------+-------------------+-------------------+
| L, l | line | x | y |
+----------+---------------------------------+-------------------+-------------------+
| Q, q | quadratic Bezier | x1, x2 | y1, y2 |
+----------+---------------------------------+-------------------+-------------------+
| C, c | cubic Bezier | x1, x2, x3 | y1, y2, y3 |
+----------+---------------------------------+-------------------+-------------------+
| A, a | arc | rx, a1, reserved | ry, a2, reserved |
+----------+---------------------------------+-------------------+-------------------+
| Z | close path | | |
+----------+---------------------------------+-------------------+-------------------+
| S | stroke | | |
+----------+---------------------------------+-------------------+-------------------+
| s | close path and stroke | | |
+----------+---------------------------------+-------------------+-------------------+
| f | close path and fill | | |
+----------+---------------------------------+-------------------+-------------------+
| F | close path, fill and stroke | | |
+----------+---------------------------------+-------------------+-------------------+
- Move: `M`, `m`
Moves the current position to (`x`, `y`). The new position is either absolute (`M`) or relative to the current
position (`m`). The initial position of :code:`path` is (0, 0).
Example:
>>> path([0.5, -0.1], [0.2, 0.1], "Mm")
The first move command in this example moves the current position to the absolute coordinates (0.5, 0.2). The
second move to performs a movement by (-0.1, 0.1) relative to the current position resulting in the point
(0.4, 0.3).
- Line: `L`, `l`
Draws a line from the current position to the given position (`x`, `y`). The end point of the line is either
absolute (`L`) or relative to the current position (`l`). The current position is set to the end point of the
line.
Example:
>>> path([0.1, 0.5, 0.0], [0.1, 0.1, 0.2], "MLlS")
The first line to command draws a straight line from the current position (0.1, 0.1) to the absolute position
(0.5, 0.1) resulting in a horizontal line. The second line to command draws a vertical line relative to the
current position resulting in the end point (0.5, 0.3).
- Quadratic Bezier curve: `Q`, `q`
Draws a quadratic bezier curve from the current position to the end point (`x2`, `y2`) using (`x1`, `y1`) as the
control point. Both points are either absolute (`Q`) or relative to the current position (`q`). The current
position is set to the end point of the bezier curve.
Example:
>>> path([0.1, 0.3, 0.5, 0.2, 0.4], [0.1, 0.2, 0.1, 0.1, 0.0], "MQqS")
This example will generate two bezier curves whose start and end points are each located at y=0.1. As the control
points are horizontally in the middle of each bezier curve with a higher y value both curves are symmetrical
and bend slightly upwards in the middle. The current position is set to (0.9, 0.1) at the end.
- Cubic Bezier curve: `C`, `c`
Draws a cubic bezier curve from the current position to the end point (`x3`, `y3`) using (`x1`, `y1`) and
(`x2`, `y2`) as the control points. All three points are either absolute (`C`) or relative to the current position
(`c`). The current position is set to the end point of the bezier curve.
Example:
>>> path(
... [0.1, 0.2, 0.3, 0.4, 0.1, 0.2, 0.3],
... [0.1, 0.2, 0.0, 0.1, 0.1, -0.1, 0.0],
... "MCcS"
... )
This example will generate two bezier curves whose start and end points are each located at y=0.1. As the control
points are equally spaced along the x-axis and the first is above and the second is below the start and end
points this creates a wave-like shape for both bezier curves. The current position is set to (0.8, 0.1) at the
end.
- Ellipctical arc: `A`, `a`
Draws an elliptical arc starting at the current position. The major axis of the ellipse is aligned with the x-axis
and the minor axis is aligned with the y-axis of the plot. `rx` and `ry` are the ellipses radii along the major
and minor axis. `a1` and `a2` define the start and end angle of the arc in radians. The current position is set
to the end point of the arc. If `a2` is greater than `a1` the arc is drawn counter-clockwise, otherwise it is
drawn clockwise. The `a` and `A` commands draw the same arc. The third coordinates of the `x` and `y` array are
ignored and reserved for future use.
Examples:
>>> path([0.1, 0.2, -3.14159 / 2, 0.0], [0.1, 0.4, 3.14159 / 2, 0.0], "MAS")
This example draws an arc starting at (0.1, 0.1). As the start angle -pi/2 is smaller than the end angle pi/2 the
arc is drawn counter-clockwise. In this case the right half of an ellipse with an x radius of 0.2 and a y radius
of 0.4 is shown. Therefore the current position is set to (0.1, 0.9) at the end.
>>> path([0.1, 0.2, 3.14159 / 2, 0.0], [0.9, 0.4, -3.14159 / 2, 0.0], "MAS")
This examples draws the same arc as the previous one. The only difference is that the starting point is now at
(0.1, 0.9) and the start angle pi/2 is greater than the end angle -pi/2 so that the ellipse arc is drawn
clockwise. Therefore the current position is set to (0.1, 0.1) at the end.
- Close path: `Z`
Closes the current path by connecting the current position to the target position of the last move command
(`m` or `M`) with a straight line. If no move to was performed in this path it connects the current position to
(0, 0). When the path is stroked this line will also be drawn.
- Stroke path: `S`, `s`
Strokes the path with the current border width and border color (set with :code:`gr.setborderwidth` and
:code:`gr.setbordercolorind`). In case of `s` the path is closed beforehand, which is equivalent to `ZS`.
- Fill path: `F`, `f`
Fills the current path using the even-odd-rule using the current fill color. Filling a path implicitly closes
the path. The fill color can be set using :code:`gr.setfillcolorind`. In case of `F` the path is also
stroked using the current border width and color afterwards.
"""
function path(x, y, codes)
@assert length(x) == length(y)
n = length(x)
ccall( libGR_ptr(:gr_path),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Cstring),
n, convert(Vector{Float64}, x), convert(Vector{Float64}, y), codes)
end
function to_rgb_color(z)
z = (z .- minimum(z)) ./ (maximum(z) - minimum(z))
n = length(z)
rgb = zeros(Int, n)
for i in 1:n
rgb[i] = inqcolor(1000 + round(Int, z[i] * 255))
end
rgb
end
function polyline(x, y, linewidth, line_z)
if length(linewidth) == 1
linewidth = ones(length(x)) .* linewidth
end
linewidth = round.(Int, 1000 .* linewidth)
@assert length(x) == length(y) == length(linewidth) == length(line_z)
color = to_rgb_color(line_z)
attributes = vec(hcat(linewidth, color)')
gdp(x, y, GDP_DRAW_LINES, attributes)
end
function polymarker(x, y, markersize, marker_z)
if length(markersize) == 1
markersize = ones(length(x)) .* markersize
end
markersize = round.(Int, 1000 .* markersize)
@assert length(x) == length(y) == length(markersize) == length(marker_z)
color = to_rgb_color(marker_z)
attributes = vec(hcat(markersize, color)')
gdp(x, y, GDP_DRAW_MARKERS, attributes)
end
"""
spline(x, y, m, method)
Generate a cubic spline-fit, starting from the first data point and
ending at the last data point.
**Parameters:**
`x` :
A list containing the X coordinates
`y` :
A list containing the Y coordinates
`m` :
The number of points in the polygon to be drawn (`m` > len(`x`))
`method` :
The smoothing method
The values for `x` and `y` are in world coordinates. The attributes that
control the appearance of a spline-fit are linetype, linewidth and color
index.
If `method` is > 0, then a generalized cross-validated smoothing spline is calculated.
If `method` is 0, then an interpolating natural cubic spline is calculated.
If `method` is < -1, then a cubic B-spline is calculated.
"""
function spline(x, y, m, method)
@assert length(x) == length(y)
n = length(x)
ccall( libGR_ptr(:gr_spline),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Int32, Int32),
n, convert(Vector{Float64}, x), convert(Vector{Float64}, y), m, method)
end
function gridit(xd, yd, zd, nx, ny)
@assert length(xd) == length(yd) == length(zd)
nd = length(xd)
x = Cdouble[1 : nx ;]
y = Cdouble[1 : ny ;]
z = Cdouble[1 : nx*ny ;]
ccall( libGR_ptr(:gr_gridit),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Int32, Int32, Ptr{Cdouble}, Ptr{Cdouble}, Ptr{Cdouble}),
nd, convert(Vector{Float64}, xd), convert(Vector{Float64}, yd), convert(Vector{Float64}, zd), nx, ny, x, y, z)
return x, y, z
end
"""
setlinetype(style::Int)
Specify the line style for polylines.
**Parameters:**
`style` :
The polyline line style
The available line types are:
+---------------------------+----+---------------------------------------------------+
|LINETYPE_SOLID | 1|Solid line |
+---------------------------+----+---------------------------------------------------+
|LINETYPE_DASHED | 2|Dashed line |
+---------------------------+----+---------------------------------------------------+
|LINETYPE_DOTTED | 3|Dotted line |
+---------------------------+----+---------------------------------------------------+
|LINETYPE_DASHED_DOTTED | 4|Dashed-dotted line |
+---------------------------+----+---------------------------------------------------+
|LINETYPE_DASH_2_DOT | -1|Sequence of one dash followed by two dots |
+---------------------------+----+---------------------------------------------------+
|LINETYPE_DASH_3_DOT | -2|Sequence of one dash followed by three dots |
+---------------------------+----+---------------------------------------------------+
|LINETYPE_LONG_DASH | -3|Sequence of long dashes |
+---------------------------+----+---------------------------------------------------+
|LINETYPE_LONG_SHORT_DASH | -4|Sequence of a long dash followed by a short dash |
+---------------------------+----+---------------------------------------------------+
|LINETYPE_SPACED_DASH | -5|Sequence of dashes double spaced |
+---------------------------+----+---------------------------------------------------+
|LINETYPE_SPACED_DOT | -6|Sequence of dots double spaced |
+---------------------------+----+---------------------------------------------------+
|LINETYPE_DOUBLE_DOT | -7|Sequence of pairs of dots |
+---------------------------+----+---------------------------------------------------+
|LINETYPE_TRIPLE_DOT | -8|Sequence of groups of three dots |
+---------------------------+----+---------------------------------------------------+
"""
function setlinetype(style::Int)
ccall( libGR_ptr(:gr_setlinetype),
Nothing,
(Int32, ),
style)
end
"""
setlinewidth(width::Real)
Define the line width of subsequent polyline output primitives.
**Parameters:**
`width` :
The polyline line width scale factor
The line width is calculated as the nominal line width generated
on the workstation multiplied by the line width scale factor.
This value is mapped by the workstation to the nearest available line width.
The default line width is 1.0, or 1 times the line width generated on the graphics device.
"""
function setlinewidth(width::Real)
ccall( libGR_ptr(:gr_setlinewidth),
Nothing,
(Float64, ),
width)
end
"""
setlinecolorind(color::Int)
Define the color of subsequent polyline output primitives.
**Parameters:**
`color` :
The polyline color index (COLOR < 1256)
"""
function setlinecolorind(color::Int)
ccall( libGR_ptr(:gr_setlinecolorind),
Nothing,
(Int32, ),
color)
end
"""
setmarkertype(mtype::Int)
Specifiy the marker type for polymarkers.
**Parameters:**
`style` :
The polymarker marker type
The available marker types are:
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_DOT | 1|Smallest displayable dot |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_PLUS | 2|Plus sign |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_ASTERISK | 3|Asterisk |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_CIRCLE | 4|Hollow circle |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_DIAGONAL_CROSS | 5|Diagonal cross |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_SOLID_CIRCLE | -1|Filled circle |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_TRIANGLE_UP | -2|Hollow triangle pointing upward |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_SOLID_TRI_UP | -3|Filled triangle pointing upward |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_TRIANGLE_DOWN | -4|Hollow triangle pointing downward |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_SOLID_TRI_DOWN | -5|Filled triangle pointing downward |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_SQUARE | -6|Hollow square |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_SOLID_SQUARE | -7|Filled square |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_BOWTIE | -8|Hollow bowtie |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_SOLID_BOWTIE | -9|Filled bowtie |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_HGLASS | -10|Hollow hourglass |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_SOLID_HGLASS | -11|Filled hourglass |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_DIAMOND | -12|Hollow diamond |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_SOLID_DIAMOND | -13|Filled Diamond |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_STAR | -14|Hollow star |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_SOLID_STAR | -15|Filled Star |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_TRI_UP_DOWN | -16|Hollow triangles pointing up and down overlaid |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_SOLID_TRI_RIGHT | -17|Filled triangle point right |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_SOLID_TRI_LEFT | -18|Filled triangle pointing left |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_HOLLOW PLUS | -19|Hollow plus sign |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_SOLID PLUS | -20|Solid plus sign |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_PENTAGON | -21|Pentagon |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_HEXAGON | -22|Hexagon |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_HEPTAGON | -23|Heptagon |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_OCTAGON | -24|Octagon |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_STAR_4 | -25|4-pointed star |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_STAR_5 | -26|5-pointed star (pentagram) |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_STAR_6 | -27|6-pointed star (hexagram) |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_STAR_7 | -28|7-pointed star (heptagram) |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_STAR_8 | -29|8-pointed star (octagram) |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_VLINE | -30|verical line |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_HLINE | -31|horizontal line |
+-----------------------------+-----+------------------------------------------------+
|MARKERTYPE_OMARK | -32|o-mark |
+-----------------------------+-----+------------------------------------------------+
Polymarkers appear centered over their specified coordinates.
"""
function setmarkertype(mtype::Int)
ccall( libGR_ptr(:gr_setmarkertype),
Nothing,
(Int32, ),
mtype)
end
"""
setmarkersize(mtype::Real)
Specify the marker size for polymarkers.
**Parameters:**
`size` :
Scale factor applied to the nominal marker size
The polymarker size is calculated as the nominal size generated on the graphics device
multiplied by the marker size scale factor.
"""
function setmarkersize(mtype::Real)
ccall( libGR_ptr(:gr_setmarkersize),
Nothing,
(Float64, ),
mtype)
end
"""
setmarkercolorind(color::Int)
Define the color of subsequent polymarker output primitives.
**Parameters:**
`color` :
The polymarker color index (COLOR < 1256)
"""
function setmarkercolorind(color::Int)
ccall( libGR_ptr(:gr_setmarkercolorind),
Nothing,
(Int32, ),
color)
end
"""
settextfontprec(font::Int, precision::Int)
Specify the text font and precision for subsequent text output primitives.
**Parameters:**
`font` :
Text font (see tables below)
`precision` :
Text precision (see table below)
The available text fonts are:
+--------------------------------------+-----+
|FONT_TIMES_ROMAN | 101|
+--------------------------------------+-----+
|FONT_TIMES_ITALIC | 102|
+--------------------------------------+-----+
|FONT_TIMES_BOLD | 103|
+--------------------------------------+-----+
|FONT_TIMES_BOLDITALIC | 104|
+--------------------------------------+-----+
|FONT_HELVETICA | 105|
+--------------------------------------+-----+
|FONT_HELVETICA_OBLIQUE | 106|
+--------------------------------------+-----+
|FONT_HELVETICA_BOLD | 107|
+--------------------------------------+-----+
|FONT_HELVETICA_BOLDOBLIQUE | 108|
+--------------------------------------+-----+
|FONT_COURIER | 109|
+--------------------------------------+-----+
|FONT_COURIER_OBLIQUE | 110|
+--------------------------------------+-----+
|FONT_COURIER_BOLD | 111|
+--------------------------------------+-----+
|FONT_COURIER_BOLDOBLIQUE | 112|
+--------------------------------------+-----+
|FONT_SYMBOL | 113|
+--------------------------------------+-----+
|FONT_BOOKMAN_LIGHT | 114|
+--------------------------------------+-----+
|FONT_BOOKMAN_LIGHTITALIC | 115|
+--------------------------------------+-----+
|FONT_BOOKMAN_DEMI | 116|
+--------------------------------------+-----+
|FONT_BOOKMAN_DEMIITALIC | 117|
+--------------------------------------+-----+
|FONT_NEWCENTURYSCHLBK_ROMAN | 118|
+--------------------------------------+-----+
|FONT_NEWCENTURYSCHLBK_ITALIC | 119|
+--------------------------------------+-----+
|FONT_NEWCENTURYSCHLBK_BOLD | 120|
+--------------------------------------+-----+
|FONT_NEWCENTURYSCHLBK_BOLDITALIC | 121|
+--------------------------------------+-----+
|FONT_AVANTGARDE_BOOK | 122|
+--------------------------------------+-----+
|FONT_AVANTGARDE_BOOKOBLIQUE | 123|
+--------------------------------------+-----+
|FONT_AVANTGARDE_DEMI | 124|
+--------------------------------------+-----+
|FONT_AVANTGARDE_DEMIOBLIQUE | 125|
+--------------------------------------+-----+
|FONT_PALATINO_ROMAN | 126|
+--------------------------------------+-----+
|FONT_PALATINO_ITALIC | 127|
+--------------------------------------+-----+
|FONT_PALATINO_BOLD | 128|
+--------------------------------------+-----+
|FONT_PALATINO_BOLDITALIC | 129|
+--------------------------------------+-----+
|FONT_ZAPFCHANCERY_MEDIUMITALIC | 130|
+--------------------------------------+-----+
|FONT_ZAPFDINGBATS | 131|
+--------------------------------------+-----+
The available text precisions are:
+---------------------------+---+--------------------------------------+
|TEXT_PRECISION_STRING | 0|String precision (higher quality) |
+---------------------------+---+--------------------------------------+
|TEXT_PRECISION_CHAR | 1|Character precision (medium quality) |
+---------------------------+---+--------------------------------------+
|TEXT_PRECISION_STROKE | 2|Stroke precision (lower quality) |
+---------------------------+---+--------------------------------------+
The appearance of a font depends on the text precision value specified.
STRING, CHARACTER or STROKE precision allows for a greater or lesser
realization of the text primitives, for efficiency. STRING is the default
precision for GR and produces the highest quality output.
"""
function settextfontprec(font::Int, precision::Int)
ccall( libGR_ptr(:gr_settextfontprec),
Nothing,
(Int32, Int32),
font, precision)
end
"""
setcharexpan(factor::Real)
Set the current character expansion factor (width to height ratio).
**Parameters:**
`factor` :
Text expansion factor applied to the nominal text width-to-height ratio
`setcharexpan` defines the width of subsequent text output primitives. The expansion
factor alters the width of the generated characters, but not their height. The default
text expansion factor is 1, or one times the normal width-to-height ratio of the text.
"""
function setcharexpan(factor::Real)
ccall( libGR_ptr(:gr_setcharexpan),
Nothing,
(Float64, ),
factor)
end
function setcharspace(spacing::Real)
ccall( libGR_ptr(:gr_setcharspace),
Nothing,
(Float64, ),
spacing)
end
"""
settextcolorind(color::Int)
Sets the current text color index.
**Parameters:**
`color` :
The text color index (COLOR < 1256)
`settextcolorind` defines the color of subsequent text output primitives.
GR uses the default foreground color (black=1) for the default text color index.
"""
function settextcolorind(color::Int)
ccall( libGR_ptr(:gr_settextcolorind),
Nothing,
(Int32, ),
color)
end
"""
setcharheight(height::Real)
Set the current character height.
**Parameters:**
`height` :
Text height value
`setcharheight` defines the height of subsequent text output primitives. Text height
is defined as a percentage of the default window. GR uses the default text height of
0.027 (2.7% of the height of the default window).
"""
function setcharheight(height::Real)
ccall( libGR_ptr(:gr_setcharheight),
Nothing,
(Float64, ),
height)
end
function inqcharheight()
_height = Cdouble[0]
ccall( libGR_ptr(:gr_inqcharheight),
Nothing,
(Ptr{Cdouble}, ),
_height)
return _height[1]
end
"""
setcharup(ux::Real, uy::Real)
Set the current character text angle up vector.
**Parameters:**
`ux`, `uy` :
Text up vector
`setcharup` defines the vertical rotation of subsequent text output primitives.
The text up vector is initially set to (0, 1), horizontal to the baseline.
"""
function setcharup(ux::Real, uy::Real)
ccall( libGR_ptr(:gr_setcharup),
Nothing,
(Float64, Float64),
ux, uy)
end
"""
settextpath(path::Int)
Define the current direction in which subsequent text will be drawn.
**Parameters:**
`path` :
Text path (see table below)
+----------------------+---+---------------+
|TEXT_PATH_RIGHT | 0|left-to-right |
+----------------------+---+---------------+
|TEXT_PATH_LEFT | 1|right-to-left |
+----------------------+---+---------------+
|TEXT_PATH_UP | 2|downside-up |
+----------------------+---+---------------+
|TEXT_PATH_DOWN | 3|upside-down |
+----------------------+---+---------------+
"""
function settextpath(path::Int)
ccall( libGR_ptr(:gr_settextpath),
Nothing,
(Int32, ),
path)
end
"""
settextalign(horizontal::Int, vertical::Int)
Set the current horizontal and vertical alignment for text.
**Parameters:**
`horizontal` :
Horizontal text alignment (see the table below)
`vertical` :
Vertical text alignment (see the table below)
`settextalign` specifies how the characters in a text primitive will be aligned
in horizontal and vertical space. The default text alignment indicates horizontal left
alignment and vertical baseline alignment.
+-------------------------+---+----------------+
|TEXT_HALIGN_NORMAL | 0| |
+-------------------------+---+----------------+
|TEXT_HALIGN_LEFT | 1|Left justify |
+-------------------------+---+----------------+
|TEXT_HALIGN_CENTER | 2|Center justify |
+-------------------------+---+----------------+
|TEXT_HALIGN_RIGHT | 3|Right justify |
+-------------------------+---+----------------+
+-------------------------+---+------------------------------------------------+
|TEXT_VALIGN_NORMAL | 0| |
+-------------------------+---+------------------------------------------------+
|TEXT_VALIGN_TOP | 1|Align with the top of the characters |
+-------------------------+---+------------------------------------------------+
|TEXT_VALIGN_CAP | 2|Aligned with the cap of the characters |
+-------------------------+---+------------------------------------------------+
|TEXT_VALIGN_HALF | 3|Aligned with the half line of the characters |
+-------------------------+---+------------------------------------------------+
|TEXT_VALIGN_BASE | 4|Aligned with the base line of the characters |
+-------------------------+---+------------------------------------------------+
|TEXT_VALIGN_BOTTOM | 5|Aligned with the bottom line of the characters |
+-------------------------+---+------------------------------------------------+
"""
function settextalign(horizontal::Int, vertical::Int)
ccall( libGR_ptr(:gr_settextalign),
Nothing,
(Int32, Int32),
horizontal, vertical)
end
"""
setfillintstyle(style::Int)
Set the fill area interior style to be used for fill areas.
**Parameters:**
`style` :
The style of fill to be used
`setfillintstyle` defines the interior style for subsequent fill area output
primitives. The default interior style is HOLLOW.
+---------+---+--------------------------------------------------------------------------------+
|HOLLOW | 0|No filling. Just draw the bounding polyline |
+---------+---+--------------------------------------------------------------------------------+
|SOLID | 1|Fill the interior of the polygon using the fill color index |
+---------+---+--------------------------------------------------------------------------------+
|PATTERN | 2|Fill the interior of the polygon using the style index as a pattern index |
+---------+---+--------------------------------------------------------------------------------+
|HATCH | 3|Fill the interior of the polygon using the style index as a cross-hatched style |
+---------+---+--------------------------------------------------------------------------------+
"""
function setfillintstyle(style::Int)
ccall( libGR_ptr(:gr_setfillintstyle),
Nothing,
(Int32, ),
style)
end
"""
setfillstyle(index::Int)
Sets the fill style to be used for subsequent fill areas.
**Parameters:**
`index` :
The fill style index to be used
`setfillstyle` specifies an index when PATTERN fill or HATCH fill is requested by the
`setfillintstyle` function. If the interior style is set to PATTERN, the fill style
index points to a device-independent pattern table. If interior style is set to HATCH
the fill style index indicates different hatch styles. If HOLLOW or SOLID is specified
for the interior style, the fill style index is unused.
"""
function setfillstyle(index::Int)
ccall( libGR_ptr(:gr_setfillstyle),
Nothing,
(Int32, ),
index)
end
"""
setfillcolorind(color::Int)
Sets the current fill area color index.
**Parameters:**
`color` :
The fill area color index (COLOR < 1256)
`setfillcolorind` defines the color of subsequent fill area output primitives.
GR uses the default foreground color (black=1) for the default fill area color index.
"""
function setfillcolorind(color::Int)
ccall( libGR_ptr(:gr_setfillcolorind),
Nothing,
(Int32, ),
color)
end
"""
setcolorrep(index::Int, red::Real, green::Real, blue::Real)
`setcolorrep` allows to redefine an existing color index representation by specifying
an RGB color triplet.
**Parameters:**
`index` :
Color index in the range 0 to 1256
`red` :
Red intensity in the range 0.0 to 1.0
`green` :
Green intensity in the range 0.0 to 1.0
`blue`:
Blue intensity in the range 0.0 to 1.0
"""
function setcolorrep(index::Int, red::Real, green::Real, blue::Real)
ccall( libGR_ptr(:gr_setcolorrep),
Nothing,
(Int32, Float64, Float64, Float64),
index, red, green, blue)
end
"""
setscale(options::Int)
`setscale` sets the type of transformation to be used for subsequent GR output
primitives.
**Parameters:**
`options` :
Scale specification (see Table below)
+---------------+--------------------+
|OPTION_X_LOG |Logarithmic X-axis |
+---------------+--------------------+
|OPTION_Y_LOG |Logarithmic Y-axis |
+---------------+--------------------+
|OPTION_Z_LOG |Logarithmic Z-axis |
+---------------+--------------------+
|OPTION_FLIP_X |Flip X-axis |
+---------------+--------------------+
|OPTION_FLIP_Y |Flip Y-axis |
+---------------+--------------------+
|OPTION_FLIP_Z |Flip Z-axis |
+---------------+--------------------+
|OPTION_X_LOG2 |log2 scaled X-axis |
+---------------+--------------------+
|OPTION_Y_LOG2 |log2 scaled Y-axis |
+---------------+--------------------+
|OPTION_Z_LOG2 |log2 scaled Z-axis |
+---------------+--------------------+
|OPTION_X_LN |ln scaled X-axis |
+---------------+--------------------+
|OPTION_Y_LN |ln scaled Y-axis |
+---------------+--------------------+
|OPTION_Z_LN |ln scaled Z-axis |
+---------------+--------------------+
`setscale` defines the current transformation according to the given scale
specification which may be or'ed together using any of the above options. GR uses
these options for all subsequent output primitives until another value is provided.
The scale options are used to transform points from an abstract logarithmic or
semi-logarithmic coordinate system, which may be flipped along each axis, into the
world coordinate system.
Note: When applying a logarithmic transformation to a specific axis, the system
assumes that the axes limits are greater than zero.
"""
function setscale(options::Int)
scale = ccall( libGR_ptr(:gr_setscale),
Int32,
(Int32, ),
options)
return scale
end
function inqscale()
_options = Cint[0]
ccall( libGR_ptr(:gr_inqscale),
Nothing,
(Ptr{Int32}, ),
_options)
return _options[1]
end
"""
setwindow(xmin::Real, xmax::Real, ymin::Real, ymax::Real)
`setwindow` establishes a window, or rectangular subspace, of world coordinates to be
plotted. If you desire log scaling or mirror-imaging of axes, use the SETSCALE function.
**Parameters:**
`xmin` :
The left horizontal coordinate of the window (`xmin` < `xmax`).
`xmax` :
The right horizontal coordinate of the window.
`ymin` :
The bottom vertical coordinate of the window (`ymin` < `ymax`).
`ymax` :
The top vertical coordinate of the window.
`setwindow` defines the rectangular portion of the World Coordinate space (WC) to be
associated with the specified normalization transformation. The WC window and the
Normalized Device Coordinates (NDC) viewport define the normalization transformation
through which all output primitives are mapped. The WC window is mapped onto the
rectangular NDC viewport which is, in turn, mapped onto the display surface of the
open and active workstation, in device coordinates. By default, GR uses the range
[0,1] x [0,1], in world coordinates, as the normalization transformation window.
"""
function setwindow(xmin::Real, xmax::Real, ymin::Real, ymax::Real)
ccall( libGR_ptr(:gr_setwindow),
Nothing,
(Float64, Float64, Float64, Float64),
xmin, xmax, ymin, ymax)
end
function inqwindow()
_xmin = Cdouble[0]
_xmax = Cdouble[0]
_ymin = Cdouble[0]
_ymax = Cdouble[0]
ccall( libGR_ptr(:gr_inqwindow),
Nothing,
(Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}),
_xmin, _xmax, _ymin, _ymax)
return _xmin[1], _xmax[1], _ymin[1], _ymax[1]
end
"""
setviewport(xmin::Real, xmax::Real, ymin::Real, ymax::Real)
`setviewport` establishes a rectangular subspace of normalized device coordinates.
**Parameters:**
`xmin` :
The left horizontal coordinate of the viewport.
`xmax` :
The right horizontal coordinate of the viewport (0 <= `xmin` < `xmax` <= 1).
`ymin` :
The bottom vertical coordinate of the viewport.
`ymax` :
The top vertical coordinate of the viewport (0 <= `ymin` < `ymax` <= 1).
`setviewport` defines the rectangular portion of the Normalized Device Coordinate
(NDC) space to be associated with the specified normalization transformation. The
NDC viewport and World Coordinate (WC) window define the normalization transformation
through which all output primitives pass. The WC window is mapped onto the rectangular
NDC viewport which is, in turn, mapped onto the display surface of the open and active
workstation, in device coordinates.
"""
function setviewport(xmin::Real, xmax::Real, ymin::Real, ymax::Real)
ccall( libGR_ptr(:gr_setviewport),
Nothing,
(Float64, Float64, Float64, Float64),
xmin, xmax, ymin, ymax)
end
function inqviewport()
_xmin = Cdouble[0]
_xmax = Cdouble[0]
_ymin = Cdouble[0]
_ymax = Cdouble[0]
ccall( libGR_ptr(:gr_inqviewport),
Nothing,
(Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}),
_xmin, _xmax, _ymin, _ymax)
return _xmin[1], _xmax[1], _ymin[1], _ymax[1]
end
"""
selntran(transform::Int)
`selntran` selects a predefined transformation from world coordinates to normalized
device coordinates.
**Parameters:**
`transform` :
A normalization transformation number.
+------+----------------------------------------------------------------------------------------------------+
| 0|Selects the identity transformation in which both the window and viewport have the range of 0 to 1 |
+------+----------------------------------------------------------------------------------------------------+
| >= 1|Selects a normalization transformation as defined by `setwindow` and `setviewport` |
+------+----------------------------------------------------------------------------------------------------+
"""
function selntran(transform::Int)
ccall( libGR_ptr(:gr_selntran),
Nothing,
(Int32, ),
transform)
end
"""
setclip(indicator::Int)
Set the clipping indicator.
**Parameters:**
`indicator` :
An indicator specifying whether clipping is on or off.
+----+---------------------------------------------------------------+
| 0|Clipping is off. Data outside of the window will be drawn. |
+----+---------------------------------------------------------------+
| 1|Clipping is on. Data outside of the window will not be drawn. |
+----+---------------------------------------------------------------+
`setclip` enables or disables clipping of the image drawn in the current window.
Clipping is defined as the removal of those portions of the graph that lie outside of
the defined viewport. If clipping is on, GR does not draw generated output primitives
past the viewport boundaries. If clipping is off, primitives may exceed the viewport
boundaries, and they will be drawn to the edge of the workstation window.
By default, clipping is on.
"""
function setclip(indicator::Int)
ccall( libGR_ptr(:gr_setclip),
Nothing,
(Int32, ),
indicator)
end
"""
setwswindow(xmin::Real, xmax::Real, ymin::Real, ymax::Real)
Set the area of the NDC viewport that is to be drawn in the workstation window.
**Parameters:**
`xmin` :
The left horizontal coordinate of the workstation window.
`xmax` :
The right horizontal coordinate of the workstation window (0 <= `xmin` < `xmax` <= 1).
`ymin` :
The bottom vertical coordinate of the workstation window.
`ymax` :
The top vertical coordinate of the workstation window (0 <= `ymin` < `ymax` <= 1).
`setwswindow` defines the rectangular area of the Normalized Device Coordinate space
to be output to the device. By default, the workstation transformation will map the
range [0,1] x [0,1] in NDC onto the largest square on the workstation’s display
surface. The aspect ratio of the workstation window is maintained at 1 to 1.
"""
function setwswindow(xmin::Real, xmax::Real, ymin::Real, ymax::Real)
ccall( libGR_ptr(:gr_setwswindow),
Nothing,
(Float64, Float64, Float64, Float64),
xmin, xmax, ymin, ymax)
end
"""
setwsviewport(xmin::Real, xmax::Real, ymin::Real, ymax::Real)
Define the size of the workstation graphics window in meters.
**Parameters:**
`xmin` :
The left horizontal coordinate of the workstation viewport.
`xmax` :
The right horizontal coordinate of the workstation viewport.
`ymin` :
The bottom vertical coordinate of the workstation viewport.
`ymax` :
The top vertical coordinate of the workstation viewport.
`setwsviewport` places a workstation window on the display of the specified size in
meters. This command allows the workstation window to be accurately sized for a
display or hardcopy device, and is often useful for sizing graphs for desktop
publishing applications.
"""
function setwsviewport(xmin::Real, xmax::Real, ymin::Real, ymax::Real)
ccall( libGR_ptr(:gr_setwsviewport),
Nothing,
(Float64, Float64, Float64, Float64),
xmin, xmax, ymin, ymax)
end
function createseg(segment::Int)
ccall( libGR_ptr(:gr_createseg),
Nothing,
(Int32, ),
segment)
end
function copyseg(segment::Int)
ccall( libGR_ptr(:gr_copysegws),
Nothing,
(Int32, ),
segment)
end
function redrawseg()
ccall( libGR_ptr(:gr_redrawsegws),
Nothing,
()
)
end
function setsegtran(segment::Int, fx::Real, fy::Real, transx::Real, transy::Real, phi::Real, scalex::Real, scaley::Real)
ccall( libGR_ptr(:gr_setsegtran),
Nothing,
(Int32, Float64, Float64, Float64, Float64, Float64, Float64, Float64),
segment, fx, fy, transx, transy, phi, scalex, scaley)
end
function closeseg()
ccall( libGR_ptr(:gr_closeseg),
Nothing,
()
)
end
function samplelocator()
x = Cdouble[0]
y = Cdouble[0]
buttons = Cint[0]
ccall( libGR_ptr(:gr_samplelocator),
Nothing,
(Ptr{Float64}, Ptr{Float64}, Ptr{Int32}),
x, y, buttons)
return x[1], y[1], buttons[1]
end
function emergencyclosegks()
ccall( libGR_ptr(:gr_emergencyclosegks),
Nothing,
()
)
end
function updategks()
ccall( libGR_ptr(:gr_updategks),
Nothing,
()
)
end
"""
setspace(zmin::Real, zmax::Real, rotation::Int, tilt::Int)
Set the abstract Z-space used for mapping three-dimensional output primitives into
the current world coordinate space.
**Parameters:**
`zmin` :
Minimum value for the Z-axis.
`zmax` :
Maximum value for the Z-axis.
`rotation` :
Angle for the rotation of the X axis, in degrees.
`tilt` :
Viewing angle of the Z axis in degrees.
`setspace` establishes the limits of an abstract Z-axis and defines the angles for
rotation and for the viewing angle (tilt) of a simulated three-dimensional graph,
used for mapping corresponding output primitives into the current window.
These settings are used for all subsequent three-dimensional output primitives until
other values are specified. Angles of rotation and viewing angle must be specified
between 0° and 90°.
"""
function setspace(zmin::Real, zmax::Real, rotation::Int, tilt::Int)
space = ccall( libGR_ptr(:gr_setspace),
Int32,
(Float64, Float64, Int32, Int32),
zmin, zmax, rotation, tilt)
return space
end
"""
textext(x::Real, y::Real, string)
Draw a text at position `x`, `y` using the current text attributes. Strings can be
defined to create basic mathematical expressions and Greek letters.
**Parameters:**
`x` :
The X coordinate of starting position of the text string
`y` :
The Y coordinate of starting position of the text string
`string` :
The text to be drawn
The values for X and Y are in normalized device coordinates.
The attributes that control the appearance of text are text font and precision,
character expansion factor, character spacing, text color index, character
height, character up vector, text path and text alignment.
The character string is interpreted to be a simple mathematical formula.
The following notations apply:
Subscripts and superscripts: These are indicated by carets ('^') and underscores
('_'). If the sub/superscript contains more than one character, it must be enclosed
in curly braces ('{}').
Fractions are typeset with A '/' B, where A stands for the numerator and B for the
denominator.
To include a Greek letter you must specify the corresponding keyword after a
backslash ('\') character. The text translator produces uppercase or lowercase
Greek letters depending on the case of the keyword.
+--------+---------+
|Letter |Keyword |
+--------+---------+
|Α α |alpha |
+--------+---------+
|Β β |beta |
+--------+---------+
|Γ γ |gamma |
+--------+---------+
|Δ δ |delta |
+--------+---------+
|Ε ε |epsilon |
+--------+---------+
|Ζ ζ |zeta |
+--------+---------+
|Η η |eta |
+--------+---------+
|Θ θ |theta |
+--------+---------+
|Ι ι |iota |
+--------+---------+
|Κ κ |kappa |
+--------+---------+
|Λ λ |lambda |
+--------+---------+
|Μ μ |mu |
+--------+---------+
|Ν ν |nu |
+--------+---------+
|Ξ ξ |xi |
+--------+---------+
|Ο ο |omicron |
+--------+---------+
|Π π |pi |
+--------+---------+
|Ρ ρ |rho |
+--------+---------+
|Σ σ |sigma |
+--------+---------+
|Τ τ |tau |
+--------+---------+
|Υ υ |upsilon |
+--------+---------+
|Φ φ |phi |
+--------+---------+
|Χ χ |chi |
+--------+---------+
|Ψ ψ |psi |
+--------+---------+
|Ω ω |omega |
+--------+---------+
For more sophisticated mathematical formulas, you should use the `gr.mathtex`
function.
"""
function textext(x::Real, y::Real, string)
result = ccall( libGR_ptr(:gr_textext),
Int32,
(Float64, Float64, Ptr{UInt8}),
x, y, latin1(string))
return result
end
function inqtextext(x::Real, y::Real, string)
tbx = Cdouble[0, 0, 0, 0]
tby = Cdouble[0, 0, 0, 0]
ccall( libGR_ptr(:gr_inqtextext),
Nothing,
(Float64, Float64, Ptr{UInt8}, Ptr{Cdouble}, Ptr{Cdouble}),
x, y, latin1(string), tbx, tby)
return tbx, tby
end
"""
axes2d(x_tick::Real, y_tick::Real, x_org::Real, y_org::Real, major_x::Int, major_y::Int, tick_size::Real)
Draw X and Y coordinate axes with linearly and/or logarithmically spaced tick marks.
**Parameters:**
`x_tick`, `y_tick` :
The interval between minor tick marks on each axis.
`x_org`, `y_org` :
The world coordinates of the origin (point of intersection) of the X
and Y axes.
`major_x`, `major_y` :
Unitless integer values specifying the number of minor tick intervals
between major tick marks. Values of 0 or 1 imply no minor ticks.
Negative values specify no labels will be drawn for the associated axis.
`tick_size` :
The length of minor tick marks specified in a normalized device
coordinate unit. Major tick marks are twice as long as minor tick marks.
A negative value reverses the tick marks on the axes from inward facing
to outward facing (or vice versa).
Tick marks are positioned along each axis so that major tick marks fall on the axes
origin (whether visible or not). Major tick marks are labeled with the corresponding
data values. Axes are drawn according to the scale of the window. Axes and tick marks
are drawn using solid lines; line color and width can be modified using the
`setlinetype` and `setlinewidth` functions. Axes are drawn according to
the linear or logarithmic transformation established by the `setscale` function.
"""
function axes2d(x_tick::Real, y_tick::Real, x_org::Real, y_org::Real, major_x::Int, major_y::Int, tick_size::Real)
ccall( libGR_ptr(:gr_axes),
Nothing,
(Float64, Float64, Float64, Float64, Int32, Int32, Float64),
x_tick, y_tick, x_org, y_org, major_x, major_y, tick_size)
end
axes(x_tick::Real, y_tick::Real, x_org::Real, y_org::Real, major_x::Int, major_y::Int, tick_size::Real) = axes2d(x_tick::Real, y_tick::Real, x_org::Real, y_org::Real, major_x::Int, major_y::Int, tick_size::Real)
"""
function axeslbl(x_tick::Real, y_tick::Real, x_org::Real, y_org::Real, major_x::Int, major_y::Int, tick_size::Real, fpx::Function, fpy::Function)
Draw X and Y coordinate axes with linearly and/or logarithmically spaced tick marks.
Tick marks are positioned along each axis so that major tick marks fall on the
axes origin (whether visible or not). Major tick marks are labeled with the
corresponding data values. Axes are drawn according to the scale of the window.
Axes and tick marks are drawn using solid lines; line color and width can be
modified using the `setlinetype` and `setlinewidth` functions.
Axes are drawn according to the linear or logarithmic transformation established
by the `setscale` function.
**Parameters:**
`x_tick`, `y_tick` :
The interval between minor tick marks on each axis.
`x_org`, `y_org` :
The world coordinates of the origin (point of intersection) of the X
and Y axes.
`major_x`, `major_y` :
Unitless integer values specifying the number of minor tick intervals
between major tick marks. Values of 0 or 1 imply no minor ticks.
Negative values specify no labels will be drawn for the associated axis.
`tick_size` :
The length of minor tick marks specified in a normalized device
coordinate unit. Major tick marks are twice as long as minor tick marks.
A negative value reverses the tick marks on the axes from inward facing
to outward facing (or vice versa).
`fx`, `fy` :
Functions that returns a label for a given tick on the X or Y axis.
Those functions should have the following arguments:
`x`, `y` :
Normalized device coordinates of the label in X and Y directions.
`svalue` :
Internal string representation of the text drawn at `(x,y)`.
`value` :
Floating point representation of the label drawn at `(x,y)`.
"""
function axeslbl(x_tick::Real, y_tick::Real, x_org::Real, y_org::Real, major_x::Int, major_y::Int, tick_size::Real, fx::Function, fy::Function)
fx_c = @cfunction($fx, Int32, (Float64, Float64, Cstring, Float64))
fy_c = @cfunction($fy, Int32, (Float64, Float64, Cstring, Float64))
ccall( libGR_ptr(:gr_axeslbl),
Nothing,
(Float64, Float64, Float64, Float64, Int32, Int32, Float64, Ptr{Nothing}, Ptr{Nothing}),
x_tick, y_tick, x_org, y_org, major_x, major_y, tick_size, fx_c, fy_c)
end
"""
grid(x_tick::Real, y_tick::Real, x_org::Real, y_org::Real, major_x::Int, major_y::Int)
Draw a linear and/or logarithmic grid.
**Parameters:**
`x_tick`, `y_tick` :
The length in world coordinates of the interval between minor grid
lines.
`x_org`, `y_org` :
The world coordinates of the origin (point of intersection) of the grid.
`major_x`, `major_y` :
Unitless integer values specifying the number of minor grid lines
between major grid lines. Values of 0 or 1 imply no grid lines.
Major grid lines correspond to the axes origin and major tick marks whether visible
or not. Minor grid lines are drawn at points equal to minor tick marks. Major grid
lines are drawn using black lines and minor grid lines are drawn using gray lines.
"""
function grid(x_tick::Real, y_tick::Real, x_org::Real, y_org::Real, major_x::Int, major_y::Int)
ccall( libGR_ptr(:gr_grid),
Nothing,
(Float64, Float64, Float64, Float64, Int32, Int32),
x_tick, y_tick, x_org, y_org, major_x, major_y)
end
function grid3d(x_tick::Real, y_tick::Real, z_tick::Real, x_org::Real, y_org::Real, z_org::Real, major_x::Int, major_y::Int, major_z::Int)
ccall( libGR_ptr(:gr_grid3d),
Nothing,
(Float64, Float64, Float64, Float64, Float64, Float64, Int32, Int32, Int32),
x_tick, y_tick, z_tick, x_org, y_org, z_org, major_x, major_y, major_z)
end
"""
verrorbars(px, py, e1, e2)
Draw a standard vertical error bar graph.
**Parameters:**
`px` :
A list of length N containing the X coordinates
`py` :
A list of length N containing the Y coordinates
`e1` :
The absolute values of the lower error bar data
`e2` :
The absolute values of the upper error bar data
"""
function verrorbars(px, py, e1, e2)
@assert length(px) == length(py) == length(e1) == length(e2)
n = length(px)
ccall( libGR_ptr(:gr_verrorbars),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}),
n, convert(Vector{Float64}, px), convert(Vector{Float64}, py), convert(Vector{Float64}, e1), convert(Vector{Float64}, e2))
end
"""
herrorbars(px, py, e1, e2)
Draw a standard horizontal error bar graph.
**Parameters:**
`px` :
A list of length N containing the X coordinates
`py` :
A list of length N containing the Y coordinates
`e1` :
The absolute values of the lower error bar data
`e2` :
The absolute values of the upper error bar data
"""
function herrorbars(px, py, e1, e2)
@assert length(px) == length(py) == length(e1) == length(e2)
n = length(px)
ccall( libGR_ptr(:gr_herrorbars),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}),
n, convert(Vector{Float64}, px), convert(Vector{Float64}, py), convert(Vector{Float64}, e1), convert(Vector{Float64}, e2))
end
"""
polyline3d(px, py, pz)
Draw a 3D curve using the current line attributes, starting from the
first data point and ending at the last data point.
**Parameters:**
`x` :
A list of length N containing the X coordinates
`y` :
A list of length N containing the Y coordinates
`z` :
A list of length N containing the Z coordinates
The values for `x`, `y` and `z` are in world coordinates. The attributes that
control the appearance of a polyline are linetype, linewidth and color
index.
"""
function polyline3d(px, py, pz)
@assert length(px) == length(py) == length(pz)
n = length(px)
ccall( libGR_ptr(:gr_polyline3d),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}),
n, convert(Vector{Float64}, px), convert(Vector{Float64}, py), convert(Vector{Float64}, pz))
end
"""
polymarker3d(px, py, pz)
Draw marker symbols centered at the given 3D data points.
**Parameters:**
`x` :
A list of length N containing the X coordinates
`y` :
A list of length N containing the Y coordinates
`z` :
A list of length N containing the Z coordinates
The values for `x`, `y` and `z` are in world coordinates. The attributes
that control the appearance of a polymarker are marker type, marker size
scale factor and color index.
"""
function polymarker3d(px, py, pz)
@assert length(px) == length(py) == length(pz)
n = length(px)
ccall( libGR_ptr(:gr_polymarker3d),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}),
n, convert(Vector{Float64}, px), convert(Vector{Float64}, py), convert(Vector{Float64}, pz))
end
function axes3d(x_tick::Real, y_tick::Real, z_tick::Real, x_org::Real, y_org::Real, z_org::Real, major_x::Int, major_y::Int, major_z::Int, tick_size::Real)
ccall( libGR_ptr(:gr_axes3d),
Nothing,
(Float64, Float64, Float64, Float64, Float64, Float64, Int32, Int32, Int32, Float64),
x_tick, y_tick, z_tick, x_org, y_org, z_org, major_x, major_y, major_z, tick_size)
end
"""
settitles3d(x_title, y_title, z_title)
Set axis titles to be displayed in subsequent axes calls.
**Parameters:**
`x_title`, `y_title`, `z_title` :
The text to be displayed on each axis
"""
function settitles3d(x_title, y_title, z_title)
ccall( libGR_ptr(:gr_settitles3d),
Nothing,
(Ptr{UInt8}, Ptr{UInt8}, Ptr{UInt8}),
latin1(x_title), latin1(y_title), latin1(z_title))
end
"""
titles3d(x_title, y_title, z_title)
Display axis titles just outside of their respective axes.
**Parameters:**
`x_title`, `y_title`, `z_title` :
The text to be displayed on each axis
"""
function titles3d(x_title, y_title, z_title)
ccall( libGR_ptr(:gr_titles3d),
Nothing,
(Ptr{UInt8}, Ptr{UInt8}, Ptr{UInt8}),
latin1(x_title), latin1(y_title), latin1(z_title))
end
"""
surface(px, py, pz, option::Int)
Draw a three-dimensional surface plot for the given data points.
**Parameters:**
`x` :
A list containing the X coordinates
`y` :
A list containing the Y coordinates
`z` :
A list of length `len(x)` * `len(y)` or an appropriately dimensioned
array containing the Z coordinates
`option` :
Surface display option (see table below)
`x` and `y` define a grid. `z` is a singly dimensioned array containing at least
`nx` * `ny` data points. Z describes the surface height at each point on the grid.
Data is ordered as shown in the following table:
+------------------+--+--------------------------------------------------------------+
|LINES | 0|Use X Y polylines to denote the surface |
+------------------+--+--------------------------------------------------------------+
|MESH | 1|Use a wire grid to denote the surface |
+------------------+--+--------------------------------------------------------------+
|FILLED_MESH | 2|Applies an opaque grid to the surface |
+------------------+--+--------------------------------------------------------------+
|Z_SHADED_MESH | 3|Applies Z-value shading to the surface |
+------------------+--+--------------------------------------------------------------+
|COLORED_MESH | 4|Applies a colored grid to the surface |
+------------------+--+--------------------------------------------------------------+
|CELL_ARRAY | 5|Applies a grid of individually-colored cells to the surface |
+------------------+--+--------------------------------------------------------------+
|SHADED_MESH | 6|Applies light source shading to the 3-D surface |
+------------------+--+--------------------------------------------------------------+
"""
function surface(px, py, pz, option::Int)
nx = length(px)
ny = length(py)
if isa(pz, Function)
f = pz
pz = Float64[f(x,y) for y in py, x in px]
end
nz = length(pz)
if ndims(pz) == 1
out_of_bounds = nz != nx * ny
elseif ndims(pz) == 2
out_of_bounds = size(pz)[1] != nx || size(pz)[2] != ny
else
out_of_bounds = true
end
if !out_of_bounds
if ndims(pz) == 2
pz = reshape(pz, nx * ny)
end
ccall( libGR_ptr(:gr_surface),
Nothing,
(Int32, Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Int32),
nx, ny, convert(Vector{Float64}, px), convert(Vector{Float64}, py), convert(Vector{Float64}, pz), option)
else
error("Arrays have incorrect length or dimension.")
end
end
"""
contour(px, py, h, pz, major_h::Int)
Draw contours of a three-dimensional data set whose values are specified over a
rectangular mesh. Contour lines may optionally be labeled.
**Parameters:**
`x` :
A list containing the X coordinates
`y` :
A list containing the Y coordinates
`h` :
A list containing the Z coordinate for the height values
`z` :
A list of length `len(x)` * `len(y)` or an appropriately dimensioned
array containing the Z coordinates
`major_h` :
Directs GR to label contour lines. For example, a value of 3 would label
every third line. A value of 1 will label every line. A value of 0
produces no labels. To produce colored contour lines, add an offset
of 1000 to `major_h`.
"""
function contour(px, py, h, pz, major_h::Int)
nx = length(px)
ny = length(py)
nh = length(h)
if isa(pz, Function)
f = pz
pz = Float64[f(x,y) for y in py, x in px]
end
nz = length(pz)
if ndims(pz) == 1
out_of_bounds = nz != nx * ny
elseif ndims(pz) == 2
out_of_bounds = size(pz)[1] != nx || size(pz)[2] != ny
else
out_of_bounds = true
end
if !out_of_bounds
if ndims(pz) == 2
pz = reshape(pz, nx * ny)
end
ccall( libGR_ptr(:gr_contour),
Nothing,
(Int32, Int32, Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Int32),
nx, ny, nh, convert(Vector{Float64}, px), convert(Vector{Float64}, py), convert(Vector{Float64}, h), convert(Vector{Float64}, pz), major_h)
else
error("Arrays have incorrect length or dimension.")
end
end
"""
contourf(px, py, h, pz, major_h::Int)
Draw filled contours of a three-dimensional data set whose values are
specified over a rectangular mesh.
**Parameters:**
`x` :
A list containing the X coordinates
`y` :
A list containing the Y coordinates
`h` :
A list containing the Z coordinate for the height values
`z` :
A list of length `len(x)` * `len(y)` or an appropriately dimensioned
array containing the Z coordinates
`major_h` :
(intended for future use)
"""
function contourf(px, py, h, pz, major_h::Int)
nx = length(px)
ny = length(py)
nh = length(h)
if isa(pz, Function)
f = pz
pz = Float64[f(x,y) for y in py, x in px]
end
nz = length(pz)
if ndims(pz) == 1
out_of_bounds = nz != nx * ny
elseif ndims(pz) == 2
out_of_bounds = size(pz)[1] != nx || size(pz)[2] != ny
else
out_of_bounds = true
end
if !out_of_bounds
if ndims(pz) == 2
pz = reshape(pz, nx * ny)
end
ccall( libGR_ptr(:gr_contourf),
Nothing,
(Int32, Int32, Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Int32),
nx, ny, nh, convert(Vector{Float64}, px), convert(Vector{Float64}, py), convert(Vector{Float64}, h), convert(Vector{Float64}, pz), major_h)
else
error("Arrays have incorrect length or dimension.")
end
end
function hexbin(x, y, nbins)
@assert length(x) == length(y)
n = length(x)
cntmax = ccall( libGR_ptr(:gr_hexbin),
Int32,
(Int32, Ptr{Float64}, Ptr{Float64}, Int32),
n, convert(Vector{Float64}, x), convert(Vector{Float64}, y), nbins)
return cntmax
end
function setcolormap(index::Int)
ccall( libGR_ptr(:gr_setcolormap),
Nothing,
(Int32, ),
index)
end
function colorbar()
ccall( libGR_ptr(:gr_colorbar),
Nothing,
()
)
end
function inqcolor(color::Int)
rgb = Cint[0]
ccall( libGR_ptr(:gr_inqcolor),
Nothing,
(Int32, Ptr{Int32}),
color, rgb)
return rgb[1]
end
function inqcolorfromrgb(red::Real, green::Real, blue::Real)
color = ccall( libGR_ptr(:gr_inqcolorfromrgb),
Int32,
(Float64, Float64, Float64),
red, green, blue)
return color
end
function hsvtorgb(h::Real, s::Real, v::Real)
r = Cdouble[0]
g = Cdouble[0]
b = Cdouble[0]
ccall( libGR_ptr(:gr_hsvtorgb),
Nothing,
(Float64, Float64, Float64, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}),
h, s, v, r, g, b)
return r[1], g[1], b[1]
end
function tick(amin::Real, amax::Real)
return ccall( libGR_ptr(:gr_tick),
Float64,
(Float64, Float64),
amin, amax)
end
function validaterange(amin::Real, amax::Real)
return ccall( libGR_ptr(:gr_validaterange),
Int32,
(Float64, Float64),
amin, amax)
end
function adjustlimits(amin::Real, amax::Real)
_amin = Cdouble[amin]
_amax = Cdouble[amax]
ccall( libGR_ptr(:gr_adjustlimits),
Nothing,
(Ptr{Float64}, Ptr{Float64}),
_amin, _amax)
return _amin[1], _amax[1]
end
function adjustrange(amin::Real, amax::Real)
_amin = Cdouble[amin]
_amax = Cdouble[amax]
ccall( libGR_ptr(:gr_adjustrange),
Nothing,
(Ptr{Float64}, Ptr{Float64}),
_amin, _amax)
return _amin[1], _amax[1]
end
"""
beginprint(pathname)
Open and activate a print device.
**Parameters:**
`pathname` :
Filename for the print device.
`beginprint` opens an additional graphics output device. The device type is obtained
from the given file extension. The following file types are supported:
+-------------+---------------------------------------+
|.ps, .eps |PostScript |
+-------------+---------------------------------------+
|.pdf |Portable Document Format |
+-------------+---------------------------------------+
|.bmp |Windows Bitmap (BMP) |
+-------------+---------------------------------------+
|.jpeg, .jpg |JPEG image file |
+-------------+---------------------------------------+
|.png |Portable Network Graphics file (PNG) |
+-------------+---------------------------------------+
|.tiff, .tif |Tagged Image File Format (TIFF) |
+-------------+---------------------------------------+
|.fig |Xfig vector graphics file |
+-------------+---------------------------------------+
|.svg |Scalable Vector Graphics |
+-------------+---------------------------------------+
|.wmf |Windows Metafile |
+-------------+---------------------------------------+
"""
function beginprint(pathname)
ccall( libGR_ptr(:gr_beginprint),
Nothing,
(Ptr{Cchar}, ),
pathname)
end
"""
beginprintext(pathname, mode, fmt, orientation)
Open and activate a print device with the given layout attributes.
**Parameters:**
`pathname` :
Filename for the print device.
`mode` :
Output mode (Color, GrayScale)
`fmt` :
Output format (see table below)
`orientation` :
Page orientation (Landscape, Portait)
The available formats are:
+-----------+---------------+
|A4 |0.210 x 0.297 |
+-----------+---------------+
|B5 |0.176 x 0.250 |
+-----------+---------------+
|Letter |0.216 x 0.279 |
+-----------+---------------+
|Legal |0.216 x 0.356 |
+-----------+---------------+
|Executive |0.191 x 0.254 |
+-----------+---------------+
|A0 |0.841 x 1.189 |
+-----------+---------------+
|A1 |0.594 x 0.841 |
+-----------+---------------+
|A2 |0.420 x 0.594 |
+-----------+---------------+
|A3 |0.297 x 0.420 |
+-----------+---------------+
|A5 |0.148 x 0.210 |
+-----------+---------------+
|A6 |0.105 x 0.148 |
+-----------+---------------+
|A7 |0.074 x 0.105 |
+-----------+---------------+
|A8 |0.052 x 0.074 |
+-----------+---------------+
|A9 |0.037 x 0.052 |
+-----------+---------------+
|B0 |1.000 x 1.414 |
+-----------+---------------+
|B1 |0.500 x 0.707 |
+-----------+---------------+
|B10 |0.031 x 0.044 |
+-----------+---------------+
|B2 |0.500 x 0.707 |
+-----------+---------------+
|B3 |0.353 x 0.500 |
+-----------+---------------+
|B4 |0.250 x 0.353 |
+-----------+---------------+
|B6 |0.125 x 0.176 |
+-----------+---------------+
|B7 |0.088 x 0.125 |
+-----------+---------------+
|B8 |0.062 x 0.088 |
+-----------+---------------+
|B9 |0.044 x 0.062 |
+-----------+---------------+
|C5E |0.163 x 0.229 |
+-----------+---------------+
|Comm10E |0.105 x 0.241 |
+-----------+---------------+
|DLE |0.110 x 0.220 |
+-----------+---------------+
|Folio |0.210 x 0.330 |
+-----------+---------------+
|Ledger |0.432 x 0.279 |
+-----------+---------------+
|Tabloid |0.279 x 0.432 |
+-----------+---------------+
"""
function beginprintext(pathname, mode, fmt, orientation)
ccall( libGR_ptr(:gr_beginprintext),
Nothing,
(Ptr{Cchar}, Ptr{Cchar}, Ptr{Cchar}, Ptr{Cchar}),
pathname, mode, fmt, orientation)
end
function endprint()
ccall( libGR_ptr(:gr_endprint),
Nothing,
()
)
end
function ndctowc(x::Real, y::Real)
_x = Cdouble[x]
_y = Cdouble[y]
ccall( libGR_ptr(:gr_ndctowc),
Nothing,
(Ptr{Float64}, Ptr{Float64}),
_x, _y)
return _x[1], _y[1]
end
function wctondc(x::Real, y::Real)
_x = Cdouble[x]
_y = Cdouble[y]
ccall( libGR_ptr(:gr_wctondc),
Nothing,
(Ptr{Float64}, Ptr{Float64}),
_x, _y)
return _x[1], _y[1]
end
function wc3towc(x::Real, y::Real, z::Real)
_x = Cdouble[x]
_y = Cdouble[y]
_z = Cdouble[z]
ccall( libGR_ptr(:gr_wc3towc),
Nothing,
(Ptr{Float64}, Ptr{Float64}, Ptr{Float64}),
_x, _y, _z)
return _x[1], _y[1], _z[1]
end
"""
drawrect(xmin::Real, xmax::Real, ymin::Real, ymax::Real)
Draw a rectangle using the current line attributes.
**Parameters:**
`xmin` :
Lower left edge of the rectangle
`xmax` :
Lower right edge of the rectangle
`ymin` :
Upper left edge of the rectangle
`ymax` :
Upper right edge of the rectangle
"""
function drawrect(xmin::Real, xmax::Real, ymin::Real, ymax::Real)
ccall( libGR_ptr(:gr_drawrect),
Nothing,
(Float64, Float64, Float64, Float64),
xmin, xmax, ymin, ymax)
end
"""
fillrect(xmin::Real, xmax::Real, ymin::Real, ymax::Real)
Draw a filled rectangle using the current fill attributes.
**Parameters:**
`xmin` :
Lower left edge of the rectangle
`xmax` :
Lower right edge of the rectangle
`ymin` :
Upper left edge of the rectangle
`ymax` :
Upper right edge of the rectangle
"""
function fillrect(xmin::Real, xmax::Real, ymin::Real, ymax::Real)
ccall( libGR_ptr(:gr_fillrect),
Nothing,
(Float64, Float64, Float64, Float64),
xmin, xmax, ymin, ymax)
end
"""
drawarc(xmin::Real, xmax::Real, ymin::Real, ymax::Real, a1::Real, a2::Real)
Draw a circular or elliptical arc covering the specified rectangle.
**Parameters:**
`xmin` :
Lower left edge of the rectangle
`xmax` :
Lower right edge of the rectangle
`ymin` :
Upper left edge of the rectangle
`ymax` :
Upper right edge of the rectangle
`a1` :
The start angle
`a2` :
The end angle
The resulting arc begins at `a1` and ends at `a2` degrees. Angles are interpreted
such that 0 degrees is at the 3 o'clock position. The center of the arc is the center
of the given rectangle.
"""
function drawarc(xmin::Real, xmax::Real, ymin::Real, ymax::Real, a1::Real, a2::Real)
ccall( libGR_ptr(:gr_drawarc),
Nothing,
(Float64, Float64, Float64, Float64, Float64, Float64),
xmin, xmax, ymin, ymax, a1, a2)
end
"""
fillarc(xmin::Real, xmax::Real, ymin::Real, ymax::Real, a1::Real, a2::Real)
Fill a circular or elliptical arc covering the specified rectangle.
**Parameters:**
`xmin` :
Lower left edge of the rectangle
`xmax` :
Lower right edge of the rectangle
`ymin` :
Upper left edge of the rectangle
`ymax` :
Upper right edge of the rectangle
`a1` :
The start angle
`a2` :
The end angle
The resulting arc begins at `a1` and ends at `a2` degrees. Angles are interpreted
such that 0 degrees is at the 3 o'clock position. The center of the arc is the center
of the given rectangle.
"""
function fillarc(xmin::Real, xmax::Real, ymin::Real, ymax::Real, a1::Real, a2::Real)
ccall( libGR_ptr(:gr_fillarc),
Nothing,
(Float64, Float64, Float64, Float64, Float64, Float64),
xmin, xmax, ymin, ymax, a1, a2)
end
"""
drawpath(points, codes, fill::Int)
Draw simple and compound outlines consisting of line segments and bezier curves.
**Parameters:**
`points` :
(N, 2) array of (x, y) vertices
`codes` :
N-length array of path codes
`fill` :
A flag indication whether resulting path is to be filled or not
The following path codes are recognized:
+----------+-----------------------------------------------------------+
| STOP|end the entire path |
+----------+-----------------------------------------------------------+
| MOVETO|move to the given vertex |
+----------+-----------------------------------------------------------+
| LINETO|draw a line from the current position to the given vertex |
+----------+-----------------------------------------------------------+
| CURVE3|draw a quadratic Bézier curve |
+----------+-----------------------------------------------------------+
| CURVE4|draw a cubic Bézier curve |
+----------+-----------------------------------------------------------+
| CLOSEPOLY|draw a line segment to the start point of the current path |
+----------+-----------------------------------------------------------+
"""
function drawpath(points, codes, fill::Int)
len = length(codes)
ccall( libGR_ptr(:gr_drawpath),
Nothing,
(Int32, Ptr{Float64}, Ptr{UInt8}, Int32),
len, convert(Vector{Float64}, points), convert(Vector{UInt8}, codes), fill)
end
"""
setarrowstyle(style::Int)
Set the arrow style to be used for subsequent arrow commands.
**Parameters:**
`style` :
The arrow style to be used
`setarrowstyle` defines the arrow style for subsequent arrow primitives.
The default arrow style is 1.
+---+----------------------------------+
| 1|simple, single-ended |
+---+----------------------------------+
| 2|simple, single-ended, acute head |
+---+----------------------------------+
| 3|hollow, single-ended |
+---+----------------------------------+
| 4|filled, single-ended |
+---+----------------------------------+
| 5|triangle, single-ended |
+---+----------------------------------+
| 6|filled triangle, single-ended |
+---+----------------------------------+
| 7|kite, single-ended |
+---+----------------------------------+
| 8|filled kite, single-ended |
+---+----------------------------------+
| 9|simple, double-ended |
+---+----------------------------------+
| 10|simple, double-ended, acute head |
+---+----------------------------------+
| 11|hollow, double-ended |
+---+----------------------------------+
| 12|filled, double-ended |
+---+----------------------------------+
| 13|triangle, double-ended |
+---+----------------------------------+
| 14|filled triangle, double-ended |
+---+----------------------------------+
| 15|kite, double-ended |
+---+----------------------------------+
| 16|filled kite, double-ended |
+---+----------------------------------+
| 17|double line, single-ended |
+---+----------------------------------+
| 18|double line, double-ended |
+---+----------------------------------+
"""
function setarrowstyle(style::Int)
ccall( libGR_ptr(:gr_setarrowstyle),
Nothing,
(Int32, ),
style)
end
"""
setarrowsize(size::Real)
Set the arrow size to be used for subsequent arrow commands.
**Parameters:**
`size` :
The arrow size to be used
`setarrowsize` defines the arrow size for subsequent arrow primitives.
The default arrow size is 1.
"""
function setarrowsize(size::Real)
ccall( libGR_ptr(:gr_setarrowsize),
Nothing,
(Float64, ),
size)
end
"""
drawarrow(x1::Real, y1::Real, x2::Real, y2::Real)
Draw an arrow between two points.
**Parameters:**
`x1`, `y1` :
Starting point of the arrow (tail)
`x2`, `y2` :
Head of the arrow
Different arrow styles (angles between arrow tail and wing, optionally filled
heads, double headed arrows) are available and can be set with the `setarrowstyle`
function.
"""
function drawarrow(x1::Real, y1::Real, x2::Real, y2::Real)
ccall( libGR_ptr(:gr_drawarrow),
Nothing,
(Float64, Float64, Float64, Float64),
x1, y1, x2, y2)
end
function readimage(path)
width = Cint[0]
height = Cint[0]
data = Array{Ptr{UInt32}}(undef, 1)
ret = ccall( libGR_ptr(:gr_readimage),
Int32,
(Ptr{Cchar}, Ptr{Int32}, Ptr{Int32}, Ptr{Ptr{UInt32}}),
path, width, height, data)
if width[1] > 0 && height[1] > 0
img = unsafe_wrap(Array{UInt32}, data[1], (width[1], height[1]))
return Int(width[1]), Int(height[1]), img
else
return 0, 0, zeros(UInt32, 0)
end
end
"""
drawimage(xmin::Real, xmax::Real, ymin::Real, ymax::Real, width::Int, height::Int, data, model::Int = 0)
Draw an image into a given rectangular area.
**Parameters:**
`xmin`, `ymin` :
First corner point of the rectangle
`xmax`, `ymax` :
Second corner point of the rectangle
`width`, `height` :
The width and the height of the image
`data` :
An array of color values dimensioned `width` by `height`
`model` :
Color model (default=0)
The available color models are:
+-----------------------+---+-----------+
|MODEL_RGB | 0| AABBGGRR|
+-----------------------+---+-----------+
|MODEL_HSV | 1| AAVVSSHH|
+-----------------------+---+-----------+
The points (`xminx`, `ymin`) and (`xmax`, `ymax`) are world coordinates defining
diagonally opposite corner points of a rectangle. This rectangle is divided into
`width` by `height` cells. The two-dimensional array `data` specifies colors
for each cell.
"""
function drawimage(xmin::Real, xmax::Real, ymin::Real, ymax::Real, width::Int, height::Int, data, model::Int = 0)
if ndims(data) == 2
data = reshape(data, width * height)
end
ccall( libGR_ptr(:gr_drawimage),
Nothing,
(Float64, Float64, Float64, Float64, Int32, Int32, Ptr{UInt32}, Int32),
xmin, xmax, ymin, ymax, width, height, convert(Vector{UInt32}, data), model)
end
function importgraphics(path)
return ccall( libGR_ptr(:gr_importgraphics),
Int32,
(Ptr{Cchar}, ),
path)
end
"""
setshadow(offsetx::Real, offsety::Real, blur::Real)
`setshadow` allows drawing of shadows, realized by images painted underneath,
and offset from, graphics objects such that the shadow mimics the effect of a light
source cast on the graphics objects.
**Parameters:**
`offsetx` :
An x-offset, which specifies how far in the horizontal direction the
shadow is offset from the object
`offsety` :
A y-offset, which specifies how far in the vertical direction the shadow
is offset from the object
`blur` :
A blur value, which specifies whether the object has a hard or a diffuse
edge
"""
function setshadow(offsetx::Real, offsety::Real, blur::Real)
ccall( libGR_ptr(:gr_setshadow),
Nothing,
(Float64, Float64, Float64),
offsetx, offsety, blur)
end
"""
settransparency(alpha::Real)
Set the value of the alpha component associated with GR colors.
**Parameters:**
`alpha` :
An alpha value (0.0 - 1.0)
"""
function settransparency(alpha::Real)
ccall( libGR_ptr(:gr_settransparency),
Nothing,
(Float64, ),
alpha)
end
"""
setcoordxform(mat)
Change the coordinate transformation according to the given matrix.
**Parameters:**
`mat[3][2]` :
2D transformation matrix
"""
function setcoordxform(mat)
@assert length(mat) == 6
ccall( libGR_ptr(:gr_setcoordxform),
Nothing,
(Ptr{Float64}, ),
convert(Vector{Float64}, mat))
end
"""
begingraphics(path)
Open a file for graphics output.
**Parameters:**
`path` :
Filename for the graphics file.
`begingraphics` allows to write all graphics output into a XML-formatted file until
the `endgraphics` functions is called. The resulting file may later be imported with
the `importgraphics` function.
"""
function begingraphics(path)
ccall( libGR_ptr(:gr_begingraphics),
Nothing,
(Ptr{Cchar}, ),
path)
end
function endgraphics()
ccall( libGR_ptr(:gr_endgraphics),
Nothing,
()
)
end
function getgraphics()
string = ccall( libGR_ptr(:gr_getgraphics),
Ptr{Cchar},
(),
)
return string != C_NULL ? unsafe_string(string) : ""
end
function drawgraphics(string)
ret = ccall( libGR_ptr(:gr_drawgraphics),
Int32,
(Ptr{Cchar}, ),
string)
return Int(ret)
end
function startlistener()
ret = ccall( libGR_ptr(:gr_startlistener),
Int32,
(),
)
return Int(ret)
end
"""
mathtex(x::Real, y::Real, string)
Generate a character string starting at the given location. Strings can be defined
to create mathematical symbols and Greek letters using LaTeX syntax.
**Parameters:**
`x`, `y` :
Position of the text string specified in world coordinates
`string` :
The text string to be drawn
"""
function mathtex(x::Real, y::Real, string)
if length(string) >= 2 && string[1] == '$' && string[end] == '$'
string = string[2:end-1]
end
ccall( libGR_ptr(:gr_mathtex),
Nothing,
(Float64, Float64, Ptr{Cchar}),
x, y, string)
end
function inqmathtex(x, y, string)
if length(string) >= 2 && string[1] == '$' && string[end] == '$'
string = string[2:end-1]
end
tbx = Cdouble[0, 0, 0, 0]
tby = Cdouble[0, 0, 0, 0]
ccall( libGR_ptr(:gr_inqmathtex),
Nothing,
(Float64, Float64, Ptr{UInt8}, Ptr{Cdouble}, Ptr{Cdouble}),
x, y, string, tbx, tby)
return tbx, tby
end
const ASF_BUNDLED = 0
const ASF_INDIVIDUAL = 1
const NOCLIP = 0
const CLIP = 1
const COORDINATES_WC = 0
const COORDINATES_NDC = 1
const INTSTYLE_HOLLOW = 0
const INTSTYLE_SOLID = 1
const INTSTYLE_PATTERN = 2
const INTSTYLE_HATCH = 3
const TEXT_HALIGN_NORMAL = 0
const TEXT_HALIGN_LEFT = 1
const TEXT_HALIGN_CENTER = 2
const TEXT_HALIGN_RIGHT = 3
const TEXT_VALIGN_NORMAL = 0
const TEXT_VALIGN_TOP = 1
const TEXT_VALIGN_CAP = 2
const TEXT_VALIGN_HALF = 3
const TEXT_VALIGN_BASE = 4
const TEXT_VALIGN_BOTTOM = 5
const TEXT_PATH_RIGHT = 0
const TEXT_PATH_LEFT = 1
const TEXT_PATH_UP = 2
const TEXT_PATH_DOWN = 3
const TEXT_PRECISION_STRING = 0
const TEXT_PRECISION_CHAR = 1
const TEXT_PRECISION_STROKE = 2
const TEXT_PRECISION_OUTLINE = 3
const LINETYPE_SOLID = 1
const LINETYPE_DASHED = 2
const LINETYPE_DOTTED = 3
const LINETYPE_DASHED_DOTTED = 4
const LINETYPE_DASH_2_DOT = -1
const LINETYPE_DASH_3_DOT = -2
const LINETYPE_LONG_DASH = -3
const LINETYPE_LONG_SHORT_DASH = -4
const LINETYPE_SPACED_DASH = -5
const LINETYPE_SPACED_DOT = -6
const LINETYPE_DOUBLE_DOT = -7
const LINETYPE_TRIPLE_DOT = -8
const MARKERTYPE_DOT = 1
const MARKERTYPE_PLUS = 2
const MARKERTYPE_ASTERISK = 3
const MARKERTYPE_CIRCLE = 4
const MARKERTYPE_DIAGONAL_CROSS = 5
const MARKERTYPE_SOLID_CIRCLE = -1
const MARKERTYPE_TRIANGLE_UP = -2
const MARKERTYPE_SOLID_TRI_UP = -3
const MARKERTYPE_TRIANGLE_DOWN = -4
const MARKERTYPE_SOLID_TRI_DOWN = -5
const MARKERTYPE_SQUARE = -6
const MARKERTYPE_SOLID_SQUARE = -7
const MARKERTYPE_BOWTIE = -8
const MARKERTYPE_SOLID_BOWTIE = -9
const MARKERTYPE_HOURGLASS = -10
const MARKERTYPE_SOLID_HGLASS = -11
const MARKERTYPE_DIAMOND = -12
const MARKERTYPE_SOLID_DIAMOND = -13
const MARKERTYPE_STAR = -14
const MARKERTYPE_SOLID_STAR = -15
const MARKERTYPE_TRI_UP_DOWN = -16
const MARKERTYPE_SOLID_TRI_RIGHT = -17
const MARKERTYPE_SOLID_TRI_LEFT = -18
const MARKERTYPE_HOLLOW_PLUS = -19
const MARKERTYPE_SOLID_PLUS = -20
const MARKERTYPE_PENTAGON = -21
const MARKERTYPE_HEXAGON = -22
const MARKERTYPE_HEPTAGON = -23
const MARKERTYPE_OCTAGON = -24
const MARKERTYPE_STAR_4 = -25
const MARKERTYPE_STAR_5 = -26
const MARKERTYPE_STAR_6 = -27
const MARKERTYPE_STAR_7 = -28
const MARKERTYPE_STAR_8 = -29
const MARKERTYPE_VLINE = -30
const MARKERTYPE_HLINE = -31
const MARKERTYPE_OMARK = -32
const OPTION_X_LOG = 1
const OPTION_Y_LOG = 2
const OPTION_Z_LOG = 4
const OPTION_FLIP_X = 8
const OPTION_FLIP_Y = 16
const OPTION_FLIP_Z = 32
const OPTION_X_LOG2 = 64
const OPTION_Y_LOG2 = 128
const OPTION_Z_LOG2 = 256
const OPTION_X_LN = 512
const OPTION_Y_LN = 1024
const OPTION_Z_LN = 2048
const SPEC_LINE = 1
const SPEC_MARKER = 2
const SPEC_COLOR = 4
const OPTION_LINES = 0
const OPTION_MESH = 1
const OPTION_FILLED_MESH = 2
const OPTION_Z_SHADED_MESH = 3
const OPTION_COLORED_MESH = 4
const OPTION_CELL_ARRAY = 5
const OPTION_SHADED_MESH = 6
const OPTION_3D_MESH = 7
const MODEL_RGB = 0
const MODEL_HSV = 1
const COLORMAP_UNIFORM = 0
const COLORMAP_TEMPERATURE = 1
const COLORMAP_GRAYSCALE = 2
const COLORMAP_GLOWING = 3
const COLORMAP_RAINBOWLIKE = 4
const COLORMAP_GEOLOGIC = 5
const COLORMAP_GREENSCALE = 6
const COLORMAP_CYANSCALE = 7
const COLORMAP_BLUESCALE = 8
const COLORMAP_MAGENTASCALE = 9
const COLORMAP_REDSCALE = 10
const COLORMAP_FLAME = 11
const COLORMAP_BROWNSCALE = 12
const COLORMAP_PILATUS = 13
const COLORMAP_AUTUMN = 14
const COLORMAP_BONE = 15
const COLORMAP_COOL = 16
const COLORMAP_COPPER = 17
const COLORMAP_GRAY = 18
const COLORMAP_HOT = 19
const COLORMAP_HSV = 20
const COLORMAP_JET = 21
const COLORMAP_PINK = 22
const COLORMAP_SPECTRAL = 23
const COLORMAP_SPRING = 24
const COLORMAP_SUMMER = 25
const COLORMAP_WINTER = 26
const COLORMAP_GIST_EARTH = 27
const COLORMAP_GIST_HEAT = 28
const COLORMAP_GIST_NCAR = 29
const COLORMAP_GIST_RAINBOW = 30
const COLORMAP_GIST_STERN = 31
const COLORMAP_AFMHOT = 32
const COLORMAP_BRG = 33
const COLORMAP_BWR = 34
const COLORMAP_COOLWARM = 35
const COLORMAP_CMRMAP = 36
const COLORMAP_CUBEHELIX = 37
const COLORMAP_GNUPLOT = 38
const COLORMAP_GNUPLOT2 = 39
const COLORMAP_OCEAN = 40
const COLORMAP_RAINBOW = 41
const COLORMAP_SEISMIC = 42
const COLORMAP_TERRAIN = 43
const COLORMAP_VIRIDIS = 44
const COLORMAP_INFERNO = 45
const COLORMAP_PLASMA = 46
const COLORMAP_MAGMA = 47
const FONT_TIMES_ROMAN = 101
const FONT_TIMES_ITALIC = 102
const FONT_TIMES_BOLD = 103
const FONT_TIMES_BOLDITALIC = 104
const FONT_HELVETICA = 105
const FONT_HELVETICA_OBLIQUE = 106
const FONT_HELVETICA_BOLD = 107
const FONT_HELVETICA_BOLDOBLIQUE = 108
const FONT_COURIER = 109
const FONT_COURIER_OBLIQUE = 110
const FONT_COURIER_BOLD = 111
const FONT_COURIER_BOLDOBLIQUE = 112
const FONT_SYMBOL = 113
const FONT_BOOKMAN_LIGHT = 114
const FONT_BOOKMAN_LIGHTITALIC = 115
const FONT_BOOKMAN_DEMI = 116
const FONT_BOOKMAN_DEMIITALIC = 117
const FONT_NEWCENTURYSCHLBK_ROMAN = 118
const FONT_NEWCENTURYSCHLBK_ITALIC = 119
const FONT_NEWCENTURYSCHLBK_BOLD = 120
const FONT_NEWCENTURYSCHLBK_BOLDITALIC = 121
const FONT_AVANTGARDE_BOOK = 122
const FONT_AVANTGARDE_BOOKOBLIQUE = 123
const FONT_AVANTGARDE_DEMI = 124
const FONT_AVANTGARDE_DEMIOBLIQUE = 125
const FONT_PALATINO_ROMAN = 126
const FONT_PALATINO_ITALIC = 127
const FONT_PALATINO_BOLD = 128
const FONT_PALATINO_BOLDITALIC = 129
const FONT_ZAPFCHANCERY_MEDIUMITALIC = 130
const FONT_ZAPFDINGBATS = 131
const TEXT_USE_WC = 1
const TEXT_ENABLE_INLINE_MATH = 2
const PATH_STOP = 0x00
const PATH_MOVETO = 0x01
const PATH_LINETO = 0x02
const PATH_CURVE3 = 0x03
const PATH_CURVE4 = 0x04
const PATH_CLOSEPOLY = 0x4f
const GDP_DRAW_PATH = 1
const GDP_DRAW_LINES = 2
const GDP_DRAW_MARKERS = 3
const MPL_SUPPRESS_CLEAR = 1
const MPL_POSTPONE_UPDATE = 2
const XFORM_BOOLEAN = 0
const XFORM_LINEAR = 1
const XFORM_LOG = 2
const XFORM_LOGLOG = 3
const XFORM_CUBIC = 4
const XFORM_EQUALIZED = 5
const AXES_SIMPLE_AXES = 1
const AXES_TWIN_AXES = 2
const AXES_WITH_GRID = 4
# GR3 functions
include("gr3.jl")
const gr3 = GR.GR3
# Convenience functions
include("jlgr.jl")
# Rather than redefining the methods in GR
# 1. Export them in jlgr
# 2. Import them here via using
using .jlgr
mutable struct SVG s::Array{UInt8} end
mutable struct PNG s::Array{UInt8} end
mutable struct HTML s::AbstractString end
Base.show(io::IO, ::MIME"image/svg+xml", x::SVG) = write(io, x.s)
Base.show(io::IO, ::MIME"image/png", x::PNG) = write(io, x.s)
Base.show(io::IO, ::MIME"text/html", x::HTML) = print(io, x.s)
struct c_tick_t
value::Cdouble
is_major::Cint
end
struct c_tick_label_t
tick::Cdouble
label::Cstring
width::Cdouble
end
Base.@kwdef mutable struct c_axis_t
min::Cdouble = NaN
max::Cdouble = NaN
tick::Cdouble = NaN
org::Cdouble = NaN
position::Cdouble = NaN
major_count::Cint = 1
num_ticks::Cint = 0
ticks::Ptr{c_tick_t} = C_NULL
tick_size::Cdouble = NaN
num_tick_labels::Cint = 0
tick_labels::Ptr{c_tick_label_t} = C_NULL
label_position::Cdouble = NaN
draw_axis_line::Cint = 1
end
mutable struct GRTick
value::Real
is_major::Int
end
mutable struct GRTickLabel
tick::Real
label::String
width::Real
end
Base.@kwdef mutable struct GRAxis
min::Real = NaN
max::Real = NaN
tick::Real = NaN
org::Real = NaN
position::Real = NaN
major_count::Int = 1
num_ticks::Int = 0
ticks::Vector{GRTick} = nothing
tick_size::Real = NaN
tick_labels::Vector{GRTickLabel} = nothing
label_position::Real = NaN
draw_axis_line::Int = 1
end
function _readfile(path)
data = Array{UInt8}(undef, filesize(path))
s = open(path, "r")
content = read!(s, data)
close(s)
content
end
function isinline()
return !(mime_type[] in ("", "mov", "mp4", "webm"))
end
function displayname()
return display_name[]
end
function inline(mime="svg", scroll=true)
init()
if mime_type[] != mime
@debug "MIME type change" mime_type[] mime
if mime == "iterm"
file_path[] = tempname() * ".png"
ENV["GKS_WSTYPE"] = "png"
usecolorscheme(is_dark_mode() ? 2 : 1)
elseif mime == "mlterm"
file_path[] = tempname() * ".six"
ENV["GKS_WSTYPE"] = "six"
elseif mime == "js"
file_path[] = nothing
ENV["GRDISPLAY"] = "js"
send_c[], recv_c[] = js.initjs()
else
file_path[] = tempname() * "." * mime
ENV["GKS_WSTYPE"] = mime
end
if file_path[] !== nothing
ENV["GKS_FILEPATH"] = file_path[]
end
@debug mime file_path[] ENV["GKS_WSTYPE"]
emergencyclosegks()
mime_type[] = mime
end
figure_count[] = scroll ? -1 : 0
@debug mime_type[]
mime_type[]
end
function reset()
mime_type[] = ""
file_path[] = ""
figure_count[] = -1
delete!(ENV, "GKS_WSTYPE")
delete!(ENV, "GKS_FILEPATH")
emergencyclosegks()
end
function show()
if !isempty(mime_type[])
emergencyclosegks()
end
if mime_type[] == "svg"
content = SVG(_readfile(file_path[]))
rm(file_path[])
return content
elseif mime_type[] == "png"
content = PNG(_readfile(file_path[]))
rm(file_path[])
return content
elseif mime_type[] in ("mov", "mp4", "webm")
mimespec = mime_type[] == "mov" ? "video/mp4" : "video/$(mime_type[])"
content = HTML(string("""<video autoplay controls><source type="$mimespec" src="data:$mimespec;base64,""", Base64.base64encode(open(read,file_path[])),""""></video>"""))
rm(file_path[])
return content
elseif mime_type[] == "iterm"
content = string(osc_seq(), "1337;File=inline=1;height=24;preserveAspectRatio=0:", Base64.base64encode(open(read,file_path[])), st_seq())
if figure_count[] != -1
figure_count[] += 1
(figure_count[] > 1) && print("\e[24A")
end
println(content)
rm(file_path[])
return nothing
elseif mime_type[] == "mlterm"
content = read(file_path[], String)
println(content)
rm(file_path[])
return nothing
end
return nothing
end
function setregenflags(flags=0)
ccall( libGR_ptr(:gr_setregenflags),
Nothing,
(Int32, ),
flags)
end
function inqregenflags()
flags = ccall( libGR_ptr(:gr_inqregenflags),
Int32,
()
)
return flags
end
function savestate()
ccall( libGR_ptr(:gr_savestate),
Nothing,
()
)
end
function restorestate()
ccall( libGR_ptr(:gr_restorestate),
Nothing,
()
)
end
function selectcontext(context::Int)
ccall( libGR_ptr(:gr_selectcontext),
Nothing,
(Int32, ),
context)
end
function destroycontext(context::Int)
ccall( libGR_ptr(:gr_destroycontext),
Nothing,
(Int32, ),
context)
end
function uselinespec(linespec)
return ccall( libGR_ptr(:gr_uselinespec),
Int32,
(Ptr{Cchar}, ),
linespec)
end
function delaunay(x, y)
@assert length(x) == length(y)
npoints = length(x)
ntri = Cint[0]
dim = Cint[3]
triangles = Array{Ptr{Int32}}(undef, 1)
ccall( libGR_ptr(:gr_delaunay),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Int32}, Ptr{Ptr{Int32}}),
npoints, convert(Vector{Float64}, x), convert(Vector{Float64}, y),
ntri, triangles)
if ntri[1] > 0
tri = unsafe_wrap(Array{Int32}, triangles[1], (dim[1], ntri[1]))
return Int(ntri[1]), tri' .+ 1
else
return 0, zeros(Int32, 0)
end
end
function interp2(X, Y, Z, Xq, Yq, method::Int=0, extrapval=0)
nx = length(X)
ny = length(Y)
if isa(Z, Function)
f = Z
Z = Float64[f(x,y) for x in X, y in Y]
end
nz = length(Z)
if ndims(Z) == 1
out_of_bounds = nz != nx * ny
elseif ndims(Z) == 2
out_of_bounds = size(Z)[1] != ny || size(Z)[2] != nx
else
out_of_bounds = true
end
Zq = []
if !out_of_bounds
if ndims(Z) == 2
Z = reshape(Z, nx * ny)
end
nxq = length(Xq)
nyq = length(Yq)
Zq = Cdouble[1 : nxq * nyq; ]
ccall( libGR_ptr(:gr_interp2),
Nothing,
(Int32, Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Int32, Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Cdouble}, Int32, Float64),
ny, nx, convert(Vector{Float64}, Y), convert(Vector{Float64}, X), convert(Vector{Float64}, Z), nyq, nxq, convert(Vector{Float64}, Yq), convert(Vector{Float64}, Xq), Zq, method, extrapval)
reshape(Zq, nyq, nxq)
else
error("Arrays have incorrect length or dimension.")
Z
end
end
"""
trisurface(x, y, z)
Draw a triangular surface plot for the given data points.
**Parameters:**
`x` :
A list containing the X coordinates
`y` :
A list containing the Y coordinates
`z` :
A list containing the Z coordinates
"""
function trisurface(x, y, z)
nx = length(x)
ny = length(y)
nz = length(z)
n = min(nx, ny, nz)
ccall( libGR_ptr(:gr_trisurface),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}),
n, convert(Vector{Float64}, x), convert(Vector{Float64}, y), convert(Vector{Float64}, z))
end
"""
tricontour(x, y, z, levels)
Draw a contour plot for the given triangle mesh.
**Parameters:**
`x` :
A list containing the X coordinates
`y` :
A list containing the Y coordinates
`z` :
A list containing the Z coordinates
`levels` :
A list containing the contour levels
"""
function tricontour(x, y, z, levels)
npoints = length(x)
nlevels = length(levels)
ccall( libGR_ptr(:gr_tricontour),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Int32, Ptr{Float64}),
npoints, convert(Vector{Float64}, x), convert(Vector{Float64}, y), convert(Vector{Float64}, z), nlevels, convert(Vector{Float64}, levels))
end
function gradient(x, y, z)
nx = length(x)
ny = length(y)
nz = length(z)
if ndims(z) == 1
out_of_bounds = nz != nx * ny
elseif ndims(z) == 2
out_of_bounds = size(z)[1] != nx || size(z)[2] != ny
else
out_of_bounds = true
end
if !out_of_bounds
if ndims(z) == 2
z = reshape(z, nx * ny)
end
u = Cdouble[1 : nx*ny ;]
v = Cdouble[1 : nx*ny ;]
ccall( libGR_ptr(:gr_gradient),
Nothing,
(Int32, Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Ptr{Cdouble}, Ptr{Cdouble}),
nx, ny, convert(Vector{Float64}, x), convert(Vector{Float64}, y), convert(Vector{Float64}, z), u, v)
return u, v
else
return [], []
end
end
function quiver(x, y, u, v, color::Bool=false)
nx = length(x)
ny = length(y)
nu = length(u)
nv = length(v)
if ndims(u) == 1
out_of_bounds = nu != nx * ny
elseif ndims(u) == 2
out_of_bounds = size(u)[1] != nx || size(u)[2] != ny
else
out_of_bounds = true
end
if !out_of_bounds
if ndims(v) == 1
out_of_bounds = nv != nx * ny
elseif ndims(v) == 2
out_of_bounds = size(v)[1] != nx || size(v)[2] != ny
else
out_of_bounds = true
end
end
if !out_of_bounds
if ndims(u) == 2
u = reshape(u, nx * ny)
end
if ndims(v) == 2
v = reshape(v, nx * ny)
end
ccall( libGR_ptr(:gr_quiver),
Nothing,
(Int32, Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Int32),
nx, ny, convert(Vector{Float64}, x), convert(Vector{Float64}, y), convert(Vector{Float64}, u), convert(Vector{Float64}, v), convert(Int32, color))
else
error("Arrays have incorrect length or dimension.")
end
end
function reducepoints(xd, yd, n)
@assert length(xd) == length(yd)
nd = length(xd)
x = Cdouble[1 : n ;]
y = Cdouble[1 : n ;]
ccall( libGR_ptr(:gr_reducepoints),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Int32, Ptr{Cdouble}, Ptr{Cdouble}),
nd, convert(Vector{Float64}, xd), convert(Vector{Float64}, yd), n, x, y)
return x, y
end
function version()
info = ccall( libGR_ptr(:gr_version),
Cstring,
()
)
unsafe_string(info)
end
function check_for_updates()
@eval GR begin
import HTTP
requ = HTTP.request("GET", "https://api.github.com/repos/sciapp/gr/releases/latest")
body = String(requ.body)
import JSON
tag = JSON.parse(body)["tag_name"]
end
release = replace(string("v", version()), ".post" => " patchlevel ")
if release < tag
println("An update is available: GR $tag. You're using GR $release.")
elseif release == tag
println("You're up-to-date. GR $tag is currently the newest version available.")
else
println("You're using a pre-release version: GR $release.")
end
release < tag
end
function openmeta(target=0, device="localhost", port=8002)
handle = ccall(libGRM_ptr(:grm_open),
Ptr{Nothing},
(Int32, Cstring, Int64, Ptr{Cvoid}, Ptr{Cvoid}),
target, device, port, send_c[], recv_c[])
return handle
end
function sendmeta(handle, string::AbstractString)
ccall(libGRM_ptr(:grm_send),
Nothing,
(Ptr{Nothing}, Cstring),
handle, string)
end
function sendmetaref(handle, key::AbstractString, fmt::Char, data, len=-1)
if typeof(data) <: String
if len == -1
len = length(data)
end
ccall(libGRM_ptr(:grm_send_ref),
Nothing,
(Ptr{Nothing}, Cstring, Cchar, Cstring, Int32),
handle, key, fmt, data, len)
else
if len == -1
len = length(data)
end
if typeof(data) <: Array
if typeof(data[1]) <: String
ccall(libGRM_ptr(:grm_send_ref),
Nothing,
(Ptr{Nothing}, Cstring, Cchar, Ptr{Ptr{Cchar}}, Int32),
handle, key, fmt, data, len)
return
else
ref = Ref(data, 1)
end
else
ref = Ref(data)
end
ccall(libGRM_ptr(:grm_send_ref),
Nothing,
(Ptr{Nothing}, Cstring, Cchar, Ptr{Nothing}, Int32),
handle, key, fmt, ref, len)
end
end
function recvmeta(handle, args=C_NULL)
args = ccall(libGRM_ptr(:grm_recv),
Ptr{Nothing},
(Ptr{Nothing}, Ptr{Nothing}),
handle, args)
return args
end
function plotmeta(args)
ccall(libGRM_ptr(:grm_plot),
Nothing,
(Ptr{Nothing}, ),
args)
end
function deletemeta(args)
ccall(libGRM_ptr(:grm_args_delete),
Nothing,
(Ptr{Nothing}, ),
args)
end
function closemeta(handle)
ccall(libGRM_ptr(:grm_close),
Nothing,
(Ptr{Nothing}, ),
handle)
end
function shadepoints(x, y; dims=[1200, 1200], xform=1)
@assert length(x) == length(y)
n = length(x)
w, h = dims
ccall( libGR_ptr(:gr_shadepoints),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Int32, Int32, Int32),
n, convert(Vector{Float64}, x), convert(Vector{Float64}, y),
xform, w, h)
end
function shadelines(x, y; dims=[1200, 1200], xform=1)
@assert length(x) == length(y)
n = length(x)
w, h = dims
ccall( libGR_ptr(:gr_shadelines),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Int32, Int32, Int32),
n, convert(Vector{Float64}, x), convert(Vector{Float64}, y),
xform, w, h)
end
function setcolormapfromrgb(r, g, b; positions=Nothing)
@assert length(r) == length(g) == length(b)
n = length(r)
if positions === Nothing
positions = C_NULL
else
@assert length(positions) == n
positions = convert(Vector{Float64}, positions)
end
ccall( libGR_ptr(:gr_setcolormapfromrgb),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}),
n, convert(Vector{Float64}, r), convert(Vector{Float64}, g),
convert(Vector{Float64}, b), positions)
end
function panzoom(x, y, zoom)
xmin = Cdouble[0]
xmax = Cdouble[0]
ymin = Cdouble[0]
ymax = Cdouble[0]
ccall( libGR_ptr(:gr_panzoom),
Nothing,
(Float64, Float64, Float64, Float64, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}),
x, y, zoom, zoom, xmin, xmax, ymin, ymax)
return xmin[1], xmax[1], ymin[1], ymax[1]
end
"""
setborderwidth(width::Real)
Define the border width of subsequent path output primitives.
**Parameters:**
`width` :
The border width scale factor
"""
function setborderwidth(width::Real)
ccall( libGR_ptr(:gr_setborderwidth),
Nothing,
(Float64, ),
width)
end
"""
setbordercolorind(color::Int)
Define the color of subsequent path output primitives.
**Parameters:**
`color` :
The border color index (COLOR < 1256)
"""
function setbordercolorind(color::Int)
ccall( libGR_ptr(:gr_setbordercolorind),
Nothing,
(Int32, ),
color)
end
function setprojectiontype(type::Int)
ccall( libGR_ptr(:gr_setprojectiontype),
Nothing,
(Int32, ),
type)
end
function setperspectiveprojection(near_plane::Real, far_plane::Real, fov::Real)
ccall( libGR_ptr(:gr_setperspectiveprojection),
Nothing,
(Float64, Float64, Float64),
near_plane, far_plane, fov)
end
function setorthographicprojection(left::Real, right::Real, bottom::Real, top::Real, near_plane::Real, far_plane::Real)
ccall( libGR_ptr(:gr_setorthographicprojection),
Nothing,
(Float64, Float64, Float64, Float64, Float64, Float64),
left, right, bottom, top, near_plane, far_plane)
end
function settransformationparameters(camera_pos_x::Real, camera_pos_y::Real, camera_pos_z::Real,
up_x::Real, up_y::Real, up_z::Real,
focus_point_x::Real, focus_point_y::Real, focus_point_z::Real)
ccall( libGR_ptr(:gr_settransformationparameters),
Nothing,
(Float64, Float64, Float64, Float64, Float64, Float64, Float64, Float64, Float64),
camera_pos_x, camera_pos_y, camera_pos_z, up_x, up_y, up_z, focus_point_x, focus_point_y, focus_point_z)
end
function setresamplemethod(method::UInt32)
ccall( libGR_ptr(:gr_setresamplemethod),
Nothing,
(UInt32, ),
method)
end
function setwindow3d(xmin::Real, xmax::Real, ymin::Real, ymax::Real, zmin::Real, zmax::Real)
ccall( libGR_ptr(:gr_setwindow3d),
Nothing,
(Float64, Float64, Float64, Float64, Float64, Float64),
xmin, xmax, ymin, ymax, zmin, zmax)
end
function setspace3d(rot::Real, tilt::Real, fov::Real, dist::Real)
ccall( libGR_ptr(:gr_setspace3d),
Nothing,
(Float64, Float64, Float64, Float64),
rot, tilt, fov, dist)
end
function text3d(x::Real, y::Real, z::Real, string, axis::Int)
ccall( libGR_ptr(:gr_text3d),
Nothing,
(Float64, Float64, Float64, Ptr{UInt8}, Int32),
x, y, z, latin1(string), axis)
end
function inqtext3d(x::Real, y::Real, z::Real, string, axis::Int)
tbx = Cdouble[0 for i in 1:16]
tby = Cdouble[0 for i in 1:16]
ccall( libGR_ptr(:gr_inqtext3d),
Nothing,
(Float64, Float64, Float64, Ptr{UInt8}, Int32, Ptr{Cdouble}, Ptr{Cdouble}),
x, y, z, latin1(string), axis, tbx, tby)
return tbx, tby
end
function settextencoding(encoding)
ccall( libGR_ptr(:gr_settextencoding),
Nothing,
(Int32, ),
encoding)
text_encoding[] = encoding
end
function inqtextencoding()
encoding = Cint[0]
ccall( libGR_ptr(:gr_inqtextencoding),
Nothing,
(Ptr{Cint}, ),
encoding)
return encoding[1]
end
function loadfont(name::String)
font = Cint[0]
ccall( libGR_ptr(:gr_loadfont),
Cstring,
(Cstring, Ptr{Cint}),
name, font)
return Int(font[1])
end
function inqvpsize()
width = Cint[0]
height = Cint[0]
device_pixel_ratio = Cdouble[0]
ccall( libGR_ptr(:gr_inqvpsize),
Nothing,
(Ptr{Cint}, Ptr{Cint}, Ptr{Cdouble}),
width, height, device_pixel_ratio)
return width[1], height[1], device_pixel_ratio[1]
end
function setpicturesizeforvolume(width::Int, height::Int)
ccall( libGR_ptr(:gr_setpicturesizeforvolume),
Nothing,
(Cint, Cint),
width, height)
end
function inqtransformationparameters()
cam_x = Cdouble[0]
cam_y = Cdouble[0]
cam_z = Cdouble[0]
up_x = Cdouble[0]
up_y = Cdouble[0]
up_z = Cdouble[0]
foc_x = Cdouble[0]
foc_y = Cdouble[0]
foc_z = Cdouble[0]
ccall( libGR_ptr(:gr_inqtransformationparameters),
Nothing,
(Ptr{Cdouble}, Ptr{Cdouble}, Ptr{Cdouble}, Ptr{Cdouble}, Ptr{Cdouble}, Ptr{Cdouble}, Ptr{Cdouble}, Ptr{Cdouble}, Ptr{Cdouble}),
cam_x, cam_y, cam_z, up_x, up_y, up_z, foc_x, foc_y, foc_z)
return cam_x[1], cam_y[1], cam_z[1], up_x[1], up_y[1], up_z[1], foc_x[1], foc_y[1], foc_z[1]
end
function polygonmesh3d(px, py, pz, connections, colors)
@assert length(px) == length(py) == length(pz)
num_points = length(px)
num_connections = length(colors)
ccall( libGR_ptr(:gr_polygonmesh3d),
Nothing,
(Int32, Ptr{Float64}, Ptr{Float64}, Ptr{Float64}, Int32, Ptr{Int32}, Ptr{Int32}),
num_points, convert(Vector{Float64}, px), convert(Vector{Float64}, py), convert(Vector{Float64}, pz), num_connections, convert(Vector{Int32}, connections), convert(Vector{Int32}, colors))
end
function setscientificformat(format_option)
ccall( libGR_ptr(:gr_setscientificformat),
Nothing,
(Int32, ),
format_option)
end
function setresizebehaviour(flag)
ccall( libGR_ptr(:gr_setresizebehaviour),
Nothing,
(Int32, ),
flag)
end
function inqprojectiontype()
proj = Cint[0]
ccall( libGR_ptr(:gr_inqprojectiontype),
Nothing,
(Ptr{Cint}, ),
proj)
return Int(proj[1])
end
function beginselection(index, type)
ccall( libGR_ptr(:gr_beginselection),
Nothing,
(Cint, Cint),
index, type)
end
function endselection()
ccall( libGR_ptr(:gr_endselection),
Nothing,
(),
)
end
function moveselection(x, y)
ccall( libGR_ptr(:gr_moveselection),
Nothing,
(Cdouble, Cdouble),
x, y)
end
function setmathfont(font::Int)
ccall( libGR_ptr(:gr_setmathfont),
Nothing,
(Int32, ),
font)
end
function inqmathfont()
_font = Cint[0]
ccall( libGR_ptr(:gr_inqmathfont),
Nothing,
(Ptr{Cint}, ),
_font)
return _font[1]
end
function setclipregion(region::Int)
ccall( libGR_ptr(:gr_setclipregion),
Nothing,
(Int32, ),
region)
end
function inqclipregion()
_region = Cint[0]
ccall( libGR_ptr(:gr_inqclipregion),
Nothing,
(Ptr{Cint}, ),
_region)
return _region[1]
end
function axis(which::Char; min::Real = NaN, max::Real = NaN, tick::Real = NaN, org::Real = NaN, position::Real = NaN, major_count::Int = 1, ticks::Union{Vector{GRTick}, Nothing} = nothing, tick_size::Real = NaN, tick_labels::Union{Vector{GRTickLabel}, Nothing} = nothing, label_position::Real = NaN, draw_axis_line::Int = 1)::GRAxis
c_axis = c_axis_t(min=min, max=max, tick=tick, org=org, position=position, major_count=major_count, tick_size=tick_size, label_position=label_position, draw_axis_line=draw_axis_line)
if ticks != nothing
c_axis.ticks = pointer(ticks)
c_axis.num_ticks = size(ticks)[1]
else
c_axis.ticks = C_NULL
c_axis.num_ticks = 0
end
if tick_labels != nothing
c_axis.tick_labels = pointer(tick_labels)
c_axis.num_tick_labels = size(tick_labels)[1]
else
c_axis.tick_labels = C_NULL
c_axis.num_tick_labels = 0
end
ccall( libGR_ptr(:gr_axis),
Nothing,
(Cchar, Ptr{c_axis_t}),
which, Ref(c_axis))
ticks = GRTick[]
for i in 1:c_axis.num_ticks
tick = unsafe_load(c_axis.ticks, i)
push!(ticks, GRTick(tick.value, tick.is_major))
end
tick_labels = GRTickLabel[]
for i in 1:c_axis.num_tick_labels
tick_label = unsafe_load(c_axis.tick_labels, i)
if tick_label.label != C_NULL
push!(tick_labels, GRTickLabel(tick_label.tick, unsafe_string(tick_label.label), tick_label.width))
end
end
return GRAxis(min=c_axis.min, max=c_axis.max, tick=c_axis.tick, org=c_axis.org, position=c_axis.position, major_count=c_axis.major_count, ticks=ticks, tick_size=c_axis.tick_size, tick_labels=tick_labels, label_position=c_axis.label_position, draw_axis_line=c_axis.draw_axis_line)
end
function to_c_axis(axis::GRAxis)::c_axis_t
c_axis = c_axis_t(min=axis.min, max=axis.max, tick=axis.tick, org=axis.org, position=axis.position, major_count=axis.major_count, tick_size=axis.tick_size, label_position=axis.label_position, draw_axis_line=axis.draw_axis_line)
if axis.ticks != nothing
ticks = c_tick_t[]
for tick in axis.ticks
push!(ticks, c_tick_t(tick.value, tick.is_major))
end
c_axis.ticks = pointer(ticks)
c_axis.num_ticks = size(axis.ticks)[1]
else
c_axis.ticks = C_NULL
c_axis.num_ticks = 0
end
if axis.tick_labels != nothing
tick_labels = c_tick_label_t[]
for tick_label in axis.tick_labels
push!(tick_labels, c_tick_label_t(tick_label.tick, pointer(tick_label.label), tick_label.width))
end
c_axis.tick_labels = pointer(tick_labels)
c_axis.num_tick_labels = size(axis.tick_labels)[1]
else
c_axis.tick_labels = C_NULL
c_axis.num_tick_labels = 0
end
c_axis
end
function drawaxis(which::Char, axis::GRAxis)
c_axis = to_c_axis(axis)
ccall( libGR_ptr(:gr_drawaxis),
Nothing,
(Cchar, Ptr{c_axis_t}),
which, Ref(c_axis))
end
function drawaxes(x_axis::Union{GRAxis, Nothing}, y_axis::Union{GRAxis, Nothing}, options::Int=AXES_SIMPLE_AXES|AXES_TWIN_AXES|AXES_WITH_GRID)
if x_axis !== nothing
c_x_axis = to_c_axis(x_axis)
end
if y_axis !== nothing
c_y_axis = to_c_axis(y_axis)
end
ccall( libGR_ptr(:gr_drawaxes),
Nothing,
(Ptr{c_axis_t}, Ptr{c_axis_t}, Cint),
x_axis !== nothing ? Ref(c_x_axis) : C_NULL, y_axis !== nothing ? Ref(c_y_axis) : C_NULL, options)
end
# JS functions
include("js.jl")
include("precompile.jl")
_precompile_()
end # module
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 9140 | """
GR.GRPreferences.Downloader is a Module that contains the GR download script.
GR.GRPreferences.Downloader.download() can be invoked manually.
"""
module Downloader
using Tar
using Downloads
using p7zip_jll
const version = v"0.73.7"
"""
get_grdir()
Try to locate an existing GR install. The search will look
in the following places:
1. ENV["GRDIR"]
2. ~/gr
3. /opt/gr
4. /usr/local/gr
5. /usr/gr
It will confirm the install by checking for the existence of a fonts
subdirectory.
The function will return a `String` representing one of the paths above
or `nothing` if a GR install is not located in any of those locations.
"""
function get_grdir()
if "GRDIR" in keys(ENV)
grdir = ENV["GRDIR"]
if (have_dir = !isempty(grdir))
have_dir = isdir(joinpath(grdir, "fonts"))
end
else
have_dir = false
for d in (homedir(), "/opt", "/usr/local", "/usr")
grdir = joinpath(d, "gr")
if isdir(joinpath(grdir, "fonts"))
have_dir = true
break
end
end
end
have_dir && @info "Found existing GR run-time in $grdir"
return have_dir ? grdir : nothing
end
"""
get_version()
Get the version of GR.jl package.
If ENV["GRDIR"] exists and non-empty, this will return "latest"
"""
function get_version()
_version = version
if "GRDIR" in keys(ENV)
if isempty(ENV["GRDIR"])
_version = "latest"
end
end
return _version
end
"""
get_os_release(key)
Grep for key in /etc/os-release
"""
function get_os_release(key)
value = ""
try
#String(read(pipeline(`cat /etc/os-release`, `grep ^$key=`, `cut -d= -f2`)))[1:end-1]
for line in readlines("/etc/os-release")
if startswith(line, "$key=")
value = split(line, "=")[2]
end
end
catch
end
return replace(value, "\"" => "")
end
"""
get_os_and_arch()
Figure out which specific operating system this, including the specific Linux distribution.
"""
function get_os_and_arch()
os = Sys.iswindows() ? "Windows" : string(Sys.KERNEL)
arch = Sys.ARCH
if Sys.islinux() && Sys.ARCH == :x86_64
if isfile("/etc/redhat-release")
# example = "Red Hat Enterprise Linux Server release 6.5 (Santiago)"
#=
rel = String(
read(
pipeline(
`cat /etc/redhat-release`,
`sed s/.\*release\ //`,
`sed s/\ .\*//`,
),
),
)[1:end-1]
=#
rel = read("/etc/redhat-release", String)
rel = replace(rel, r".*release " => "", r" .*" => "")
rel = strip(rel)
if rel > "7.0"
os = "Redhat"
end
elseif isfile("/etc/os-release")
#=
example = """
PRETTY_NAME="Ubuntu 22.04.1 LTS"
NAME="Ubuntu"
VERSION_ID="22.04"
VERSION="22.04.1 LTS (Jammy Jellyfish)"
VERSION_CODENAME=jammy
ID=ubuntu
ID_LIKE=debian
HOME_URL="https://www.ubuntu.com/"
SUPPORT_URL="https://help.ubuntu.com/"
BUG_REPORT_URL="https://bugs.launchpad.net/ubuntu/"
PRIVACY_POLICY_URL="https://www.ubuntu.com/legal/terms-and-policies/privacy-policy"
UBUNTU_CODENAME=jammy
"""
=#
id = get_os_release("ID")
id_like = get_os_release("ID_LIKE")
if id == "ubuntu" || id == "pop" || id_like == "ubuntu"
os = "Ubuntu"
elseif id == "debian" || id_like == "debian"
os = "Debian"
elseif id == "arch" || id_like == "arch" || id_like == "archlinux"
os = "ArchLinux"
elseif id == "opensuse-tumbleweed"
os = "CentOS"
end
end
elseif Sys.islinux() && Sys.ARCH in [:i386, :i686]
arch = :i386
elseif Sys.islinux() && Sys.ARCH == :arm
id = get_os_release("ID")
if id == "raspbian"
os = "Debian"
end
arch = "armhf"
elseif Sys.islinux() && Sys.ARCH == :aarch64
id = get_os_release("ID")
id_like = get_os_release("ID_LIKE")
if id == "debian" ||
id_like == "debian" ||
id == "archarm" ||
id_like == "arch"
os = "Debian"
end
end
return os, arch
end
"""
try_download(url, file)
Try to download url to file. Return `true` if successful, or `false` otherwise.
"""
function try_download(url, file)
try
Downloads.download(url, file)
true
catch err
rethrow()
false
end
end
"""
check_dependencies(grdir::String)
Check dependencies using ldd on Linux and FreeBSD
"""
function check_dependencies(grdir::String)
try
gksqt = joinpath(grdir, "bin", "gksqt")
res = read(`ldd $gksqt`, String)
if occursin("not found", res)
@warn """
Missing dependencies for GKS QtTerm. Did you install the Qt5 run-time ?
Please refer to https://gr-framework.org/julia.html for further information.
"""
end
catch
# Fail silently
end
end
"""
apple_install(grdir::String)
Register launch services and install rpath for qt5plugin.so
"""
function apple_install(grdir::String)
app = joinpath(grdir, "Applications", "GKSTerm.app")
run(
`/System/Library/Frameworks/CoreServices.framework/Frameworks/LaunchServices.framework/Support/lsregister -f $app`,
)
nothing
end
"""
get_default_install_dir()
Return the default install directory where we have write permissions.
Currently, this is the deps directory of the GR package:
`joinpath(pathof(GR), "..", "deps")`
"""
get_default_install_dir() =
abspath(joinpath(@__DIR__, "..", "deps"))
"""
download_tarball(version, os, arch, downloads_dir = mktempdir())
Download tarball to downloads_dir.
"""
function download_tarball(version, os, arch, downloads_dir = mktempdir())
tarball = "gr-$version-$os-$arch.tar.gz"
file = joinpath(downloads_dir, tarball)
@info("Downloading pre-compiled GR $version $os binary", file)
# Download versioned tarballs from Github
ok = if version != "latest"
try_download(
"https://github.com/sciapp/gr/releases/download/v$version/$tarball",
file,
)
else
false
end
# Download latest tarball from gr-framework.org
if !ok && !try_download("https://gr-framework.org/downloads/$tarball", file)
@warn "Using insecure connection"
try_download("http://gr-framework.org/downloads/$tarball", file) ||
error("Cannot download GR run-time")
end
return file
end
"""
download(install_dir)
Download tarball from https://github.com/sciapp/gr/releases and extract the
tarball into install_dir.
"""
function download(install_dir = get_default_install_dir(); force = false)
# If the install_dir path ends in gr, then install in the parent dir
# Use a trailing slash if you really want the install in gr/gr
basename(install_dir) == "gr" && (install_dir = dirname(install_dir))
# Configure directories
destination_dir = joinpath(install_dir, "gr")
# Ensure the install directory exists
mkpath(install_dir)
grdir = if force
# Download regardless if an existing installation exists
nothing
else
# Check for an existing installation
get_grdir()
end
# We did not find an existing installation
if isnothing(grdir)
# Identify the following so we can download a proper tarball
# 1. version (version of the GR package)
version = get_version()
# 2. os (operating system)
# 3. arch (processor architecture)
os, arch = get_os_and_arch()
# Download the tarball
mktempdir() do downloads_dir
file = download_tarball(version, os, arch, downloads_dir)
# Extract the tarball
if isdir(destination_dir) || force
rm(destination_dir; force=force, recursive=true)
end
mktempdir() do extract_dir
@static if VERSION >= v"1.7"
kwargs = (; set_permissions = !Sys.iswindows())
else
kwargs = (;)
end
Tar.extract(`$(p7zip_jll.p7zip()) x $file -so`, extract_dir; kwargs...)
mv(joinpath(extract_dir, "gr"), destination_dir)
end
rm(file)
end
# Address Mac specific framework and rpath issues
Sys.isapple() && apple_install(destination_dir)
grdir = destination_dir
end # if isnothing(grdir)
# Check dependencies when using Linux or FreeBSD
(Sys.islinux() || Sys.isfreebsd()) && check_dependencies(grdir)
@info "grdir" grdir
return grdir
end # download()
end # module Builder
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 3359 | # Create mutable structs to cache function pointers
macro create_func_ptr_struct(name, syms)
e = :(mutable struct $name end)
for s in eval(syms)
push!(e.args[3].args, :($s::Ptr{Nothing}))
end
push!(e.args[3].args, :(
$name() = new( fill(C_NULL,length($syms))... )
) )
e
end
@create_func_ptr_struct LibGR_Ptrs include("libgr_syms.jl")
@create_func_ptr_struct LibGRM_Ptrs include("libgrm_syms.jl")
@create_func_ptr_struct LibGR3_Ptrs include("libgr3_syms.jl")
const libGR_handle = Ref{Ptr{Nothing}}()
const libGR3_handle = Ref{Ptr{Nothing}}()
const libGRM_handle = Ref{Ptr{Nothing}}()
const libGR_ptrs = LibGR_Ptrs()
const libGRM_ptrs = LibGRM_Ptrs()
const libGR3_ptrs = LibGR3_Ptrs()
const libs_loaded = Ref(false)
@static if Sys.iswindows()
# See AddDllDirectory
const dll_directory_cookies = Ptr{Nothing}[]
end
"""
load_libs(always = false)
Load shared GR libraries from either GR_jll or from GR tarball.
always is a boolean flag that is passed through to init.
"""
function load_libs(always::Bool = false)
libGR_handle[] = Libdl.dlopen(GRPreferences.libGR[])
libGR3_handle[] = Libdl.dlopen(GRPreferences.libGR3[])
libGRM_handle[] = Libdl.dlopen(GRPreferences.libGRM[])
lp = GRPreferences.libpath[]
@static if Sys.iswindows()
try
# Use Win32 lib loader API
# https://learn.microsoft.com/en-us/windows/win32/api/libloaderapi/nf-libloaderapi-adddlldirectory
# To use these paths, use
# LoadLibraryExW with LOAD_LIBRARY_SEARCH_USER_DIRS (0x00000400)
for d in split(lp, ";")
if !isempty(d)
cookie = @ccall "kernel32".AddDllDirectory(push!(transcode(UInt16, String(d)),0x0000)::Ptr{UInt16})::Ptr{Nothing}
if cookie == C_NULL
error("`windows`: Could not run kernel32.AddDllDirectory(\"$d\"). $(Libc.FormatMessage())")
end
push!(dll_directory_cookies, cookie)
end
@debug "`windows`: AddDllDirectory($d)"
end
catch err
@debug "`windows`: Could not use Win32 lib loader API. Using PATH environment variable instead." exception=(err, catch_backtrace())
# Set PATH as a fallback option
ENV["PATH"] = join((lp, get(ENV, "PATH", "")), ';')
@debug "`windows`: set library search path to" ENV["PATH"]
end
elseif Sys.isapple()
# Might not be needed if ENV["GRDIR"] is set
ENV["DYLD_FALLBACK_LIBRARY_PATH"] = join((lp, get(ENV, "DYLD_FALLBACK_LIBRARY_PATH", "")), ':')
@debug "`macOS`: set fallback library search path to" ENV["DYLD_FALLBACK_LIBRARY_PATH"]
end
libs_loaded[] = true
check_env[] = true
init(always)
end
function get_func_ptr(handle::Ref{Ptr{Nothing}}, ptrs::Union{LibGR_Ptrs, LibGRM_Ptrs, LibGR3_Ptrs}, func::Symbol, loaded=libs_loaded[])
loaded || load_libs(true)
s = getfield(ptrs, func)
if s == C_NULL
s = Libdl.dlsym(handle[], func)
setfield!(ptrs, func, s)
end
return getfield(ptrs,func)
end
libGR_ptr(func) = get_func_ptr(libGR_handle, libGR_ptrs, func)
libGRM_ptr(func) = get_func_ptr(libGRM_handle, libGRM_ptrs, func)
libGR3_ptr(func) = get_func_ptr(libGR3_handle, libGR3_ptrs, func)
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 22707 | module GR3
import GR
import Libdl
macro triplet(t)
:( Tuple{$t, $t, $t} )
end
macro ArrayToVector(ctype, data)
return :( convert(Vector{$(esc(ctype))}, vec($(esc(data)))) )
end
mutable struct PNG s::Array{UInt8} end
mutable struct HTML s::AbstractString end
Base.show(io::IO, ::MIME"image/png", x::PNG) = write(io, x.s)
Base.show(io::IO, ::MIME"text/html", x::HTML) = print(io, x.s)
function _readfile(path)
data = Array(UInt8, filesize(path))
s = open(path, "r")
read!(s, data)
end
mutable struct GR3Exception <: Exception
msg::AbstractString
end
Base.showerror(io::IO, e::GR3Exception) = print(io, e.msg);
const msgs = [ "none", "invalid value", "invalid attribute", "init failed",
"OpenGL error", "out of memory", "not initialized",
"camera not initialized", "unknown file extension",
"cannot open file", "export failed" ]
function _check_error()
line = Cint[0]
file = Ptr{UInt8}[0]
error_code = ccall(GR.libGR3_ptr(:gr3_geterror), Int32, (Int32, Ptr{Cint}, Ptr{Ptr{UInt8}}), 1, line, file)
if (error_code != 0)
line = line[1]
file = unsafe_string(file[1])
if 0 <= error_code < length(msgs)
msg = msgs[error_code + 1]
else
msg = "unknown error"
end
message = string("GR3 error (", file, ", l. ", line, "): ", msg)
throw(GR3Exception(message))
end
end
function init(attrib_list)
ccall(GR.libGR3_ptr(:gr3_init), Int32, (Ptr{Int}, ), attrib_list)
_check_error()
end
export init
function free(pointer)
ccall(GR.libGR3_ptr(:gr3_free), Nothing, (Ptr{Nothing}, ), pointer)
_check_error()
end
export free
function terminate()
ccall(GR.libGR3_ptr(:gr3_terminate), Nothing, ())
_check_error()
end
export terminate
function useframebuffer(framebuffer)
ccall(GR.libGR3_ptr(:gr3_useframebuffer), Nothing, (UInt32, ), framebuffer)
_check_error()
end
export useframebuffer
function usecurrentframebuffer()
ccall(GR.libGR3_ptr(:gr3_usecurrentframebuffer), Nothing, ())
_check_error()
end
export usecurrentframebuffer
function getimage(width, height, use_alpha=true)
bpp = use_alpha ? 4 : 3
bitmap = zeros(UInt8, width * height * bpp)
ccall(GR.libGR3_ptr(:gr3_getimage),
Int32,
(Int32, Int32, Int32, Ptr{UInt8}),
width, height, use_alpha, bitmap)
_check_error()
return bitmap
end
export getimage
function save(filename, width, height)
ccall(GR.libGR3_ptr(:gr3_export),
Int32,
(Ptr{Cchar}, Int32, Int32),
filename, width, height)
_check_error()
ext = splitext(filename)[end:end][1]
if ext == ".png"
content = PNG(_readfile(filename))
elseif ext == ".html"
content = HTML("<iframe src=\"files/$filename\" width=$width height=$height></iframe>")
else
content = nothing
end
return content
end
export save
function getrenderpathstring()
val = ccall(GR.libGR3_ptr(:gr3_getrenderpathstring),
Ptr{UInt8}, (), )
_check_error()
unsafe_string(val)
end
export getrenderpathstring
function drawimage(xmin, xmax, ymin, ymax, pixel_width, pixel_height, window)
ccall(GR.libGR3_ptr(:gr3_drawimage),
Int32,
(Float32, Float32, Float32, Float32, Int32, Int32, Int32),
xmin, xmax, ymin, ymax, pixel_width, pixel_height, window)
_check_error()
end
export drawimage
function createmesh(n, vertices, normals, colors)
mesh = Cint[0]
_vertices = [ Float32(x) for x in vertices ]
_normals = [ Float32(x) for x in normals ]
_colors = [ Float32(x) for x in colors ]
ccall(GR.libGR3_ptr(:gr3_createmesh),
Int32,
(Ptr{Cint}, Int32, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}),
mesh, n, @ArrayToVector(Float32, _vertices), @ArrayToVector(Float32, _normals), @ArrayToVector(Float32, _colors))
_check_error()
return mesh[1]
end
export createmesh
function createindexedmesh(num_vertices, vertices, normals, colors, num_indices, indices)
mesh = Cint[0]
_vertices = [ Float32(x) for x in vertices ]
_normals = [ Float32(x) for x in normals ]
_colors = [ Float32(x) for x in colors ]
_indices = [ Float32(x) for x in indices ]
ccall(GR.libGR3_ptr(:gr3_createindexedmesh),
Int32,
(Ptr{Cint}, Int32, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}, Int32, Ptr{Int32}),
mesh, num_vertices, @ArrayToVector(Float32, _vertices), @ArrayToVector(Float32, _normals), @ArrayToVector(Float32, _colors), num_indices, @ArrayToVector(Float32, _indices))
_check_error()
return mesh[1]
end
export createindexedmesh
function drawmesh(mesh::Int32, n, positions::@triplet(Real), directions::@triplet(Real), ups::@triplet(Real), colors::@triplet(Real), scales::@triplet(Real))
_positions = [ Float32(x) for x in positions ]
_directions = [ Float32(x) for x in directions ]
_ups = [ Float32(x) for x in ups ]
_colors = [ Float32(x) for x in colors ]
_scales = [ Float32(x) for x in scales ]
ccall(GR.libGR3_ptr(:gr3_drawmesh),
Nothing,
(Int32, Int32, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}),
mesh, n, @ArrayToVector(Float32, _positions), @ArrayToVector(Float32, _directions), @ArrayToVector(Float32, _ups), @ArrayToVector(Float32, _colors), @ArrayToVector(Float32, _scales))
_check_error()
end
export drawmesh
function createheightmapmesh(heightmap, num_columns, num_rows)
if num_columns * num_rows == length(heightmap)
if ndims(heightmap) == 2
heightmap = reshape(heightmap, num_columns * num_rows)
end
ccall(GR.libGR3_ptr(:gr3_createheightmapmesh),
Nothing,
(Ptr{Float32}, Int32, Int32),
@ArrayToVector(Float32, heightmap), num_columns, num_rows)
_check_error()
else
error("Array has incorrect length or dimension.")
end
end
export createheightmapmesh
function drawheightmap(heightmap, num_columns, num_rows, positions, scales)
if num_columns * num_rows == length(heightmap)
if ndims(heightmap) == 2
heightmap = reshape(heightmap, num_columns * num_rows)
end
_positions = [ Float32(x) for x in positions ]
_scales = [ Float32(x) for x in scales ]
ccall(GR.libGR3_ptr(:gr3_drawheightmap),
Nothing,
(Ptr{Float32}, Int32, Int32, Ptr{Float32}, Ptr{Float32}),
@ArrayToVector(Float32, heightmap), num_columns, num_rows, @ArrayToVector(Float32, _positions), @ArrayToVector(Float32, _scales))
_check_error()
else
error("Array has incorrect length or dimension.")
end
end
export drawheightmap
function deletemesh(mesh)
ccall(GR.libGR3_ptr(:gr3_deletemesh), Nothing, (Int32, ), mesh)
_check_error()
end
export deletemesh
function setquality(quality)
ccall(GR.libGR3_ptr(:gr3_setquality), Nothing, (Int32, ), quality)
_check_error()
end
export setquality
function clear()
ccall(GR.libGR3_ptr(:gr3_clear), Nothing, ())
_check_error()
end
export clear
function cameralookat(camera_x, camera_y, camera_z,
center_x, center_y, center_z,
up_x, up_y, up_z)
ccall(GR.libGR3_ptr(:gr3_cameralookat),
Nothing,
(Float32, Float32, Float32, Float32, Float32, Float32, Float32, Float32, Float32),
camera_x, camera_y, camera_z, center_x, center_y, center_z, up_x, up_y, up_z)
_check_error()
end
export cameralookat
function setcameraprojectionparameters(vertical_field_of_view, zNear, zFar)
ccall(GR.libGR3_ptr(:gr3_setcameraprojectionparameters),
Nothing,
(Float32, Float32, Float32),
vertical_field_of_view, zNear, zFar)
_check_error()
end
export setcameraprojectionparameters
function setlightdirection(x, y, z)
ccall(GR.libGR3_ptr(:gr3_setlightdirection),
Nothing,
(Float32, Float32, Float32),
x, y, z)
_check_error()
end
export setlightdirection
function drawcylindermesh(n, positions, directions, colors, radii, lengths)
_positions = [ Float32(x) for x in positions ]
_directions = [ Float32(x) for x in directions ]
_colors = [ Float32(x) for x in colors ]
_radii = [ Float32(x) for x in radii ]
_lengths = [ Float32(x) for x in lengths ]
ccall(GR.libGR3_ptr(:gr3_drawcylindermesh),
Nothing,
(Int32, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}),
n, @ArrayToVector(Float32, _positions), @ArrayToVector(Float32, _directions), @ArrayToVector(Float32, _colors), @ArrayToVector(Float32, _radii), @ArrayToVector(Float32, _lengths))
_check_error()
end
export drawcylindermesh
function drawconemesh(n, positions, directions, colors, radii, lengths)
_positions = [ Float32(x) for x in positions ]
_directions = [ Float32(x) for x in directions ]
_colors = [ Float32(x) for x in colors ]
_radii = [ Float32(x) for x in radii ]
_lengths = [ Float32(x) for x in lengths ]
ccall(GR.libGR3_ptr(:gr3_drawconemesh),
Nothing,
(Int32, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}),
n, @ArrayToVector(Float32, _positions), @ArrayToVector(Float32, _directions), @ArrayToVector(Float32, _colors), @ArrayToVector(Float32, _radii), @ArrayToVector(Float32, _lengths))
_check_error()
end
export drawconemesh
function drawspheremesh(n, positions, colors, radii)
_positions = [ Float32(x) for x in positions ]
_colors = [ Float32(x) for x in colors ]
_radii = [ Float32(x) for x in radii ]
ccall(GR.libGR3_ptr(:gr3_drawspheremesh),
Nothing,
(Int32, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}),
n, @ArrayToVector(Float32, _positions), @ArrayToVector(Float32, _colors), @ArrayToVector(Float32, _radii))
_check_error()
end
export drawspheremesh
function drawcubemesh(n, positions, directions, ups, colors, scales)
_positions = [ Float32(x) for x in positions ]
_directions = [ Float32(x) for x in directions ]
_ups = [ Float32(x) for x in ups ]
_colors = [ Float32(x) for x in colors ]
_scales = [ Float32(x) for x in scales ]
ccall(GR.libGR3_ptr(:gr3_drawcubemesh),
Nothing,
(Int32, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}),
n, @ArrayToVector(Float32, _positions), @ArrayToVector(Float32, _directions), @ArrayToVector(Float32, _ups), @ArrayToVector(Float32, _colors), @ArrayToVector(Float32, _scales))
_check_error()
end
export drawcubemesh
function setbackgroundcolor(red, green, blue, alpha)
ccall(GR.libGR3_ptr(:gr3_setbackgroundcolor),
Nothing,
(Float32, Float32, Float32, Float32),
red, green, blue, alpha)
_check_error()
end
export setbackgroundcolor
function createisosurfacemesh(grid::Array{UInt16,3}, step::@triplet(Float64), offset::@triplet(Float64), isolevel::Int64)
mesh = Cint[0]
dim_x, dim_y, dim_z = size(grid)
data = reshape(grid, dim_x * dim_y * dim_z)
stride_x, stride_y, stride_z = strides(grid)
step_x, step_y, step_z = [ float(x) for x in step ]
offset_x, offset_y, offset_z = [ float(x) for x in offset ]
err = ccall(GR.libGR3_ptr(:gr3_createisosurfacemesh),
Int32,
(Ptr{Cint}, Ptr{UInt16}, UInt16, UInt32, UInt32, UInt32, UInt32, UInt32, UInt32, Float64, Float64, Float64, Float64, Float64, Float64),
mesh, @ArrayToVector(UInt16, data), UInt16(isolevel), dim_x, dim_y, dim_z, stride_x, stride_y, stride_z, step_x, step_y, step_z, offset_x, offset_y, offset_z)
_check_error()
return mesh[1]
end
export createisosurfacemesh
function surface(px, py, pz, option::Int)
nx = length(px)
ny = length(py)
nz = length(pz)
if ndims(pz) == 1
out_of_bounds = nz != nx * ny
elseif ndims(px) == ndims(py) == ndims(pz) == 2
nx, ny = size(pz)
out_of_bounds = size(px)[1] != ny || size(px)[2] != nx ||
size(py)[1] != ny || size(py)[2] != nx
elseif ndims(pz) == 2
out_of_bounds = size(pz)[1] != nx || size(pz)[2] != ny
else
out_of_bounds = true
end
if !out_of_bounds
if option != GR.OPTION_3D_MESH
if ndims(pz) == 2
pz = reshape(pz, nx * ny)
end
_px = [ Float32(x) for x in px ]
_py = [ Float32(y) for y in py ]
_pz = [ Float32(z) for z in pz ]
else
_px = px'
_py = py'
_pz = pz
end
ccall(GR.libGR3_ptr(:gr3_surface),
Nothing,
(Int32, Int32, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}, Int32),
nx, ny, @ArrayToVector(Float32, _px), @ArrayToVector(Float32, _py), @ArrayToVector(Float32, _pz), option)
_check_error()
else
error("Arrays have incorrect length or dimension.")
end
end
function setalphamode(mode)
ccall(GR.libGR3_ptr(:gr3_setalphamode),
Nothing,
(Int32, ),
mode)
_check_error()
end
function getalphamode(mode)
mode = Cint[0]
ccall(GR.libGR3_ptr(:gr3_getalphamode),
Cint,
(Ptr{Cint}, ),
mode)
_check_error()
return mode
end
function setlightparameters(ambient, diffuse, specular, specular_power)
ccall(GR.libGR3_ptr(:gr3_setlightparameters),
Nothing,
(Float32, Float32, Float32, Float32),
ambient, diffuse, specular, specular_power)
_check_error()
end
function getlightparameters()
ambient = Cfloat[1]
diffuse = Cfloat[1]
specular = Cfloat[1]
specular_power = Cfloat[1]
ccall(GR.libGR3_ptr(:gr3_getlightparameters),
Nothing,
(Ptr{Float32}, Ptr{Float32}, Ptr{Float32}, Ptr{Float32}),
ambient, diffuse, specular, specular_power)
_check_error()
return ambient[1], diffuse[1], specular[1], specular_power[1]
end
function isosurface(data::Array{Float64,3}, iso::Float64, color)
_data = [ Float32(value) for value in data ]
nx, ny, nz = size(data)
_color = [ Float32(value) for value in color ]
ccall(GR.libGR3_ptr(:gr3_isosurface),
Nothing,
(Int32, Int32, Int32, Ptr{Float32}, Float32, Ptr{Float32}, Ptr{Int32}),
nx, ny, nz, @ArrayToVector(Float32, _data), Float32(iso), @ArrayToVector(Float32, _color), C_NULL)
_check_error()
end
function volume(data::Array{Float64,3}, algorithm::Int64)
dmin = Cdouble[-1]
dmax = Cdouble[-1]
nx, ny, nz = size(data)
data = reshape(data, nx * ny * nz)
ccall(GR.libGR3_ptr(:gr_volume),
Nothing,
(Cint, Cint, Cint, Ptr{Cdouble}, Cint, Ptr{Cdouble}, Ptr{Cdouble}),
nx, ny, nz, data, algorithm, dmin, dmax)
return dmin[1], dmax[1]
end
function createslicemeshes(grid; x::Union{Real, Nothing}=nothing, y::Union{Real, Nothing}=nothing, z::Union{Real, Nothing}=nothing, step::Union{Tuple{Real, Real, Real}, Nothing}=nothing, offset::Union{Tuple{Real, Real, Real}, Nothing}=nothing)
if x === nothing && y === nothing && z === nothing
x = 0.5
y = 0.5
z = 0.5
end
if typeof(grid[1,1,1]) <: Unsigned
input_max = typemax(typeof(grid[1,1,1]))
elseif typeof(grid[1,1,1]) <: Real
input_max = convert(typeof(grid[1,1,1]), 1.0)
grid = min.(grid, input_max)
else
error("grid must be three dimensional array of Real numbers")
return(nothing)
end
scaling_factor = typemax(UInt16) / input_max
grid = Array{UInt16, 3}(floor.(grid * scaling_factor))
nx, ny, nz = size(grid)
if step === nothing && offset === nothing
step = (2.0/(nx-1), 2.0/(ny-1), 2.0/(nz-1))
offset = (-1.0, -1.0, -1.0)
elseif offset === nothing
offset = (-step[1] * (nx-1) / 2.0,
-step[2] * (ny-1) / 2.0,
-step[3] * (nz-1) / 2.0)
elseif step === nothing
step = (-offset[1] * 2.0 / (nx-1),
-offset[2] * 2.0 / (ny-1),
-offset[3] * 2.0 / (nz-1))
end
stride_x, stride_y, stride_z = 1, nx, nx*ny
dim_x, dim_y, dim_z = convert(Tuple{UInt32, UInt32, UInt32}, size(grid))
step_x, step_y, step_z = convert(Tuple{Float64, Float64, Float64}, step)
offset_x, offset_y, offset_z = convert(Tuple{Float64, Float64, Float64}, offset)
if x !== nothing
x = convert(UInt32, floor(clamp(x, 0, 1) * nx))
mesh = Cint[0]
ccall(GR.libGR3_ptr(:gr3_createxslicemesh),
Nothing,
(Ptr{UInt32}, Ptr{UInt16}, UInt32,
UInt32, UInt32, UInt32,
UInt32, UInt32, UInt32,
Float64, Float64, Float64,
Float64, Float64, Float64),
mesh, grid, x,
dim_x, dim_y, dim_z,
stride_x, stride_y, stride_z,
step_x, step_y, step_z,
offset_x, offset_y, offset_z)
_check_error()
_mesh_x = mesh[1]
else
_mesh_x = nothing
end
if y !== nothing
y = convert(UInt32, floor(clamp(y, 0, 1) * ny))
mesh = Cint[0]
ccall(GR.libGR3_ptr(:gr3_createyslicemesh),
Nothing,
(Ptr{UInt32}, Ptr{UInt16}, UInt32,
UInt32, UInt32, UInt32,
UInt32, UInt32, UInt32,
Float64, Float64, Float64,
Float64, Float64, Float64),
mesh, grid, y,
dim_x, dim_y, dim_z,
stride_x, stride_y, stride_z,
step_x, step_y, step_z,
offset_x, offset_y, offset_z)
_check_error()
_mesh_y = mesh[1]
else
_mesh_y = nothing
end
if z !== nothing
z = convert(UInt32, floor(clamp(z, 0, 1) * nz))
mesh = Cint[0]
ccall(GR.libGR3_ptr(:gr3_createzslicemesh),
Nothing,
(Ptr{UInt32}, Ptr{UInt16}, UInt32,
UInt32, UInt32, UInt32,
UInt32, UInt32, UInt32,
Float64, Float64, Float64,
Float64, Float64, Float64),
mesh, grid, z,
dim_x, dim_y, dim_z,
stride_x, stride_y, stride_z,
step_x, step_y, step_z,
offset_x, offset_y, offset_z)
_check_error()
_mesh_z = mesh[1]
else
_mesh_z = nothing
end
return(_mesh_x, _mesh_y, _mesh_z)
end
export createslicemeshes
function createxslicemesh(grid, x::Real=0.5; step::Union{Tuple{Real, Real, Real}, Nothing}=nothing, offset::Union{Tuple{Real, Real, Real}, Nothing}=nothing)
return createslicemeshes(grid, x=x, step=step, offset=offset)[1]
end
export createxslicemesh
function createyslicemesh(grid, y::Real=0.5; step::Union{Tuple{Real, Real, Real}, Nothing}=nothing, offset::Union{Tuple{Real, Real, Real}, Nothing}=nothing)
return createslicemeshes(grid, y=y, step=step, offset=offset)[2]
end
export createyslicemesh
function createzslicemesh(grid, z::Real=0.5; step::Union{Tuple{Real, Real, Real}, Nothing}=nothing, offset::Union{Tuple{Real, Real, Real}, Nothing}=nothing)
return createslicemeshes(grid, z=z, step=step, offset=offset)[3]
end
export createzslicemesh
function drawxslicemesh(grid, x::Real=0.5; step::Union{Tuple{Real, Real, Real}, Nothing}=nothing, offset::Union{Tuple{Real, Real, Real}, Nothing}=nothing, position::Tuple{Real, Real, Real}=(0, 0, 0), direction::Tuple{Real, Real, Real}=(0, 0, 1), up::Tuple{Real, Real, Real}=(0, 1, 0), color::Tuple{Real, Real, Real}=(1, 1, 1), scale::Tuple{Real, Real, Real}=(1, 1, 1))
mesh = createxslicemesh(grid, x, step=step, offset=offset)
drawmesh(mesh, 1, position, direction, up, color, scale)
deletemesh(mesh)
end
export drawxslicemesh
function drawyslicemesh(grid, y::Real=0.5; step::Union{Tuple{Real, Real, Real}, Nothing}=nothing, offset::Union{Tuple{Real, Real, Real}, Nothing}=nothing, position::Tuple{Real, Real, Real}=(0, 0, 0), direction::Tuple{Real, Real, Real}=(0, 0, 1), up::Tuple{Real, Real, Real}=(0, 1, 0), color::Tuple{Real, Real, Real}=(1, 1, 1), scale::Tuple{Real, Real, Real}=(1, 1, 1))
mesh = createxslicemesh(grid, y, step=step, offset=offset)
drawmesh(mesh, 1, position, direction, up, color, scale)
deletemesh(mesh)
end
export drawyslicemesh
function drawzslicemesh(grid, z::Real=0.5; step::Union{Tuple{Real, Real, Real}, Nothing}=nothing, offset::Union{Tuple{Real, Real, Real}, Nothing}=nothing, position::Tuple{Real, Real, Real}=(0, 0, 0), direction::Tuple{Real, Real, Real}=(0, 0, 1), up::Tuple{Real, Real, Real}=(0, 1, 0), color::Tuple{Real, Real, Real}=(1, 1, 1), scale::Tuple{Real, Real, Real}=(1, 1, 1))
mesh = createxslicemesh(grid, z, step=step, offset=offset)
drawmesh(mesh, 1, position, direction, up, color, scale)
deletemesh(mesh)
end
export drawzslicemesh
function drawslicemeshes(data; x::Union{Real, Nothing}=nothing, y::Union{Real, Nothing}=nothing, z::Union{Real, Nothing}=nothing, step::Union{Tuple{Real, Real, Real}, Nothing}=nothing, offset::Union{Tuple{Real, Real, Real}, Nothing}=nothing, position::Tuple{Real, Real, Real}=(0, 0, 0), direction::Tuple{Real, Real, Real}=(0, 0, 1), up::Tuple{Real, Real, Real}=(0, 1, 0), color::Tuple{Real, Real, Real}=(1, 1, 1), scale::Tuple{Real, Real, Real}=(1, 1, 1))
meshes = createslicemeshes(data, x=x, y=y, z=z, step=step, offset=offset)
for mesh in meshes
if mesh !== nothing
drawmesh(mesh, 1, position, direction, up, color, scale)
deletemesh(mesh)
end
end
end
export drawslicemeshes
function setlightsources(num_lights, directions, colors)
@assert length(directions) == length(colors)
_directions = [ Float32(x) for x in directions ]
_colors = [ Float32(x) for x in colors ]
ccall(GR.libGR3_ptr(:gr3_setlightsources),
Nothing,
(Int32, Ptr{Float32}, Ptr{Float32}),
num_lights, @ArrayToVector(Float32, _directions), @ArrayToVector(Float32, _colors))
end
export setlightsources
function getlightsources(num_lights)
directions = Vector{Cfloat}(undef, num_lights * 3)
colors = Vector{Cfloat}(undef, num_lights * 3)
ccall(GR.libGR3_ptr(:gr3_getlightsources),
Nothing,
(Int32, Ptr{Cfloat}, Ptr{Cfloat}),
num_lights, directions, colors)
return directions, colors
end
export getlightsources
const IA_END_OF_LIST = 0
const IA_FRAMEBUFFER_WIDTH = 1
const IA_FRAMEBUFFER_HEIGHT = 2
const ERROR_NONE = 0
const ERROR_INVALID_VALUE = 1
const ERROR_INVALID_ATTRIBUTE = 2
const ERROR_INIT_FAILED = 3
const ERROR_OPENGL_ERR = 4
const ERROR_OUT_OF_MEM = 5
const ERROR_NOT_INITIALIZED = 6
const ERROR_CAMERA_NOT_INITIALIZED = 7
const ERROR_UNKNOWN_FILE_EXTENSION = 8
const ERROR_CANNOT_OPEN_FILE = 9
const ERROR_EXPORT = 10
const QUALITY_OPENGL_NO_SSAA = 0
const QUALITY_OPENGL_2X_SSAA = 2
const QUALITY_OPENGL_4X_SSAA = 4
const QUALITY_OPENGL_8X_SSAA = 8
const QUALITY_OPENGL_16X_SSAA = 16
const QUALITY_POVRAY_NO_SSAA = 0+1
const QUALITY_POVRAY_2X_SSAA = 2+1
const QUALITY_POVRAY_4X_SSAA = 4+1
const QUALITY_POVRAY_8X_SSAA = 8+1
const QUALITY_POVRAY_16X_SSAA = 16+1
const DRAWABLE_OPENGL = 1
const DRAWABLE_GKS = 2
const SURFACE_DEFAULT = 0
const SURFACE_NORMALS = 1
const SURFACE_FLAT = 2
const SURFACE_GRTRANSFORM = 4
const SURFACE_GRCOLOR = 8
const SURFACE_GRZSHADED = 16
end # module
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 89496 | module jlgr
import GR
# These methods are extended in jlgr
import GR: hexbin, contour, contourf, surface
using Serialization
using Sockets
export
colormap,
figure,
kvs,
gcf,
hold,
usecolorscheme,
subplot,
plot,
oplot,
stairs,
scatter,
stem,
barplot,
histogram,
polarhistogram,
contour,
contourf,
hexbin,
heatmap,
polarheatmap,
wireframe,
surface,
volume,
plot3,
scatter3,
title,
redraw,
xlabel,
ylabel,
drawgrid,
xticks,
yticks,
zticks,
xticklabels,
yticklabels,
legend,
xlim,
ylim,
savefig,
meshgrid,
meshgrid,
peaks,
imshow,
isosurface,
cart2sph,
sph2cart,
polar,
trisurf,
tricont,
shade,
setpanzoom,
mainloop
signif(x, digits; base = 10) = round(x, sigdigits = digits, base = base)
const PlotArg = Union{AbstractString, AbstractVector, AbstractMatrix, Function}
const kw_args = [:accelerate, :algorithm, :alpha, :backgroundcolor, :barwidth, :baseline, :clabels, :clines, :color, :colormap, :figsize, :font, :isovalue, :labels, :levels, :location, :nbins, :rotation, :size, :tilt, :title, :where, :xflip, :xform, :xlabel, :xlim, :xlog, :yflip, :ylabel, :ylim, :ylog, :zflip, :zlabel, :zlim, :zlog, :clim, :subplot, :linewidth, :grid, :scale, :theta_direction, :theta_zero_location, :dpi, :keepaspect]
const grm_aliases = Dict(
:nbins => :num_bins, :xform => :transform, :xlim => :x_lim, :ylim => :y_lim, :zlim => :z_lim, :clim => :c_lim, :xlabel => :x_label, :ylabel => :y_label, :zlabel => :z_label, :xflip => :x_flip, :yflip => :y_flip, :zflip => :z_flip, :xlog => :x_log, :ylog => :y_log, :zlog => :z_log)
const colors = [
[0xffffff, 0x000000, 0xff0000, 0x00ff00, 0x0000ff, 0x00ffff, 0xffff00, 0xff00ff] [0x282c34, 0xd7dae0, 0xcb4e42, 0x99c27c, 0x85a9fc, 0x5ab6c1, 0xd09a6a, 0xc57bdb] [0xfdf6e3, 0x657b83, 0xdc322f, 0x859900, 0x268bd2, 0x2aa198, 0xb58900, 0xd33682] [0x002b36, 0x839496, 0xdc322f, 0x859900, 0x268bd2, 0x2aa198, 0xb58900, 0xd33682]
]
const fonts = Dict(
"Times_Roman" => 101, "Times_Italic" => 102, "Times_Bold" => 103, "Times_BoldItalic" => 104,
"Helvetica_Regular" => 105, "Helvetica_Oblique" => 106, "Helvetica_Bold" => 107, "Helvetica_BoldOblique" => 108,
"Courier_Regular" => 109, "Courier_Oblique" => 110, "Courier_Bold" => 111, "Courier_BoldOblique" => 112,
"Symbol" => 113,
"Bookman_Light" => 114, "Bookman_LightItalic" => 115, "Bookman_Demi" => 116, "Bookman_DemiItalic" => 117,
"NewCenturySchlbk_Roman" => 118, "NewCenturySchlbk_Italic" => 119, "NewCenturySchlbk_Bold" => 120, "NewCenturySchlbk_BoldItalic" => 121,
"AvantGarde_Book" => 122, "AvantGarde_BookOblique" => 123, "AvantGarde_Demi" => 124, "AvantGarde_DemiOblique" => 125,
"Palatino_Roman" => 126, "Palatino_Italic" => 127, "Palatino_Bold" => 128, "Palatino_BoldItalic" => 129,
"ZapfChancery_MediumItalic" => 130,
"ZapfDingbats" => 131,
"CMUSerif-Math" => 232,
"DejaVuSans" => 233,
"STIXTwoMath" => 234)
const distinct_cmap = (0, 1, 984, 987, 989, 983, 994, 988)
const theta_zero_location = Dict(
"E" => 0, "N" => π/2, "W" => π, "S" => 1.5π)
const default_kvs = Dict{Symbol, Any}(
:ax => false,
:subplot => [0, 1, 0, 1],
:clear => true,
:update => true,
:panzoom => nothing)
function linspace(start, stop, length)
range(start, stop=stop, length=length)
end
function _min(a)
minimum(filter(!isnan, a))
end
function _max(a)
maximum(filter(!isnan, a))
end
mutable struct PlotObject
obj::Dict{Symbol, Any}
args::Vector{NTuple{5, Any}}
kvs::Dict{Symbol, Any}
end
function Figure(width=600, height=450, dpi=nothing)
obj = Dict{Symbol, Any}()
args = []
kvs = copy(default_kvs)
kvs[:size] = (width, height)
kvs[:dpi] = dpi
PlotObject(obj, args, kvs)
end
const plt = Ref{PlotObject}(Figure())
const ctx = Dict{Symbol, Any}()
const scheme = Ref(0)
const handle = Ref{Ptr{Nothing}}(C_NULL)
function kvs()
plt[].kvs
end
function gcf()
obj = deepcopy(plt[].obj)
args = deepcopy(plt[].args)
kvs = deepcopy(plt[].kvs)
PlotObject(obj, args, kvs)
end
function plot(p::PlotObject)
plt[] = p
plot_data()
end
isrowvec(x::AbstractArray) = ndims(x) == 2 && size(x, 1) == 1 && size(x, 2) > 1
isvector(x::AbstractVector) = true
isvector(x::AbstractMatrix) = size(x, 1) == 1
function set_viewport(kind, subplot, plt=plt[])
mwidth, mheight, width, height = GR.inqdspsize()
if haskey(plt.kvs, :figsize)
w, h = plt.kvs[:figsize]
if w < 2 && h < 2
# size values < 2 are interpreted as metric values
w = width * w / mwidth
h = height * h / mheight
end
else
if haskey(plt.kvs, :dpi)
dpi = plt.kvs[:dpi]
end
if (dpi === nothing)
dpi = round(width / mwidth * 0.0254, RoundNearestTiesUp)
end
if dpi > 200
w, h = plt.kvs[:size] .* (dpi / 100)
else
w, h = plt.kvs[:size]
end
end
viewport = zeros(4)
vp = copy(float(subplot))
if w > h
ratio = w / h
msize = mwidth * w / width
GR.setwsviewport(0, msize, 0, msize / ratio)
GR.setwswindow(0, 1, 0, 1 / ratio)
vp[3] /= ratio
vp[4] /= ratio
else
ratio = h / w
msize = mheight * h / height
GR.setwsviewport(0, msize / ratio, 0, msize)
GR.setwswindow(0, 1 / ratio, 0, 1)
vp[1] /= ratio
vp[2] /= ratio
end
if kind === :wireframe || kind === :surface || kind === :plot3 || kind === :scatter3 || kind === :trisurf || kind === :volume
extent = min(vp[2] - vp[1], vp[4] - vp[3])
vp1 = 0.5 * (vp[1] + vp[2] - extent)
vp2 = 0.5 * (vp[1] + vp[2] + extent)
vp3 = 0.5 * (vp[3] + vp[4] - extent)
vp4 = 0.5 * (vp[3] + vp[4] + extent)
else
vp1, vp2, vp3, vp4 = vp
end
left_margin = haskey(plt.kvs, :ylabel) ? 0.05 : 0
if kind === :contour || kind === :contourf || kind === :tricont || kind === :hexbin || kind === :heatmap || kind === :nonuniformheatmap || kind === :polarheatmap || kind === :nonuniformpolarheatmap || kind === :surface || kind === :trisurf || kind === :volume
right_margin = (vp2 - vp1) * 0.1
else
right_margin = 0
end
bottom_margin = haskey(plt.kvs, :xlabel) ? 0.05 : 0
top_margin = haskey(plt.kvs, :title) ? 0.075 : 0
viewport[1] = vp1 + (0.075 + left_margin) * (vp2 - vp1)
viewport[2] = vp1 + (0.95 - right_margin) * (vp2 - vp1)
viewport[3] = vp3 + (0.075 + bottom_margin) * (vp4 - vp3)
viewport[4] = vp3 + (0.975 - top_margin) * (vp4 - vp3)
if (kind === :line || kind === :stairs || kind === :scatter || kind === :stem) && haskey(plt.kvs, :labels)
location = get(plt.kvs, :location, 1)
if location == 11 || location == 12 || location == 13
w, h = legend_size()
viewport[2] -= w + 0.1
end
end
if kind === :polar || kind === :polarhist || kind === :polarheatmap || kind === :nonuniformpolarheatmap
xmin, xmax, ymin, ymax = viewport
xcenter = 0.5 * (xmin + xmax)
ycenter = 0.5 * (ymin + ymax)
r = 0.45 * min(xmax - xmin, ymax - ymin)
if haskey(plt.kvs, :title)
r *= 0.975
ycenter -= 0.025 * r
end
viewport[1] = xcenter - r;
viewport[2] = xcenter + r;
viewport[3] = ycenter - r;
viewport[4] = ycenter + r;
end
GR.setviewport(viewport[1], viewport[2], viewport[3], viewport[4])
plt.kvs[:viewport] = viewport
plt.kvs[:vp] = vp
plt.kvs[:ratio] = ratio
if haskey(plt.kvs, :backgroundcolor)
GR.savestate()
GR.selntran(0)
GR.setfillintstyle(GR.INTSTYLE_SOLID)
GR.setfillcolorind(plt.kvs[:backgroundcolor])
if w > h
GR.fillrect(subplot[1], subplot[2],
ratio * subplot[3], ratio * subplot[4])
else
GR.fillrect(ratio * subplot[1], ratio * subplot[2],
subplot[3], subplot[4])
end
GR.selntran(1)
GR.restorestate()
end
end
function fix_minmax(a, b)
if a == b
a -= a != 0 ? 0.1 * a : 0.1
b += b != 0 ? 0.1 * b : 0.1
end
a, b
end
function Extrema64(a)
amin = typemax(Float64)
amax = typemin(Float64)
for el in a
if !isnan(el)
if el < amin
amin = el
end
if el > amax
amax = el
end
end
end
amin, amax
end
function minmax(kind, plt=plt[])
xmin = ymin = zmin = cmin = typemax(Float64)
xmax = ymax = zmax = cmax = -typemax(Float64)
scale = plt.kvs[:scale]
for (x, y, z, c, spec) in plt.args
if x !== nothing
if scale & GR.OPTION_X_LOG != 0
x = map(v -> v>0 ? v : NaN, x)
end
x0, x1 = Extrema64(x)
xmin = min(x0, xmin)
xmax = max(x1, xmax)
elseif kind === :volume || kind === :isosurface
xmin, xmax = -1, 1
else
xmin, xmax = 0, 1
end
if y !== nothing
if scale & GR.OPTION_Y_LOG != 0
y = map(v -> v>0 ? v : NaN, y)
end
y0, y1 = Extrema64(y)
ymin = min(y0, ymin)
ymax = max(y1, ymax)
elseif kind === :volume || kind === :isosurface
ymin, ymax = -1, 1
else
ymin, ymax = 0, 1
end
if z !== nothing
if scale & GR.OPTION_Z_LOG != 0
z = map(v -> v>0 ? v : NaN, z)
end
z0, z1 = Extrema64(z)
zmin = min(z0, zmin)
zmax = max(z1, zmax)
elseif kind === :volume || kind === :isosurface
zmin, zmax = -1, 1
else
zmin, zmax = 0, 1
end
if c !== nothing
c0, c1 = Extrema64(c)
cmin = min(c0, cmin)
cmax = max(c1, cmax)
elseif z !== nothing
c0, c1 = Extrema64(z)
cmin = min(c0, cmin)
cmax = max(c1, cmax)
end
end
xmin, xmax = fix_minmax(xmin, xmax)
ymin, ymax = fix_minmax(ymin, ymax)
zmin, zmax = fix_minmax(zmin, zmax)
if haskey(plt.kvs, :xlim)
x0, x1 = plt.kvs[:xlim]
if x0 === nothing x0 = xmin end
if x1 === nothing x1 = xmax end
plt.kvs[:xrange] = (x0, x1)
else
plt.kvs[:xrange] = xmin, xmax
end
if haskey(plt.kvs, :ylim)
y0, y1 = plt.kvs[:ylim]
if y0 === nothing y0 = ymin end
if y1 === nothing y1 = ymax end
plt.kvs[:yrange] = (y0, y1)
else
plt.kvs[:yrange] = ymin, ymax
end
if haskey(plt.kvs, :zlim)
z0, z1 = plt.kvs[:zlim]
if z0 === nothing z0 = zmin end
if z1 === nothing z1 = zmax end
plt.kvs[:zrange] = (z0, z1)
else
plt.kvs[:zrange] = zmin, zmax
end
if haskey(plt.kvs, :clim)
c0, c1 = plt.kvs[:clim]
if c0 === nothing c0 = cmin end
if c1 === nothing c1 = cmax end
plt.kvs[:crange] = (c0, c1)
else
plt.kvs[:crange] = cmin, cmax
end
end
function to_wc(wn)
xmin, ymin = GR.ndctowc(wn[1], wn[3])
xmax, ymax = GR.ndctowc(wn[2], wn[4])
xmin, xmax, ymin, ymax
end
fract(x) = modf(x)[1]
function auto_tick(amin, amax)
scale = 10.0 ^ trunc(log10(amax - amin))
tick_size = (5.0, 2.0, 1.0, 0.5, 0.2, 0.1, 0.05, 0.02, 0.01)
tick = 1.0
for i in 1:length(tick_size)
n = trunc((amax - amin) / scale / tick_size[i])
if n > 7
tick = tick_size[i - 1]
break
end
end
tick * scale
end
function set_window(kind, plt=plt[])
if !(kind === :polar || kind === :polarhist || kind === :polarheatmap || kind === :nonuniformpolarheatmap)
scale = get(plt.kvs, :scale, 0)
get(plt.kvs, :xlog, false) && (scale |= GR.OPTION_X_LOG)
get(plt.kvs, :ylog, false) && (scale |= GR.OPTION_Y_LOG)
get(plt.kvs, :zlog, false) && (scale |= GR.OPTION_Z_LOG)
get(plt.kvs, :xflip, false) && (scale |= GR.OPTION_FLIP_X)
get(plt.kvs, :yflip, false) && (scale |= GR.OPTION_FLIP_Y)
get(plt.kvs, :zflip, false) && (scale |= GR.OPTION_FLIP_Z)
else
scale = 0
end
plt.kvs[:scale] = scale
if plt.kvs[:panzoom] !== nothing
xmin, xmax, ymin, ymax = GR.panzoom(plt.kvs[:panzoom]...)
plt.kvs[:xrange] = (xmin, xmax)
plt.kvs[:yrange] = (ymin, ymax)
else
minmax(kind)
end
if kind === :wireframe || kind === :surface || kind === :plot3 || kind === :scatter3 || kind === :polar || kind === :polarhist || kind === :polarheatmap || kind === :nonuniformpolarheatmap || kind === :trisurf || kind === :volume
major_count = 2
else
major_count = 5
end
xmin, xmax = plt.kvs[:xrange]
if kind === :heatmap && !haskey(plt.kvs, :xlim)
xmin -= 0.5
xmax += 0.5
end
if scale & GR.OPTION_X_LOG == 0
if !haskey(plt.kvs, :xlim) && plt.kvs[:panzoom] === nothing && !(kind === :heatmap || kind === :polarheatmap || kind === :nonuniformpolarheatmap)
xmin, xmax = GR.adjustlimits(xmin, xmax)
end
if haskey(plt.kvs, :xticks)
xtick, majorx = plt.kvs[:xticks]
else
majorx = major_count
xtick = auto_tick(xmin, xmax) / major_count
end
else
xtick = majorx = 1
end
if scale & GR.OPTION_FLIP_X == 0
xorg = (xmin, xmax)
else
xorg = (xmax, xmin)
end
plt.kvs[:xaxis] = xtick, xorg, majorx
ymin, ymax = plt.kvs[:yrange]
if kind === :heatmap && !haskey(plt.kvs, :ylim)
ymin -= 0.5
ymax += 0.5
end
if kind === :hist && !haskey(plt.kvs, :ylim)
ymin = scale & GR.OPTION_Y_LOG == 0 ? 0 : 1
end
if scale & GR.OPTION_Y_LOG == 0
if !haskey(plt.kvs, :ylim) && plt.kvs[:panzoom] === nothing && !(kind === :heatmap || kind === :polarheatmap || kind === :nonuniformpolarheatmap)
ymin, ymax = GR.adjustlimits(ymin, ymax)
end
if haskey(plt.kvs, :yticks)
ytick, majory = plt.kvs[:yticks]
else
majory = major_count
ytick = auto_tick(ymin, ymax) / major_count
end
else
ytick = majory = 1
end
if scale & GR.OPTION_FLIP_Y == 0
yorg = (ymin, ymax)
else
yorg = (ymax, ymin)
end
plt.kvs[:yaxis] = ytick, yorg, majory
if kind === :wireframe || kind === :surface || kind === :plot3 || kind === :scatter3 || kind === :trisurf || kind === :volume
zmin, zmax = plt.kvs[:zrange]
if scale & GR.OPTION_Z_LOG == 0
if !haskey(plt.kvs, :zlim)
zmin, zmax = GR.adjustlimits(zmin, zmax)
end
if haskey(plt.kvs, :zticks)
ztick, majorz = plt.kvs[:zticks]
else
majorz = major_count
ztick = auto_tick(zmin, zmax) / major_count
end
else
ztick = majorz = 1
end
if scale & GR.OPTION_FLIP_Z == 0
zorg = (zmin, zmax)
else
zorg = (zmax, zmin)
end
plt.kvs[:zaxis] = ztick, zorg, majorz
end
plt.kvs[:window] = xmin, xmax, ymin, ymax
if !(kind === :polar || kind === :polarhist || kind === :polarheatmap || kind === :nonuniformpolarheatmap)
GR.setwindow(xmin, xmax, ymin, ymax)
else
GR.setwindow(-1, 1, -1, 1)
end
if kind === :wireframe || kind === :surface || kind === :plot3 || kind === :scatter3 || kind === :trisurf || kind === :volume
rotation = get(plt.kvs, :rotation, 40)
tilt = get(plt.kvs, :tilt, 60)
GR.setwindow3d(xmin, xmax, ymin, ymax, zmin, zmax)
GR.setspace3d(-rotation, tilt, 30, 0)
end
plt.kvs[:scale] = scale
GR.setscale(scale)
end
function ticklabel_fun(f::Function)
return (x, y, svalue, value) -> GR.textext(x, y, string(f(value)))
end
function ticklabel_fun(labels::AbstractVecOrMat{T}) where T <: AbstractString
(x, y, svalue, value) -> begin
pos = findfirst(t->(value≈t), collect(1:length(labels)))
lab = (pos === nothing) ? "" : labels[pos]
GR.textext(x, y, lab)
end
end
function draw_axes(kind, pass=1, plt=plt[])
viewport = plt.kvs[:viewport]
vp = plt.kvs[:vp]
xtick, xorg, majorx = plt.kvs[:xaxis]
ytick, yorg, majory = plt.kvs[:yaxis]
drawgrid = get(plt.kvs, :grid, true)
# enforce scientific notation for logarithmic axes labels
if plt.kvs[:scale] & GR.OPTION_X_LOG != 0
xtick = 10
end
if plt.kvs[:scale] & GR.OPTION_Y_LOG != 0
ytick = 10
end
GR.setlinecolorind(1)
diag = sqrt((viewport[2] - viewport[1])^2 + (viewport[4] - viewport[3])^2)
GR.setlinewidth(1)
ticksize = 0.0075 * diag
if kind === :wireframe || kind === :surface || kind === :plot3 || kind === :scatter3 || kind === :trisurf || kind === :volume
charheight = max(0.024 * diag, 0.012)
ztick, zorg, majorz = plt.kvs[:zaxis]
rotation = get(plt.kvs, :rotation, 40)
tilt = get(plt.kvs, :tilt, 60)
zi = 0 <= tilt <= 90 ? 1 : 2
xlabel = get(plt.kvs, :xlabel, "")
ylabel = get(plt.kvs, :ylabel, "")
zlabel = get(plt.kvs, :zlabel, "")
GR.setcharheight(charheight * 1.5)
GR.settitles3d(xlabel, ylabel, zlabel)
GR.setcharheight(charheight)
if pass == 1 && drawgrid
if 0 <= rotation < 90
GR.grid3d(xtick, 0, ztick, xorg[1], yorg[2], zorg[zi], 2, 0, 2)
GR.grid3d(0, ytick, 0, xorg[1], yorg[2], zorg[zi], 0, 2, 0)
elseif 90 <= rotation < 180
GR.grid3d(xtick, 0, ztick, xorg[2], yorg[2], zorg[zi], 2, 0, 2)
GR.grid3d(0, ytick, 0, xorg[2], yorg[2], zorg[zi], 0, 2, 0)
elseif 180 <= rotation < 270
GR.grid3d(xtick, 0, ztick, xorg[2], yorg[1], zorg[zi], 2, 0, 2)
GR.grid3d(0, ytick, 0, xorg[2], yorg[1], zorg[zi], 0, 2, 0)
else
GR.grid3d(xtick, 0, ztick, xorg[1], yorg[1], zorg[1], 2, 0, 2)
GR.grid3d(0, ytick, 0, xorg[1], yorg[1], zorg[zi], 0, 2, 0)
end
else
if 0 <= rotation < 90
GR.axes3d(xtick, 0, ztick, xorg[1], yorg[1], zorg[zi], majorx, 0, majorz, -ticksize)
GR.axes3d(0, ytick, 0, xorg[2], yorg[1], zorg[zi], 0, majory, 0, ticksize)
elseif 90 <= rotation < 180
GR.axes3d(0, 0, ztick, xorg[1], yorg[2], zorg[zi], 0, 0, majorz, -ticksize)
GR.axes3d(xtick, ytick, 0, xorg[1], yorg[1], zorg[zi], majorx, majory, 0, -ticksize)
elseif 180 <= rotation < 270
GR.axes3d(xtick, 0, ztick, xorg[2], yorg[2], zorg[zi], majorx, 0, majorz, ticksize)
GR.axes3d(0, ytick, 0, xorg[1], yorg[1], zorg[zi], 0, majory, 0, -ticksize)
else
GR.axes3d(0, 0, ztick, xorg[2], yorg[1], zorg[zi], 0, 0, majorz, -ticksize)
GR.axes3d(xtick, ytick, 0, xorg[2], yorg[2], zorg[zi], majorx, majory, 0, ticksize)
end
end
else
charheight = max(0.018 * diag, 0.012)
GR.setcharheight(charheight)
if kind === :heatmap || kind === :nonuniformheatmap || kind === :shade
ticksize = -ticksize
if kind === :shade
drawgrid = false
end
end
if haskey(plt.kvs, :xticklabels) || haskey(plt.kvs, :yticklabels)
drawgrid && GR.grid(xtick, ytick, 0, 0, majorx, majory)
fx = get(plt.kvs, :xticklabels, identity) |> ticklabel_fun
fy = get(plt.kvs, :yticklabels, identity) |> ticklabel_fun
GR.axeslbl(xtick, ytick, xorg[1], yorg[1], majorx, majory, ticksize, fx, fy)
else
x_axis = GR.axis('X', tick=xtick, org=xorg[1], major_count=majorx, tick_size=ticksize)
y_axis = GR.axis('Y', tick=ytick, org=yorg[1], major_count=majory, tick_size=ticksize)
options = GR.AXES_SIMPLE_AXES|GR.AXES_TWIN_AXES
if drawgrid
options |= GR.AXES_WITH_GRID
end
GR.drawaxes(x_axis, y_axis, options)
end
end
if haskey(plt.kvs, :title)
GR.savestate()
GR.settextalign(GR.TEXT_HALIGN_CENTER, GR.TEXT_VALIGN_TOP)
text(0.5 * (viewport[1] + viewport[2]), vp[4], plt.kvs[:title])
GR.restorestate()
end
if !(kind === :wireframe || kind === :surface || kind === :plot3 || kind === :scatter3 || kind === :trisurf || kind === :volume)
if haskey(plt.kvs, :xlabel)
GR.savestate()
GR.settextalign(GR.TEXT_HALIGN_CENTER, GR.TEXT_VALIGN_BOTTOM)
text(0.5 * (viewport[1] + viewport[2]), vp[3] + 0.5 * charheight, plt.kvs[:xlabel])
GR.restorestate()
end
if haskey(plt.kvs, :ylabel)
GR.savestate()
GR.settextalign(GR.TEXT_HALIGN_CENTER, GR.TEXT_VALIGN_TOP)
GR.setcharup(-1, 0)
text(vp[1] + 0.5 * charheight, 0.5 * (viewport[3] + viewport[4]), plt.kvs[:ylabel])
GR.restorestate()
end
end
end
function draw_polar_axes(plt=plt[])
viewport = plt.kvs[:viewport]
vp = plt.kvs[:vp]
diag = sqrt((viewport[2] - viewport[1])^2 + (viewport[4] - viewport[3])^2)
charheight = max(0.018 * diag, 0.012)
window = plt.kvs[:window]
rmin, rmax = window[3], window[4]
GR.savestate()
GR.setcharheight(charheight)
GR.setlinetype(GR.LINETYPE_SOLID)
tick = auto_tick(rmin, rmax)
n = trunc(Int, (rmax - rmin) / tick)
for i in 0:n
r = rmin + i * tick / (rmax - rmin)
if i % 2 == 0
GR.setlinecolorind(88)
if i > 0
GR.drawarc(-r, r, -r, r, 0, 360)
end
else
GR.setlinecolorind(90)
GR.drawarc(-r, r, -r, r, 0, 360)
end
end
sign = if get(plt.kvs, :theta_direction, 1) > 0 1 else -1 end
offs = theta_zero_location[get(plt.kvs, :theta_zero_location, "E")]
for alpha in 0:45:315
sinf = sin((alpha * sign) * π / 180 + offs)
cosf = cos((alpha * sign) * π / 180 + offs)
GR.setlinecolorind(88)
GR.polyline([cosf, 0], [sinf, 0])
GR.settextalign(GR.TEXT_HALIGN_CENTER, GR.TEXT_VALIGN_HALF)
x, y = GR.wctondc(1.1 * cosf, 1.1 * sinf)
GR.text(x, y, string(alpha, "°"))
end
for i in 0:n
r = rmin + i * tick / (rmax - rmin)
if i % 2 == 0 || i == n
GR.settextalign(GR.TEXT_HALIGN_LEFT, GR.TEXT_VALIGN_HALF)
x, y = GR.wctondc(0.05, r)
GR.text(x, y, string(signif(rmin + i * tick, 12)))
end
end
if haskey(plt.kvs, :title)
GR.settextalign(GR.TEXT_HALIGN_CENTER, GR.TEXT_VALIGN_TOP)
text(0.5 * (viewport[1] + viewport[2]), vp[4] - 0.02, plt.kvs[:title])
end
GR.restorestate()
end
function inqtext(x, y, s)
if (in('\\', s) || in('_', s) || in('^', s)) && match(r".*\$[^\$]+?\$.*", String(s)) === nothing
GR.inqtextext(x, y, s)
else
GR.inqtext(x, y, s)
end
end
function text(x, y, s)
if (in('\\', s) || in('_', s) || in('^', s)) && match(r".*\$[^\$]+?\$.*", String(s)) === nothing
GR.textext(x, y, s)
else
GR.text(x, y, s)
end
nothing
end
function legend_size(plt=plt[])
scale = Int(GR.inqscale())
GR.selntran(0)
GR.setscale(0)
w = 0
h = 0
num_labels = length(plt.kvs[:labels])
for i in 1:num_labels
label = plt.kvs[:labels][i]
tbx, tby = inqtext(0, 0, label)
w = max(w, tbx[3] - tbx[1])
h += max(tby[3] - tby[1], 0.03)
end
GR.setscale(scale)
GR.selntran(1)
w, h
end
hasline(mask) = ( mask == 0x00 || (mask & GR.SPEC_LINE != 0) )
hasmarker(mask) = ( mask & GR.SPEC_MARKER != 0 )
hascolor(mask) = ( mask & GR.SPEC_COLOR != 0 )
function draw_legend(plt=plt[])
w, h = legend_size()
viewport = plt.kvs[:viewport]
location = get(plt.kvs, :location, 1)
num_labels = length(plt.kvs[:labels])
GR.savestate()
GR.selntran(0)
GR.setscale(0)
if location == 11 || location == 12 || location == 13
px = viewport[2] + 0.11
elseif location == 8 || location == 9 || location == 10
px = 0.5 * (viewport[1] + viewport[2] - w + 0.05)
elseif location == 2 || location == 3 || location == 6
px = viewport[1] + 0.11
else
px = viewport[2] - 0.05 - w
end
if location == 5 || location == 6 || location == 7 || location == 10 || location == 12
py = 0.5 * (viewport[3] + viewport[4] + h - 0.03)
elseif location == 13
py = viewport[3] + h
elseif location == 3 || location == 4 || location == 8
py = viewport[3] + h + 0.03
elseif location == 11
py = viewport[4] - 0.03
else
py = viewport[4] - 0.06
end
GR.setfillintstyle(GR.INTSTYLE_SOLID)
GR.setfillcolorind(0)
GR.fillrect(px - 0.08, px + w + 0.02, py + 0.03, py - h)
GR.setlinetype(GR.LINETYPE_SOLID)
GR.setlinecolorind(1)
GR.setlinewidth(1)
GR.drawrect(px - 0.08, px + w + 0.02, py + 0.03, py - h)
i = 1
GR.uselinespec(" ")
for (x, y, z, c, spec) in plt.args
if i <= num_labels
label = plt.kvs[:labels][i]
tbx, tby = inqtext(0, 0, label)
dy = max((tby[3] - tby[1]) - 0.03, 0)
py -= 0.5 * dy
end
GR.savestate()
mask = GR.uselinespec(spec)
hasline(mask) && GR.polyline([px - 0.07, px - 0.01], [py, py])
hasmarker(mask) && GR.polymarker([px - 0.06, px - 0.02], [py, py])
GR.restorestate()
GR.settextalign(GR.TEXT_HALIGN_LEFT, GR.TEXT_VALIGN_HALF)
if i <= num_labels
text(px, py, label)
py -= 0.5 * dy
i += 1
end
py -= 0.03
end
GR.selntran(1)
GR.restorestate()
end
function colorbar(off=0, colors=256, plt=plt[])
GR.savestate()
viewport = plt.kvs[:viewport]
zmin, zmax = plt.kvs[:zrange]
mask = (GR.OPTION_Z_LOG | GR.OPTION_FLIP_Y | GR.OPTION_FLIP_Z)
if get(plt.kvs, :zflip, false)
options = (GR.inqscale() | GR.OPTION_FLIP_Y)
GR.setscale(options & mask)
elseif get(plt.kvs, :yflip, false)
options = GR.inqscale() & ~GR.OPTION_FLIP_Y
GR.setscale(options & mask)
else
options = GR.inqscale()
GR.setscale(options & mask)
end
h = 0 ###0.5 * (zmax - zmin) / (colors - 1)
GR.setwindow(0, 1, zmin, zmax)
GR.setviewport(viewport[2] + 0.02 + off, viewport[2] + 0.05 + off,
viewport[3], viewport[4])
l = Int32[round(1000 + _i * 255, RoundNearestTiesUp) for _i in linspace(0, 1, colors)]
GR.cellarray(0, 1, zmax + h, zmin - h, 1, colors, l)
GR.setlinecolorind(1)
diag = sqrt((viewport[2] - viewport[1])^2 + (viewport[4] - viewport[3])^2)
charheight = max(0.016 * diag, 0.012)
GR.setcharheight(charheight)
if plt.kvs[:scale] & GR.OPTION_Z_LOG == 0
ztick = auto_tick(zmin, zmax)
y_axis = GR.axis('Y', position=1, tick=ztick, org=zmin, major_count=1, tick_size=0.005)
GR.drawaxis('Y', y_axis)
else
GR.setscale(GR.OPTION_Y_LOG)
y_axis = GR.axis('Y', position=1, tick=2, org=zmin, major_count=1, tick_size=0.005)
GR.drawaxis('Y', y_axis)
end
GR.restorestate()
end
function colormap()
rgb = Vector{UInt32}(undef, 256)
@inbounds for colorind in 1:256
color = GR.inqcolor(999 + colorind)
r = color & 0xff
g = (color >> 8) & 0xff
b = (color >> 16) & 0xff
rgb[colorind] = 0xff000000 + b << 16 + g << 8 + r
end
rgb
end
function to_rgba(value, cmap)
if !isnan(value)
i = round(Int, value * 255, RoundNearestTiesUp)
cmap[i + 1]
else
zero(UInt32)
end
end
function create_context(kind::Symbol, dict=plt[].kvs)
plt[].kvs[:kind] = kind
create_context(dict)
end
function create_context(dict::AbstractDict)
plt[].obj = copy(plt[].kvs)
for k in keys(dict)
if ! (k in kw_args)
error("Invalid keyword: $k")
end
end
merge!(plt[].kvs, dict)
end
function restore_context(plt=plt[])
empty!(ctx)
merge!(ctx, plt.kvs)
plt.kvs = copy(plt.obj)
end
"""
Create a new figure with the given settings.
Settings like the current colormap, title or axis limits as stored in the
current figure. This function creates a new figure, restores the default
settings and applies any settings passed to the function as keyword
arguments.
**Usage examples:**
.. code-block:: julia
julia> # Restore all default settings
julia> figure()
julia> # Restore all default settings and set the title
julia> figure(title="Example Figure")
"""
function figure(width::Int, height::Int, dpi::Int; kv...)
plt[] = Figure(width, height, dpi)
merge!(plt[].kvs, Dict(kv))
plt[]
end
function figure(; kv...)
plt[] = Figure()
merge!(plt[].kvs, Dict(kv))
plt[]
end
"""
Set the hold flag for combining multiple plots.
The hold flag prevents drawing of axes and clearing of previous plots, so
that the next plot will be drawn on top of the previous one.
:param flag: the value of the hold flag
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> x = LinRange(0, 1, 100)
julia> # Draw the first plot
julia> plot(x, x.^2)
julia> # Set the hold flag
julia> hold(true)
julia> # Draw additional plots
julia> plot(x, x.^4)
julia> plot(x, x.^8)
julia> # Reset the hold flag
julia> hold(false)
"""
function hold(flag, plt=plt[])
if !isempty(plt.args)
plt.kvs[:ax] = flag
plt.kvs[:clear] = !flag
for k in (:window, :scale, :xaxis, :yaxis, :zaxis)
if haskey(ctx, k)
plt.kvs[k] = ctx[k]
end
end
else
error("Invalid hold state")
end
flag
end
function usecolorscheme(index)
if 1 <= index <= 4
scheme[] = index
else
error("Invalid color sheme")
end
nothing
end
"""
Set current subplot index.
By default, the current plot will cover the whole window. To display more
than one plot, the window can be split into a number of rows and columns,
with the current plot covering one or more cells in the resulting grid.
Subplot indices are one-based and start at the upper left corner, with a
new row starting after every **num_columns** subplots.
:param num_rows: the number of subplot rows
:param num_columns: the number of subplot columns
:param subplot_indices:
- the subplot index to be used by the current plot
- a pair of subplot indices, setting which subplots should be covered
by the current plot
**Usage examples:**
.. code-block:: julia
julia> # Set the current plot to the second subplot in a 2x3 grid
julia> subplot(2, 3, 2)
julia> # Set the current plot to cover the first two rows of a 4x2 grid
julia> subplot(4, 2, (1, 4))
julia> # Use the full window for the current plot
julia> subplot(1, 1, 1)
"""
function subplot(nr, nc, p, plt=plt[])
xmin, xmax, ymin, ymax = 1, 0, 1, 0
for i in collect(p)
r = nr - div(i-1, nc)
c = (i-1) % nc + 1
xmin = min(xmin, (c-1)/nc)
xmax = max(xmax, c/nc)
ymin = min(ymin, (r-1)/nr)
ymax = max(ymax, r/nr)
end
plt.kvs[:subplot] = [xmin, xmax, ymin, ymax]
plt.kvs[:clear] = collect(p)[1] == 1
plt.kvs[:update] = collect(p)[end] == nr * nc
end
"""
Set the flag to draw a grid in the plot axes.
:param flag: the value of the grid flag (`true` by default)
**Usage examples:**
.. code-block:: julia
julia> # Hid the grid on the next plot
julia> grid(false)
julia> # Restore the grid
julia> grid(true)
"""
drawgrid(flag, plt=plt[]) = (plt.kvs[:grid] = flag)
const doc_ticks = """
Set the intervals of the ticks for the X, Y or Z axis.
Use the function `xticks`, `yticks` or `zticks` for the corresponding axis.
:param minor: the interval between minor ticks.
:param major: (optional) the number of minor ticks between major ticks.
**Usage examples:**
.. code-block:: julia
julia> # Minor ticks every 0.2 units in the X axis
julia> xticks(0.2)
julia> # Major ticks every 1 unit (5 minor ticks) in the Y axis
julia> yticks(0.2, 5)
"""
@doc doc_ticks xticks(minor, major::Int=1, plt=plt[]) = (plt.kvs[:xticks] = (minor, major))
@doc doc_ticks yticks(minor, major::Int=1, plt=plt[]) = (plt.kvs[:yticks] = (minor, major))
@doc doc_ticks zticks(minor, major::Int=1, plt=plt[]) = (plt.kvs[:zticks] = (minor, major))
const doc_ticklabels = """
Customize the string of the X and Y axes tick labels.
The labels of the tick axis can be defined through a function
with one argument (the numeric value of the tick position) and
returns a string, or through an array of strings that are located
sequentially at X = 1, 2, etc.
:param s: function or array of strings that define the tick labels.
**Usage examples:**
.. code-block:: julia
julia> # Label the range (0-1) of the Y-axis as percent values
julia> yticklabels(p -> Base.Printf.@sprintf("%0.0f%%", 100p))
julia> # Label the X-axis with a sequence of strings
julia> xticklabels(["first", "second", "third"])
"""
@doc doc_ticklabels xticklabels(s, plt=plt[]) = (plt.kvs[:xticklabels] = s)
@doc doc_ticklabels yticklabels(s, plt=plt[]) = (plt.kvs[:yticklabels] = s)
# Normalize a color c with the range [cmin, cmax]
# 0 <= normalize_color(c, cmin, cmax) <= 1
function normalize_color(c, cmin, cmax)
c = clamp(c, cmin, cmax) - cmin
if cmin != cmax
c /= cmax - cmin
end
c
end
function plot_img(I, plt=plt[])
viewport = plt.kvs[:vp][:]
if haskey(plt.kvs, :title)
viewport[4] -= 0.05
end
vp = plt.kvs[:vp]
if isa(I, AbstractString)
width, height, data = GR.readimage(I)
else
I = I'
width, height = size(I)
cmin, cmax = plt.kvs[:crange]
data = map(x -> normalize_color(x, cmin, cmax), I)
data = Int32[round(1000 + _i * 255, RoundNearestTiesUp) for _i in data]
end
if width * (viewport[4] - viewport[3]) <
height * (viewport[2] - viewport[1])
w = width / height * (viewport[4] - viewport[3])
xmin = max(0.5 * (viewport[1] + viewport[2] - w), viewport[1])
xmax = min(0.5 * (viewport[1] + viewport[2] + w), viewport[2])
ymin = viewport[3]
ymax = viewport[4]
else
h = height / width * (viewport[2] - viewport[1])
xmin = viewport[1]
xmax = viewport[2]
ymin = max(0.5 * (viewport[4] + viewport[3] - h), viewport[3])
ymax = min(0.5 * (viewport[4] + viewport[3] + h), viewport[4])
end
GR.selntran(0)
GR.setscale(0)
if get(plt.kvs, :xflip, false)
tmp = xmax; xmax = xmin; xmin = tmp;
end
if get(plt.kvs, :yflip, false)
tmp = ymax; ymax = ymin; ymin = tmp;
end
if isa(I, AbstractString)
GR.drawimage(xmin, xmax, ymin, ymax, width, height, data)
else
GR.cellarray(xmin, xmax, ymin, ymax, width, height, data)
end
if haskey(plt.kvs, :title)
GR.savestate()
GR.settextalign(GR.TEXT_HALIGN_CENTER, GR.TEXT_VALIGN_TOP)
text(0.5 * (viewport[1] + viewport[2]), vp[4], plt.kvs[:title])
GR.restorestate()
end
GR.selntran(1)
end
function plot_iso(V, plt=plt[])
nx, ny, nz = size(V)
isovalue = get(plt.kvs, :isovalue, 0.5)
rotation = get(plt.kvs, :rotation, 40)
tilt = get(plt.kvs, :tilt, 60)
if haskey(plt.kvs, :color)
color = plt.kvs[:color]
else
color = (0.0, 0.5, 0.8)
end
GR.setwindow3d(-1, 1, -1, 1, -1, 1)
GR.setspace3d(-rotation, tilt, 45, 2.5)
ambient, diffuse, specular, specular_power = GR.gr3.getlightparameters()
GR.gr3.setlightparameters(0.2, 0.8, 0.7, 128.0)
GR.gr3.isosurface(V, isovalue, color)
GR.gr3.setlightparameters(ambient, diffuse, specular, specular_power)
end
function plot_polar(θ, ρ, plt=plt[])
window = plt.kvs[:window]
rmin, rmax = window[3], window[4]
sign = if get(plt.kvs, :theta_direction, 1) > 0 1 else -1 end
offs = theta_zero_location[get(plt.kvs, :theta_zero_location, "E")]
ρ = ρ ./ rmax
n = length(ρ)
x, y = zeros(n), zeros(n)
for i in 1:n
x[i] = ρ[i] * cos(θ[i] * sign + offs)
y[i] = ρ[i] * sin(θ[i] * sign + offs)
end
GR.polyline(x, y)
end
function RGB(color)
float((color >> 16) & 0xff) / 255,
float((color >> 8) & 0xff) / 255,
float( color & 0xff) / 255
end
to_double(a) = Float64[float(el) for el in a]
to_int(a) = Int32[round(Int32, el) for el in a]
function send_data(handle, name, data)
GR.sendmetaref(handle, name, 'D', to_double(data))
dims = size(data)
if length(dims) > 1
GR.sendmetaref(handle, string(name, "_dims"), 'I', to_int(dims))
end
nothing
end
function shutdown()
if handle[] != C_NULL
GR.sendmetaref(handle[], "request", 's', "close");
GR.sendmetaref(handle[], "", '\0', "", 0);
end
end
function send_meta(target, plt=plt[])
if handle[] === C_NULL
handle[] = GR.openmeta(target)
atexit(shutdown)
end
if handle[] != C_NULL
for (k, v) in plt.kvs
if haskey(grm_aliases, k)
k = grm_aliases[k]
end
if k === :backgroundcolor || k === :color || k === :colormap || k === :location || k === :nbins ||
k === :rotation || k === :tilt || k === :transform
GR.sendmetaref(handle[], string(k), 'i', Int32(v))
elseif k === :alpha || k === :isovalue
GR.sendmetaref(handle[], string(k), 'd', Float64(v))
elseif k === :x_lim || k === :y_lim || k === :z_lim || k === :c_lim || k === :size
GR.sendmetaref(handle[], string(k), 'D', to_double(v))
elseif k === :title || k === :x_label || k === :y_label || k === :z_label
GR.sendmetaref(handle[], string(k), 's', String(v))
elseif k === :labels
s = [String(el) for el in v]
GR.sendmetaref(handle[], string(k), 'S', s, length(s))
elseif k === :x_flip || k === :y_flip || k === :z_flip || k === :x_log || k === :y_log || k === :z_log
GR.sendmetaref(handle[], string(k), 'i', v ? 1 : 0)
end
end
num_series = length(plt.args)
GR.sendmetaref(handle[], "series", 'O', "[", num_series)
for (i, (x, y, z, c, spec)) in enumerate(plt.args)
x !== nothing && send_data(handle[], "x", to_double(x))
y !== nothing && send_data(handle[], "y", to_double(y))
z !== nothing && send_data(handle[], "z", to_double(z))
c !== nothing && send_data(handle[], "c", to_double(c))
spec !== nothing && GR.sendmetaref(handle[], "line_spec", 's', spec)
GR.sendmetaref(handle[], "", 'O', i < num_series ? "," : "]", 1)
end
if plt.kvs[:kind] === :hist
GR.sendmetaref(handle[], "kind", 's', "barplot");
else
GR.sendmetaref(handle[], "kind", 's', string(plt.kvs[:kind]));
end
if get(plt.kvs, :keepaspect, false)
GR.sendmetaref(handle[], "keep_aspect_ratio", 'i', 1)
end
GR.sendmetaref(handle[], "", '\0', "", 0);
end
nothing
end
function send_serialized(target, plt=plt[])
sock = connect(target, 8001)
serialize(sock, Dict("kvs" => plt.kvs, "args" => plt.args))
close(sock)
end
function contains_NaN(a)
for el in a
if el === NaN
return true
end
end
false
end
function plot_data(flag=true, plt=plt[])
if isempty(plt.args)
return
end
GR.init()
target = GR.displayname()
if flag && target !== ""
if target == "js" || target == "meta" || target == "plot" || target == "edit" || target == "pluto" || target == "js-server"
send_meta(0)
else
send_serialized(target)
end
if target == "pluto" || target == "js"
return GR.js.get_html()
end
return
end
kind = get(plt.kvs, :kind, :line)
plt.kvs[:clear] && GR.clearws()
if scheme[] != 0
for colorind in 1:8
color = colors[colorind, scheme[]]
r, g, b = RGB(color)
GR.setcolorrep(colorind - 1, r, g, b)
if scheme != 1
GR.setcolorrep(distinct_cmap[colorind], r, g, b)
end
end
r, g, b = RGB(colors[1, scheme[]])
rdiff, gdiff, bdiff = RGB(colors[2, scheme[]]) .- (r, g, b)
for colorind in 1:12
f = (colorind - 1) / 11.0
GR.setcolorrep(92 - colorind, r + f*rdiff, g + f*gdiff, b + f*bdiff)
end
end
if haskey(plt.kvs, :font)
name = plt.kvs[:font]
if haskey(fonts, name)
font = fonts[name]
GR.settextfontprec(font, font > 200 ? 3 : 0)
else
font = GR.loadfont(name)
if font >= 0
GR.settextfontprec(font, 3)
end
end
else
GR.settextfontprec(232, 3) # CM Serif Roman
end
set_viewport(kind, plt.kvs[:subplot], plt)
if !plt.kvs[:ax]
set_window(kind, plt)
if kind === :polar || kind === :polarhist
draw_polar_axes()
elseif !(kind === :imshow || kind === :isosurface || kind === :polarheatmap || kind === :nonuniformpolarheatmap)
draw_axes(kind)
end
end
if haskey(plt.kvs, :colormap)
GR.setcolormap(plt.kvs[:colormap])
else
GR.setcolormap(GR.COLORMAP_VIRIDIS)
end
GR.uselinespec(" ")
for (x, y, z, c, spec) in plt.args
GR.savestate()
if haskey(plt.kvs, :alpha)
GR.settransparency(plt.kvs[:alpha])
end
if kind === :line
mask = GR.uselinespec(spec)
if c !== nothing
linewidth = get(plt.kvs, :linewidth, 1)
z = ones(length(x)) * linewidth
GR.polyline(x, y, z, c)
else
if hasline(mask)
linewidth = get(plt.kvs, :linewidth, 1)
GR.setlinewidth(linewidth)
GR.polyline(x, y)
end
hasmarker(mask) && GR.polymarker(x, y)
end
elseif kind === :stairs
mask = GR.uselinespec(spec)
if hasline(mask)
where = get(plt.kvs, :where, "mid")
if where == "pre"
n = length(x)
xs = zeros(2 * n - 1)
ys = zeros(2 * n - 1)
xs[1] = x[1]
ys[1] = y[1]
for i in 1:n-1
xs[2*i] = x[i]
xs[2*i+1] = x[i+1]
ys[2*i] = y[i+1]
ys[2*i+1] = y[i+1]
end
elseif where == "post"
n = length(x)
xs = zeros(2 * n - 1)
ys = zeros(2 * n - 1)
xs[1] = x[1]
ys[1] = y[1]
for i in 1:n-1
xs[2*i] = x[i+1]
xs[2*i+1] = x[i+1]
ys[2*i] = y[i]
ys[2*i+1] = y[i+1]
end
else
n = length(x)
xs = zeros(2 * n)
ys = zeros(2 * n)
xs[1] = x[1]
for i in 1:n-1
xs[2*i] = 0.5 * (x[i] + x[i+1])
xs[2*i+1] = 0.5 * (x[i] + x[i+1])
ys[2*i-1] = y[i]
ys[2*i] = y[i]
end
xs[2*n] = x[n]
ys[2*n-1] = y[n]
ys[2*n] = y[n]
end
GR.polyline(xs, ys)
end
hasmarker(mask) && GR.polymarker(x, y)
elseif kind === :scatter
GR.setmarkertype(GR.MARKERTYPE_SOLID_CIRCLE)
if z !== nothing || c !== nothing
if c !== nothing
cmin, cmax = plt.kvs[:crange]
c = map(x -> normalize_color(x, cmin, cmax), c)
cind = Int[round(1000 + _i * 255, RoundNearestTiesUp) for _i in c]
end
GR.polymarker(x, y, z, cind)
else
GR.polymarker(x, y)
end
elseif kind === :stem
GR.setmarkertype(GR.MARKERTYPE_SOLID_CIRCLE)
GR.uselinespec(spec)
for i = 1:length(y)
GR.polyline([x[i]; x[i]], [0; y[i]])
GR.polymarker([x[i]], [y[i]])
end
GR.setlinecolorind(1)
GR.polyline([plt.kvs[:window][1]; plt.kvs[:window][2]], [0; 0])
elseif kind === :hist
ymin = plt.kvs[:window][3]
GR.setfillcolorind(989)
GR.setfillintstyle(GR.INTSTYLE_SOLID)
for i = 1:length(y)
GR.fillrect(x[i], x[i+1], ymin, y[i])
end
GR.setfillcolorind(1)
GR.setfillintstyle(GR.INTSTYLE_HOLLOW)
for i = 1:length(y)
GR.fillrect(x[i], x[i+1], ymin, y[i])
end
elseif kind === :polarhist
ymax = plt.kvs[:window][4]
ρ = y ./ ymax
θ = x * 180/π
for i = 2:length(ρ)
GR.setfillcolorind(989)
GR.setfillintstyle(GR.INTSTYLE_SOLID)
GR.fillarc(-ρ[i], ρ[i], -ρ[i], ρ[i], θ[i-1], θ[i])
GR.setfillcolorind(1)
GR.setfillintstyle(GR.INTSTYLE_HOLLOW)
GR.fillarc(-ρ[i], ρ[i], -ρ[i], ρ[i], θ[i-1], θ[i])
end
elseif kind === :polarheatmap || kind === :nonuniformpolarheatmap
w, h = size(z)
cmap = colormap()
cmin, cmax = plt.kvs[:zrange]
data = map(x -> normalize_color(x, cmin, cmax), z)
if get(plt.kvs, :xflip, false)
data = reverse(data, dims=1)
end
if get(plt.kvs, :yflip, false)
data = reverse(data, dims=2)
end
colors = round.(Int32, 1000 .+ data .* 255, RoundNearestTiesUp)
if kind === :polarheatmap
GR.polarcellarray(0, 0, 0, 360, 0, 1, w, h, colors)
else
ymin, ymax = plt.kvs[:window][3:4]
ρ = ymin .+ y ./ (ymax - ymin)
θ = x * 180/π
GR.nonuniformpolarcellarray(θ, ρ, w, h, colors)
end
draw_polar_axes()
plt.kvs[:zrange] = cmin, cmax
colorbar(0.025)
elseif kind === :contour
zmin, zmax = plt.kvs[:zrange]
if length(x) == length(y) == length(z)
x, y, z = GR.gridit(vec(x), vec(y), vec(z'), 200, 200)
zmin, zmax = get(plt.kvs, :zlim, (_min(z), _max(z)))
end
proj = GR.inqprojectiontype()
GR.setprojectiontype(0)
GR.setspace(zmin, zmax, 0, 90)
levels = get(plt.kvs, :levels, 0)
clabels = get(plt.kvs, :clabels, false)
if typeof(levels) <: Int
if levels == 0
levels = 20
end
h = [zmin + (_i - 1) * (zmax - zmin) / levels for _i = 1:levels]
else
h = float(levels)
end
GR.contour(x, y, h, z, clabels ? 1001 : 1000)
colorbar(0, length(h))
GR.setprojectiontype(proj)
elseif kind === :contourf
zmin, zmax = plt.kvs[:zrange]
if length(x) == length(y) == length(z)
x, y, z = GR.gridit(vec(x), vec(y), vec(z'), 200, 200)
zmin, zmax = get(plt.kvs, :zlim, (_min(z), _max(z)))
end
proj = GR.inqprojectiontype()
GR.setprojectiontype(0)
GR.setspace(zmin, zmax, 0, 90)
levels = get(plt.kvs, :levels, 0)
clabels = get(plt.kvs, :clabels, false)
clines = get(plt.kvs, :clines, true)
if typeof(levels) <: Int
if levels == 0
levels = 20
end
h = [zmin + (_i - 1) * (zmax - zmin) / levels for _i = 1:levels]
else
h = float(levels)
end
GR.contourf(x, y, h, z, clines ? (clabels ? 1 : 0) : -1)
colorbar(0, length(h))
GR.setprojectiontype(proj)
elseif kind === :hexbin
nbins = get(plt.kvs, :nbins, 40)
cntmax = GR.hexbin(x, y, nbins)
if cntmax > 0
plt.kvs[:zrange] = 0, cntmax
colorbar()
end
elseif kind === :heatmap || kind === :nonuniformheatmap
w, h = size(z)
cmap = colormap()
cmin, cmax = plt.kvs[:crange]
levels = get(plt.kvs, :levels, 256)
data = map(x -> normalize_color(x, cmin, cmax), z)
if kind === :heatmap && !haskey(ENV, "GR_SCALE_FACTOR")
rgba = [to_rgba(value, cmap) for value = data]
GR.drawimage(0.5, w + 0.5, h + 0.5, 0.5, w, h, rgba)
else
colors = Int[round(isnan(_i) ? 1256 : 1000 + _i * 255, RoundNearestTiesUp) for _i in data]
GR.nonuniformcellarray(x, y, w, h, colors)
end
colorbar(0, levels)
elseif kind === :wireframe
if length(x) == length(y) == length(z)
x, y, z = GR.gridit(vec(x), vec(y), vec(z'), 50, 50)
end
GR.setfillcolorind(0)
GR.surface(x, y, z, GR.OPTION_FILLED_MESH)
draw_axes(kind, 2)
elseif kind === :surface
if isa(x, AbstractMatrix) && isa(y, AbstractMatrix) && isa(z, AbstractMatrix)
option = GR.OPTION_3D_MESH
else
option = GR.OPTION_COLORED_MESH
if length(x) == length(y) == length(z)
x, y, z = GR.gridit(vec(x), vec(y), vec(z'), 200, 200)
end
end
ambient, diffuse, specular, specular_power = GR.gr3.getlightparameters()
GR.gr3.setlightparameters(0.8, 0.2, 0.1, 10.0)
if get(plt.kvs, :accelerate, true)
GR.gr3.surface(x, y, z, option)
else
GR.surface(x, y, z, option)
end
GR.gr3.setlightparameters(ambient, diffuse, specular, specular_power)
draw_axes(kind, 2)
colorbar(0.05)
GR.gr3.terminate()
elseif kind === :volume
algorithm = get(plt.kvs, :algorithm, 0)
w, h, ratio = GR.inqvpsize()
GR.setpicturesizeforvolume(round(Int, w * ratio), round(Int, h * ratio))
ambient, diffuse, specular, specular_power = GR.gr3.getlightparameters()
GR.gr3.setlightparameters(0.8, 0.2, 0.1, 10.0)
dmin, dmax = GR.gr3.volume(c, algorithm)
GR.gr3.setlightparameters(ambient, diffuse, specular, specular_power)
draw_axes(kind, 2)
plt.kvs[:zrange] = dmin, dmax
colorbar(0.05)
elseif kind === :plot3
linewidth = get(plt.kvs, :linewidth, 1)
GR.setlinewidth(linewidth)
GR.polyline3d(x, y, z)
draw_axes(kind, 2)
elseif kind === :scatter3
GR.setmarkertype(GR.MARKERTYPE_SOLID_CIRCLE)
if c !== nothing
cmin, cmax = plt.kvs[:crange]
c = map(x -> normalize_color(x, cmin, cmax), c)
cind = Int[round(1000 + _i * 255, RoundNearestTiesUp) for _i in c]
for i in 1:length(x)
GR.setmarkercolorind(cind[i])
GR.polymarker3d([x[i]], [y[i]], [z[i]])
end
else
GR.polymarker3d(x, y, z)
end
draw_axes(kind, 2)
elseif kind === :imshow
plot_img(c)
elseif kind === :isosurface
plot_iso(c)
elseif kind === :polar
GR.uselinespec(spec)
plot_polar(x, y)
elseif kind === :trisurf
GR.trisurface(x, y, z)
draw_axes(kind, 2)
colorbar(0.05)
elseif kind === :tricont
zmin, zmax = plt.kvs[:zrange]
levels = linspace(zmin, zmax, 20)
GR.tricontour(x, y, z, levels)
colorbar()
elseif kind === :shade
xform = get(plt.kvs, :xform, 5)
if contains_NaN(x)
GR.shadelines(x, y, xform=xform)
else
GR.shadepoints(x, y, xform=xform)
end
elseif kind === :bar
for i = 1:2:length(x)
GR.setfillcolorind(989)
GR.setfillintstyle(GR.INTSTYLE_SOLID)
GR.fillrect(x[i], x[i+1], y[i], y[i+1])
GR.setfillcolorind(1)
GR.setfillintstyle(GR.INTSTYLE_HOLLOW)
GR.fillrect(x[i], x[i+1], y[i], y[i+1])
end
end
GR.restorestate()
end
if (kind === :line || kind === :stairs || kind === :scatter || kind === :stem) && haskey(plt.kvs, :labels)
draw_legend()
end
if plt.kvs[:update]
GR.updatews()
if GR.isinline()
restore_context()
return GR.show()
end
end
flag && restore_context()
return
end
function plot_args(@nospecialize args; fmt=:xys, plt=plt[])
args = Any[args...]
pltargs = NTuple{5, Any}[]
while length(args) > 0
# parse arguments for plotting
local x, y, z, c
a = popfirst!(args)
if isa(a, AbstractVecOrMat) || isa(a, Function)
elt = eltype(a)
if elt <: Complex
x = real(a)
y = imag(a)
z = nothing
c = nothing
elseif elt <: Real || isa(a, Function)
if fmt === :xys
if length(args) >= 1 &&
(isa(args[1], AbstractVecOrMat) && eltype(args[1]) <: Real || isa(args[1], Function))
x = a
y = popfirst!(args)
z = nothing
c = nothing
else
y = a
n = isrowvec(y) ? size(y, 2) : size(y, 1)
x = linspace(1, n, n)
z = nothing
c = nothing
end
elseif fmt === :xyac || fmt === :xyzc
if length(args) >= 3 &&
isa(args[1], AbstractVecOrMat) && eltype(args[1]) <: Real &&
(isa(args[2], AbstractVecOrMat) && eltype(args[2]) <: Real || isa(args[2], Function)) &&
(isa(args[3], AbstractVecOrMat) && eltype(args[3]) <: Real || isa(args[3], Function))
x = a
y = popfirst!(args)
z = popfirst!(args)
if !isa(z, Function)
z = z'
end
c = popfirst!(args)
elseif length(args) >= 2 &&
isa(args[1], AbstractVecOrMat) && eltype(args[1]) <: Real &&
(isa(args[2], AbstractVecOrMat) && eltype(args[2]) <: Real || isa(args[2], Function))
x = a
y = popfirst!(args)
z = popfirst!(args)
if !isa(z, Function)
z = z'
end
c = nothing
elseif fmt === :xyac && length(args) >= 1 &&
(isa(args[1], AbstractVecOrMat) && eltype(args[1]) <: Real || isa(args[1], Function))
x = a
y = popfirst!(args)
z = nothing
c = nothing
elseif fmt === :xyzc && length(args) == 0
z = a'
nx, ny = size(z)
x = linspace(1, nx, nx)
y = linspace(1, ny, ny)
c = nothing
end
end
else
error("expected Real or Complex")
end
else
error("expected array or function")
end
if isa(y, Function)
f = y
y = Float64[f(a) for a in x]
end
if isa(z, Function)
f = z
z = Float64[f(a,b) for a in x, b in y]
end
spec = ""
if fmt === :xys && length(args) > 0
if isa(args[1], AbstractString)
spec = popfirst!(args)
elseif length(args) == 1
c = popfirst!(args)
end
end
# Setup arguments for plotting
isa(x, UnitRange) && (x = collect(x))
isa(y, UnitRange) && (y = collect(y))
isa(z, UnitRange) && (z = collect(z))
isa(c, UnitRange) && (c = collect(c))
isvector(x) && (x = vec(x))
if isa(y, Function)
y = [y(a) for a in x]
else
isvector(y) && (y = vec(y))
end
if z !== nothing
if fmt === :xyzc && isa(z, Function)
z = [z(a,b) for a in x, b in y]
else
isvector(z) && (z = vec(z))
end
end
if c !== nothing
isvector(c) && (c = vec(c))
end
if z === nothing
if isa(x, AbstractVector) && isa(y, AbstractVector)
push!(pltargs, (x, y, z, c, spec))
elseif isa(x, AbstractVector)
if length(x) == size(y, 1)
for j = 1:size(y, 2)
push!(pltargs, (x, view(y,:,j), z, c, spec))
end
else
for i = 1:size(y, 1)
push!(pltargs, (x, view(y,i,:), z, c, spec))
end
end
elseif isa(y, AbstractVector)
if size(x, 1) == length(y)
for j = 1:size(x, 2)
push!(pltargs, (view(x,:,j), y, z, c, spec))
end
else
for i = 1:size(x, 1)
push!(pltargs, (view(x,i,:), y, z, c, spec))
end
end
else
@assert size(x) == size(y)
for j = 1:size(y, 2)
push!(pltargs, (view(x,:,j), view(y,:,j), z, c, spec))
end
end
elseif (isa(x, AbstractVector) && isa(y, AbstractVector) &&
(isa(z, AbstractVector) || typeof(z) == Array{Float64,2} ||
typeof(z) == Array{Int32,2} || typeof(z) == Array{Any,2})) ||
(isa(x, AbstractMatrix) && isa(y, AbstractMatrix) &&
(isa(z, AbstractMatrix)))
push!(pltargs, (x, y, z, c, spec))
else
push!(pltargs, (vec(float(x)), vec(float(y)), vec(float(z)), c, spec) )
end
end
pltargs
end
"""
Draw one or more line plots.
This function can receive one or more of the following:
- x values and y values, or
- x values and a callable to determine y values, or
- y values only, with their indices as x values
:param args: the data to plot
**Usage examples:**
.. code-block:: julia-repl
julia> # Create example data
julia> x = LinRange(-2, 2, 40)
julia> y = 2 .* x .+ 4
julia> # Plot x and y
julia> plot(x, y)
julia> # Plot x and a callable
julia> plot(x, t -> t^3 + t^2 + t)
julia> # Plot y, using its indices for the x values
julia> plot(y)
"""
function plot(args::PlotArg...; kv...)
create_context(:line, Dict(kv))
if plt[].kvs[:ax]
plt[].args = append!(plt[].args, plot_args(args))
else
plt[].args = plot_args(args)
end
plot_data()
end
"""
Draw one or more line plots over another plot.
This function can receive one or more of the following:
- x values and y values, or
- x values and a callable to determine y values, or
- y values only, with their indices as x values
:param args: the data to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> x = LinRange(-2, 2, 40)
julia> y = 2 .* x .+ 4
julia> # Draw the first plot
julia> plot(x, y)
julia> # Plot graph over it
julia> oplot(x, x -> x^3 + x^2 + x)
"""
function oplot(args::PlotArg...; kv...)
create_context(:line, Dict(kv))
plt[].args = append!(plt[].args, plot_args(args))
plot_data()
end
"""
Draw one or more step or staircase plots.
This function can receive one or more of the following:
- x values and y values, or
- x values and a callable to determine y values, or
- y values only, with their indices as x values
:param args: the data to plot
:param where: pre, mid or post, to decide where the step between two y values should be placed
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> x = LinRange(-2, 2, 40)
julia> y = 2 .* x .+ 4
julia> # Plot x and y
julia> stairs(x, y)
julia> # Plot x and a callable
julia> stairs(x, x -> x^3 + x^2 + x)
julia> # Plot y, using its indices for the x values
julia> stairs(y)
julia> # Use next y step directly after x each position
julia> stairs(y, where="pre")
julia> # Use next y step between two x positions
julia> stairs(y, where="mid")
julia> # Use next y step immediately before next x position
julia> stairs(y, where="post")
"""
function stairs(args...; kv...)
create_context(:stairs, Dict(kv))
plt[].args = plot_args(args, fmt=:xyac)
plot_data()
end
"""
Draw one or more scatter plots.
This function can receive one or more of the following:
- x values and y values, or
- x values and a callable to determine y values, or
- y values only, with their indices as x values
Additional to x and y values, you can provide values for the markers'
size and color. Size values will determine the marker size in percent of
the regular size, and color values will be used in combination with the
current colormap.
:param args: the data to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> x = LinRange(-2, 2, 40)
julia> y = 0.2 .* x .+ 0.4
julia> # Plot x and y
julia> scatter(x, y)
julia> # Plot x and a callable
julia> scatter(x, x -> 0.2 * x + 0.4)
julia> # Plot y, using its indices for the x values
julia> scatter(y)
julia> # Plot a diagonal with increasing size and color
julia> x = LinRange(0, 1, 11)
julia> y = LinRange(0, 1, 11)
julia> s = LinRange(50, 400, 11)
julia> c = LinRange(0, 255, 11)
julia> scatter(x, y, s, c)
"""
function scatter(args...; kv...)
create_context(:scatter, Dict(kv))
plt[].args = plot_args(args, fmt=:xyac)
plot_data()
end
"""
Draw a stem plot.
This function can receive one or more of the following:
- x values and y values, or
- x values and a callable to determine y values, or
- y values only, with their indices as x values
:param args: the data to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> x = LinRange(-2, 2, 40)
julia> y = 0.2 .* x .+ 0.4
julia> # Plot x and y
julia> stem(x, y)
julia> # Plot x and a callable
julia> stem(x, x -> x^3 + x^2 + x + 6)
julia> # Plot y, using its indices for the x values
julia> stem(y)
"""
function stem(args...; kv...)
create_context(:stem, Dict(kv))
plt[].args = plot_args(args)
plot_data()
end
function barcoordinates(heights; barwidth=0.8, baseline=0.0, kv...)
n = length(heights)
halfw = barwidth/2
wc = zeros(2n)
hc = zeros(2n)
for (i, value) in enumerate(heights)
wc[2i-1] = i - halfw
wc[2i] = i + halfw
hc[2i-1] = baseline
hc[2i] = value
end
(wc, hc)
end
"""
Draw a bar plot.
If no specific labels are given, the axis is labelled with integer
numbers starting from 1.
Use the keyword arguments **barwidth**, **baseline** or **horizontal**
to modify the default width of the bars (by default 0.8 times the separation
between bars), the baseline value (by default zero), or the direction of
the bars (by default vertical).
:param labels: the labels of the bars
:param heights: the heights of the bars
**Usage examples:**
.. code-block:: julia
julia> # World population by continents (millions)
julia> population = Dict("Africa" => 1216,
"America" => 1002,
"Asia" => 4436,
"Europe" => 739,
"Oceania" => 38)
julia> barplot(keys(population), values(population))
julia> # Horizontal bar plot
julia> barplot(keys(population), values(population), horizontal=true)
"""
function barplot(labels, heights, plt=plt[]; kv...)
kv = Dict(kv)
wc, hc = barcoordinates(heights; kv...)
horizontal = pop!(kv, :horizontal, false)
create_context(:bar, kv)
if horizontal
plt.args = [(hc, wc, nothing, nothing, "")]
yticks(1,1)
yticklabels(string.(labels))
else
plt.args = [(wc, hc, nothing, nothing, "")]
xticks(1,1)
xticklabels(string.(labels))
end
plot_data()
end
barplot(heights; kv...) = barplot(string.(1:length(heights)), heights; kv...)
function hist(x, nbins::Integer=0)
if nbins <= 1
nbins = round(Int, 3.3 * log10(length(x))) + 1
end
xmin, xmax = extrema(x)
edges = linspace(xmin, xmax, nbins + 1)
counts = zeros(nbins)
buckets = Int[max(2, min(searchsortedfirst(edges, xᵢ), length(edges)))-1 for xᵢ in x]
for b in buckets
counts[b] += 1
end
collect(edges), counts
end
"""
Draw a histogram.
If **nbins** is **nothing** or 0, this function computes the number of
bins as 3.3 * log10(n) + 1, with n as the number of elements in x,
otherwise the given number of bins is used for the histogram.
:param x: the values to draw as histogram
:param num_bins: the number of bins in the histogram
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> x = 2 .* rand(100) .- 1
julia> # Draw the histogram
julia> histogram(x)
julia> # Draw the histogram with 19 bins
julia> histogram(x, nbins=19)
"""
function histogram(x, plt=plt[]; kv...)
create_context(:hist, Dict(kv))
nbins = get(plt.kvs, :nbins, 0)
x, y = hist(x, nbins)
plt.args = [(x, y, nothing, nothing, "")]
plot_data()
end
"""
Draw a polar histogram.
If **nbins** is **nothing** or 0, this function computes the number of
bins as 3.3 * log10(n) + 1, with n as the number of elements in x,
otherwise the given number of bins is used for the histogram.
:param x: the values to draw as a polar histogram
:param num_bins: the number of bins in the polar histogram
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> x = 2 .* rand(100) .- 1
julia> # Draw the polar histogram
julia> polarhistogram(x, alpha=0.5)
julia> # Draw the polar histogram with 19 bins
julia> polarhistogram(x, nbins=19, alpha=0.5)
"""
function polarhistogram(x, plt=plt[]; kv...)
create_context(:polarhist, Dict(kv))
nbins = get(plt.kvs, :nbins, 0)
x, y = hist(x, nbins)
plt.args = [(x, y, nothing, nothing, "")]
plot_data()
end
"""
Draw a contour plot.
This function uses the current colormap to display a either a series of
points or a two-dimensional array as a contour plot. It can receive one
or more of the following:
- x values, y values and z values, or
- M x values, N y values and z values on a NxM grid, or
- M x values, N y values and a callable to determine z values
If a series of points is passed to this function, their values will be
interpolated on a grid. For grid points outside the convex hull of the
provided points, a value of 0 will be used.
:param args: the data to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example point data
julia> x = 8 .* rand(100) .- 4
julia> y = 8 .* rand(100) .- 4
julia> z = sin.(x) .+ cos.(y)
julia> # Draw the contour plot
julia> contour(x, y, z)
julia> # Create example grid data
julia> x = LinRange(-2, 2, 40)
julia> y = LinRange(0, pi, 20)
julia> z = sin.(x') .+ cos.(y)
julia> # Draw the contour plot
julia> contour(x, y, z)
julia> # Draw the contour plot using a callable
julia> contour(x, y, (x,y) -> sin(x) + cos(y))
"""
function contour(args...; kv...)
create_context(:contour, Dict(kv))
plt[].args = plot_args(args, fmt=:xyzc)
plot_data()
end
"""
Draw a filled contour plot.
This function uses the current colormap to display a either a series of
points or a two-dimensional array as a filled contour plot. It can
receive one or more of the following:
- x values, y values and z values, or
- M x values, N y values and z values on a NxM grid, or
- M x values, N y values and a callable to determine z values
If a series of points is passed to this function, their values will be
interpolated on a grid. For grid points outside the convex hull of the
provided points, a value of 0 will be used.
:param args: the data to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example point data
julia> x = 8 .* rand(100) .- 4
julia> y = 8 .* rand(100) .- 4
julia> z = sin.(x) .+ cos.(y)
julia> # Draw the contour plot
julia> contourf(x, y, z)
julia> # Create example grid data
julia> x = LinRange(-2, 2, 40)
julia> y = LinRange(0, pi, 20)
julia> z = sin.(x') .+ cos.(y)
julia> # Draw the contour plot
julia> contourf(x, y, z)
julia> # Draw the contour plot using a callable
julia> contourf(x, y, (x,y) -> sin(x) + cos(y))
"""
function contourf(args...; kv...)
create_context(:contourf, Dict(kv))
plt[].args = plot_args(args, fmt=:xyzc)
plot_data()
end
"""
Draw a hexagon binning plot.
This function uses hexagonal binning and the the current colormap to
display a series of points. It can receive one or more of the following:
- x values and y values, or
- x values and a callable to determine y values, or
- y values only, with their indices as x values
:param args: the data to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> x = randn(100000)
julia> y = randn(100000)
julia> # Draw the hexbin plot
julia> hexbin(x, y)
"""
function hexbin(args...; kv...)
create_context(:hexbin, Dict(kv))
plt[].args = plot_args(args)
plot_data()
end
"""
Draw a heatmap.
This function uses the current colormap to display a two-dimensional
array as a heatmap. The array is drawn with its first value in the bottom
left corner, so in some cases it may be neccessary to flip the columns
(see the example below).
By default the function will use the column and row indices for the x- and
y-axes, respectively, so setting the axis limits is recommended. Also note that the
values in the array must lie within the current z-axis limits so it may
be neccessary to adjust these limits or clip the range of array values.
:param data: the heatmap data
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> x = LinRange(-2, 2, 40)
julia> y = LinRange(0, pi, 20)
julia> z = sin.(x') .+ cos.(y)
julia> # Draw the heatmap
julia> heatmap(z)
"""
function heatmap(D, plt=plt[]; kv...)
create_context(:heatmap, Dict(kv))
if ndims(D) == 2
z = D'
width, height = size(z)
plt.args = [(1:width, 1:height, z, nothing, "")]
plot_data()
else
error("expected 2-D array")
end
nothing
end
is_uniformly_spaced(v; tol = 1e-6) =
let dv = diff(v)
maximum(dv) - minimum(dv) < tol * sum(abs.(dv)) / length(dv)
end
function heatmap(x, y, z, plt=plt[]; kv...)
if is_uniformly_spaced(x) && is_uniformly_spaced(y)
create_context(:heatmap, Dict(kv))
else
create_context(:nonuniformheatmap, Dict(kv))
end
if ndims(z) == 2
plt.args = [(x, y, z', nothing, "")]
plot_data()
else
error("expected 2-D array")
end
nothing
end
function polarheatmap(D; kv...)
create_context(:polarheatmap, Dict(kv))
if ndims(D) == 2
z = D'
width, height = size(z)
plt[].args = [(1:width, 1:height, z, nothing, "")]
plot_data()
else
error("expected 2-D array")
end
nothing
end
function polarheatmap(x, y, z, plt=plt[]; kv...)
if is_uniformly_spaced(x) && is_uniformly_spaced(y)
create_context(:polarheatmap, Dict(kv))
else
create_context(:nonuniformpolarheatmap, Dict(kv))
end
if ndims(z) == 2
plt.args = [(x, y, z', nothing, "")]
plot_data()
else
error("expected 2-D array")
end
nothing
end
"""
Draw a three-dimensional wireframe plot.
This function uses the current colormap to display a either a series of
points or a two-dimensional array as a wireframe plot. It can receive one
or more of the following:
- x values, y values and z values, or
- M x values, N y values and z values on a NxM grid, or
- M x values, N y values and a callable to determine z values
If a series of points is passed to this function, their values will be
interpolated on a grid. For grid points outside the convex hull of the
provided points, a value of 0 will be used.
:param args: the data to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example point data
julia> x = 8 .* rand(100) .- 4
julia> y = 8 .* rand(100) .- 4
julia> z = sin.(x) .+ cos.(y)
julia> # Draw the wireframe plot
julia> wireframe(x, y, z)
julia> # Create example grid data
julia> x = LinRange(-2, 2, 40)
julia> y = LinRange(0, pi, 20)
julia> z = sin.(x') .+ cos.(y)
julia> # Draw the wireframe plot
julia> wireframe(x, y, z)
julia> # Draw the wireframe plot using a callable
julia> wireframe(x, y, (x,y) -> sin(x) + cos(y))
"""
function wireframe(args...; kv...)
create_context(:wireframe, Dict(kv))
plt[].args = plot_args(args, fmt=:xyzc)
plot_data()
end
"""
Draw a three-dimensional surface plot.
This function uses the current colormap to display a either a series of
points or a two-dimensional array as a surface plot. It can receive one or
more of the following:
- x values, y values and z values, or
- M x values, N y values and z values on a NxM grid, or
- M x values, N y values and a callable to determine z values
If a series of points is passed to this function, their values will be
interpolated on a grid. For grid points outside the convex hull of the
provided points, a value of 0 will be used.
:param args: the data to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example point data
julia> x = 8 .* rand(100) .- 4
julia> y = 8 .* rand(100) .- 4
julia> z = sin.(x) .+ cos.(y)
julia> # Draw the surface plot
julia> surface(x, y, z)
julia> # Create example grid data
julia> x = LinRange(-2, 2, 40)
julia> y = LinRange(0, pi, 20)
julia> z = sin.(x') .+ cos.(y)
julia> # Draw the surface plot
julia> surface(x, y, z)
julia> # Draw the surface plot using a callable
julia> surface(x, y, (x,y) -> sin(x) + cos(y))
"""
function surface(args...; kv...)
create_context(:surface, Dict(kv))
plt[].args = plot_args(args, fmt=:xyzc)
plot_data()
end
function volume(V, plt=plt[]; kv...)
create_context(:volume, Dict(kv))
plt.args = [(nothing, nothing, nothing, V, "")]
plot_data()
end
"""
Draw one or more three-dimensional line plots.
:param x: the x coordinates to plot
:param y: the y coordinates to plot
:param z: the z coordinates to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> x = LinRange(0, 30, 1000)
julia> y = cos.(x) .* x
julia> z = sin.(x) .* x
julia> # Plot the points
julia> plot3(x, y, z)
"""
function plot3(args...; kv...)
create_context(:plot3, Dict(kv))
plt[].args = plot_args(args, fmt=:xyzc)
plot_data()
end
"""
Draw one or more three-dimensional scatter plots.
Additional to x, y and z values, you can provide values for the markers'
color. Color values will be used in combination with the current colormap.
:param x: the x coordinates to plot
:param y: the y coordinates to plot
:param z: the z coordinates to plot
:param c: the optional color values to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> x = 2 .* rand(100) .- 1
julia> y = 2 .* rand(100) .- 1
julia> z = 2 .* rand(100) .- 1
julia> c = 999 .* rand(100) .+ 1
julia> # Plot the points
julia> scatter3(x, y, z)
julia> # Plot the points with colors
julia> scatter3(x, y, z, c)
"""
function scatter3(args...; kv...)
create_context(:scatter3, Dict(kv))
plt[].args = plot_args(args, fmt=:xyzc)
plot_data()
end
"""
Redraw current plot
This can be used to update the current plot, after setting some
attributes like the title, axes labels, legend, etc.
**Usage examples:**
.. code-block:: julia-repl
julia> # Create example data
julia> x = LinRange(-2, 2, 40)
julia> y = 2 .* x .+ 4
julia> # Add title and labels
julia> title("Example plot")
julia> xlabel("x")
julia> ylabel("y")
julia> # Redraw the plot with the new attributes
julia> redraw()
"""
function redraw(; kv...)
create_context(Dict(kv))
plot_data()
end
"""
Set the plot title.
The plot title is drawn using the extended text function GR.textext.
You can use a subset of LaTeX math syntax, but will need to escape
certain characters, e.g. parentheses. For more information see the
documentation of GR.textext.
:param title: the plot title
**Usage examples:**
.. code-block:: julia
julia> # Set the plot title to "Example Plot"
julia> title("Example Plot")
julia> # Clear the plot title
julia> title("")
"""
function title(s, plt=plt[])
if s != ""
plt.kvs[:title] = s
else
delete!(plt.kvs, :title)
end
s
end
"""
Set the x-axis label.
The axis labels are drawn using the extended text function GR.textext.
You can use a subset of LaTeX math syntax, but will need to escape
certain characters, e.g. parentheses. For more information see the
documentation of GR.textext.
:param x_label: the x-axis label
**Usage examples:**
.. code-block:: julia
julia> # Set the x-axis label to "x"
julia> xlabel("x")
julia> # Clear the x-axis label
julia> xlabel("")
"""
function xlabel(s, plt=plt[])
if s != ""
plt.kvs[:xlabel] = s
else
delete!(plt.kvs, :xlabel)
end
s
end
"""
Set the y-axis label.
The axis labels are drawn using the extended text function GR.textext.
You can use a subset of LaTeX math syntax, but will need to escape
certain characters, e.g. parentheses. For more information see the
documentation of GR.textext.
:param y_label: the y-axis label
"""
function ylabel(s, plt=plt[])
if s != ""
plt.kvs[:ylabel] = s
else
delete!(plt.kvs, :ylabel)
end
s
end
"""
Set the legend of the plot.
The plot legend is drawn using the extended text function GR.textext.
You can use a subset of LaTeX math syntax, but will need to escape
certain characters, e.g. parentheses. For more information see the
documentation of GR.textext.
:param args: The legend strings
**Usage examples:**
.. code-block:: julia
julia> # Set the legends to "a" and "b"
julia> legend("a", "b")
"""
function legend(args::AbstractString...; kv...)
plt[].kvs[:labels] = args
end
"""
Set the limits for the x-axis.
The x-axis limits can either be passed as individual arguments or as a
tuple of (**x_min**, **x_max**). Setting either limit to **nothing** will
cause it to be automatically determined based on the data, which is the
default behavior.
:param x_min:
- the x-axis lower limit, or
- **nothing** to use an automatic lower limit, or
- a tuple of both x-axis limits
:param x_max:
- the x-axis upper limit, or
- **nothing** to use an automatic upper limit, or
- **nothing** if both x-axis limits were passed as first argument
:param adjust: whether or not the limits may be adjusted
**Usage examples:**
.. code-block:: julia
julia> # Set the x-axis limits to -1 and 1
julia> xlim((-1, 1))
julia> # Reset the x-axis limits to be determined automatically
julia> xlim()
julia> # Reset the x-axis upper limit and set the lower limit to 0
julia> xlim((0, nothing))
julia> # Reset the x-axis lower limit and set the upper limit to 1
julia> xlim((nothing, 1))
"""
function xlim(a, plt=plt[])
plt.kvs[:xlim] = a
end
"""
Set the limits for the y-axis.
The y-axis limits can either be passed as individual arguments or as a
tuple of (**y_min**, **y_max**). Setting either limit to **nothing** will
cause it to be automatically determined based on the data, which is the
default behavior.
:param y_min:
- the y-axis lower limit, or
- **nothing** to use an automatic lower limit, or
- a tuple of both y-axis limits
:param y_max:
- the y-axis upper limit, or
- **nothing** to use an automatic upper limit, or
- **nothing** if both y-axis limits were passed as first argument
:param adjust: whether or not the limits may be adjusted
**Usage examples:**
.. code-block:: julia
julia> # Set the y-axis limits to -1 and 1
julia> ylim((-1, 1))
julia> # Reset the y-axis limits to be determined automatically
julia> ylim()
julia> # Reset the y-axis upper limit and set the lower limit to 0
julia> ylim((0, nothing))
julia> # Reset the y-axis lower limit and set the upper limit to 1
julia> ylim((nothing, 1))
"""
function ylim(a, plt=plt[])
plt.kvs[:ylim] = a
end
"""
Save the current figure to a file.
This function draw the current figure using one of GR's workstation types
to create a file of the given name. Which file types are supported depends
on the installed workstation types, but GR usually is built with support
for .png, .jpg, .pdf, .ps, .gif and various other file formats.
:param filename: the filename the figure should be saved to
**Usage examples:**
.. code-block:: julia
julia> # Create a simple plot
julia> x = 1:100
julia> plot(x, 1 ./ (x .+ 1))
julia> # Save the figure to a file
julia> savefig("example.png")
"""
function savefig(filename, plt=plt[]; kv...)
merge!(plt.kvs, Dict(kv))
GR.beginprint(filename)
plot_data(false)
GR.endprint()
end
function meshgrid(vx, vy)
[x for x in vx, y in vy], [y for x in vx, y in vy]
end
function meshgrid(vx, vy, vz)
[x for x in vx, y in vy, z in vz], [y for x in vx, y in vy, z in vz], [z for x in vx, y in vy, z in vz]
end
function peaks(n=49)
x = LinRange(-3, 3, n)
y = LinRange(-3, 3, n)'
3 * (1 .- x).^2 .* exp.(-(x.^2) .- (y.+1).^2) .- 10*(x/5 .- x.^3 .- y.^5) .* exp.(-x.^2 .- y.^2) .- 1/3 * exp.(-(x.+1).^2 .- y.^2)
end
"""
Draw an image.
This function can draw an image either from reading a file or using a
two-dimensional array and the current colormap.
:param image: an image file name or two-dimensional array
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> x = LinRange(-2, 2, 40)
julia> y = LinRange(0, pi, 20)
julia> z = sin.(x') .+ cos.(y)
julia> # Draw an image from a 2d array
julia> imshow(z)
julia> # Draw an image from a file
julia> imshow("example.png")
"""
function imshow(I, plt=plt[]; kv...)
create_context(:imshow, Dict(kv))
plt.args = [(nothing, nothing, nothing, I, "")]
plot_data()
end
"""
Draw an isosurface.
This function can draw an image either from reading a file or using a
two-dimensional array and the current colormap. Values greater than the
isovalue will be seen as outside the isosurface, while values less than
the isovalue will be seen as inside the isosurface.
:param v: the volume data
:param isovalue: the isovalue
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> s = LinRange(-1, 1, 40)
julia> v = 1 .- (s .^ 2 .+ (s .^ 2)' .+ reshape(s,1,1,:) .^ 2) .^ 0.5
julia> # Draw an image from a 2d array
julia> isosurface(v, isovalue=0.2)
"""
function isosurface(V; kv...)
create_context(:isosurface, Dict(kv))
plt[].args = [(nothing, nothing, nothing, V, "")]
plot_data()
end
# Backwards compatability where isovalue can be specified as a second positional argument
isosurface(V, v; kv...) = isosurface(V; isovalue = v, kv...)
function cart2sph(x, y, z)
azimuth = atan.(y, x)
elevation = atan.(z, sqrt.(x.^2 + y.^2))
r = sqrt.(x.^2 + y.^2 + z.^2)
azimuth, elevation, r
end
function sph2cart(azimuth, elevation, r)
x = r .* cos.(elevation) .* cos.(azimuth)
y = r .* cos.(elevation) .* sin.(azimuth)
z = r .* sin.(elevation)
x, y, z
end
"""
Draw one or more polar plots.
This function can receive one or more of the following:
- angle values and radius values, or
- angle values and a callable to determine radius values
:param args: the data to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example data
julia> angles = LinRange(0, 2pi, 40)
julia> radii = LinRange(0, 2, 40)
julia> # Plot angles and radii
julia> polar(angles, radii)
julia> # Plot angles and a callable
julia> polar(angles, r -> cos(r) ^ 2)
"""
function polar(args...; kv...)
create_context(:polar, Dict(kv))
plt[].args = plot_args(args)
plot_data()
end
"""
Draw a triangular surface plot.
This function uses the current colormap to display a series of points
as a triangular surface plot. It will use a Delaunay triangulation to
interpolate the z values between x and y values. If the series of points
is concave, this can lead to interpolation artifacts on the edges of the
plot, as the interpolation may occur in very acute triangles.
:param x: the x coordinates to plot
:param y: the y coordinates to plot
:param z: the z coordinates to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example point data
julia> x = 8 .* rand(100) .- 4
julia> y = 8 .* rand(100) .- 4
julia> z = sin.(x) .+ cos.(y)
julia> # Draw the triangular surface plot
julia> trisurf(x, y, z)
"""
function trisurf(args...; kv...)
create_context(:trisurf, Dict(kv))
plt[].args = plot_args(args, fmt=:xyzc)
plot_data()
end
"""
Draw a triangular contour plot.
This function uses the current colormap to display a series of points
as a triangular contour plot. It will use a Delaunay triangulation to
interpolate the z values between x and y values. If the series of points
is concave, this can lead to interpolation artifacts on the edges of the
plot, as the interpolation may occur in very acute triangles.
:param x: the x coordinates to plot
:param y: the y coordinates to plot
:param z: the z coordinates to plot
**Usage examples:**
.. code-block:: julia
julia> # Create example point data
julia> x = 8 .* rand(100) .- 4
julia> y = 8 .* rand(100) .- 4
julia> z = sin.(x) + cos.(y)
julia> # Draw the triangular contour plot
julia> tricont(x, y, z)
"""
function tricont(args...; kv...)
create_context(:tricont, Dict(kv))
plt[].args = plot_args(args, fmt=:xyzc)
plot_data()
end
function shade(args...; kv...)
create_context(:shade, Dict(kv))
plt[].args = plot_args(args, fmt=:xys)
plot_data()
end
function setpanzoom(x, y, zoom, plt=plt[])
empty!(plt.kvs)
merge!(plt.kvs, ctx)
plt.kvs[:panzoom] = (x, y, zoom)
plot_data()
end
function mainloop(plt=plt[])
server = listen(8001)
try
while true
sock = accept(server)
while isopen(sock)
io = IOBuffer()
write(io, read(sock))
seekstart(io)
obj = deserialize(io)
merge!(plt.kvs, obj["kvs"])
plt.args = obj["args"]
plot_data(false)
end
end
catch
true
end
end
function plot(path::String; kwargs...)
args = [["julia", path]; [string(k, ":", v) for (k, v) in pairs(kwargs)]]
ccall(GR.libGRM_ptr(:grm_plot_from_file),
Nothing,
(Cint, Ptr{Ptr{UInt8}}, ),
length(args), args)
end
end # module
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 12756 | module js
import GR
pxwidth = 640
pxheight = 480
id_count = 0
const js_running = Ref(false)
const checking_js = Ref(false)
const port = Ref(0)
const connected = Ref(false)
const connect_cond = Ref(Condition())
conditions = Dict()
l = ReentrantLock()
mutable struct JSTermWidget
identifier::Int
width::Int
height::Int
disp::Int
visible::Bool
end
function inject_js()
global wss
wss = nothing
gr_js_source = joinpath(ENV["GRDIR"], "lib", "gr.js")
_gr_js = if isfile(gr_js_source)
_gr_js = try
_gr_js = open(gr_js_source) do f
_gr_js = read(f, String)
_gr_js
end
catch e
nothing
end
_gr_js
end
if _gr_js === nothing
error(string("Unable to open '", gr_js_source, "'."))
else
display(HTML(string("""
<script type="text/javascript" id="jsterm-javascript">
WEB_SOCKET_ADDRESS = 'ws://127.0.0.1:""", port[], """';
if (typeof jsterm === 'undefined') {
""", _gr_js, """
jsterm = new JSTerm();
jsterm.connectWs();
}
</script>
""")))
end
end
function check_js()
if !checking_js[]
checking_js[] = true
d = Dict("text/html"=>string("""
<script type="text/javascript">
WEB_SOCKET_ADDRESS = 'ws://127.0.0.1:""", port[], """';
if (typeof JSTerm !== 'undefined') {
if (typeof jsterm === 'undefined') {
jsterm = new JSTerm();
}
jsterm.connectWs();
} else {
ws = new WebSocket('ws://127.0.0.1:""", port[], """');
ws.onopen = function() {
ws.send("inject-js");
ws.close();
}
}
</script>
"""))
transient = Dict("display_id"=>"jsterm_check")
Main.IJulia.send_ipython(Main.IJulia.publish[], Main.IJulia.msg_pub(Main.IJulia.execute_msg, "display_data", Dict("data"=>d, "metadata"=>Dict(), "transient"=>transient)))
end
end
function JSTermWidget(id::Int, width::Int, height::Int, disp::Int)
global id_count
if GR.isijulia() && GR.displayname() == "js-server"
id_count += 1
if !js_running[]
@async check_js()
end
JSTermWidget(id, width, height, disp, false)
else
error("JSTermWidget is only available in IJulia environments")
end
end
wss = nothing
evthandler = Dict{Int32,Any}()
global_evthandler = nothing
function register_evthandler(f::Function, id)
global evthandler, l
if GR.isijulia()
send_command(Dict("command" => "enable_events"), "request", id)
lock(l) do
evthandler[id] = f
end
else
error("register_evthandler is only available in IJulia environments")
end
end
function unregister_evthandler(id)
global evthandler, l
if GR.isijulia()
if global_evthandler === nothing
send_command(Dict("command" => "disable_events"), "request", id)
end
lock(l) do
evthandler[id] = nothing
end
else
error("unregister_evthandler is only available in IJulia environments")
end
end
function register_evthandler(f::Function)
global global_evthandler, l
if GR.isijulia()
send_command(Dict("command" => "enable_events"), "request", nothing)
lock(l) do
evthandler[id] = f
end
else
error("register_evthandler is only available in IJulia environments")
end
end
function unregister_evthandler()
global global_evthandler, evthandler, l
if GR.isijulia()
send_command(Dict("command" => "disable_events"), "request", nothing)
lock(l) do
for key in keys(evthandler)
if evthandler[key] !== nothing
send_command(Dict("command" => "enable_events"), "request", key)
end
end
evthandler[id] = nothing
end
else
error("unregister_evthandler is only available in IJulia environments")
end
end
function send_command(msg, msgtype, id=nothing)
global wss, ws
if GR.isijulia() && GR.displayname() == "js-server"
if id !== nothing
m = merge(msg, Dict("type" => msgtype, "id" => id))
else
m = merge(msg, Dict("type" => msgtype))
end
if !js_running[]
conditions["sendonconnect"] = Condition()
@async check_js()
wait(conditions["sendonconnect"])
end
if ws !== nothing
try
HTTP.write(ws, Array{UInt8}(JSON.json(m)))
catch e
ws = nothing
js_running[] = false
end
end
elseif GR.displayname() == "js-server"
error("'js-server' is only available in IJulia environments.")
else
error(str("Display '", GR.displayname(), "' does not support send_command()."))
end
end
function send_evt(msg, id)
if GR.isijulia()
send_command(msg, "evt", id)
else
error("send_evt is only available in IJulia environments")
end
end
function disable_jseventhandling(id)
if GR.isijulia()
send_command(Dict("command" => "disable_jseventhandling"), "request", id)
else
error("disable_jseventhandling is only available in IJulia environments")
end
end
function enable_jseventhandling(id)
if GR.isijulia()
send_command(Dict("command" => "enable_jseventhandling"), "request", id)
else
error("enable_jseventhandling is only available in IJulia environments")
end
end
function disable_jseventhandling()
if GR.isijulia()
send_command(Dict("command" => "disable_jseventhandling"), "request", nothing)
else
error("disable_jseventhandling is only available in IJulia environments")
end
end
function enable_jseventhandling()
global ws
if GR.isijulia()
send_command(Dict("command" => "enable_jseventhandling"), "request", nothing)
else
error("enable_jseventhandling is only available in IJulia environments")
end
end
function comm_msg_callback(msg)
global conditions, evthandler, l
data = msg
if haskey(data, "type")
if data["type"] == "save"
d = Dict("text/html"=>string("<script type=\"text/javascript\" class=\"jsterm-data-widget\">", data["content"]["data"]["widget_data"], "</script>"))
transient = Dict("display_id"=>string("save_display_", data["display_id"]))
Main.IJulia.send_ipython(Main.IJulia.publish[], Main.IJulia.msg_pub(Main.IJulia.execute_msg, "update_display_data", Dict("data"=>d, "metadata"=>Dict(), "transient"=>transient)))
elseif data["type"] == "evt"
lock(l) do
if haskey(evthandler, data["id"]) && evthandler[data["id"]] !== nothing
evthandler[data["id"]](data["content"])
end
end
elseif data["type"] == "value"
elseif data["type"] == "createDisplay"
d = Dict("text/html"=>"")
transient = Dict("display_id"=>string("save_display_", data["dispid"]))
Main.IJulia.send_ipython(Main.IJulia.publish[], Main.IJulia.msg_pub(Main.IJulia.execute_msg, "display_data", Dict("data"=>d, "metadata"=>Dict(), "transient"=>transient)))
display(HTML(string("<div style=\"display: none;\" id=\"jsterm-display-", data["dispid"], "\">")))
elseif data["type"] == "ack"
notify(conditions[data["dispid"]])
end
end
end
function settooltip(tthtml, ttdata, id)
send_command(Dict("command" => "settooltip", "html" => tthtml, "data" => ttdata), "request", id)
return nothing
end
const pluto_data = Ref("")
const pluto_disp = Ref("")
function get_html()
outp = string("""
<div id="jsterm-display-""", pluto_disp[], """\">
</div>
<script type="text/javascript">
if (typeof jsterm === "undefined") {
var jsterm = null;
}
function run_on_start(data, display) {
if (typeof JSTerm === "undefined") {
setTimeout(function() {run_on_start(data, display)}, 100);
return;
}
if (jsterm === null) {
jsterm = new JSTerm(true);
}
jsterm.draw({
"json": data,
"display": display
})
}
run_on_start('""", pluto_data[], """', '""", pluto_disp[], """');
</script>
""")
if GR.isijulia()
display(HTML(string("""
<script type="text/javascript">
if (typeof JSTerm === "undefined" && document.getElementById('jstermImport') == null) {
let jstermScript = document.createElement("script");
jstermScript.setAttribute("src", \"""", jssource[], """\");
jstermScript.setAttribute("type", "text/javascript")
jstermScript.setAttribute("id", "jstermImport")
document.body.appendChild(jstermScript);
}
</script>
""", outp)))
return
end
if plutoisinit[]
return HTML(outp)
else
return HTML(string("""<script type="text/javascript" src=" """, jssource[], """ "></script>""", outp))
end
end
function jsterm_send(data::String, disp)
global draw_end_condition, ws, conditions
if GR.isijulia() && GR.displayname() == "js-server"
if !js_running[]
conditions["sendonconnect"] = Condition()
@async check_js()
wait(conditions["sendonconnect"])
end
if ws !== nothing
try
conditions[disp] = Condition()
HTTP.write(ws, Array{UInt8}(JSON.json(Dict("json" => data, "type"=>"draw", "display"=>disp))))
wait(conditions[disp])
catch e
ws = nothing
js_running[] = false
end
end
elseif GR.displayname() == "pluto" || GR.displayname() == "js"
pluto_data[] = data
pluto_disp[] = disp
else
error("jsterm_send is only available in IJulia environments and Pluto.jl notebooks")
end
end
function get_prev_plot_id()
send_command(Dict("value"=>"prev_id"), "inq")
end
function set_ref_id(id::Int)
send_command(Dict("id"=>id), "set_ref_id")
end
function recv(name::Cstring, id::Int32, msg::Cstring)
# receives string from C and sends it to JS via Comm
global draw_end_condition
id = string(Base.UUID(rand(UInt128)))
jsterm_send(unsafe_string(msg), id)
return convert(Int32, 1)
end
function send(name::Cstring, id::Int32)
# Dummy function, not in use
return convert(Cstring, "String")
end
const send_c = Ref(C_NULL)
const recv_c = Ref(C_NULL)
const init = Ref(false)
function ws_cb(webs)
global comm_msg_callback, ws, conditions
check = false
while !eof(webs)
data = readavailable(webs)
if length(data) > 0
data = String(data)
if data == "inject-js"
check = true
inject_js()
d = Dict("text/html"=>string(""))
transient = Dict("display_id"=>"jsterm_check")
Main.IJulia.send_ipython(Main.IJulia.publish[], Main.IJulia.msg_pub(Main.IJulia.execute_msg, "update_display_data", Dict("data"=>d, "metadata"=>Dict(), "transient"=>transient)))
checking_js[] = false
elseif data == "js-running"
d = Dict("text/html"=>string(""))
transient = Dict("display_id"=>"jsterm_check")
Main.IJulia.send_ipython(Main.IJulia.publish[], Main.IJulia.msg_pub(Main.IJulia.execute_msg, "update_display_data", Dict("data"=>d, "metadata"=>Dict(), "transient"=>transient)))
ws = webs
js_running[] = true
if haskey(conditions, "sendonconnect")
notify(conditions["sendonconnect"])
end
else
comm_msg_callback(JSON.parse(data))
end
end
end
if !check
ws = nothing
js_running[] = false
@async check_js()
end
end
const plutoisinit = Ref(false)
const jssource = Ref("https://gr-framework.org/downloads/gr-0.73.7.js")
function init_pluto(source=jssource[]::String)
plutoisinit[] = true
return HTML(string("""
<script type="text/javascript" src=" """, source, """ "></script>
"""))
end
function initjs()
if !init[]
init[] = true
send_c[] = @cfunction(send, Cstring, (Cstring, Int32))
recv_c[] = @cfunction(recv, Int32, (Cstring, Int32, Cstring))
@eval js begin
import JSON
end
if haskey(ENV, "GR_JS")
jssource[] = ENV["GR_JS"]
elseif occursin(".post", GR.version())
jssource[] = "https://gr-framework.org/downloads/gr-latest.js"
end
if GR.displayname() == "js-server"
if GR.isijulia()
@eval js begin
import HTTP
import Sockets
end
connect_cond[] = Condition()
connected[] = false
ws_server_task = @async begin
port[], server = Sockets.listenany(8081)
@async HTTP.listen(server=server) do webs
HTTP.WebSockets.upgrade(ws_cb, webs)
end
connected[] = true
notify(connect_cond[])
end
if !connected[]
wait(connect_cond[])
end
else
error("'js-server' is only available in IJulia environments.")
end
end
end
send_c[], recv_c[]
end
precompile(initjs, ())
end # module
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 742 | [
:gr3_geterror
:gr3_init
:gr3_free
:gr3_terminate
:gr3_useframebuffer
:gr3_usecurrentframebuffer
:gr3_getimage
:gr3_export
:gr3_getrenderpathstring
:gr3_drawimage
:gr3_createmesh
:gr3_createindexedmesh
:gr3_drawmesh
:gr3_createheightmapmesh
:gr3_drawheightmap
:gr3_deletemesh
:gr3_setquality
:gr3_clear
:gr3_cameralookat
:gr3_setcameraprojectionparameters
:gr3_setlightdirection
:gr3_drawcylindermesh
:gr3_drawconemesh
:gr3_drawspheremesh
:gr3_drawcubemesh
:gr3_setbackgroundcolor
:gr3_createisosurfacemesh
:gr3_surface
:gr3_setalphamode
:gr3_getalphamode
:gr3_setlightparameters
:gr3_getlightparameters
:gr3_isosurface
:gr_volume
:gr3_createxslicemesh
:gr3_createyslicemesh
:gr3_createzslicemesh
:gr3_setlightsources
:gr3_getlightsources
]
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 2641 | [
:gr_setcallback
:gr_initgr
:gr_opengks
:gr_closegks
:gr_inqdspsize
:gr_openws
:gr_closews
:gr_activatews
:gr_deactivatews
:gr_clearws
:gr_updatews
:gr_polyline
:gr_polymarker
:gr_text
:gr_inqtext
:gr_textx
:gr_inqtextx
:gr_fillarea
:gr_cellarray
:gr_nonuniformcellarray
:gr_polarcellarray
:gr_nonuniformpolarcellarray
:gr_gdp
:gr_path
:gr_spline
:gr_gridit
:gr_setlinetype
:gr_setlinewidth
:gr_setlinecolorind
:gr_setmarkertype
:gr_setmarkersize
:gr_setmarkercolorind
:gr_settextfontprec
:gr_setcharexpan
:gr_setcharspace
:gr_settextcolorind
:gr_setcharheight
:gr_inqcharheight
:gr_setcharup
:gr_settextpath
:gr_settextalign
:gr_setfillintstyle
:gr_setfillstyle
:gr_setfillcolorind
:gr_setcolorrep
:gr_setscale
:gr_inqscale
:gr_setwindow
:gr_inqwindow
:gr_setviewport
:gr_inqviewport
:gr_selntran
:gr_setclip
:gr_setwswindow
:gr_setwsviewport
:gr_createseg
:gr_copysegws
:gr_redrawsegws
:gr_setsegtran
:gr_closeseg
:gr_samplelocator
:gr_emergencyclosegks
:gr_updategks
:gr_setspace
:gr_textext
:gr_inqtextext
:gr_axes
:gr_axeslbl
:gr_grid
:gr_grid3d
:gr_verrorbars
:gr_herrorbars
:gr_polyline3d
:gr_polymarker3d
:gr_axes3d
:gr_settitles3d
:gr_titles3d
:gr_surface
:gr_contour
:gr_contourf
:gr_hexbin
:gr_setcolormap
:gr_colorbar
:gr_inqcolor
:gr_inqcolorfromrgb
:gr_hsvtorgb
:gr_tick
:gr_validaterange
:gr_adjustlimits
:gr_adjustrange
:gr_beginprint
:gr_beginprintext
:gr_endprint
:gr_ndctowc
:gr_wctondc
:gr_wc3towc
:gr_drawrect
:gr_fillrect
:gr_drawarc
:gr_fillarc
:gr_drawpath
:gr_setarrowstyle
:gr_setarrowsize
:gr_drawarrow
:gr_readimage
:gr_drawimage
:gr_importgraphics
:gr_setshadow
:gr_settransparency
:gr_setcoordxform
:gr_begingraphics
:gr_endgraphics
:gr_getgraphics
:gr_drawgraphics
:gr_startlistener
:gr_mathtex
:gr_inqmathtex
:gr_setregenflags
:gr_inqregenflags
:gr_savestate
:gr_restorestate
:gr_selectcontext
:gr_destroycontext
:gr_uselinespec
:gr_delaunay
:gr_interp2
:gr_trisurface
:gr_tricontour
:gr_gradient
:gr_quiver
:gr_reducepoints
:gr_version
:gr_shadepoints
:gr_shadelines
:gr_setcolormapfromrgb
:gr_panzoom
:gr_setborderwidth
:gr_setbordercolorind
:gr_setprojectiontype
:gr_setperspectiveprojection
:gr_setorthographicprojection
:gr_settransformationparameters
:gr_setresamplemethod
:gr_setwindow3d
:gr_setspace3d
:gr_text3d
:gr_inqtext3d
:gr_settextencoding
:gr_inqtextencoding
:gr_loadfont
:gr_inqvpsize
:gr_setpicturesizeforvolume
:gr_inqtransformationparameters
:gr_polygonmesh3d
:gr_setscientificformat
:gr_setresizebehaviour
:gr_inqprojectiontype
:gr_beginselection
:gr_endselection
:gr_moveselection
:gr_setmathfont
:gr_inqmathfont
:gr_setclipregion
:gr_inqclipregion
:gr_axis
:gr_drawaxis
:gr_drawaxes
]
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 106 | [
:grm_open
:grm_send
:grm_send_ref
:grm_recv
:grm_plot
:grm_args_delete
:grm_close
:grm_plot_from_file
]
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1061 |
macro warnpcfail(ex::Expr)
modl = __module__
file = __source__.file === nothing ? "?" : String(__source__.file)
line = __source__.line
quote
$(esc(ex)) || @warn """precompile directive
$($(Expr(:quote, ex)))
failed. Please report an issue in $($modl) (after checking for duplicates) or remove this directive.""" _file=$file _line=$line
end
end
function _precompile_()
ccall(:jl_generating_output, Cint, ()) == 1 || return nothing
@warnpcfail precompile(init,(Bool,))
@warnpcfail precompile(ispluto,())
@warnpcfail precompile(isvscode,())
@warnpcfail precompile(isatom,())
@warnpcfail precompile(get_func_ptr, (Base.RefValue{Ptr{Nothing}},LibGR_Ptrs, Symbol) )
@warnpcfail precompile(get_func_ptr, (Base.RefValue{Ptr{Nothing}},LibGRM_Ptrs, Symbol) )
@warnpcfail precompile(get_func_ptr, (Base.RefValue{Ptr{Nothing}},LibGR3_Ptrs, Symbol) )
@warnpcfail precompile(libGR_ptr, (Symbol,))
@warnpcfail precompile(libGRM_ptr, (Symbol,))
@warnpcfail precompile(libGR3_ptr, (Symbol,))
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 11454 | module GRPreferences
using Preferences
using Artifacts
using TOML
try
import GR_jll
catch err
@error """
import GR_jll failed.
Consider using `GR.GRPreferences.use_jll_binary()` or
`GR.GRPreferences.use_upstream_binary()` to repair.
Importing GR a second time will allow use of these functions.
"""
# We use GR_jll on __init__. We cannot ignore the error.
# Ignoring the error causes issues with precompilation.
rethrow()
end
include("downloader.jl")
const grdir = Ref{Union{Nothing,String}}()
const gksqt = Ref{Union{Nothing,String}}()
const grplot = Ref{Union{Nothing,String}}()
const libGR = Ref{Union{Nothing,String}}()
const libGR3 = Ref{Union{Nothing,String}}()
const libGRM = Ref{Union{Nothing,String}}()
const libGKS = Ref{Union{Nothing,String}}()
const libpath = Ref{Union{Nothing,String}}()
lib_path(grdir::AbstractString, lib::AbstractString) =
if Sys.iswindows()
joinpath(grdir, "bin", lib)
else
joinpath(grdir, "lib", lib)
end
# Default grdir to deps/gr if nothing
lib_path(grdir::Nothing, lib::AbstractString) =
lib_path(joinpath(@__DIR__, "..", "deps", "gr"), lib)
gksqt_path(grdir) =
if Sys.iswindows()
joinpath(grdir, "bin", "gksqt.exe")
elseif Sys.isapple()
joinpath(grdir, "Applications", "gksqt.app", "Contents", "MacOS", "gksqt")
else
joinpath(grdir, "bin", "gksqt")
end
# Default grdir to deps/gr if nothing
gksqt_path(grdir::Nothing) =
gksqt_path(joinpath(@__DIR__, "..", "deps", "gr"))
grplot_path(grdir) =
if Sys.iswindows()
joinpath(grdir, "bin", "grplot.exe")
elseif Sys.isapple()
joinpath(grdir, "Applications", "grplot.app", "Contents", "MacOS", "grplot")
else
joinpath(grdir, "bin", "grplot")
end
# Default grdir to deps/gr if nothing
grplot_path(grdir::Nothing) =
grplot_path(joinpath(@__DIR__, "..", "deps", "gr"))
function __init__()
gr_jll_artifact_dir = GR_jll.artifact_dir
default_binary = haskey(ENV, "GRDIR") &&
ENV["GRDIR"] != gr_jll_artifact_dir ? "system" : "GR_jll"
binary = @load_preference("binary", default_binary)
if binary == "GR_jll"
grdir[] = gr_jll_artifact_dir
gksqt[] = GR_jll.gksqt_path
grplot[] = GR_jll.grplot_path
libGR[] = GR_jll.libGR
libGR3[] = GR_jll.libGR3
libGRM[] = GR_jll.libGRM
libGKS[] = GR_jll.libGKS
# Because GR_jll does not dlopen as of 0.69.1+1, we need to append
# the LIBPATH_list similar to JLLWrappers.@init_library_product
push!(GR_jll.LIBPATH_list, dirname(GR_jll.libGR))
# Recompute LIBPATH similar to JLLWrappers.@generate_init_footer
unique!(GR_jll.LIBPATH_list)
pathsep = GR_jll.JLLWrappers.pathsep
GR_jll.LIBPATH[] = join(vcat(GR_jll.LIBPATH_list, Base.invokelatest(GR_jll.JLLWrappers.get_julia_libpaths))::Vector{String}, pathsep)
libpath[] = GR_jll.LIBPATH[]
ENV["GRDIR"] = grdir[]
elseif binary == "system"
grdir[] = haskey(ENV, "GRDIR") ? ENV["GRDIR"] : @load_preference("grdir")
gksqt[] = gksqt_path(grdir[])
grplot[] = grplot_path(grdir[])
libGR[] = lib_path(grdir[], "libGR")
libGR3[] = lib_path(grdir[], "libGR3")
libGRM[] = lib_path(grdir[], "libGRM")
libGKS[] = lib_path(grdir[], "libGKS")
libpath[] = lib_path(grdir[], "")
ENV["GRDIR"] = grdir[]
else
error("Unknown GR binary: $binary")
end
end
"""
use_system_binary(grdir; export_prefs = false, force = false, override = :depot)
Use the system binaries located at `grdir`.
See `Preferences.set_preferences!` for the `export_prefs` and `force` keywords.
The override keyword can be either:
* :depot, Override GR_jll using the depot Overrides.toml
* :project, Override GR_jll using the project Preferences.toml
* (:depot, :project), Overide GR_jll in both the depot and the project
"""
function use_system_binary(grdir; export_prefs = false, force = false, override = :depot)
try
set_preferences!(
GRPreferences,
"binary" => "system",
"grdir" => grdir,
export_prefs = export_prefs,
force = force
)
catch err
if err isa ArgumentError
throw(ArgumentError("Could not set GR system binary preference. Consider using the `force = true` keyword argument."))
end
end
if override isa Symbol
override = (override,)
end
:depot in override && override_depot(grdir)
:project in override && override_project(grdir; force)
__init__()
@info "Please restart Julia to change the GR binary configuration."
end
"""
use_jll_binary(; export_prefs = false, force = false)
Use GR_jll in the its standard configuration from BinaryBuilder.org.
See `Preferences.set_preferences!` for the `export_prefs` and `force` keywords.
"""
function use_jll_binary(; export_prefs = false, force = false)
try
set_preferences!(
GRPreferences,
"binary" => "GR_jll",
"grdir" => nothing,
export_prefs = export_prefs,
force = force
)
catch err
if err isa ArgumentError
throw(ArgumentError("Could not set GR jll binary preference. Consider using the `force = true` keyword argument."))
end
end
unoverride_depot()
unoverride_project(; force)
__init__()
@info "Please restart Julia to change the GR binary configuration."
end
"""
use_upstream_binary([install_dir]; export_prefs = false, force = false, override = :depot)
Download the binaries from https://github.com/sciapp/gr/ and configure GR to use those.
A directory "gr" will be placed within `install_dir` containing the upstream binaries.
By default install_dir will be `joinpath(pathof(GR), "deps")`.
See `use_system_binary` for details.
"""
function use_upstream_binary(args...; export_prefs = false, force = false, override = :depot)
grdir = Downloader.download(args...; force)
use_system_binary(grdir; export_prefs, force, override)
end
"""
get_overrides_toml_path()
Get the path the depot's Overrides.toml
"""
function get_overrides_toml_path()
overrides_toml_path = joinpath(Artifacts.artifacts_dirs()[1], "Overrides.toml")
end
"""
override_depot([grdir])
Override GR_jll in the DEPOT_PATH[1]/artifacts/Overrides.toml with `grdir`.
"""
function override_depot(grdir = grdir[])
overrides_toml_path = get_overrides_toml_path()
override_dict = if isfile(overrides_toml_path)
TOML.parsefile(overrides_toml_path)
else
Dict{String,Any}()
end
override_dict["d2c73de3-f751-5644-a686-071e5b155ba9"] = Dict("GR" => grdir)
open(overrides_toml_path, "w") do io
TOML.print(io, override_dict)
end
end
"""
unoverride_depot()
Remove the override for GR_jll in DEPOT_PATH[1]/artifats/Overrides.toml
"""
function unoverride_depot()
overrides_toml_path = get_overrides_toml_path()
override_dict = if isfile(overrides_toml_path)
TOML.parsefile(overrides_toml_path)
else
Dict{String,Any}()
end
delete!(override_dict, "d2c73de3-f751-5644-a686-071e5b155ba9")
open(overrides_toml_path, "w") do io
TOML.print(io, override_dict)
end
end
"""
override_project([grdir])
Override individual GR_jll artifacts in the (Local)Preferences.toml of the project.
"""
function override_project(grdir = grdir[]; force = false)
set_preferences!(
Base.UUID("d2c73de3-f751-5644-a686-071e5b155ba9"), # GR_jll
"libGR_path" => lib_path(grdir, "libGR"),
"libGR3_path" => lib_path(grdir, "libGR3"),
"libGRM_path" => lib_path(grdir, "libGRM"),
"libGKS_path" => lib_path(grdir, "libGKS"),
"gksqt_path" => gksqt_path(grdir),
"grplot_path" => grplot_path(grdir);
force
)
end
"""
unoverride_project()
Remove overrides for GR_jll artifacts in the (Local)Preferences.toml of the project.
"""
function unoverride_project(; force = false)
delete_preferences!(
Base.UUID("d2c73de3-f751-5644-a686-071e5b155ba9"), # GR_jll
"libGR_path",
"libGR3_path",
"libGRM_path",
"libGKS_path",
"gksqt_path",
"grplot_path";
force
)
end
"""
diagnostics()
Output diagnostics about preferences and overrides for GR and GR_jll.
"""
function diagnostics()
# GR Preferences
binary = @load_preference("binary")
grdir = @load_preference("grdir")
# GR_jll Preferences
GR_jll_uuid = Base.UUID("d2c73de3-f751-5644-a686-071e5b155ba9")
libGR_path = load_preference(GR_jll_uuid, "libGR_path")
libGR3_path = load_preference(GR_jll_uuid, "libGR3_path")
libGRM_path = load_preference(GR_jll_uuid, "libGRM_path")
libGKS_path = load_preference(GR_jll_uuid, "libGKS_path")
gksqt_path = load_preference(GR_jll_uuid, "gksqt_path")
grplot_path = load_preference(GR_jll_uuid, "grplot_path")
# Override.toml in DEPOT_PATH
overrides_toml_path = get_overrides_toml_path()
override_dict = if isfile(overrides_toml_path)
TOML.parsefile(overrides_toml_path)
else
Dict{String,Any}()
end
gr_jll_override_dict = get(override_dict, string(GR_jll_uuid), Dict{String,Any}())
resolved_grdir = haskey(ENV, "GRDIR") ? ENV["GRDIR"] : grdir
# Output
@info "GRDIR Environment Variable" get(ENV, "GRDIR", missing)
@info "GR Preferences" binary grdir
isnothing(resolved_grdir) ||
@info "resolved_grdir" resolved_grdir isdir(resolved_grdir) isdir.(joinpath.((resolved_grdir,), ("bin", "lib", "include", "fonts")))
@info "GR_jll Preferences" libGR_path libGR3_path libGRM_path libGKS_path gksqt_path grplot_path
@info "GR_jll Overrides.toml" overrides_toml_path isfile(overrides_toml_path) get(gr_jll_override_dict, "GR", nothing)
if(isdefined(@__MODULE__, :GR_jll))
@info "GR_jll" GR_jll.libGR_path GR_jll.libGR3_path GR_jll.libGRM_path GR_jll.libGKS_path GR_jll.gksqt_path GR_jll.grplot_path
else
@info "GR_jll is not loaded"
end
return (;
binary, grdir,
libGR_path, libGR3_path, libGRM_path, libGKS_path, gksqt_path, grplot_path,
overrides_toml_path, gr_jll_override_dict
)
end
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 1840 | using Pkg, GR
LibGit2 = Pkg.GitTools.LibGit2
TOML = Pkg.TOML
Plots_jl = joinpath(mkpath(tempname()), "Plots.jl")
Plots_toml = joinpath(Plots_jl, "Project.toml")
# clone and checkout the latest stable version of Plots
stable = try
rg = first(Pkg.Registry.reachable_registries())
Plots_UUID = first(Pkg.Registry.uuids_from_name(rg, "Plots"))
Plots_PkgEntry = rg.pkgs[Plots_UUID]
Plots_version_info = Pkg.Registry.registry_info(Plots_PkgEntry).version_info
maximum(keys(Plots_version_info))
catch
depot = joinpath(first(DEPOT_PATH), "registries", "General", "P", "Plots", "Versions.toml")
maximum(VersionNumber.(keys(TOML.parse(read(depot, String)))))
end
for i ∈ 1:6
try
global repo = Pkg.GitTools.ensure_clone(stdout, Plots_jl, "https://github.com/JuliaPlots/Plots.jl")
break
catch err
@warn err
sleep(20i)
end
end
@assert isfile(Plots_toml) "spurious network error: clone failed, bailing out"
tag = LibGit2.GitObject(repo, "v$stable")
hash = string(LibGit2.target(tag))
LibGit2.checkout!(repo, hash)
# fake the supported GR version for testing (for `Pkg.develop`)
toml = TOML.parse(read(Plots_toml, String))
toml["compat"]["GR"] = GR.version()
open(Plots_toml, "w") do io
TOML.print(io, toml)
end
Pkg.develop(path=Plots_jl)
Pkg.status(["GR", "Plots"])
# test basic plots creation and bitmap or vector exports
using Plots, Test
prefix = tempname()
@time for i ∈ 1:length(Plots._examples)
i ∈ Plots._backend_skips[:gr] && continue # skip unsupported examples
Plots._examples[i].imports ≡ nothing || continue # skip examples requiring optional test deps
pl = Plots.test_examples(:gr, i; disp = false)
for ext in (".png", ".pdf") # TODO: maybe more ?
fn = string(prefix, i, ext)
Plots.savefig(pl, fn)
@test filesize(fn) > 1_000
end
end
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | code | 6228 | ENV["JULIA_DEBUG"] = "GR"
using Random
using Test
using GR
rng = MersenneTwister(1234)
mutable struct Example
title::AbstractString
code::Expr
end
const _examples = Example[
Example("Simple line plot", quote
x = 0:π/100:2π
y = sin.(x)
plot(x, y)
end),
Example("Scatter plot", quote
x = LinRange(0, 1, 51)
y = x .- x.^2
scatter(x, y)
end),
Example("Colored scatter plot", quote
sz = LinRange(0.5, 3, length(x))
c = LinRange(0, 255, length(x))
scatter(x, y, sz, c)
end),
Example("Stem plot", quote
stem(x, y)
end),
Example("Histogram plot", quote
histogram(randn(rng, 10000))
end),
Example("Multi-line plot", quote
plot(randn(rng, 50))
end),
Example("Overlay plot", quote
oplot(randn(rng, 50, 3))
end),
Example("3-d line plot", quote
x = LinRange(0, 30, 1000)
y = cos.(x) .* x
z = sin.(x) .* x
plot3(x, y, z)
end),
Example("Polar plot", quote
angles = LinRange(0, 2pi, 40)
radii = LinRange(0, 2, 40)
polar(angles, radii)
end),
Example("3-d point plot", quote
x = 2 .* rand(rng, 100) .- 1
y = 2 .* rand(rng, 100) .- 1
z = 2 .* rand(rng, 100) .- 1
scatter3(x, y, z)
end),
Example("Colored 3-d point plot", quote
c = 999 .* rand(rng, 100) .+ 1
scatter3(x, y, z, c)
end),
Example("Hexbin plot", quote
x = randn(rng, 100000)
y = randn(rng, 100000)
hexbin(x, y)
end),
Example("Contour plot", quote
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
contour(x, y, z)
end),
Example("Contour plot of matrix", quote
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
contour(x, y, z)
end),
Example("Filled contour plot", quote
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
contourf(x, y, z)
end),
Example("Filled contour plot of matrix", quote
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
contourf(x, y, z)
end),
Example("Filled contour plot on a triangular mesh", quote
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) + cos.(y)
tricont(x, y, z)
end),
Example("Surface plot", quote
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
surface(x, y, z)
end),
Example("Surface plot of matrix", quote
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
surface(x, y, z)
end),
Example("Surface plot on a triangular mesh", quote
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
trisurf(x, y, z)
end),
Example("Simple surface plot", quote
z = peaks()
surface(z)
end),
Example("Wireframe plot", quote
x = 8 .* rand(rng, 100) .- 4
y = 8 .* rand(rng, 100) .- 4
z = sin.(x) .+ cos.(y)
wireframe(x, y, z)
end),
Example("Wireframe plot of matrix", quote
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
wireframe(x, y, z)
end),
Example("Heatmap plot", quote
x = LinRange(-2, 2, 40)
y = LinRange(0, pi, 20)
z = sin.(x') .+ cos.(y)
heatmap(z)
end),
Example("Image plot", quote
imshow(z)
end),
Example("Polar heatmap plot", quote
ρ = LinRange(0, 7, 200)
θ = LinRange(0, 2π, 360)
polarheatmap(θ, ρ, sin.(2ρ) .* cos.(θ'))
end),
Example("Isosurface plot", quote
s = LinRange(-1, 1, 40)
v = 1 .- (s .^ 2 .+ (s .^ 2)' .+ reshape(s, 1, 1, :) .^ 2) .^ 0.5
isosurface(v, isovalue=0.2)
end),
Example("Volume plot", quote
GR.GR3.terminate()
volume(randn(rng, 50, 50, 50))
end),
Example("Shade points", quote
N = 1_000_000
x = randn(rng, N)
y = randn(rng, N)
shade(x, y)
end),
Example("Discrete plot", quote
xd = -2 .+ 4 * rand(rng, 100)
yd = -2 .+ 4 * rand(rng, 100)
zd = [xd[i] * exp(-xd[i]^2 - yd[i]^2) for i = 1:100]
setprojectiontype(0)
setwsviewport(0, 0.1, 0, 0.1)
setwswindow(0, 1, 0, 1)
setviewport(0.1, 0.95, 0.1, 0.95)
setwindow(-2, 2, -2, 2)
setspace(-0.5, 0.5, 0, 90)
setcolormap(0)
setlinecolorind(1)
setmarkersize(1)
setmarkertype(-1)
setmarkercolorind(1)
setcharheight(0.024)
settextalign(2, 0)
settextfontprec(3, 0)
x, y, z = gridit(xd, yd, zd, 200, 200)
h = -0.5:0.05:0.5
surface(x, y, z, 5)
contour(x, y, h, z, 0)
polymarker(xd, yd)
GR.axes(0.25, 0.25, -2, -2, 2, 2, 0.01)
end)
]
function basic_tests(title; wstype="nul", fig="")
if wstype != "nul"
file_path = tempname() * '.' * wstype
ENV["GKS_WSTYPE"] = wstype
ENV["GKS_FILEPATH"] = file_path
elseif fig == ""
delete!(ENV, "GKS_WSTYPE")
delete!(ENV, "GKS_FILEPATH")
end
ENV["GR3_USE_SR"] = "true"
@info("Testing $(title)")
res = nothing
ok = failed = 0
for ex in _examples
if fig != ""
fn = tempname() * '.' * fig
end
try
clearws()
eval(ex.code)
updatews()
if fig != ""
savefig(fn)
end
res = "\e[32mok\e[0m"
ok += 1
catch e
res = "\e[31mfailed\e[0m"
failed += 1
end
if fig != ""
@test isfile(fn)
@test filesize(fn) > 0
end
@info("Testing plot: $(ex.title) => $res")
end
@info("$ok tests passed. $failed tests failed.")
emergencyclosegks()
if wstype != "nul"
@test isfile(file_path)
@test filesize(file_path) > 0
end
end
@timev basic_tests("interactive graphics")
@timev basic_tests("multi-page PDF", wstype="pdf")
@timev basic_tests("single-page PDF files", fig="pdf")
@timev basic_tests("SVG output", fig="svg")
@timev basic_tests("PNG images", fig="png")
GR.GRPreferences.Downloader.download(pwd(); force = true)
GR.GRPreferences.Downloader.download(joinpath(pwd(), "gr"); force = true)
readdir("gr") .|> println
GR.GRPreferences.Downloader.download(; force = true)
GR.GRPreferences.use_upstream_binary(; force = true)
GR.GRPreferences.diagnostics()
GR.GRPreferences.use_jll_binary(; force = true)
GR.GRPreferences.diagnostics()
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.73.7 | 629693584cef594c3f6f99e76e7a7ad17e60e8d5 | docs | 6488 | # The GR module for Julia
[](LICENSE.md)
[](https://github.com/jheinen/GR.jl/releases)
[](https://pkgs.genieframework.com?packages=GR)
[](https://zenodo.org/badge/latestdoi/29193648)
[](https://mybinder.org/v2/gh/jheinen/GR.jl/master)
[](https://gitter.im/jheinen/GR.jl?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
[](https://juliaci.github.io/NanosoldierReports/pkgeval_badges/G/GR.html)
[](https://github.com/jheinen/GR.jl/actions/workflows/ci.yml)
[](https://app.codecov.io/gh/jheinen/GR.jl)
[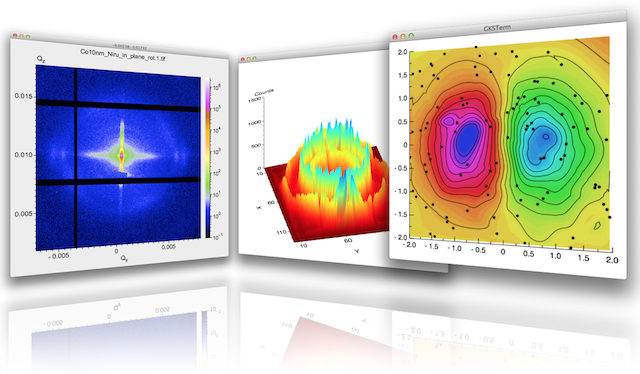](https://gr-framework.org)
This module provides a Julia interface to
[GR](http://gr-framework.org/), a framework for
visualisation applications.
## Installation
From the Julia REPL an up to date version can be installed with:
```julia
julia> using Pkg
julia> Pkg.add("GR")
```
or in the [Pkg REPL-mode](https://docs.julialang.org/en/v1/stdlib/Pkg/index.html#Getting-Started-1):
```julia
pkg> add GR
```
The Julia package manager will download and install a pre-compiled
run-time (for your hardware architecture), if the GR software is not
already installed in the recommended locations.
## Getting started
In Julia simply type ``using GR`` and begin calling functions
in the [GR framework](http://gr-framework.org/julia-gr.html) API.
Let's start with a simple example. We generate 10,000 random numbers and
create a histogram. The histogram function automatically chooses an appropriate
number of bins to cover the range of values in x and show the shape of the
underlying distribution.
```julia
using GR
histogram(randn(10000))
```
## Using GR as backend for Plots.jl
``Plots`` is a powerful wrapper around other Julia visualization
"backends", where ``GR`` seems to be one of the favorite ones.
To get an impression how complex visualizations may become
easier with [Plots](https://juliaplots.github.io), take a look at
[these](https://docs.juliaplots.org/stable/gallery/gr/) examples.
``Plots`` is great on its own, but the real power comes from the ecosystem surrounding it. You can find more information
[here](https://docs.juliaplots.org/latest/ecosystem/).
## Alternatives
Besides ``GR`` and ``Plots`` there is a nice package called [GRUtils](https://github.com/heliosdrm/GRUtils.jl) which provides a user-friendly interface to the low-level ``GR`` subsytem, but in a more "Julian" and modular style. Newcomers are recommended to use this package. A detailed documentation can be found [here](https://heliosdrm.github.io/GRUtils.jl/stable/).
``GR`` and ``GRUtils`` are currently still being developed in parallel - but there are plans to merge the two modules in the future.
## Run-time environment
``GR.jl`` is a wrapper for the ``GR`` Framework. Therefore, the ``GR`` run-time libraries are required to use the software. These are provided via the [GR_jll.jl](https://github.com/JuliaBinaryWrappers/GR_jll.jl) package, which is an autogenerated package constructed using [BinaryBuilder](https://github.com/JuliaPackaging/BinaryBuilder.jl). This is the default setting.
Another alternative is the use of binaries from GR tarballs, which are provided directly by the GR developers as stand-alone distributions for selected platforms - regardless of the programming language. In this case, only one GR runtime environment is required for different language environments (Julia, Python, C/C++), whose installation path can be specified by the environment variable `GRDIR`.
```julia
ENV["JULIA_DEBUG"] = "GR" # Turn on debug statements for the GR package
ENV["GRDIR"] = "<path of you GR installation>" # e.g. "/usr/local/gr"
using GR
```
For more information about setting up a local GR installation, see the [GR Framework](https://gr-framework.org) website.
However, if you want to permanently use your own GR run-time, you have to set the environment variable ``GRDIR`` accordingly before starting Julia, e.g.
- macOS or Linux: ```export GRDIR=/usr/local/gr```
- Windows: ```set GRDIR=C:\gr```
Please note that with the method shown here, `GR_jll` is not imported.
### Switching binaries via GR.GRPreferences
To aid in switching between BinaryBuilder and upstream framework binaries, the `GR.GRPReferences` module implements three methods `use_system_binary()`, `use_upstream_binary()`, and `use_jll_binary()`. These use [Preferences.jl](https://github.com/JuliaPackaging/Preferences.jl) to configure GR.jl
and GR_jll.jl.
To use an existing GR install, invoke `use_system_binary`.
```julia
using GR
GR.GRPreferences.use_system_binary("/path/to/gr"; force = true)
```
To download and switch to [upstream binaries](https://github.com/sciapp/gr/releases) invoke `use_upstream_binary`.
```julia
using GR # repeat this if there is an error
GR.GRPreferences.use_upstream_binary(; force = true)
```
`use_system_binary` and `use_upstream_binary` accept an `override` keyword. This may be set to one of the following:
* `:depot` (default) - Use Overrides.toml in the Julia depot. This normally resides in `.julia/artifacts/Overrides.toml`
* `:project` - Use LocalPreferences.toml. This is usually located near the Project.toml of your active project environment.
* `(:depot, :project)` - Use both of the override mechanisms above.
To switch back to BinaryBuilder binaries supplied with [GR_jll](https://github.com/JuliaBinaryWrappers/GR_jll.jl), invoke `use_jll_binary`:
```julia
using GR # repeat this if there is an error
GR.GRPreferences.use_jll_binary(; force = true)
```
This will reset both the `:depot` and `:project` override mechanisms above.
If you encounter difficulties switching between binaries, the `diagnostics()` function will provide useful information.
Please include this information when asking for assistance.
```julia
using GR
GR.GRPreferences.diagnostics()
```
| GR | https://github.com/jheinen/GR.jl.git |
|
[
"MIT"
] | 0.2.0 | f01266fa4f65a75f737f214cbd7a5e5eb3a00194 | code | 545 | import Dates.AbstractTime
using BrazilCentralBank
using Documenter
DocMeta.setdocmeta!(BrazilCentralBank, :DocTestSetup, :(using BrazilCentralBank); recursive=true)
makedocs(
sitename = "BrazilCentralBank.jl",
format = Documenter.HTML(),
modules = [BrazilCentralBank],
pages = [
"Home" => "index.md",
"Notes on Implementation" => "impl.md",
"API Documentation" => "api_docs.md"
],
doctest = false
)
deploydocs(
repo="github.com/azeredo-e/BrazilCentralBank.jl.git",
push_preview=true
)
| BrazilCentralBank | https://github.com/azeredo-e/BrazilCentralBank.jl.git |
|
[
"MIT"
] | 0.2.0 | f01266fa4f65a75f737f214cbd7a5e5eb3a00194 | code | 446 | """
The BrazilCentralBank package aims to provide a user interface to Brazil's Central Bank (BCB) web data API.
AUTHOR: azeredo-e@github\\
GITHUB: github.com/azeredo-e/BCB.jl\\
LICENSE: MIT License\\
VERSION: 0.1.0
"""
module BrazilCentralBank
include("Currency.jl")
include("Sgs.jl")
export getcurrency_list, getcurrencyseries, Currency,
# Sgs
gettimeseries
greetBCB() = println("Hello, BCB! It\'s Julia!\n")
end # module BCB
| BrazilCentralBank | https://github.com/azeredo-e/BrazilCentralBank.jl.git |
|
[
"MIT"
] | 0.2.0 | f01266fa4f65a75f737f214cbd7a5e5eb3a00194 | code | 15831 |
#* Created by azeredo-e@GitHub
"""
The GetCurrency module is responsible for managing all querys to the BCB FOREX (Foreign Exchange) API
"""
#module GetCurrency
# Changed implementation of modules, previously each file contained a module as defined in the line above,
# changing to files where the module defined in BCB.jl manages everything. May change this in the future to
# use the PatModules package or go back to the previous implementation of each file a module.
import Base.@kwdef
import Dates.AbstractTime
using CSV
using DataFrames
using Dates
using Gumbo
using HTTP
using StringEncodings
#export gettimeseries, getcurrency_list
#TODO: Add a multiple dispatch option to plot
const CACHE = Dict()
const ENCODING = "ISO-8859-1"
#* #-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-
#* STRUCT
#* #-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-
"
Instance of the Currency type.
A `Currency` is an instance of the Currency type attribuited to a specific currency supported by the
BrazilCentralBank.jl API, you can check the list of avaliable currencies with. `getcurrency_list()`.
A `Currency` has many fields that describe not only the information for the coin but also provides methods
applied directly on the instance for retrieving information about the currency. For more notes on the
implementation check the \"Strucs Methods\" section in the documentation.
# Fields
code(<:Integer): Currency code as in `getcurrency_list`.\\
name(<:AbstractString): Name of the currency.\\
symbol(<:AbstractString): ISO three letter currency code.\\
country_code(<:AbstractString): ISO country code.\\
country_name(<:AbstractString): Country name in portuguese.\\
type(<:AbstractString): In ype A currencies, to convert the value to USD divide the currency. In type B\\
you multiply.\\
exclusion_date(<:AbstractTime): Exclusion date of currency. When it was discontinued.
# \"Methods\"
getforex(target::Union{AbstractString, Array{AbstractString}}; kwargs...)
## Args
target(Union{AbstractString, Array{AbstractString}}): ISO code of selected currencies.\\
kwargs: `kwargs` passed to `gettimeseries()`
## Returns
DataFrame: Selected currencies information.
"
@kwdef struct Currency{I<:Integer, F<:Function, S<:AbstractString, D<:Union{AbstractTime, Missing}}
code::I
name::S
symbol::S
country_code::I
country_name::S
type::S
exclusion_date::D
# "Methods"
getcurrencyseries::F = function _getcurrencyseries(
target::Union{AbstractString, Array},
start::Union{AbstractTime, AbstractString, Number},
finish::Union{AbstractTime, AbstractString, Number};
kwargs...
)
if target isa Array
return getcurrencyseries([symbol, target...], start, finish; kwargs...)
else
return getcurrencyseries([symbol, target], start, finish; kwargs...)
end
end
end
#* #-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-
#* FUNCTIONS
#* #-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-
function _currency_url(currency_id, start_date, end_date)
start_date = Date(start_date)
end_date = Date(end_date)
url = "https://ptax.bcb.gov.br/ptax_internet/consultaBoletim.do?"*
"method=gerarCSVFechamentoMoedaNoPeriodo&"*
"ChkMoeda=$currency_id"*
"&DATAINI=$(Dates.format(start_date, "dd/mm/Y"))"*
"&DATAFIM=$(Dates.format(end_date, "dd/mm/Y"))"
return url
end
function _get_currency_id_list()
if haskey(CACHE, :CURRENCY_ID_LIST)
return get(CACHE, :CURRENCY_ID_LIST, missing)
end
url = "https://ptax.bcb.gov.br/ptax_internet/consultaBoletim.do?"*
"method=exibeFormularioConsultaBoletim"
res = HTTP.get(url).body |> String |> parsehtml
xpath_currency_id = children(res.root[2][2][1][3][1][4][2][1])
select_vals = [(select[1].text, getattr(select, "value")) for select in xpath_currency_id]
df = DataFrame(map(idx -> getindex.(select_vals, idx), eachindex(first(select_vals))), [:name, :id])
df.id = parse.(Int32, df.id)
CACHE[:CURRENCY_ID_LIST] = df
return df
end
function _get_current_currency_list(_date, n=0)
url = "http://www4.bcb.gov.br/Download/fechamento/M$(Dates.format(_date, "yyyymmdd")).csv"
consulta() = try
return HTTP.get(url)
catch err
if isa(err, HTTP.Exceptions.ConnectError)
if n >= 3
throw(HTTP.Exceptions.ConnectError(url=url, error="Connection failed"))
end
end
return _get_current_currency_list(_date, n+1)
end
res = consulta()
if res.status == 200
return res
else
return _get_current_currency_list(_date - Day(1), 0)
end
end
function _get_currency_id(symbol::String)
id_list = _get_currency_id_list()
all_currencies = getcurrency_list()
df = innerjoin(id_list, all_currencies, on=:name)
if symbol in df.symbol
return maximum(df[df.symbol .== symbol, :].id)
else
throw(ArgumentError("Symbol not found. Check valid currencies list"))
end
end
function _get_symbol(symbol::String, start_date, end_date)
cid = _get_currency_id(symbol)
url = _currency_url(cid, start_date, end_date)
res = HTTP.get(url)
#For some god forsaken reason, HTTP.jl uses a vector of pairs in res.headers, that's why the weird syntax
if startswith(res.headers[3][2], "text/html")
doc = parsehtml(String(decode(res.body, ENCODING)))
res_msg::String = children(doc.root[2][1])[1].text
res_msg = replace(res_msg, r"^\W+" => "")
res_msg = replace(res_msg, r"^\W+$" => "")
msg = "BCB API returned error: $res_msg - $symbol"
@warn msg
return nothing
end
col_types = Dict(
:Column1 => Date,
:Column2 => Int64,
:Column3 => String,
:Column4 => String,
:Column5 => Float64,
:Column6 => Float64,
:Column7 => Float64,
:Column8 => Float64,
)
df = CSV.read(
IOBuffer(decode(res.body, ENCODING)), DataFrame;
header=false,
delim=';',
decimal=',',
types=col_types,
dateformat="ddmmyyyy"
)
rename!(df,
[:Date,
:aa,
:bb,
:cc,
:bid,
:ask,
:dd,
:ee]
)
#TODO: How the f* do I do a multilayer index in julia?!
#? Answer, I can't, changing approach until they fix this
df_bidask = df[:, [:Date, :bid, :ask]]
rename!(df_bidask,
:bid => "bid_$symbol",
:ask => "ask_$symbol"
)
return df_bidask
end
"""
Currency(code::Integer) -> Currency
A `Currency` is an instance of the Currency type attribuited to a specific currency supported by the
BrazilCentralBank.jl API, you can check the list of avaliable currencies with. `getcurrency_list()`.
A `Currency` has many fields that describe not only the information for the coin but also provides methods
applied directly on the instance for retrieving information about the currency. For more notes on the
implementation check the "Strucs Methods" section in the documentation.
# Args
code(Integer): Code for the currency as is in `getcurrency_list()`.
# Returns
Currency: Desired currency
```
"""
function Currency(code::Integer)
if haskey(CACHE, :CURRENCY_LIST)
df = get(CACHE, :CURRENCY_LIST, missing)
else
df = getcurrency_list()
end
return Currency(
code = df[df.code .== code, 1][1],
name = df[df.code .== code, 2][1],
symbol = df[df.code .== code, 3][1],
country_code = df[df.code .== code, 4][1],
country_name = df[df.code .== code, 5][1],
type = df[df.code .== code, 6][1],
exclusion_date = df[df.code .== code, 7][1]
)
end
"""
Currency(code::String) -> Currency
A `Currency` is an instance of the Currency type attribuited to a specific currency supported by the
BrazilCentralBank.jl API, you can check the list of avaliable currencies with. `getcurrency_list()`.
A `Currency` has many fields that describe not only the information for the coin but also provides methods
applied directly on the instance for retrieving information about the currency. For more notes on the
implementation check the "Strucs Methods" section in the documentation.
# Args:
symbol(String): ISO three letter code for the currency.
# Returns
Currency: Desired currency
"""
function Currency(symbol::String)
if haskey(CACHE, :CURRENCY_LIST)
df = get(CACHE, :CURRENCY_LIST, missing)
else
df = getcurrency_list()
end
#TODO: Include ID
return Currency(
code = df[df.symbol .== symbol, 1][1],
name = df[df.symbol .== symbol, 2][1],
symbol = df[df.symbol .== symbol, 3][1],
country_code = df[df.symbol .== symbol, 4][1],
country_name = df[df.symbol .== symbol, 5][1],
type = df[df.symbol .== symbol, 6][1],
exclusion_date = df[df.symbol .== symbol, 7][1]
)
end
"""
get_currency_list(;convert_to_utf=true) -> DataFrame
List all avaliables currencies in the BCB API, as well as basic information such as currency code,
country of origin, etc.
# Args:
convert_to_utf (Bool, optional): By default BCB information comes in the ISO-8859-1 encoding,
different from the UTF-8 pattern used by Julia. This argument forces the API result to come in UTF-8,
preventing encoding errors. Defaults to true.
# Returns:
DataFrames.DataFrame: DataFrame with all avaliable currencies information.
# Examples:
```jldoctest
julia> getcurrency_list()
273×7 DataFrame
Row │ code name symbol country_code country_name ⋯
│ Int32 String String Int32 String ⋯
─────┼──────────────────────────────────────────────────────────────────────────
1 │ 5 AFEGANE AFEGANIST AFN 132 AFEGANISTAO ⋯
2 │ 785 RANDE/AFRICA SUL ZAR 7560 AFRICA DO SU
3 │ 490 LEK ALBANIA REP ALL 175 ALBANIA, REP
4 │ 610 MARCO ALEMAO DEM 230 ALEMANHA
5 │ 978 EURO EUR 230 ALEMANHA ⋯
6 │ 690 PESETA/ANDORA ADP 370 ANDORRA
7 │ 635 KWANZA/ANGOLA AOA 400 ANGOLA
8 │ 215 DOLAR CARIBE ORIENTAL XCD 418 ANGUILLA
⋮ │ ⋮ ⋮ ⋮ ⋮ ⋱
267 │ 26 BOLIVAR VENEZUELANO VEF 8508 VENEZUELA ⋯
268 │ 260 DONGUE/VIETNAN VND 8583 VIETNA
269 │ 220 DOLAR DOS EUA USD 8630 VIRGENS,ILHA
270 │ 220 DOLAR DOS EUA USD 8664 VIRGENS,ILHA
271 │ 766 QUACHA ZAMBIA ZMW 8907 ZAMBIA ⋯
272 │ 765 QUACHA ZAMBIA ZMK 8907 ZAMBIA
273 │ 217 DOLAR ZIMBABUE ZWL 6653 ZIMBABUE
3 columns and 258 rows omitted
```
"""
function getcurrency_list(;convert_to_utf::Bool=true)
if haskey(CACHE, :CURRENCY_LIST)
return get(CACHE, :CURRENCY_LIST, missing)
end
res = _get_current_currency_list(today())
if convert_to_utf
df = CSV.read(IOBuffer(decode(res.body, ENCODING)), DataFrame)
else
df = CSV.read(IOBuffer(res.body), DataFrame)
end
# nomes_originais = names(df)
rename!(df,
[:code,
:name,
:symbol,
:country_code,
:country_name,
:type,
:exclusion_date]
)
df = subset(df, :country_code => ByRow(!ismissing))
df.symbol = map(x -> strip(x, [' ', '\n', '\t']), df.symbol)
df.name = map(x -> strip(x, [' ', '\n', '\t']), df.name)
df.country_name = map(x -> strip(x, [' ', '\n', '\t']), df.country_name)
df.code = passmissing(convert).(Int32, df.code)
df.name = passmissing(convert).(String, df.name)
df.symbol = passmissing(convert).(String, df.symbol)
df.country_code = passmissing(convert).(Int32, df.country_code)
df.country_name = passmissing(convert).(String, df.country_name)
df.type = passmissing(convert).(String, df.type)
df.exclusion_date = passmissing(x -> Date(x, DateFormat("dd/mm/yyyy"))).(df.exclusion_date)
CACHE[:CURRENCY_LIST] = df
return df
# if english_names
# CACHE["CURRENCY_LIST"] = df
# return df
# else
# rename!(df, nomes_originais)
# CACHE["CURRENCY_LIST"] = df
# return df
# end
end
"""
getcurrencyseries(symbols::Union{String, Array},
start::Any,
finish::Any,
side::String="ask",
groupby::String="symbol")
DataFrame with the time series of selected currencies.
# Args:
symbol (Union{String, Array}): ISO code of desired currencies.\\
start (Union{AbstractTime, AbstractString, Number}): Desired start date. The type are set this way because
it can accept any valid input to Dates.Date().\\
end (Union{AbstractTime, AbstractString, Number}): Desired end date.\\
side (String, optional): Which FOREX prices to return "ask" prices, "side" prices or "both".
Defaults to "ask".\\
groupby (String, optional): In what way the columns are grouped, "symbol" or "side".
# Returns:
DataFrames.DataFrame: DataFrame with foreign currency prices.
# Raises:
ArgumentError: Values passed to `side` or `groupby` are not valid.
# Examples:
```jldoctest
julia> getcurrencyseries("USD", "2023-12-01", "2023-12-10")
6×2 DataFrame
Row │ Date ask_USD
│ Date Float64
─────┼─────────────────────
1 │ 2023-12-01 4.9191
2 │ 2023-12-04 4.9091
3 │ 2023-12-05 4.9522
4 │ 2023-12-06 4.9031
5 │ 2023-12-07 4.8949
6 │ 2023-12-08 4.9158
```
"""
function getcurrencyseries(symbols::Union{String, Array},
start::Union{AbstractTime, AbstractString, Number},
finish::Union{AbstractTime, AbstractString, Number}; #Keyword arguments starts here
side::String="ask",
groupby::String="symbol")
if isa(symbols, String)
symbols = [symbols]
end
dss = []
for symbol ∈ symbols
df_symbol = _get_symbol(symbol, start, finish)
if !isnothing(df_symbol)
push!(dss, df_symbol)
end
end
if length(dss) == 1
df = dss[1]
if side ∈ ("bid", "ask")
return df[:, Regex("Date|$side")]
elseif side == "both"
if groupby == "symbol"
return df
elseif groupby == "side"
return select(df, Regex("$side"), :)
else
thow(ArgumentError("Unknown groupby value, use: symbol, side"))
end
else
thow(ArgumentError("Unknown side value, use: bid, ask, both"))
end
elseif length(dss) > 1
df = innerjoin(dss..., on=:Date)
if side ∈ ("bid", "ask")
return df[:, Regex("Date|$side")]
elseif side == "both"
if groupby == "symbol"
return df
elseif groupby == "side"
return select(df, Regex("$side"), :)
else
thow(ArgumentError("Unknown groupby value, use: symbol, side"))
end
else
thow(ArgumentError("Unknown side value, use: bid, ask, both"))
end
else
return nothing
end
end
#end # GetCurrency module
| BrazilCentralBank | https://github.com/azeredo-e/BrazilCentralBank.jl.git |
|
[
"MIT"
] | 0.2.0 | f01266fa4f65a75f737f214cbd7a5e5eb3a00194 | code | 7738 |
# Created by azeredo-e@GitHub
using Dates
using HTTP
using JSON
using DataFrames
# A função get usa a função _codes para entender o input do usuário
# com isso ela manda para a classe SGSCode para guardar o valor como
# se fosse um struct
#* #-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-
#* STRUCTS
#* #-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-
struct SGSCode{S<:AbstractString, N<:Integer}
name::S
value::N
function SGSCode(code::Union{Integer, AbstractString})
new{AbstractString, Integer}(string(code), convertToInt32(code))
end
function SGSCode(code::Pair)
new{AbstractString, Integer}(code.first, convertToInt32(code.second))
end
#TODO: Add support to named tuples
# function SGSCode(code::Tuple{AbstractString, Number})
# typeof(code[1]) <: AbstractString ?
# new{AbstractString, Number}(string(code[1]), convertToInt32(code[2])) :
# new{AbstractString, Number}(string(code[2]), code[1])
# end
# function SGSCode(code::AbstractArray)
# typeof(code[1]) <: AbstractString ?
# new{AbstractString, Number}(string(code[1]), convertToInt32(code[2])) :
# new{AbstractString, Number}(string(code[2]), convertToInt32(code[1]))
# end
end
#* #-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-
#* FUNCTIONS
#* #-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-#-
function convertToInt32(input)
if typeof(input) <: Number
return Int32(input)
elseif typeof(input) <: AbstractString
return parse(Int32, input)
else
throw(ArgumentError("Input must be a number or string"))
end
end
function _get_url_payload(code, start_date, end_date, last)
payload = Dict(:format => "json")
end_date_exists = @isdefined end_date
if last == 0
if start_date !== nothing || end_date !== nothing
payload[:dataInicial] = Dates.format(Date(start_date), "dd/mm/Y")
end_date = end_date_exists ? end_date : today()
payload[:dataFinal] = Dates.format(Date(end_date), "dd/mm/Y")
end
url = "http://api.bcb.gov.br/dados/serie/bcdata.sgs.$code/dados"
else
url = "http://api.bcb.gov.br/dados/serie/bcdata.sgs.$code/dados/ultimos/$last"
end
return Dict(:payload => payload, :url => url)
end
function _format_df(df, code)
if :datafim in names(df)
rename!(df,
:data => "Date",
:valor => code.name,
:datafim => "enddate"
)
else
rename!(df,
:data => "Date",
:valor => code.name,
)
end
df = select(df, "Date", names(df, Not("Date")))
if "Date" in names(df)
df.Date = passmissing(x -> Date(x, DateFormat("dd/mm/yyyy"))).(df.Date)
end
if "enddate" in names(df)
df.enddate = passmissing(x -> Date(x, DateFormat("dd/mm/yyyy"))).(df.enddate)
end
df[!, code.name] = parse.(Float64, df[:, code.name])
#TODO: Implement frequency, maybe create a type or something
return df
end
"""
gettimeseries(codes; start=nothing, finish=nothing,
last=0, multi=true)
Returns a DataFrame with the SGS time series.
# Args
codes(Integer, AbstractString, Dict{AbstractString, Number}, Tuple{Integer, Vararg{Int}}):\\
The codes for the desired time series.\\
The codes can be in one of the following formats:\\
- `Integer`: time-series code\\
- `Tuple`: tuple containing the desired time-series' codes\\
- `Dict`: Dictionary with the pair ("SeriesName" => code)\\
When using a Dict, you can define a name for the series. This is the name to be used in the column
name, if not defined, it will default to the code.
start(Number, String...): Any value that can be converted to a date with `Date()` is valid.\\
Start date of the series.
end(Number, String...): Any value that can be converted to a date with `Date()` is valid.\\
End date of the series.
last(Integer): If last is bigger than 0, `start` and `end` are ignored. Return the
last *n* values of the series.
multi(Bool): If true, returns a single series with multiple variable, if false,
returns a tuple of single variable series.
# Returns
`DataFrame`: univariate or multivariate time series when `multi=true`.
`Vector{DataFrame}`: vector of univariate time series when `multi=false`.
# Raises
ErrorException: Failed to fetch time-series data.
# Examples
```jldoctest
julia> gettimeseries(1, last=5)
5×2 DataFrame
Row │ Date 1
│ Date Float64
─────┼─────────────────────
1 │ 2024-04-16 5.2635
2 │ 2024-04-17 5.2469
3 │ 2024-04-18 5.2512
4 │ 2024-04-19 5.2269
5 │ 2024-04-22 5.2043
julia> gettimeseries(Dict("USDBRL" => 1), last=5)
5×2 DataFrame
Row │ Date USDBRL
│ Date Float64
─────┼─────────────────────
1 │ 2024-04-15 5.1746
2 │ 2024-04-16 5.2635
3 │ 2024-04-17 5.2469
4 │ 2024-04-18 5.2512
5 │ 2024-04-19 5.2269
julia> gettimeseries(Dict("USDBRL" => 1), start="2021-01-18", finish="2021-01-22")
5×2 DataFrame
Row │ Date USDBRL
│ Date Float64
─────┼─────────────────────
1 │ 2021-01-18 5.2788
2 │ 2021-01-19 5.2945
3 │ 2021-01-20 5.3033
4 │ 2021-01-21 5.3166
5 │ 2021-01-22 5.4301
julia> gettimeseries((1, 433), last=5)
10×3 DataFrame
Row │ Date 1 433
│ Date Float64? Float64?
─────┼──────────────────────────────────────
1 │ 2023-11-01 missing 0.28
2 │ 2023-12-01 missing 0.56
3 │ 2024-01-01 missing 0.42
4 │ 2024-02-01 missing 0.83
5 │ 2024-03-01 missing 0.16
6 │ 2024-04-16 5.2635 missing
7 │ 2024-04-17 5.2469 missing
8 │ 2024-04-18 5.2512 missing
9 │ 2024-04-19 5.2269 missing
10 │ 2024-04-22 5.2043 missing
julia> gettimeseries((1, 433), last=5, multi=false)
2-element Vector{DataFrames.DataFrame}:
5×2 DataFrame
Row │ Date 1
│ Date Float64
─────┼─────────────────────
1 │ 2024-04-16 5.2635
2 │ 2024-04-17 5.2469
3 │ 2024-04-18 5.2512
4 │ 2024-04-19 5.2269
5 │ 2024-04-22 5.2043
5×2 DataFrame
Row │ Date 433
│ Date Float64
─────┼─────────────────────
1 │ 2023-11-01 0.28
2 │ 2023-12-01 0.56
3 │ 2024-01-01 0.42
4 │ 2024-02-01 0.83
5 │ 2024-03-01 0.16
```
"""
function gettimeseries(codes; start=nothing, finish=nothing,
last=0, multi=true)
dfs::Vector{DataFrame} = []
if typeof(codes) <: AbstractString
codes = [codes]
end
for code in (SGSCode(i) for i in codes)
urd = _get_url_payload(code.value, start, finish, last)
res = HTTP.get(urd[:url]; query=urd[:payload])
if res.status != 200
throw(ErrorException("Download error: code = $(code.value)"))
end
try
df = res.body |> String |> JSON.parse |> DataFrame
df = _format_df(df, code)
push!(dfs, df)
catch err
if err isa ErrorException
@warn "Invalid time series code, code = $(code.value)"
end
end
end
if length(dfs) == 0
return nothing
elseif length(dfs) == 1
return dfs[1]
else
if multi
df_join = outerjoin(dfs..., on=:Date)
sort!(df_join, :Date)
return df_join
else
return dfs
end
end
end
| BrazilCentralBank | https://github.com/azeredo-e/BrazilCentralBank.jl.git |
|
[
"MIT"
] | 0.2.0 | f01266fa4f65a75f737f214cbd7a5e5eb3a00194 | code | 2820 | include("../src/BrazilCentralBank.jl")
using .BrazilCentralBank
using DataFrames
using Dates
using Test
BrazilCentralBank.greetBCB()
#For Ver. 0.2.0
function test_series_code()
code = BrazilCentralBank.SGSCode(1)
@assert code.name == "1"
@assert code.value == 1
code = BrazilCentralBank.SGSCode("name" => 1)
@assert code.name == "name"
@assert code.value == 1
return true
end
function test_series_code_iter(codes)
i = 0
for code in (BrazilCentralBank.SGSCode(i) for i in codes)
@assert code.name == "$(i+1)"
@assert code.value == i+1
i += 1
end
@assert length(codes) == i
return true
end
function test_gettimeseries()
x = gettimeseries(1, last=5)
println(x)
@assert x isa DataFrame
@assert names(x) == ["Date", "1"]
@assert nrow(x) == 5
x = gettimeseries(Dict("USDBRL" => 1), last=5)
println(x)
@assert x isa DataFrame
@assert names(x) == ["Date", "USDBRL"]
@assert nrow(x) == 5
x = gettimeseries(Dict("USDBRL" => 1), start="2021-01-18", finish="2021-01-22")
println(x)
@assert x isa DataFrame
@assert names(x) == ["Date", "USDBRL"]
@assert nrow(x) == 5
@assert x[1, "Date"] == Date("2021-01-18")
@assert x[end, "Date"] == Date("2021-01-22")
x = gettimeseries((1, 433), last=5)
println(x)
@assert x isa DataFrame
@assert names(x) |> length == 3
x = gettimeseries((1, 433), last=5, multi=false)
println(x)
@assert x isa Array{DataFrame}
# Invalid series value test
gettimeseries(2, last=5)
return true
end
@testset begin
# Ver. 0.1.0
@test BrazilCentralBank._get_currency_id("USD") == 61
@test getcurrency_list() isa DataFrame
@test BrazilCentralBank.CACHE[:CURRENCY_LIST] isa DataFrame
@test getcurrencyseries("USD", 2023, 2024; side="both") isa DataFrame
@test getcurrencyseries(["USD", "CHF"], Date(2023), Date(2024)) isa DataFrame
try
df = getcurrencyseries("test", Date(2020, 12, 01), Date(2020, 12, 05))
catch err
@test err isa ArgumentError
end
# Ver. 0.1.1
@test Currency("EUR") isa BrazilCentralBank.Currency
EUR = Currency(978)
@test EUR isa BrazilCentralBank.Currency
@test EUR.symbol == "EUR"
@test EUR.getcurrencyseries("USD", Date(2020, 12, 01), Date(2020, 12, 05)) isa DataFrame
@test EUR.getcurrencyseries(["USD", "CHF"], Date(2020, 12, 01), Date(2020, 12, 05)) isa DataFrame
@test EUR.getcurrencyseries(["USD", "CHF"], Date(2020, 12, 01), Date(2020, 12, 05), groupby="symbol") isa DataFrame
# Ver. 0.2.0
@test test_series_code()
@test test_series_code_iter((1, 2))
@test test_series_code_iter([1, 2])
@test test_series_code_iter(Dict("1" => 1, "2" => 2))
@test test_gettimeseries()
end
| BrazilCentralBank | https://github.com/azeredo-e/BrazilCentralBank.jl.git |
|
[
"MIT"
] | 0.2.0 | f01266fa4f65a75f737f214cbd7a5e5eb3a00194 | docs | 1042 | # CHANGELOG
## Ver. 0.1.0 (First release!) - 2024-03-14
### Release Highlights
Creation of the foreign currency API. Implementation of two function `getcurrency_list` e `gettimeseries`, the first returns a dataframe of all avaliable currencies in the BCB's data API, and the second returns a time series in a dataframe format of foreign exchange prices between any currencies avaliable.
## Ver. 0.1.1 - 2024-03-25
## Release Highlights
Creation of the Currency type.
## Bug fixes
- Fixed bug where country name would come with trailing white spaces when using `getcurrency_list`.
## Breaking Changes
None.
### Future breaks
In version 0.2.0 the `gettimeseries` function will be renamed to `getcurrencyseries`.
## Ver. 0.2.0 - 2024-04-23
## Release highlights
Creation of the SGS module, giving the API access to the time series database of the Brazilian Central Bank.
## Breaking changes
- `gettimeseries` from the currency module renamed to `getcurrencyseries`
- `getCurrency` from the currency module renamed to `Currency`
| BrazilCentralBank | https://github.com/azeredo-e/BrazilCentralBank.jl.git |
|
[
"MIT"
] | 0.2.0 | f01266fa4f65a75f737f214cbd7a5e5eb3a00194 | docs | 1414 | # BrazilCentralBank.jl
BrazilCentralBank.jl é uma interface na linguagem de programação Julia para a API de dados do Banco Central do Brasil.
O projeto foi inspirado por [python-bcb](https://github.com/wilsonfreitas/python-bcb) criado por wilsonfreitas@GitHub.
BrazilCentralBank.jl is an interface in the Julia programming language for the data API of Brazil's Central Bank (BCB).
The project was inspired by [python-bcb](https://github.com/wilsonfreitas/python-bcb) created by wilsonfreitas@GitHub.
## About the project
> Current release: 0.2.0 (2024-04-23)
Based on the python-bcb package for python, BrazilCentralBank.jl aims to provide the same level of features to the Julia Language. Currently the package can interact with the foreign exchange (FOREX) prices as shows in the time series availiable in the Brazil's Central Bank (BCB) website.
Right now the project is in its early days and the implementation still resembles a lot the original python project. Future updates include the addition other data sources from the BCB's site such as interest rates, inflation, etc.
The final goal is for this to be a comprehensive set of tools for anyone trying to analyse the brazilian economy using Julia!
## Instalation
Source code are avaliable in this project directory, but instalation through Julia's package manager is also avaliable.
```julia
julia> using Pkg; Pkg.add(BrazilCentralBank)
```
| BrazilCentralBank | https://github.com/azeredo-e/BrazilCentralBank.jl.git |
|
[
"MIT"
] | 0.2.0 | f01266fa4f65a75f737f214cbd7a5e5eb3a00194 | docs | 420 | # API Documentation
```@docs
BrazilCentralBank
getcurrency_list(;convert_to_utf=true)
Currency
Currency(code::Integer)
Currency(code::String)
getcurrencyseries(symbols::Union{String, Array}, start::Union{AbstractTime, AbstractString, Number}, finish::Union{AbstractTime, AbstractString, Number}; side::String="side", groupby::String="symbol")
gettimeseries(codes; start=nothing, finish=nothing, last=0, multi=true)
```
| BrazilCentralBank | https://github.com/azeredo-e/BrazilCentralBank.jl.git |
|
[
"MIT"
] | 0.2.0 | f01266fa4f65a75f737f214cbd7a5e5eb3a00194 | docs | 5995 | # Notes in implementation
This page is a more in depth look into how some concepts were implemetes in the package. Although most of it is relatively straight forward for anyone with some familiarity with Julia, some parts may own a deeper explanation.
## Struct Methods
### Personal ramblings before content
Julia is not a OO language in the same way that Ruby, Python, or even C++ is. Although some form of concepts such as polymorphism or inheritance are integrated in the language, they do not behave in the same way an obejct oriented language would.
Another great example is how Julia opted in for multiple dispatch where methods are global and don't belong to a single class (or, in this case, a struct), contrary to languages like Python where a class methods exists and you can implement a method of class A in class B.
Those are different paradigms and that is fine.
However, as programmers, we have preferences, and I prefer to keep my option to what method to use just a dot away. In this section I will explain how it was done for anyone interested in an explanation (even though is a relatively simple one).
### "Struct-method"
Throught this package there are instances of sructs with methods directly associated with them. The functions they are correlated to were declared directly inside the struct. Doing so two things happen: 1) functions declared in this manner are accessible outside of the struct, you can call the method while referencing an instance of the struct, but never the method on it's own because the method is not defined in the global scope an `UndefVarError` will be thrown at runtime; 2) the "struct-method", as I will call it henceforth, will have access to all arguments of the struct as if they were local variables. Both of this topics will be explored, but first lets look at how a struct with methods is created
```julia
julia> import Base.@kwdef
julia> @kwdef struct St_with_anon_func
... #Any number of properties can be put here
f = x -> x + 1
end
```
There's a lot to unpack here, so lets go line by line.
In the first line we call `import Base.@kwdef`, that allow us to use the `@kwdef` macro. The docstring for this macro is as follows:
> This is a helper macro that automatically defines a keyword-based constructor for the type declared in the expression typedef, which must be a struct or mutable struct expression. The default argument is supplied by declaring fields of the form field::T = default or field = default. If no default is provided then the keyword argument becomes a required keyword argument in the resulting type constructor.
Meaning, we can have deafult value in structs when this macro is used. The importance of default values is that it allows us to apply to one of our properties of the struct a default value of a function as we will see next.
!!! note "Unexpected behaviour"
The current version of `BrazilCentralBank` doesn't implement an inner-constructor for its structs. If you are planning on using `@kwdef` it on your projects know that some unexpected behavious may occur as well as some other not well defined behaviour when using this macro. For a more complete version check `@with_kw` from Parameters.jl
In the second line we call the `@kwdef` macro to our struct, and in the third line we see its effect. There we define the property such that `St().f` exists, but not only that, because of the keyword def macro we may assign to it a value, in this case a function, an anonynmous function. We can see its use in the following:
```julia
julia> st = St(...) #Any number of arguments can be passed here
julia> st.f(1)
2
```
The function `f` is a member of the struct `St` and it's instances, and because of that it is not accessible from outside of it. For example the first example works, but the second returns an error:
```julia
julia> st.f(1)
2
julia> f(1)
# ERROR: UndefVarError: `f` not defined
```
This behaviour can be quite useful in handling which method to be used. This approach is different from Julia's standard multiple dispatch approach (a system that, I must say, is excellent), and is probably a bit frowned upon in the Julia community. Regardless, I think is the best way for an end user to know what tools are avaliable to them, by listing every single one with just the touch of a dot.
However, in respect to Julia's core philosophy of multiple dispatch, both option are avaliable. You can call a method directly from a struct or as a function imported from the module directly as so:
```julia
julia> using BrazilCentralBank
julia> USD = getCurrency("USD")
julia> USD.getforexseries("EUR")
# OUTPUT
julia> gettimeseries(["USD", "EUR"]) # As of version 0.2.0 the gettimeseries function will be renamed to getforexseries
# OUTPUT
```
Throught this explanation I've used anonymous functions for when binding the struct value to a function, however is also possible to use a named function written outside of the declaration of the struct.
```julia
julia> import Base.@kwdef
julia> _f(x) = x + 1
julia> @kwdef struct St_with_out_func
... #Any number of properties can be put here
f = x -> _f(x)
end
```
Note that you still need to call it as a anonymous function. If it's not done, Julia will thrown an error because it will treat the declaration inside the struct `f = _f(x)` as f being equal to the result of `x` being applied to function `_f`, and because `x` is not defined an `UndefVarError` will be thrown.
An alternative to escape the anonymous functions declaration is declaring the function inside the body of the struct as such:
```julia
julia> import Base.@kwdef
julia> _f(x) = x + 1
julia> @kwdef struct St_with_inner_func
... #Any number of properties can be put here
f = function f(x)
return x + 1
end
end
```
The above implementation is the one chosen for the BrazilCentralBank package.
### Performance tests
W.I.P.
| BrazilCentralBank | https://github.com/azeredo-e/BrazilCentralBank.jl.git |
|
[
"MIT"
] | 0.2.0 | f01266fa4f65a75f737f214cbd7a5e5eb3a00194 | docs | 981 | # BrazilCentralBank.jl Documentation
Welcome to the documentation page!
## About the Project
> Current release: 0.2.0 (2024-04-23)
Based on the python-bcb package for python, BrazilCentralBank.jl aims to provide the same level of features to the Julia Language. Currently the package can interact with the foreign exchange (FOREX) prices as shows in the time series availiable in the Brazil's Central Bank (BCB) website.
Right now the project is in its early days and the implementation still resembles a lot the original python project. Future updates include the addition other data sources from the BCB's site such as interest rates, inflation, etc.
The final goal is for this to be a comprehensive set of tools for anyone trying to analyse the brazilian economy using Julia!
## Instalation
Source code are avaliable in this project directory, but instalation through Julia's package manager is also avaliable.
```julia
julia> using Pkg; Pkg.add(BrazilCentralBank)
```
| BrazilCentralBank | https://github.com/azeredo-e/BrazilCentralBank.jl.git |